In this tutorial, we will explore everything about how to create a Tkinter Button in python, everything will be beginner friendly. Tkinter is one of the simplest GUI libraries among all the GUI libraries supported by Python. Tkinter is Python’s de facto standard GUI means this is the official GUI library for Python which is not official by the owners of Python.
Tkinter Button in Python
Creating a simple tkinter button
We can use Button() to create a button in Python using tkinter.
b = Button(top, text="I am a Tkinter Button")
from tkinter import * top = Tk() top.geometry("250x100") top.title('Button in Tkinter') b = Button(top, text="I am a Tkinter Button") b.place(x=60, y=50) top.mainloop()
Output:

Adding function to the button
With the command option of Button(), we can call a function using a tkinter button in Python. To call a function, we just need to write the function name:
def fun():
print('Tkinter Button clicked!')
b = Button(top, text="I am a Tkinter Button", command=fun)
from tkinter import * top = Tk() top.geometry("250x150") top.title('Button in Tkinter') def fun(): print('Tkinter Button clicked!') b = Button(top, text="I am a Tkinter Button", command=fun) b.place(x=60, y=50) top.mainloop()
Output:
Showing a message
To show a message in tkinter, we need to import messagebox from tkinter.
from tkinter import messagebox
To show message using messagebox, we need to use showinfo().
messagebox.showinfo("Title of MessageBox", "Message")
from tkinter import * from tkinter import messagebox top = Tk() top.geometry("250x150") top.title('Button in Tkinter') def fun(): messagebox.showinfo("MessageBox", "Button clicked") b = Button(top, text="Show Message", command=fun) b.place(x=80, y=50) top.mainloop()
Output:
Giving padding
To give padding in buttons in tkinter, we should use padx(for x direction) and pady(for y direction).
b = Button(top, padx=10, pady=15)
from tkinter import * top = Tk() top.geometry("250x100") top.title('Button in Tkinter') b = Button(top, text="I am a Tkinter Button", padx=10, pady=15) b.place(x=60, y=20) top.mainloop()
Output:

Color customization
Tkinter in Python offers many color customization options to make tkinter buttons beautiful. For example, bg to change the background, fg to change text color, activebackground to change the background on click of a button, and activeforeground to change text color on click of a button.
b = Button(top, text="Button", bg='gray', fg='green', activebackground='Red', activeforeground='white')
from tkinter import * top = Tk() top.geometry("250x100") top.title('Button in Tkinter') b = Button(top, text="I am a Tkinter Button", bg='gray', fg='green', activebackground='Red', activeforeground='white') b.place(x=60, y=50) top.mainloop()
Output:
Width and Height of tkinter buttons
We can change the width of the border of a tkinter button.
b = Button(top, text="I am a Tkinter Button", bd=5)
We can also change the width and height of a button in Python tkinter.
b = Button(top, text="I am a Tkinter Button", width=20, height=4)
from tkinter import * top = Tk() top.geometry("270x150") top.title('Button in Tkinter') b = Button(top, text="I am a Tkinter Button", bd=5, width=20, height=4) b.place(x=55, y=50) top.mainloop()
Output:

Using image as a button
We can use an image as a button, we need to use PhotoImage from tkinter.ttk. Then we can import an image in our program which we can use in our button.
from tkinter.ttk import *
photo = PhotoImage(file="path_to_image")
b = Button(top, image=photo)
from tkinter import * from tkinter.ttk import * top = Tk() top.geometry("270x150") top.title('Button in Tkinter') photo = PhotoImage(file="path_to_image") b = Button(top, image=photo) b.place(x=55, y=50) top.mainloop()
Output:
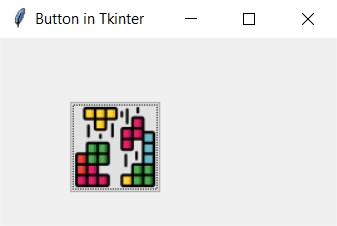
Font in tkinter button
We can also customize a button in the tkinter. For that, we need to import font from tkinter.font. Then, we can use Font() from font. After giving all values to font(), we can use it inside our button. We can set font family, size, weight, underline, etc of the font.
import tkinter.font as font
myFont = font.Font(family='Helvetica', size=9, weight='bold', underline=True)
b = Button(top, text="Customizing fonts in Tkinter Button", font=myFont)
from tkinter import * import tkinter.font as font top = Tk() myFont = font.Font(family='Helvetica', size=9, weight='bold', underline=True) top.geometry("270x150") top.title('Button in Tkinter') b = Button(top, text="Customizing fonts in Tkinter Button", font=myFont) b.place(x=70, y=50) top.mainloop()
Output:

State of a button in tkinter
By default, a tkinter button is active, we can disable it using the state option of Button().
b2 = Button(top, text="Disabled Tkinter Button", state=DISABLED)
from tkinter import * top = Tk() top.geometry("270x150") top.title('Button in Tkinter') b1 = Button(top, text="Active Tkinter Button") b2 = Button(top, text="Disabled Tkinter Button", state=DISABLED) b1.place(x=78, y=35) b2.place(x=70, y=70) top.mainloop()
Output:
We recommend you bookmark this page for the future in case you lost it and you may need it.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter