
Introduction
Hello everyone, welcome to violet-cat-415996.hostingersite.com. In this article, we are going to learn to draw a dell logo using python turtle module. This is a very simple and easy design that is easily understandable to all python beginners. The code is explained line by line for easy understanding. The comments are provided in the code for documentation and understanding of each block of code.
For the complete code, you can go to the bottom of the page where you will get the entire code with the output.
So let’s begin to draw a dell logo
Import Turtle
import turtle t=turtle.Turtle()
Here, we import the turtle module to access its methods and functions in the project. We have created the object t of the turtle.
Draw the circle of the dell logo
t.pensize(15)
t.color(“#3287c1″,”#3287c1”)
t.penup()
t.goto(20,-200)
t.pendown()
t.circle(180)
t.penup()
In this piece of code, we are drawing the circle of the dell logo keeping the pensize of 15. The pen color and color are the same i.e #3287c1. The position of the circle is initialized to goto(20,-200). The circle is of a radius of 180.
Draw the ‘D’of the dell logo
#Draw the "D" of the DELL logo t.pensize(2) t.goto(-130,-70) t.pendown() t.setheading(90) t.forward(90) t.right(90) t.forward(50) t.penup() t.goto(-130,-70) t.setheading(0) t.pendown() t.forward(50) t.left(10) t.circle(46,165) t.penup() #Draw the inner D outline t.color("#3287c1") t.goto(-130,-70) #points direction of the logo to east t.setheading(0) t.pendown() t.begin_fill() t.forward(20) t.left(90) t.forward(90) t.left(90) t.forward(20) t.end_fill() t.backward(20) t.left(90) t.forward(17) t.setheading(0) t.begin_fill() t.forward(30) t.right(25) t.circle(-30,130) t.setheading(180) t.forward(30) t.left(90) t.forward(17) t.setheading(0) t.forward(30) t.left(10) t.circle(45,165) t.setheading(180) t.forward(47) t.end_fill() t.penup()
In this code block, we have to draw the ‘D of the Dell logo. The position is initialized to goto(-130,-70). The pen size is now changed to 2. The color is initialized to “#3287c1”.Here we have to make use of some inbuilt functions of the turtle.
Penup()=Turtle doesn’t write anything if you use the penup() function.
Pendown()=Turtle operates in pendown() function by default
Setheading()=Turtles pointing direction
Setheading(0)=points to east
Setheading(180)=points to west
Setheading(270)=points to south
Setheading(90)=points to north
Right()=moves the turtle to the right side with the specified angle
Left()=moves the turtle to the left side with the specified angle.
Begin_fill()= Starts filling the object with the specified color.
End_fill()=The end where the color filling completes.
Draw the ‘E’ of the Dell logo
#Draw the ‘E’ of the DELL logo
t.goto(-45,-15)
t.pendown()
t.setheading(0)
t.left(35)
t.begin_fill()
t.forward(65)
t.right(90)
t.forward(18)
t.right(90)
t.forward(50)
t.left(90)
t.forward(10)
t.left(90)
t.forward(50)
t.right(90)
t.forward(18)
t.right(90)
t.forward(50)
t.left(90)
t.forward(10)
t.left(90)
t.forward(50)
t.right(90)
t.forward(18)
t.right(90)
t.forward(65)
t.right(85)
t.forward(65)
t.penup()
t.end_fill()
In this piece of code, we have initialized the position of the turtle to goto(-45,-15). Initialized the turtle to setheading(0) and an angle left(35). Started drawing E with a distance of 65. The angle of right(90) and draw the small lines in E with a distance of 20. Inner lines in ‘E’ is provided with a distance of 50.
Draw the ‘L’of the Dell logo
#Draw the ‘L’ of the DELL logo
t.goto(40,-70)
t.setheading(90)
t.pendown()
t.begin_fill()
t.forward(90)
t.right(90)
t.forward(20)
t.right(90)
t.forward(70)
t.left(90)
t.forward(40)
t.right(90)
t.forward(20)
t.right(90)
t.forward(60)
t.end_fill()s
t.penup()
In this piece of code, we have to draw the L logo of the dell using a python turtle. The position is initialized to goto(40,-70). The L is drawn by providing the specified distance and angle.
Draw the 2nd ‘L’ of the DELL Logo
#Draw the ‘l’ of the DELL Logo
t.goto(110,-70)
t.setheading(90)
t.pendown()
t.begin_fill()
t.forward(90)
t.right(90)
t.forward(20)
t.right(90)
t.forward(70)
t.left(90)
t.forward(40)
t.right(90)
t.forward(20)
t.right(90)
t.forward(60)
t.end_fill()
t.penup()
Here, we have to draw the 2nd L of the dell Logo. It is drawn in a similar way as the first ‘L’ the only change is in the position of the turtle i.e goto(110,-70).
Complete Code to Draw Dell Logo using Python Turtle
import turtle t=turtle.Turtle() t.pensize(15) t.color("#3287c1","#3287c1") t.penup() t.goto(20,-200) t.pendown() t.circle(180) t.penup() #Draw the "D" of the DELL logo t.pensize(2) t.goto(-130,-70) t.pendown() t.setheading(90) t.forward(90) t.right(90) t.forward(50) t.penup() t.goto(-130,-70) t.setheading(0) t.pendown() t.forward(50) t.left(10) t.circle(46,165) t.penup() t.color("#3287c1") t.goto(-130,-70) t.setheading(0) t.pendown() t.begin_fill() t.forward(20) t.left(90) t.forward(90) t.left(90) t.forward(20) t.end_fill() t.backward(20) t.left(90) t.forward(17) t.setheading(0) t.begin_fill() t.forward(30) t.right(25) t.circle(-30,130) t.setheading(180) t.forward(30) t.left(90) t.forward(17) t.setheading(0) t.forward(30) t.left(10) t.circle(45,165) t.setheading(180) t.forward(47) t.end_fill() t.penup() #Draw the 'E' of the DELL logo t.goto(-45,-15) t.pendown() t.setheading(0) t.left(35) t.begin_fill() t.forward(65) t.right(90) t.forward(18) t.right(90) t.forward(50) t.left(90) t.forward(10) t.left(90) t.forward(50) t.right(90) t.forward(18) t.right(90) t.forward(50) t.left(90) t.forward(10) t.left(90) t.forward(50) t.right(90) t.forward(18) t.right(90) t.forward(65) t.right(85) t.forward(65) t.penup() t.end_fill() #Draw the 'L' of the DELL logo t.goto(40,-70) t.setheading(90) t.pendown() t.begin_fill() t.forward(90) t.right(90) t.forward(20) t.right(90) t.forward(70) t.left(90) t.forward(40) t.right(90) t.forward(20) t.right(90) t.forward(60) t.end_fill() t.penup() #Draw the 'l' of the DELL Logo t.goto(110,-70) t.setheading(90) t.pendown() t.begin_fill() t.forward(90) t.right(90) t.forward(20) t.right(90) t.forward(70) t.left(90) t.forward(40) t.right(90) t.forward(20) t.right(90) t.forward(60) t.end_fill() t.penup() t.hideturtle() turtle.done()
Output
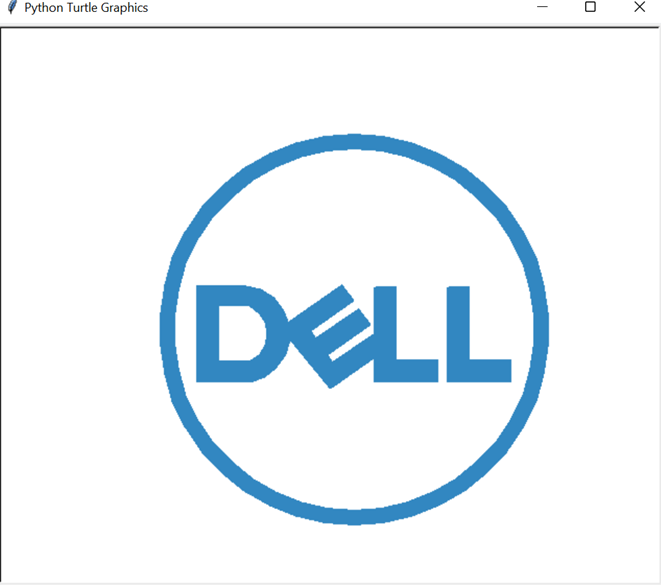
In this way, we have successfully completed the drawing of the DELL logo using the python Turtle module.
Also Read:
- Simple Code to compare Speed of Python, Java, and C++?
- Falling Stars Animation on Python.Hub October 2024
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value