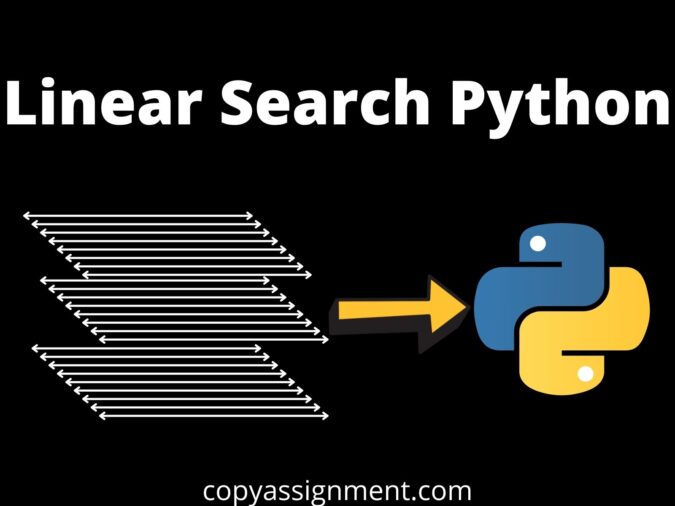
Introduction
Linear Search in Python is quite different and much simpler as compared to Binary Searching in Python. Linear Searching in Python involves going through every element until we find the desired element. It is also known as sequential searching. So, in this article, we are going to create a linear search python program.
Considering that python is a generally slow language, this type of search takes a long time to run when compared to Binary Search. This is the only disadvantage to this form of searching. On the upside, Linear Search in Python can be used to search in a list that is not sorted.
There are a number of ways of implementing this program.
1: Index Linear Search in Python
This method simply means we check elements following the index sequence of the array or the list. Hence it will iterate over the list until we run out of elements to search. It is very simple right!
However, this will only search for an instance of one element in the list. It will return the position of the first instance of the element in the list. If there are two instances of the element, the second one or any other for that matter, won’t be indicted.
# Index Linear search in python def linearSearch1(values, element): # This function takes two parameters. # The first is the list or array that acts as a dictionary to be searched in. # The second is the element being searched. for position in range(0, (len(values))): # We implement a for loop to iterate over the list. if values[position] == element: # We check if the element at that particular index is the element being searched for. # If it is, then we return the position return "Element " + str(element) + " is at " + str(position + 1) #If we fail to get the element in the list, we return the following statement. return "Element " + str(element) + " is not in the list."
2: Index Linear Search in Python
This Linear Search Python program method is quite similar to the previous one. In simple terms, the only difference is that it will be used to obtain positions of elements that are multiple in the list or array.
In order to do this, we have to remove the return statements. This is because the return statement breaks the loop whenever we find an element. In place of the return statement, we use a print statement. In order to maintain the functionality of the function, we implement a True-False statement.
This means that initially, the state of the function is False. If an element or more is found, the state is changed to True and the selection statement continues and if another element is found, its position is printed out. If the element is not found, then the state of the function remains False. An if statement at the end checks for the state of the function. If it is still False then the element is not in the list and we print the appropriate statement.
# Index linear search in python. def linearSearch2(values, element): # The function takes two paramaters. # The first is the list that the element will be searched within. # The second is the element that is being looked for. found = False # The state of the function is defined here. # It will be False as long as no instance of the element has been found. for position in range(0, (len(values))): # We use a for loop to iterate over the elements in the list. if values[position] == element: # We then check if the Element is indeed the element being looked for. # If so, then the state of the function changes to True found = True print("Element ", element, " is at ", (position+1)) # We print out the position(s) of the element. # If we didn't find any instance of the element, then the state of the function remains as False if found == False: # We print out the following statement then. print("Element ", element, "is not in the list.")
3: Non-iterated Linear Search in Python
This is a method of Linear Search Python program that will involve iteration as the name suggests. This means that we won’t be iterating over the list. Therefore, we won’t implement a for loop as was the case before.
Instead, we will implement a while loop. This means that the function will run as long as some conditions are met. One condition is mandatory. This is the domain of the function. The function will run as long as we have not exhausted the list. The length of the list will be taken and a counter will be incremented until it is equal to the length of the array.
We will introduce a state statement to define whether the element was found or not.
The second condition is the state statement. It can be used if only one instance of the element is being searched. It is omitted in several instances of the element might or will be present in the list or array.
# Unindexed linear Search in Python. def linearSearch3(values, element): # The function takes in two parameters. # The first is the list and the second is the element being looked for. found = False # We define the state of the function. position = 0 # This is where the search will begin in the array. while position < len(values): # We have implemented a while statement. # Since we want to make it able for the function to search for multiple instances of the element. # We have omited the state as one of the conditions being checked. if values[position] == element: # We check if the element of the value is the element being searched. print("Element ", element, " is at ", position+1) # We print out its position position += 1 # The value of the position is incremented to move to the next element. found = True # The state of the function is changed to True is an instance of the element has been found. else: # We increment the postion to move to the next element in the list. position += 1 # If no instance of the element was found in the list, then the state remains as False. if found == False : print("Element ", element, " is not in the list.")
Thank you for reading through my post.
Leave a comment on any query you might have.
You can check other posts below:
YouTube Video Downloader in Python