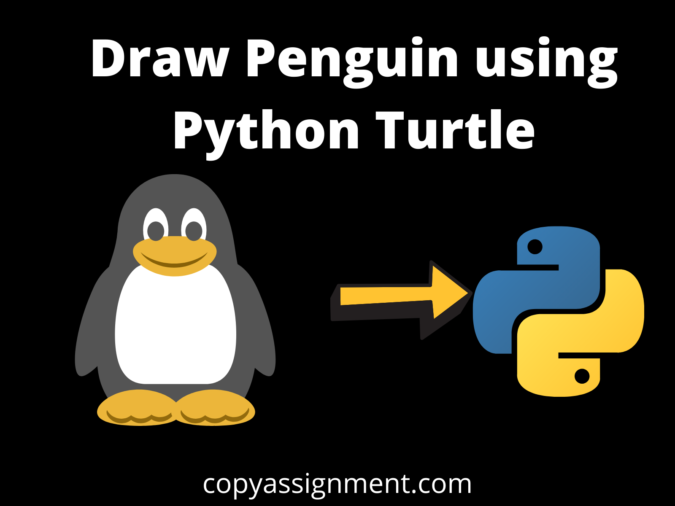
Introduction
Hello my dear Friends, hope all of you are fine. Today we have come up with a simple and easy program of python turtle i.e. to Draw Penguin using Python Turtle. That is the penguin which is drawn by anyone who understands the basics of the python Turtle.
Here we have provided very simple lines of code. Each line of code is easily understandable to draw a particular object
You can check our website for more articles related to python turtle and its projects.
If you want only code, please go to the bottom of the page and copy the code from there using the copy button. So let’s get started.
1. Import turtle
Import turtle
The import function in this allows us to import the turtle package. It contains some inbuilt methods and functionalities which help in the proper functioning of a turtle. It helps us to draw anything using a python turtle.
2. Creating Turtle Object
pen=turtle.Turtle() pen.setheading(270)
In this part, we have created the turtle object i.e pen. With the help of this object turtle, we can access all the inbuilt functions of the turtle. In this part, we have also set the orientation of the turtle to the angle of 270
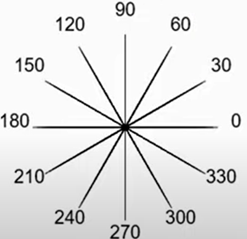
3. Creating the body of the penguin
pen.begin_fill() pen.circle(50,180) pen.forward(80) pen.circle(50,180) pen.forward(80) pen.end_fill()
In this part, we have started to begin_fill function which fills the body color with black. pen. The circle (50,180) function allows us to draw 2 half semicircles of radius 50 with an angle of 180 degrees. The forward(80) function allows the turtle to move 80 steps forward.
4. Creating the Belly of Penguin
pen.fillcolor("white") pen.goto(10,0) pen.begin_fill() pen.circle(40) pen.end_fill()
The belly of the penguin is filled with white color as we set the fill color to white. The goto(10,0) function allows the turtle to move 10 steps forward from the x-axis. draw a circle of radius 40 filled with white.
5 Creating white eyes of a penguin
pen.setheading(0) pen.goto(30,80) pen.begin_fill() pen.circle(20) pen.end_fill() pen.goto(70,80) pen.begin_fill() pen.circle(20) pen.end_fill()
In this part of the code, we have created 2 eyes of a penguin. Again we have set the angle of the turtle to 0. the turtle is set at specified coordinates.
Drawn the left circle with a radius of 20. similar to the right eye .goto(70,80) turtle moved 70 steps to the x-axis and 80 to the y-axis to draw the right circle.
5. Creating the inner black dot of the eye
pen.shape("circle") pen.fillcolor("black") pen.penup() pen.goto(30,100) pen.stamp() pen.goto(70,100) pen.stamp()
This block of code makes the turtle draw the shape of a circle. Put the shape in the stamp format at the specified coordinates.
6 Making the nose of a penguin
pen.shape("triangle") pen.fillcolor("orange") pen.goto(50,70) pen.setheading(270) pen.stamp()
Here we have made the nose shape to triangle filled with orange color. We have set the heading position to 270 degrees to draw the nose at coordinates (50,70).
7. Drawing hands of penguin
pen.fillcolor("black") pen.pencolor("white") pen.setheading(0) pen.goto(0,50) pen.stamp() pen.setheading(180) pen.goto(100,50) pen.stamp()
In this code we have created the hands of the penguin making the fill color black and the pen color white, we have set the heading position to 0 and 180 degrees so the triangle points accordingly.
8. Drawing the legs of the penguin
pen.shape("square") pen.fillcolor("orange") pen.goto(35,-50) pen.stamp() pen.goto(65,-50) pen.stamp() turtle.done()
This part of the code draws the penguin legs filled with orange color. Setting the coordinates accordingly i.e goto(35,-50) and goto(65,-50) . This results in the x coordinate changed and y will remain the same.
Complete Code to Draw Penguin using Python Turtle
#Import turtle import turtle #Setting the turtle object pen=turtle.Turtle() pen.speed(1) #Setting the headposition of turtle at angle 270 degree pen.setheading(270) pen.begin_fill() #draws the penguin body pen.circle(50,180) pen.forward(80) pen.circle(50,180) pen.forward(80) pen.end_fill() #Belly of penguin pen.fillcolor("white") pen.goto(10,0) pen.begin_fill() pen.circle(40) pen.end_fill() #Eyes of penguin pen.setheading(0) pen.goto(30,80) pen.begin_fill() pen.circle(20) pen.end_fill() pen.goto(70,80) pen.begin_fill() pen.circle(20) pen.end_fill() # Eye dot of penguins eye pen.shape("circle") pen.fillcolor("black") pen.penup() pen.goto(30,100) pen.stamp() pen.goto(70,100) pen.stamp() #Nose of penguin pen.shape("triangle") pen.fillcolor("orange") pen.goto(50,70) pen.setheading(270) pen.stamp() #Hands of penguin pen.fillcolor("black") pen.pencolor("white") pen.setheading(180) pen.goto(0,50) pen.stamp() pen.setheading(0) pen.goto(100,50) pen.stamp() #legs of penguin pen.shape("square") pen.fillcolor("orange") pen.goto(35,-50) pen.stamp() pen.goto(65,-50) pen.stamp() turtle.done()
Output

Thank you for reading this article.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Radha Krishna using Python Turtle
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Drawing letter A using Python Turtle
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Wishing Happy New Year 2023 in Python Turtle
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Draw Goku in Python Turtle
- Medical Store Management System Project in Python
- Draw Mickey Mouse in Python Turtle
- Creating Dino Game in Python
- Tic Tac Toe Game in Python