
Introduction
Welcome to another helpful project. Today we are going to develop a Loan Management System Project in Java. I think that this project can be useful and helpful for those who are at an intermediate level in programming. For this project, we used XAMPP for MYSQL and Database and for developing it IntelliJ Idea Professional. This project required a GUI, so I used JavaFX and Scene Builder as we can also be upgraded our knowledge and use new tools avoiding using Java Swing and AWT libraries. This application works only using administrator credentials and he will be able to:
- Checking the list of clients in the database
- Editing a selected client
- Deleting a selected client
- Updating a selected client
- Performing log out
Loan Management System Project in Java: Project Overview
Project Name: | Loan Management System Project in Java |
Abstract | It’s a GUI-based project used with the JavaFx module to organize all the elements that work under loan management. |
Language/s Used: | Java |
IDE | IntelliJ Idea Professional(Recommended) |
Python version (Recommended): | Java SE 18.0. 2.1 |
Database: | MySQL |
Type: | Desktop Application |
Recommended for | Final Year Students |
Project Setup
Notice that you must have already installed a JDK on your system. The first step will be creating a new project, so we will open our IntelliJ program and select:
File → New → Project… → JavaFX
We will give it the following name: “LoanManagementSystem” and select the JDK you have (we used the 16th version). At the creation, you will notice that it will automatically load two classes in the ‘src’ folder:
HelloApplication.java and HelloController.java then there will be an XML file located in the ‘resources’ folder that we can open with SceneBuilder by simply clicking on it and then clicking on “Open in SceneBuilder”. We can create with Scene Builder our GUI panels/windows etc.
MySQL Setup
1. Launching XAMPP (detailed for Ubuntu users)
If you are a Windows user simply launch the ‘xampp.exe’ shortcut on the Desktop. Otherwise, if you are an Ubuntu user the first thing that you must do is to run the XAMPP application:
- ~→ type ‘cd ..’ + Enter
- /home → type ‘cd ..’ + Enter
- / → type ‘cd opt/lampp’ + Enter
- opt/lampp → type sudo ./manager-linux-x64.run + Enter
Put your Ubuntu user password and then you can go to “Manage Servers” and click on Start All. In IntelliJ, under your project expand external libraries and right-click on junit4, and select Open Library Settings. Select the libraries tab and click on the + button. Browse your jar file downloaded from the above step and click on it. This will add dependency to your project. The steps will differ if you are using a different IDE.
2. Create a database called ‘loan’
create database loan
3. Create table details
CREATE TABLE details (
Times varchar(50) NOT NULL,
Cus_Name varchar(50) NOT NULL,
Item_Type varchar(50) NOT NULL,
Item_Price varchar(50) NOT NULL,
Down_Payment varchar(50) NOT NULL,
Loan_Balance varchar(50) NOT NULL,
Payment varchar(50) NOT NULL,
Due_Amount varchar(50) NOT NULL,
Datee varchar(50) NOT NULL
) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4;
4. Insert some values in the table details
INSERT INTO details (Times, Cus_Name, Item_Type, Item_Price, Down_Payment, Loan_Balance, Payment, Due_Amount, Datee) VALUES ('11:30:00', 'Giuseppe', 'Clothes', '1200', '1000', '1000', '800', '200', '2022-09-02'), ('16:00:00', 'Alexander', 'CNC', '2005', '300', '200', '1700', '300', '2022-08-31');
Implementing Loan Management System Project in Java
Here we are in the best part ever: the coding part. It’s important to notice that I have a default user, so I thought that for this project there was no need for a table ‘user’ in the database since the only user that can log in is the administrator.
1. Importing external libraries
import javafx.fxml.FXML;
import javafx.scene.Scene;
import javafx.scene.control.*;
import java.sql.Connection;
2. Create a connection with MySQL
As we are having an intermediate-level project we want to use classes so we can create a class called ‘db.java’.
Since we need a connection with the database it’s important to make a connection method that can allow us to perform on it SQL queries.
For this class we will use these external libraries:
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.ResulSet;
Now we write the method getDBCon() throws Exception, that will allow us to establish the connection with database loan in this way:
private static Connection c;
public static Connection getDBCon() throws Exception {
if (c == null) { //checking if c is null then launch method under.
setUpConnection();
}//else return c
return c;
}
In method setUpConnection we will write:
private static void setUpConnection() throws Exception {
//Class.forName("com.mysql.jdbc.Driver"); ← old method
DriverManager.registerDriver(new com.mysql.jdbc.Driver()); //works better
System.out.println("Driver correttamente registrato.");
c = DriverManager.getConnection("jdbc:mysql://localhost:3306/loan", "root", "root");
}
3. Admin Login
For this part, we will go to Scene1Controller and we will use two private Strings in this way:
private String default_user = “admin”;
private String default_pass = “admin”;
//for connecting a method to a button just use @FXML and also specify it in xml file (called scene1.fxml)
@FXML
public void switchScene() throws Exception {
if(username.getText().isBlank() || pass.getText().isEmpty()){
System.out.println("Invalid input data!");
}else{
/* Connection with database for retrieving the correct data */
Connection conn = db.getDBCon();
//this check will allow us to go and fecthing data for admin user
if((conn != null) && (username.getText().equals(default_user) &&
pass.getText().equals(default_pass))){
//here an alert will appear on the screen (see image under with tag #successful_login)
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setContentText("Login Passed!");
alert.showAndWait();
//we go to second scenario!
main.setScene(scene2);
username.setText("");
pass.setText("");
labelError.setText("");
}else{
Alert alert = new Alert(Alert.AlertType.WARNING);
alert.setTitle("Login Incorrect!");
alert.setContentText("Username or password incorrect!\nRetry again!");
alert.showAndWait();
labelError.setText("Username or password not correct!");
}
}
}
Output:
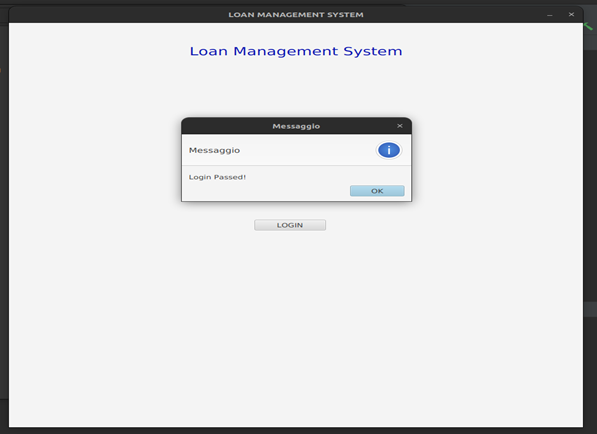
4. Fetching data on Client List
For fetching the data on the client list after login, we will write in Scene2Controller.java this line:
static final String showAllClients = "SELECT DISTINCT * FROM details";
Then in the method called ‘fillList(ListView l) throws Exception’ we will fetch each row in the result and store it in our listview.
if(click == 1){
ResultSet rs = db.search(showAllClients);
ListView listDetails = l;
Detail d = null;
while(rs.next()){
//System.out.println("CusName : "+rs.getString("Cus_Name")+", ItemType : "+rs.getString("Item_Type"));
d = new Detail(rs.getString("Times"),rs.getString("Cus_Name"), rs.getString("Item_Type"), rs.getString("Item_Price"),rs.getString("Down_Payment"), rs.getString("Loan_Balance"), rs.getString("Payment"), rs.getString("Due_Amount"), rs.getString("Datee"));
listDetails.getItems().add(d);
}
listDetails.getSelectionModel().setSelectionMode(SelectionMode.SINGLE);
click = 0;
}
Output:
The result will be shown as this after clicking on the button “View Client List“:

5. Edit data of the selected client
To edit the data of a selected client we will write a method called ‘editClient() throws Exception’:
@FXML
public void editClient() throws Exception{
updateClient.setDisable(false);
ObservableList clientSelected = listDetails.getSelectionModel().getSelectedItems();
Alert alert = new Alert(Alert.AlertType.INFORMATION);
alert.setTitle("Edit Client");
alert.setContentText("Selected Client : "+clientSelected.toString());
alert.showAndWait();
String entity = clientSelected.get(0).toString();
System.out.println("Client : "+entity);
String[] substring = entity.split(",");/* Splitting the string by ',' */
time = substring[0];
nameUserSelected.setText(substring[1]);
itType = substring[2];
itemType.setText(itType);
itPrice = substring[3];
itemPrice.setText(itPrice);
downPayment = substring[4];
loanBalance = substring[5];
loanBal.setText(loanBalance);
payment = substring[6];
Payment.setText(payment);
due_Amount = substring[7];
data = substring[8];
}
Output:
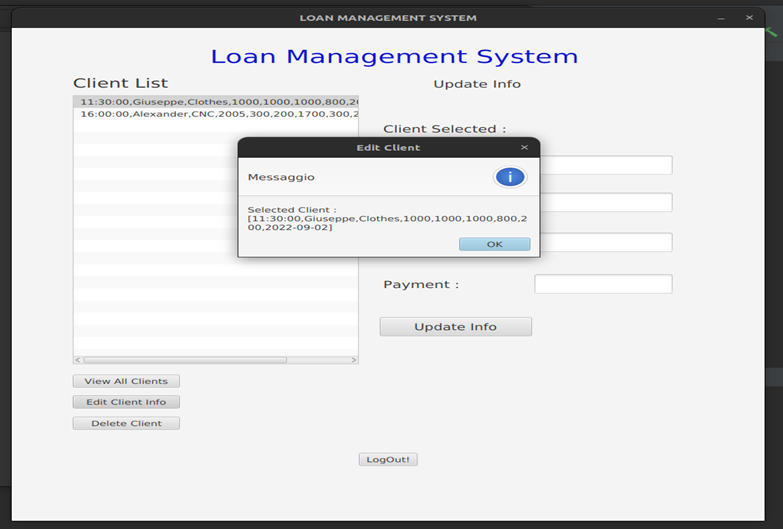
After clicking on the Edit Client Info button you will see this message, after it in the ‘Update Info’ section it will appear the data of the item selected.
6. Update data of a selected client (first do step 5)
To update info into the database after the field that has to be filled, we will write the under method ‘updateInfo() throws Exception’:
@FXML
public void updateInfo() throws Exception{
Alert aInfo = new Alert(Alert.AlertType.INFORMATION);
aInfo.setTitle("Updating client data...");
aInfo.setContentText("!!! Data are changed !!!\nItem Type New : "+itemType.getText()+"\nItem Price New : "+itemPrice.getText()+"\nLoan Balance New : "+loanBal.getText()+"\nPayment : "+Payment.getText());
/*Executing query update...*/
String qUp = "UPDATE details SET Item_Type='"+itemType.getText()+"', Item_Price='"+itemPrice.getText()+"', Loan_Balance='"+loanBal.getText()+"',Payment='"+Payment.getText()+"' WHERE Cus_Name='"+nameUserSelected.getText()+"'";
Connection conn = db.getDBCon();
Statement stmt = conn.createStatement();
stmt.executeUpdate(qUp);
int selectedIndex = listDetails.getSelectionModel().getSelectedIndex();
listDetails.getItems().remove(selectedIndex);
listDetails.getItems().add(selectedIndex, new Detail(time, nameUserSelected.getText(), itemType.getText(), itemPrice.getText(), downPayment, loanBal.getText(), Payment.getText(), due_Amount, data));
updateClient.setDisable(true);
}
Output:

You can edit these fields, after updating info the ‘Update Info’ button will be disabled as the user can upload one time only.
7. Delete selected client from the Client List
For the Delete method of the selected client we will write the following lines of code:
@FXML
public void deleteClient() throws Exception {
clientSelected = listDetails.getSelectionModel().getSelectedItems();
int selectedItem = listDetails.getSelectionModel().getSelectedIndex();
System.out.println("Before parsing : "+selectedItem);
String entity = clientSelected.get(0).toString();
String[] substring = entity.split(",");/* Splitting the string by ',' */
nameSel = substring[1];
if(clientSelected.isEmpty() || clientSelected.contains("")){
Alert alert = new Alert(Alert.AlertType.ERROR);
alert.setTitle("Error Occured!");
alert.setContentText("It's not possible remove null client!");
alert.showAndWait();
}else{
Alert alert = new Alert(Alert.AlertType.CONFIRMATION);
alert.setTitle("Removing Client...");
alert.setContentText("This client will be removed : "+nameSel+"\nDo you want to remove it?");
Optional<ButtonType> result = alert.showAndWait();
if(result.get() == ButtonType.OK){
Connection conn = db.getDBCon();
Statement stmt = conn.createStatement();
String queryRemoveClientSelected = "DELETE FROM details WHERE Cus_Name='"+nameSel+"'";
stmt.executeUpdate(queryRemoveClientSelected);
System.out.println("Item selected : "+selectedItem);
listDetails.getItems().remove(selectedItem);
}
}
}
You can click on the Delete Client button, at that time the list on the screen will be uploaded automatically, without again fetching data from the database.
8. Log Out from the View
For performing LogOut operation we will produce this method:
@FXML
public void goBack(){
Alert a = new Alert(Alert.AlertType.INFORMATION);
a.setTitle("Logging out");
a.setContentText("Log Out Successful!");
a.showAndWait();
main.setScene(scene1);
}
It will produce this message:

Complete code for Loan Management System Project in Java
File- db.java
package com.project.project2scenes; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; /** * @author Giuseppe */ public class db { private static Connection c; private static void setUpConnection() throws Exception { //Class.forName("com.mysql.jdbc.Driver"); DriverManager.registerDriver(new com.mysql.jdbc.Driver()); System.out.println("Driver correttamente registrato."); c = DriverManager.getConnection("jdbc:mysql://localhost:3306/loan", "root", "root"); } public static void iud(String sql) throws Exception { if (c == null) { setUpConnection(); } c.createStatement().executeUpdate(sql); } public static ResultSet search(String sql) throws Exception { if (c == null) { setUpConnection(); } return c.createStatement().executeQuery(sql); } public static Connection getDBCon() throws Exception { if (c == null) { setUpConnection(); } return c; } }
File- Main.java
package com.project.project2scenes; import javafx.application.*; import javafx.fxml.FXMLLoader; import javafx.stage.*; import javafx.scene.*; import javafx.scene.layout.*; import java.io.IOException; public class Main extends Application { // Primary Stage Stage window; // Two scenes Scene scene1, scene2, scene3; // The panes are associated with the respective .fxml files private Pane pane1; private Pane pane2; private Pane pane3; public static void main(String[] args) { launch(args); } @Override public void start(Stage primaryStage) { try { // Set the window as primary stage window = primaryStage; // Load the fxml files and their controllers FXMLLoader loader = new FXMLLoader(); loader.setLocation(Main.class.getResource("scene1.fxml")); pane1 = loader.load(); Scene1Controller controller1 = loader.getController(); loader = new FXMLLoader(); loader.setLocation(Main.class.getResource("scene2.fxml")); pane2 = loader.load(); Scene2Controller controller2 = loader.getController(); // The scenes are based on what has been loaded from the .fxml files Scene scene1 = new Scene(pane1); Scene scene2 = new Scene(pane2); /* Pass reference the each scenes controller */ controller1.setScene2(scene2); controller1.setMain(this); controller2.setScene1(scene1); controller2.setMain(this); //Display scene 1 at first window.setScene(scene1); window.setTitle("LOAN MANAGEMENT SYSTEM"); window.show(); } catch (IOException e) { e.printStackTrace(); } } // used by the controllers to switch the scenes public void setScene(Scene scene){ window.setScene(scene); } }
File- Detail.java
package com.project.project2scenes; public class Detail { String Times; String Cus_Name; String Item_Type; String Item_Price; String Down_Payment; String Loan_Balance; String Payment; String Due_Amount; String Datee; public Detail(String times, String cus_Name, String item_Type, String item_Price, String down_Payment, String loan_Balance, String payment, String due_Amount, String datee) { this.Times = times; this.Cus_Name = cus_Name; this.Item_Type = item_Type; this.Item_Price = item_Price; this.Down_Payment = down_Payment; this.Loan_Balance = loan_Balance; this.Payment = payment; this.Due_Amount = due_Amount; this.Datee = datee; } public String getTimes() { return Times; } public void setTimes(String time) { this.Times = time; } public String getCus_Name() { return Cus_Name; } public void setCus_Name(String cus_Name) { Cus_Name = cus_Name; } public String getItem_Type() { return Item_Type; } public void setItem_Type(String item_Type) { Item_Type = item_Type; } public String getItem_Price() { return Item_Price; } public void setItem_Price(String item_Price) { Item_Price = item_Price; } public String getDown_Payment() { return Down_Payment; } public void setDown_Payment(String down_Payment) { Down_Payment = down_Payment; } public String getLoan_Balance() { return Loan_Balance; } public void setLoan_Balance(String loan_Balance) { Loan_Balance = loan_Balance; } public String getPayment() { return Payment; } public void setPayment(String payment) { Payment = payment; } public String getDue_Amount() { return Due_Amount; } public void setDue_Amount(String due_Amount) { Due_Amount = due_Amount; } public String getDatee() { return Datee; } public void setDatee(String datee) { Datee = datee; } @Override public String toString(){ String res = ""; res += getTimes() +","+getCus_Name()+","+getItem_Type() +","+ getItem_Price()+","+ getDown_Payment() +","+ getLoan_Balance() +","+ getPayment() +","+ getDue_Amount()+","+getDatee(); return res; } }
File- Scene1Controller.java
package com.project.project2scenes; import javafx.fxml.FXML; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.stage.Window; import java.io.IOException; import java.sql.Connection; import java.sql.ResultSet; import java.util.ArrayList; public class Scene1Controller { private Scene scene2; private Main main; private String default_user = "admin"; private String default_pass = "admin"; @FXML Label label, labelError; @FXML TextField username; @FXML PasswordField pass; public void setMain(Main main){ this.main = main; } public void setScene2(Scene scene2){ this.scene2 = scene2; } // this method is called by clicking the button @FXML public void switchScene() throws Exception { if(username.getText().isBlank() || pass.getText().isEmpty()){ System.out.println("Invalid input data!"); }else{ /* Connection with database for retrieving the correct data */ Connection conn = db.getDBCon(); if((conn != null) && (username.getText().equals(default_user) && pass.getText().equals(default_pass))){ Alert alert = new Alert(Alert.AlertType.INFORMATION); alert.setContentText("Login Passed!"); alert.showAndWait(); //we go to second scenario! main.setScene(scene2); username.setText(""); pass.setText(""); labelError.setText(""); }else{ Alert alert = new Alert(Alert.AlertType.WARNING); alert.setTitle("Login Incorrect!"); alert.setContentText("Username or password incorrect!\nRetry again!"); alert.showAndWait(); labelError.setText("Username or password not correct!"); } } } }
File- Scene2Controller.java
package com.project.project2scenes; import javafx.collections.ObservableList; import javafx.event.ActionEvent; import javafx.fxml.FXML; import javafx.fxml.FXMLLoader; import javafx.scene.Scene; import javafx.scene.control.*; import javafx.scene.layout.Pane; import javafx.stage.Window; import java.sql.Connection; import java.sql.ResultSet; import java.sql.Statement; import java.util.HashMap; import java.util.Optional; public class Scene2Controller { @FXML public ListView listDetails; public String data, nameSel, due_Amount, time, downPayment, itType, itPrice, loanBalance, payment; @FXML private Button searchClient, editClient, deleteClient, updateClient; private Scene scene1, scene3; private Main main; @FXML private Label nameUserSelected; @FXML private TextField itemType, itemPrice, loanBal, Payment; int click = 1; public ObservableList clientSelected; static final String showAllClients = "SELECT DISTINCT * FROM details"; public void setList(ListView l) { this.listDetails = l; } public void setMain(Main main){this.main = main;} public void setScene1(Scene scene1){ this.scene1 = scene1; } // this method is called by clicking the button @FXML public void goBack(){ Alert a = new Alert(Alert.AlertType.INFORMATION); a.setTitle("Logging out"); a.setContentText("Log Out Successful!"); a.showAndWait(); main.setScene(scene1); } /* Update function : it updates the selected client info*/ @FXML public void updateInfo() throws Exception{ Alert aInfo = new Alert(Alert.AlertType.INFORMATION); aInfo.setTitle("Updating client data..."); aInfo.setContentText("!!! Data are changed !!!\nItem Type New : "+itemType.getText()+"\nItem Price New : "+itemPrice.getText()+"\nLoan Balance New : "+loanBal.getText()+"\nPayment : "+Payment.getText()); /*Executing query update...*/ String qUp = "UPDATE details SET Item_Type='"+itemType.getText()+"', Item_Price='"+itemPrice.getText()+"', Loan_Balance='"+loanBal.getText()+"',Payment='"+Payment.getText()+"' WHERE Cus_Name='"+nameUserSelected.getText()+"'"; Connection conn = db.getDBCon(); Statement stmt = conn.createStatement(); stmt.executeUpdate(qUp); int selectedIndex = listDetails.getSelectionModel().getSelectedIndex(); listDetails.getItems().remove(selectedIndex); listDetails.getItems().add(selectedIndex, new Detail(time, nameUserSelected.getText(), itemType.getText(), itemPrice.getText(), downPayment, loanBal.getText(), Payment.getText(), due_Amount, data)); updateClient.setDisable(true); } /* Delete Client function : it deletes the selected client from the list!*/ @FXML public void deleteClient() throws Exception { clientSelected = listDetails.getSelectionModel().getSelectedItems(); int selectedItem = listDetails.getSelectionModel().getSelectedIndex(); System.out.println("Before parsing : "+selectedItem); String entity = clientSelected.get(0).toString(); String[] substring = entity.split(",");/* Splitting the string by ',' */ nameSel = substring[1]; if(clientSelected.isEmpty() || clientSelected.contains("")){ Alert alert = new Alert(Alert.AlertType.ERROR); alert.setTitle("Error Occured!"); alert.setContentText("It's not possible remove null client!"); alert.showAndWait(); }else{ Alert alert = new Alert(Alert.AlertType.CONFIRMATION); alert.setTitle("Removing Client..."); alert.setContentText("This client will be removed : "+nameSel+"\nDo you want to remove it?"); Optional<ButtonType> result = alert.showAndWait(); if(result.get() == ButtonType.OK){ Connection conn = db.getDBCon(); Statement stmt = conn.createStatement(); String queryRemoveClientSelected = "DELETE FROM details WHERE Cus_Name='"+nameSel+"'"; stmt.executeUpdate(queryRemoveClientSelected); System.out.println("Item selezionato : "+selectedItem); listDetails.getItems().remove(selectedItem); } } } /* Edit Client Function */ @FXML public void editClient() throws Exception{ updateClient.setDisable(false); ObservableList clientSelected = listDetails.getSelectionModel().getSelectedItems(); Alert alert = new Alert(Alert.AlertType.INFORMATION); alert.setTitle("Edit Client"); alert.setContentText("Selected Client : "+clientSelected.toString()); alert.showAndWait(); String entity = clientSelected.get(0).toString(); System.out.println("Client : "+entity); String[] substring = entity.split(",");/* Splitting the string by ',' */ time = substring[0]; nameUserSelected.setText(substring[1]); itType = substring[2]; itemType.setText(itType); itPrice = substring[3]; itemPrice.setText(itPrice); downPayment = substring[4]; loanBalance = substring[5]; loanBal.setText(loanBalance); payment = substring[6]; Payment.setText(payment); due_Amount = substring[7]; data = substring[8]; } /* View List Item function : it fills all the rows connecting to database*/ @FXML public void fillList(ListView l) throws Exception { if(click == 1){ ResultSet rs = db.search(showAllClients); ListView listDetails = l; Detail d = null; while(rs.next()){ //System.out.println("CusName : "+rs.getString("Cus_Name")+", ItemType : "+rs.getString("Item_Type")); d = new Detail(rs.getString("Times"),rs.getString("Cus_Name"), rs.getString("Item_Type"), rs.getString("Item_Price"),rs.getString("Down_Payment"), rs.getString("Loan_Balance"), rs.getString("Payment"), rs.getString("Due_Amount"), rs.getString("Datee")); listDetails.getItems().add(d); } listDetails.getSelectionModel().setSelectionMode(SelectionMode.SINGLE); click = 0; } } public void fillList(ActionEvent actionEvent) throws Exception { fillList(listDetails); } }
For FXML Scene1 ‘scene1.fxml’
<?xml version="1.0" encoding="UTF-8"?> <?import javafx.scene.control.Button?> <?import javafx.scene.control.Label?> <?import javafx.scene.control.PasswordField?> <?import javafx.scene.control.TextField?> <?import javafx.scene.layout.Pane?> <?import javafx.scene.text.Font?> <Pane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="900.0" prefWidth="900.0" xmlns="http://javafx.com/javafx/17" xmlns:fx="http://javafx.com/fxml/1" fx:controller="com.project.project2scenes.Scene1Controller"> <children> <Label fx:id="USERNAME" layoutX="301.0" layoutY="253.0" prefHeight="16.0" prefWidth="84.0" text="Username : " visible="true" /> <Button layoutX="385.0" layoutY="438.0" mnemonicParsing="false" onAction="#switchScene" prefHeight="24.0" prefWidth="112.0" text="LOGIN" /> <TextField fx:id="username" layoutX="401.0" layoutY="249.0" promptText="Username" /> <Label layoutX="301.0" layoutY="303.0" prefHeight="16.0" prefWidth="84.0" text="Password : " /> <PasswordField fx:id="pass" layoutX="398.0" layoutY="299.0" promptText="Insert password" /> <Label fx:id="labelError" layoutX="303.0" layoutY="398.0" prefHeight="16.0" prefWidth="267.0" text="" /> <Label alignment="CENTER" layoutX="220.0" layoutY="34.0" prefHeight="58.0" prefWidth="461.0" text="Loan Management System" textFill="#0712b2"> <font> <Font size="25.0" /> </font> </Label> </children> </Pane>
File Scene2 FXML ‘scene2.fxml’
<?xml version="1.0" encoding="UTF-8"?> <?import javafx.scene.control.Button?> <?import javafx.scene.control.Label?> <?import javafx.scene.control.ListView?> <?import javafx.scene.control.TextField?> <?import javafx.scene.layout.Pane?> <?import javafx.scene.layout.VBox?> <?import javafx.scene.text.Font?> <Pane maxHeight="-Infinity" maxWidth="-Infinity" minHeight="-Infinity" minWidth="-Infinity" prefHeight="900.0" prefWidth="900.0" xmlns="http://javafx.com/javafx/17" xmlns:fx="http://javafx.com/fxml/1" fx:controller="com.project.project2scenes.Scene2Controller"> <children> <Button layoutX="415.0" layoutY="776.0" mnemonicParsing="false" onAction="#goBack" text="LogOut!" /> <Label alignment="CENTER" layoutX="229.0" layoutY="26.0" prefHeight="52.0" prefWidth="457.0" text="Loan Management System" textFill="#0a14d0"> <font> <Font size="33.0" /> </font> </Label> <VBox layoutX="73.0" layoutY="123.0" prefHeight="491.0" prefWidth="342.0"> <children> <ListView fx:id="listDetails" prefHeight="491.0" prefWidth="495.0" /> </children> </VBox> <Button layoutX="73.0" layoutY="633.0" mnemonicParsing="false" onAction="#fillList" prefHeight="24.0" prefWidth="128.0" text="View All Clients" /> <Button layoutX="73.0" layoutY="710.0" mnemonicParsing="false" onAction="#deleteClient" prefHeight="24.0" prefWidth="128.0" text="Delete Client" /> <Label layoutX="73.0" layoutY="86.0" prefHeight="24.0" prefWidth="238.0" text="Client List"> <font> <Font size="23.0" /> </font> </Label> <Button layoutX="73.0" layoutY="671.0" mnemonicParsing="false" onAction="#editClient" prefHeight="24.0" prefWidth="128.0" text="Edit Client Info" /> <Label layoutX="504.0" layoutY="82.0" prefHeight="40.0" prefWidth="300.0" text="Update Info"> <font> <Font size="18.0" /> </font> </Label> <Label layoutX="444.0" layoutY="233.0" prefHeight="35.0" prefWidth="150.0" text="Item Type :"> <font> <Font size="18.0" /> </font> </Label> <Label layoutX="444.0" layoutY="292.0" prefHeight="44.0" prefWidth="150.0" text="Item Price : "> <font> <Font size="18.0" /> </font> </Label> <Label layoutX="440.0" layoutY="369.0" prefHeight="44.0" prefWidth="150.0" text="Loan Balance :"> <font> <Font size="18.0" /> </font> </Label> <Label layoutX="444.0" layoutY="446.0" prefHeight="44.0" prefWidth="104.0" text="Payment :"> <font> <Font size="18.0" /> </font> </Label> <TextField fx:id="itemType" layoutX="625.0" layoutY="233.0" prefHeight="35.0" prefWidth="165.0" /> <TextField fx:id="itemPrice" layoutX="625.0" layoutY="301.0" prefHeight="35.0" prefWidth="165.0" /> <TextField fx:id="loanBal" layoutX="625.0" layoutY="374.0" prefHeight="35.0" prefWidth="165.0" /> <TextField fx:id="Payment" layoutX="625.0" layoutY="450.0" prefHeight="35.0" prefWidth="165.0" /> <Label layoutX="444.0" layoutY="170.0" prefHeight="29.0" prefWidth="150.0" text="Client Selected :"> <font> <Font size="18.0" /> </font> </Label> <Label fx:id="nameUserSelected" layoutX="625.0" layoutY="162.0" prefHeight="40.0" prefWidth="214.0"> <font> <Font size="18.0" /> </font> </Label> <Button fx:id="updateClient" layoutX="440.0" layoutY="528.0" mnemonicParsing="false" prefHeight="35.0" prefWidth="182.0" text="Update Info" onAction="#updateInfo"> <font> <Font size="17.0" /> </font> </Button> </children> </Pane>
Output:
We hope you liked our article on Loan Management System Project in Java. If you have any queries, please comment.
Thank you for reading this article.
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Supply Chain Management System in Java
- Survey Management System In Java
- Phone Book in Java
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- CRUD Operations in Servlet & JSP
- 100+ Java Projects for Beginners 2023
- Airline Reservation System Project in Java
- Password and Notes Manager in Java
- GUI Number Guessing Game in Java
- How to create Notepad in Java?
- Memory Game in Java
- Simple Car Race Game in Java
- ATM program in Java
- Drawing Application In Java
- Tetris Game in Java
- Pong Game in Java
- Hospital Management System Project in Java
- Ludo Game in Java
- Restaurant Management System Project in Java
- Flappy Bird Game in Java
- ATM Simulator In Java
- Brick Breaker Game in Java
- Best Java Roadmap for Beginners 2023