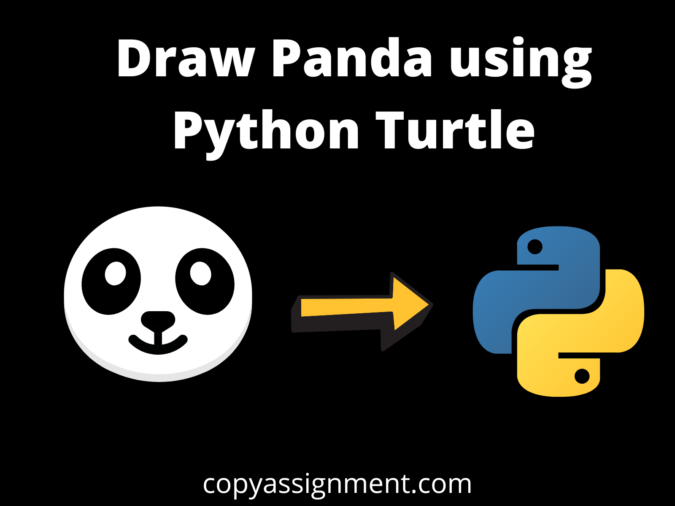
Introduction
We’re working on a project in which we’ll learn how to Draw Panda using Python Turtle library. In this section, we will divide the code and explain how to make a panda’s face using functions and methods.
Drawing a panda can be difficult if you’re new to Python, but don’t worry, I’ll explain everything and provide you with the code for this application.
Our website contains additional articles about the python turtle and its projects. You can use our website’s search box or go to our Home page to find what you’re looking for.
Step 1: Here we are just importing the libraries.
from turtle import *
import turtle
Step 2: Creating an object for this turtle.
t = turtle.Turtle()
turtle.Screen().bgcolor("yellow")
#Bg color is use to set the background color
Now we are using the function to create a Face. For the Creation of a Face, we have to use the following functions.
Fillcolor(): Choose the fill color by calling fillcolor() function
Begin_fill(): This method is used to call just before drawing a shape to be filled.
End_fill(): This method is used to Fill the shape drawn after the call begin_fill().
Setpos(): can be used to set a position for the turtle.
Step 3: Declare a function to draw a circle
def circles(color, radius):
# Set the fill
t.fillcolor(color)
# Start filling the color
t.begin_fill()
# Draw a circle
t.circle(radius)
# Ending the filling of the color
t.end_fill()
Step 4: Creating ears with the black color
# Draw first ear
t.up()
t.setpos(-35, 95)
t.down
circles('black', 15)
# Draw second ear
t.up()
t.setpos(35, 95)
t.down()
circles('black', 15)
Step 5: Create panda’s face with white color
#-------Draw face-------#
t.up()
t.setpos(0, 35)
t.down()
circles('white', 40)
Step 6: Draw the eyes of Panda
# Draw first eye
t.up()
t.setpos(-18, 75)
t.down
circles('black', 8)
# Draw second eye
t.up()
t.setpos(18, 75)
t.down()
circles('black', 8)
Step 7: Draw the eyes of Panda with black and white color concentric circles.
# Draw first eye
t.up()
t.setpos(-18, 77)
t.down()
circles(' white ', 4)
# Draw second eye
t.up()
t.setpos(18, 77)
t.down()
circles(' white ', 4)
Step 8: Drawing the nose of a panda
#-------Draw nose-------#
t.up()
t.setpos(0, 55)
t.down
circles('black', 5)
Step 9: Creating a panda’s mouth
#-------Draw mouth-------#
t.setpos(0, 55)
t.down()
t.right(90)
t.circle(5, 180)
t.up()
t.setpos(0, 55)
t.down()
t.left(360)
t.circle(5, -180)
t.hideturtle()
Complete Source Code to Draw Panda using Python Turtle :
import turtle t = turtle.Turtle() turtle.Screen().bgcolor("yellow") def circles(color, radius): # Set the fill t.fillcolor(color) # Start filling the color t.begin_fill() # Draw a circle t.circle(radius) # Ending the filling of the color t.end_fill() # Draw first ear t.up() t.setpos(-35, 95) t.down circles('black', 15) # Draw second ear t.up() t.setpos(35, 95) t.down() circles('black', 15) #....... Draw face ......# t.up() t.setpos(0, 35) t.down() circles('white', 40) #-------Draw eyes black-------# # Draw first eye t.up() t.setpos(-18, 75) t.down circles('black', 8) # Draw second eye t.up() t.setpos(18, 75) t.down() circles('black', 8) #-------Draw eyes white-------# # Draw first eye t.up() t.setpos(-18, 77) t.down() circles('white', 4) # Draw second eye t.up() t.setpos(18, 77) t.down() circles('white', 4) #-------Draw nose-------# t.up() t.setpos(0, 55) t.down circles('black', 5) #-------Draw mouth-------# t.setpos(0, 55) t.down() t.right(90) t.circle(5, 180) t.up() t.setpos(0, 55) t.down() t.left(360) t.circle(5, -180) t.hideturtle()
Output :
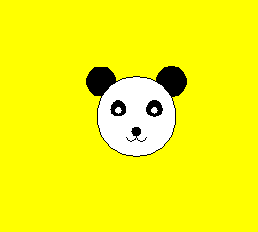
As you can see, we successfully made an adorable panda face using a python turtle. I hope you were able to complete this program successfully.
If you’re looking for more amazing turtle tutorials like this one, check out this: Python Turtle Codes.
If you don’t want to create all of the files and folders, you can run this program now using this online turtle compiler, which is quick and simple.
Also Read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Wishing Happy New Year 2023 in Python Turtle
- Snake and Ladder Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods
- Happy Birthday Python Program In Turtle
- I Love You Program In Python Turtle
- Draw Python Logo in Python Turtle
- Space Invaders game using Python
- Draw Google Drive Logo Using Python
- Draw Instagram Reel Logo Using Python
- Draw The Spotify Logo in Python Turtle
- Draw The CRED Logo Using Python Turtle
- Draw Javascript Logo using Python Turtle
- Draw Dell Logo using Python Turtle
- Draw Spider web using Python Turtle