
This blog will discuss how we can format numbers as currency (an integer representing currency in standard currency format) with Python. While programming, most of our data comes in normal integer or float format; hence, when representing it in our app, we need to format it as though the app users have a good experience. There are several ways to do this.
1. Format Numbers as Currency with the Python format() method:
The easiest way to format numbers as currency in Python is using the .format() method of string. This way is one of the basic methods taught for strings in Python. We will write plain Python code and not use any modules, which might sometimes mess things up and make our application buggy. Documentation of .format() method: https://docs.python.org/3/library/stdtypes.html#str.format
my_currency = 1283493.23
desired_representation = "{:,}".format(my_currency)
print("$", desired_representation)
>> $ 1,283,493.23
Output:

Call str.split(sep)[0] and str.split(sep)[1] with str as the first result and sep as “.” to get the main currency and fractional currency, respectively, if the currency utilizes decimals to separate every three digits of the main currency and commas to separate fractional from main currency. To acquire the new main currency, call str.replace(old, new) with str as the main currency, old as “,”, and new as “.”. The fractional-main separator, the fractional currency value, and this result are combined using the operator ‘+’.
price = 213439.28
seperator_of_thousand = "."
seperator_of_fraction = ","
my_currency = "${:,.2f}".format(price)
if seperator_of_thousand == ".":
main_currency, fractional_currency = my_currency.split(".")[0], my_currency.split(".")[1]
new_main_currency = main_currency.replace(",", ".")
currency = new_main_currency + seperator_of_fraction + fractional_currency
print(my_currency)
>> $213,439.28
Output:
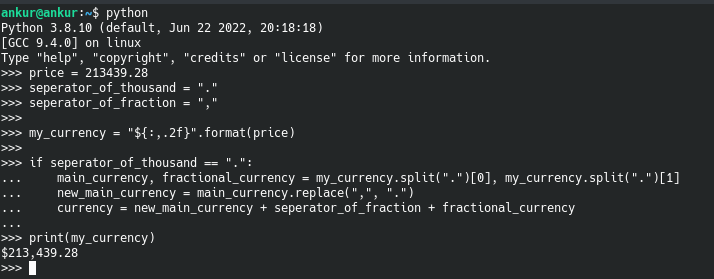
2. Format Numbers as Currency using the Python locale module:
In this method, we will use a module named “locale” built into Python while installing. This module will help us localize our python application and localize the representation of currency. This method will also help us write a single set of codes and not worry about which region the user will run the code. Python will automatically detect the region and serve our application data based on our localization.
import locale
locale.setlocale(locale.LC_ALL, '')
print(locale.currency(12345.67, grouping=True))
>> रू 12,345.67
Output:

3. Format Numbers as Currency using the Babel module:
This method will use ‘babel.numbers’ from the “Babel” module. This module will help us to convert the given standard integer numeral into a currency format for our application. This method might be a little tedious because it does not come built-in in Python version 3.8 and before.
First, install Babel using the following command in the command line or terminal.
$ pip install Babel
Code:
import babel.numbers
currency_in_number = 3212834.2
babel.numbers.format_currency(currency_in_number, 'INR', locale="en_IN")
>> ₹ 32,12,834.20
Output:

Thanks for reading this blog until the end. To learn more about basic scripting with Python, you can read other blogs on this website with the category: https://copyassignment.com/category/python-projects/.
Checkout Short Video:
Keep Learning, Keep Coding
Also Read:
- What is web development for beginners?Introduction In web development, we refer to website so web development refers to website development. “Web” word has been taken from the spider’s web because of the analogy that like a web is connected similarly websites are also connected to each other through the Internet. History of web development 3 Pillars of web development HTML…
- What does if __name__ == __main__ do in Python?Introduction Most of us have come across the if __name__ == “__main__” in Python while doing a program or we specifically write this function while doing a program. So what does this function basically do? Here, we are going to see why we are using this if __name__ == “__main__” statement in the programs and…
- Python | CRUD operations in MongoDBToday in this article, we will talk about some operations that can be performed on the database using the queries of MongoDB. In this article on CRUD operations in MongoDB with Python, we will learn how to perform the operations like Create, Read, Update, and Delete to manipulate the documents in the database. This time…
- CRUD operations in DjangoToday, we will learn how to perform CRUD operations in Django Python. We will perform CRUD operations using a form and without a form. We will use the absolute beginner-friendly approach in this article so that even a 1-day beginner of Django can understand how to perform CRUD operations in Django Python. Let’s start. CRUD…
- Install and setup Python in Windows 11Microsoft has released its latest version of the Windows Operating System. This blog will discuss how to Install Python in Windows 11 along with testing and setting up the interpreter. There will step-by-step guide without leaving any step behind. So, this blog is targetting absolute beginners as well as references for advanced programmers and users….
- Python Tkinter Button: Tutorial for BeginnersIn this tutorial, we will explore everything about how to create a Tkinter Button in python, everything will be beginner friendly. Tkinter is one of the simplest GUI libraries among all the GUI libraries supported by Python. Tkinter is Python’s de facto standard GUI means this is the official GUI library for Python which is…
- Sequel Programming Languages(SQL)In this article, we are going to learn about Sequel Programming Languages(SQL). Big enterprises like Facebook, Instagram, and LinkedIn, use SQL for storing the data in the back-end. So, If you want to get a job in the field of data, then it is the most important query language to learn. Before getting started, let…
- Run Python Code, Install Libraries, Create a Virtual Environment | VS CodeVisual Studio Code is one of the most efficient code compilers/interpreters. It is very promising because of the vast and widely available go-to extensions that help programmers. This article is an elaborative detail about how we can run Python code, install Python libraries, and create a virtual environment in Visual Studio Code. Write and Run…
- Calendar using Java with best examplesIn this article, we are going to learn how to code Calendar using Java. The calendar application is occasionally asked in interviews to be built by the candidate. If you are intermediate in Java, it helps to improve your coding skills also, it is interesting to make this application. Let’s get started! Calendar class in…
- How to make a Process Monitor in Python?In this article, we will build an application, Process Monitor in python using psutil. Python has a wide range of libraries and packages, which makes it the best choice for many developers. In the same way, we are going to make use of the psutil package to build our application, Process Monitor in Python. What…