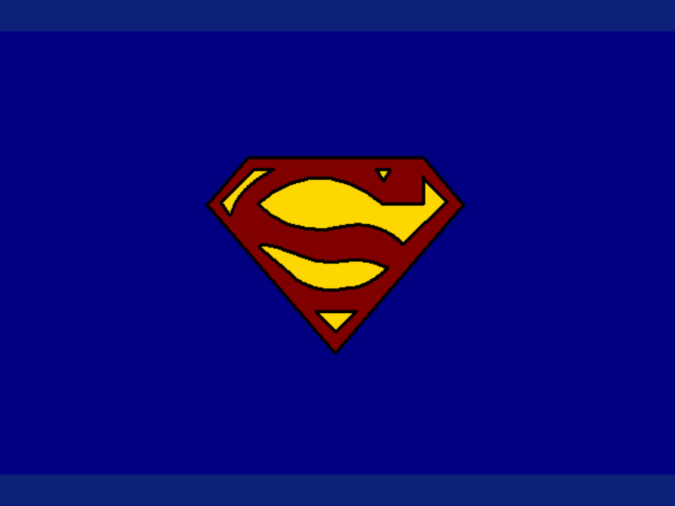
Python is an interpreted, object-oriented, high-level programming language. It has interfaces to many system calls and libraries, as well as to various windowing systems (X11, Motif, Tk, Mac, and MFC).
Programmers can write GUI applications using the Tkinter module that comes with Python or one of the other toolkits listed on this site. Turtle graphics require a version of Python to be installed with Tk support in order to run.
The turtle module provides an easy way to program geometric figures created with a pen plotter or “turtle.” You can implement some interesting algorithms with turtle graphics. For example, you can draw fractals by recursively drawing smaller copies of your figure.
Turtle graphics are commonly used in Matlab or some other languages as well. However, the main use of turtle is as a learning tool, just like how a calculus tutor can help you with complex problems in mathematics. Python can even be used to generate 3D graphics with vpython if you’d like to take your art to the next dimension.
Let’s draw the Superman Logo on the screen using Python. It is just like drawing on paper using pens but instead of your hand moving a pen, there will be written commands for the program to move the pen up, down, left, right, and so on.
Complete Code to Draw Superman Logo with Detailed Comments
import turtle #import the required package to draw the logo t=turtle.Turtle() #set the variable ‘t’ to the function turtle.Turtle() to shorten the code throughout turtle.Screen().bgcolor('navy') #set the color of the screen to navy to match Superman’s costume def curve(value): #create a function to generate curves in turtle for i in range(value): #for loop to repeat the inputted value number of times t.right(1) #step by step curve t.forward(1) t.penup() #pen is in the up position so it will not draw t.setposition(0,43) #move the pen to these x and y coordinates t.pendown() #pen is in the down position so it will draw t.begin_fill() #start filling in the shape t.pencolor('black') #change the pen color to black t.fillcolor('maroon') #change the shape fill color to maroon to match the Superman logo t.pensize(3) #use a pen size of 3 to create a black border t.forward(81.5) #the pen will move forward this number to start the shield of the logo t.right(49.4) #rotate the pen right 49.4 degrees t.forward(58) #move the pen forward by 58 t.right(81.42) #rotate right by 81.42 degrees t.forward(182) #move the pen forward by 182 t.right(98.36) #rotate the pen right by 98.36 degrees t.forward(182) #move the pen forward by 182 t.right(81.42) #rotate the pen by 81.42 degrees to the right t.forward(58) #move the pen forward 58 t.right(49.4) #rotate the pen to the right by 49.4 t.forward(81.5) #move the pen forward by 81.5 to meet the start at the top of the shield t.end_fill() #complete the fill of the shield so the shape is closed off t.penup() #pen will not draw t.setposition(38,32) #now to create the yellow parts of the Superman logo t.pendown() #the pen is now poised to draw t.begin_fill() #start a new shape t.fillcolor('gold') #change the fill color to gold to match the Superman logo t.forward(13) #move the pen forward by 13 t.right(120) #rotate the pen right by 120 degrees t.forward(13) #move the pen forward by 13 t.right(120) #rotate the pen right by 120 degrees t.forward(13) #move the pen forward by 13 t.end_fill() #the small triangle on the right is now complete t.penup() #stop the pen from drawing t.setposition(81.5,25) #now to create the larger yellow part of the Superman logo, change the position of the pen t.pendown() #the pen will now draw again t.begin_fill() #the fill is still the same color set before t.right(210) #rotate the pen right by 210 degrees t.forward(25) #move the pen forward by 25 t.right(90) #rotate the pen right by 90 degrees t.forward(38) #move the pen forward by 38 t.right(45) #rotate the pen right by 45 degrees t.circle(82,90) #this function is used to draw a circle in turtle, the first integer is the radius and the second is the number of degrees of the circle drawn t.left(90) #rotate the pen left by 90 degrees t.circle(82,60) #create a circle of radius 82 and only complete 60 degrees of the circle curve(61) #call the curve function that was previously defined, pass an integer value equal to the length of the curve desired t.left(90) #rotate the pen left by 90 degrees t.forward(57) #move the pen forward by 57 t.left(90) #rotate the pen left by 90 degrees t.forward(32) #move the pen forward by 32 t.end_fill() #fill in the larger yellow part of the logo t.penup() #stop drawing t.home() #reset the pen location to the origin so the orientation is also reset t.setposition(-69,-38) #finish the major parts of the S superimposition on the shield t.pendown() t.begin_fill() curve(20) t.forward(33) t.left(10) t.circle(82,20) curve(30) t.forward(10) t.right(110) curve(40) t.right(10) t.circle(50,10) curve(45) t.right(5) t.forward(45) t.end_fill() t.penup() t.home() t.setposition(20,-100) t.pendown() t.begin_fill() t.right(135) t.forward(27) t.right(90) t.forward(27) t.right(135) t.forward(38.18) t.end_fill() t.penup() t.home() t.setposition(-57,32) t.pendown() t.begin_fill() t.right(180) t.forward(18) t.left(45) t.forward(44) t.left(80) t.forward(15) t.left(130) curve(40) t.forward(20) t.end_fill() t.hideturtle() #use this command to hide the turtle so it is not visible in the final image turtle.exitonclick() #this command will leave the window open until it is clicked
Output
Window output of Superman Logo generated with Python turtle
Explanation
The Superman logo has undergone many changes over the years. It consists of a shield and a stylized letter “S” in red and yellow. The design of the logo is often attributed to Joe Shuster and Jerry Siegel, the creators of the Superman character.
Superman was introduced in Action Comics #1 which was published by DC Comics in June 1938. The “S” has been thought to stand for Superman, but after Superman: The Movie, Superman’s Kryptonian family is shown bearing a similar emblem, meaning that the Superman logo might actually be a family coat of arms.
The below functions were used in programming the Superman logo:
turtle.Screen().bgcolor() – This function can be used to change the color of the screen
turtle.right() – This function is used to rotate the cursor to the right by the indicated number of degrees. The default degree unit is set to degrees but radians can be specified with turtle.radians().
turtle.left() – Similarly, this function rotates the drawing pen to the left by the indicated number of degrees.
turtle.pencolor() – This will change the color of the line with the inputted argument. Python accepts numerous color names and also r,g,b values. All CSS named colors are available in Python and can be used here.
turtle.fillcolor() – This will fill the drawn shape with the specified color.
turtle.begin_fill() – The fill will start with this command.
turtle.end_fill() – The fill will end with this command.
turtle.penup() – With the pen in the up position, no lines will be drawn.
turtle.pendown() – The pen in the down position will draw when commands are given.
turtle.setposition(x,y) – The pen will be moved to the specified position following the standard Cartesian plane coordinate system.
turtle.home() – The pen will be moved back to the origin on the Cartesian plane and the orientation is reset.
turtle.circle() – The pen will move in a circle. The radius can be specified as well as the extent to which the pen is traveled. Steps can also be specified to create equilateral-sided shapes.
turtle.hideturtle() – This function hides the cursor; if hidden, drawing can be faster in the turtle.
turtle.exitonclick() – This function waits for the user to click on the window to close it, otherwise the window will automatically close when the code is finished running.
You can input the above code straight into your Python code editor and run it to create the logo!
For more information on how to use turtle in Python take a look at the beginner’s guide, and if you’d like to draw something else like an Among Us character!
Also Read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Wishing Happy New Year 2023 in Python Turtle
- Snake and Ladder Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods
- Happy Birthday Python Program In Turtle
- I Love You Program In Python Turtle
- Draw Python Logo in Python Turtle
- Space Invaders game using Python
- Draw Google Drive Logo Using Python
- Draw Instagram Reel Logo Using Python
- Draw The Spotify Logo in Python Turtle
- Draw The CRED Logo Using Python Turtle
- Draw Javascript Logo using Python Turtle
- Draw Dell Logo using Python Turtle
- Draw Spider web using Python Turtle