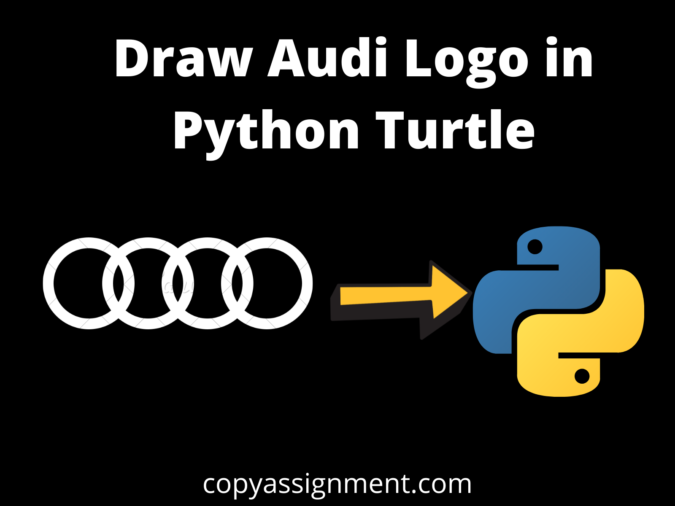
Introduction
Hello and welcome to violet-cat-415996.hostingersite.com. In this post, we’ll look at how to Draw Audi Logo in Python Turtle. This will be a very short but interesting article, especially for newcomers, because we have commented on every line of code so that even a beginner can comprehend the concept.
Let’s start
1. You’ll use the turtle module, which you must import, to create a Turtle().
import turtle
vr = turtle.Turtle()
2. After you’ve adjusted the pensize to make the lines thicker and the constant radius, you can begin drawing the rings.
vr.pensize(7)
turtle.Screen().bgcolor("black")
#Bg color is use to set the background color
vr.pencolor("#D0CFCF")
#pencolor() is use to set the Pen color
vr.speed(10)
3. You’ll switch between drawing a circle with the pendown option and moving the ring turtle to the bottom of the next circle with the penup option, For that here, we use for loop.
for i in range(4):
vr.penup()
#Penup() ensures that the moving object you've created does not draw anything on the window.
vr.goto(i*70, 0)
#goto() : can be used to set a position for turtle.
vr.pendown()
#pendown() tell the turtle to leave ink on the screen as it moves or not to leave ink.
vr.circle(50)
#circle() : use for to draw a circle
When moving from one ring to the next, you must shift the turtle by slightly more than the diameter of the circle because the rings are separated by a gap.
4. Create a Label for the logo
vr.color("white")
vr.penup()
vr.goto(77, -40)
vr.pendown()
#creating a label
vr.write("Audi",font=("Arial", 16, "bold","italic"))
Complete Code
import turtle vr = turtle.Turtle() vr.pensize(7) turtle.Screen().bgcolor("black") vr.pencolor("#D0CFCF") vr.speed(10) for i in range(4): vr.penup() #Penup() ensures that the moving object you've created does not draw anything on the window. vr.goto(i*70, 0) #goto() : can be used to set a position for turtle. vr.pendown() #pendown() tell the turtle to leave ink on the screen as it moves or not to leave ink. vr.circle(50) #circle() : use for to draw a circle vr.color("white") vr.penup() vr.goto(77, -40) vr.pendown() #creating a label vr.write("Audi",font=("Arial", 16, "bold","italic")) turtle.done()
Output :
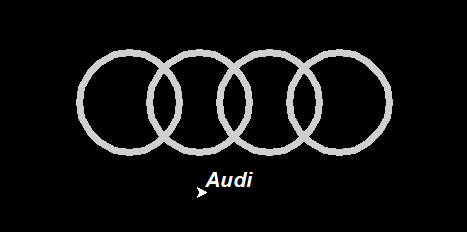
As you can see, we successfully Draw Audi Logo in Python Turtle. I hope you were able to complete this program successfully.
If you’re looking for more amazing turtle tutorials like this one, check out this: Awesome Python Turtle Codes.
If you don’t want to create all of the files and folders, you can run this program now using this online Python compiler, which is quick and simple.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Radha Krishna using Python Turtle
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Drawing letter A using Python Turtle
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Wishing Happy New Year 2023 in Python Turtle
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Draw Goku in Python Turtle
- Medical Store Management System Project in Python
- Draw Mickey Mouse in Python Turtle
- Creating Dino Game in Python
- Tic Tac Toe Game in Python