
Introduction
Command line applications often return text in the same color as the terminal. There are times when we wish to draw the user’s attention to output, such as a warning or error message. In certain circumstances, a splash of color may make all the difference. This article demonstrates how to Print Colored Text Output in Python terminal using and without libraries.
Using various colors, text may be represented in the Python programming language. Python utilities for colors and formatting in the terminal are pretty easy to use. The coder uses colorful text to gain better results.
In this article, we are presenting 6 ways to get colorful text in your terminal. Let’s look at a few practical Python examples of text coloring.
1. Python command to print color text using Simple-color
2. Python command to print color text using ANSI Escape Sequence
3. Python command to print color text using Termcolor Module
4. Python command to print color text using Colorma Module
5. Python command to print color text using Colored Module
6. Python command to print color text using Creating an HTML Object
1. Print Colored Text Output in Python terminal using Simple-color
Python has a fantastic module called “simple-color” that allows us to show colorful text on the terminal.
Blue, black, green, magenta, red, yellow, and cyan are among the colors included in the simple colors package.
You may also format your text using the supplied styles such as bold, dim, italic, brilliant, underlined, reverse, and blink.
Because the simple-color package is not included in Python’s standard library, you must install it before using it. Copy the following code into your terminal to install the simple color package:
pip install simple-colors
Or
python -m pip install simple-colors
After successfully installing the module, you may customize/style your code by using the syntax shown in the sample below.
The following example shows how to use the simple colors module to add color, format text, make it bold, or even underline it.
import simple_colors
# colored text
print('Normal:', simple_colors.blue('Welcome at Copyassignment.com!'))
# BOLD and colored text
print('BOLD: ', simple_colors.green('Welcome at Copyassignment.com!', 'bold'))
# BOLD and Underlined and colored text
print('BOLD and Underlined: ', simple_colors.red('Welcome at Copyassignment.com!', ['bold', 'underlined']))
The output of the simple_color Module:
How it Works!
We utilized the specified color technique and gave the kind of formatting (bold, italic, underline, etc.) as an input to the color function to apply styles and colors to the text. To add several formatting styles to your text, just send the formatting styles as input to the function by packing them within a list.
2. Print Colored Text Output in Python using ANSI Escape Sequence
You may utilize ANSI escape codes to alter the color of the text output in a Python application, which will make your text more readable and artistic. The highlighting of mistakes is an excellent use for this. The print statement is immediately updated with the escape codes.
To print colored text in Python, use the specific escape sequence print("\033[48;5;236m")
.
In the terminals, two color schemes are commonly used:
- 16 colors (8 for the background and 8 for the foreground)
- 256 different colors
Let’s start with the 16-color option and color our output
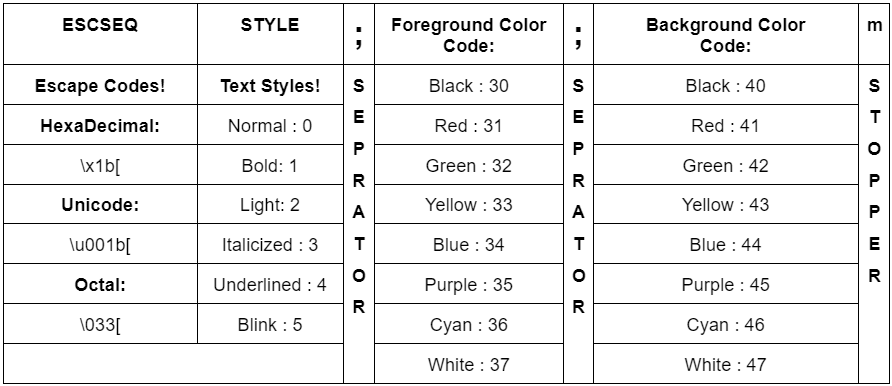
To put this to the test, print a tacky color pattern with red strong writing and a yellow backdrop. Bold text is represented by style code 2. The color codes for the red text in the foreground are 31 and 43 for the yellow backdrop. With that in mind, the syntax for describing this layout is as follows:
print('\033[2;31;43m CopyAssignment.com \033[0;0m')
This result will be displayed:
256 different colors:
We can dive in right after we understand the syntax of a 256-color scheme. Working with 256 colors is a bit different than working with the 16-color scheme:
Next, let’s explore some additional justifications for why this works.
Some terminals allow you to enter specific escape sequences to change the printed material’s tone, color, and look. These escape sequences are known as ANSI escape sequences because of the ANSI standard that specifies their application.
As a result, you may generate colored material by using the built-in ANSI escape sequence. The ANSI sequences that you can utilize in your code are broken down as follows:

38;5; is used for the text background and 48;5; is used for the foreground, depending on the placeholder, which decides whether the color should be applied to the text or the text background.
This is followed by a color code ranging from 0 to 255.
In our interactive Jupyter notebook, you can test this out for yourself:
ca = "CopyAssignment.com"
print("Uncoloured text: ", ca)
colored_s = "\033[38;5;4m" + ca
print("Coloured text: ", colored_s)
The Output of the ANSI Escape Sequence:
3. Print Colored Text Output in Python using Termcolor Module
A Python library called termcolor is used for ANSII color formatting.
The module has specific text formatting attributes as well as different properties for various terminals. Additionally, it has several font hues like blue, red, and green as well as text highlights like magenta, cyan, and white.
The termcolor module is not included in the core Python library. As a result, installing it is necessary before using it. Copy the following code to your terminal in order to install the termcolor module:
pip install termcolor
Let’s visualize how you can use the module to print colorful text once you’ve installed it.
Code:
from termcolor import colored
text = colored('Welcome at Copyassignment.com!', 'green’', attrs=['bold'])
print(text)
The output of The Termcolor Module:
4. Print Colored Text Output in Python using Colorma Module
Colorama does nothing on other platforms. Calling init() in Windows will replace the ANSI escape sequence of any material given to stdout or stderr with Win32 calls.
Call deinit to leave Colorama before your program ends(). It will set stdout and stderr back to their original values.
The following command must be used in your terminal to install coloroma, which is not part of Python’s standard library.
pip install colorama
To further understand how the Python Colorama module may be used to print bold text, let’s look at an example.
from colorama import Fore, Style
print(Style.BRIGHT + 'Bold text')
print(Fore.RED + 'Printing Red colored text')
print(Style.RESET_ALL)
print('This line has no effect of coloroma')
The Output of the Colorma Module:
Another example of using the termcolor module and the coloroma package together.
from colorama import init
from termcolor import colored
init()
print(colored('Hello and Welcome to at Copyassignment.com!', 'green', attrs=['bold']))
Output:
5. Print Colored Text Output in Python using Colored Module
A very basic Python package called colored is used to format and color text on terminals. It must first be installed using pip because it is not a built-in module:
pip install colored
Example of Use:
from colored import fore, back, style
print (fore.RED + back.YELLOW + style.BOLD + "Hello at Copyassignment.com!!!" + style.RESET)
The Output of print color text using Colored Module:
6. Print Colored Text Output in Python by Creating an HTML Object
A print formatted text() method in the Prompt toolkit is (as much as is practicable) compatible with the built-in print() function. Additionally, it enables formatting and colors.
It is possible to show that a string includes HTML-based formatting by using HTML. As a result, the HTML object understands the <b>, <i>, and <u> tags, which are required for bold, italic, and underline.
But first, you need to install the package:
pip install prompt-toolkit
Source Code To Implement:
from prompt_toolkit import print_formatted_text, HTML
print_formatted_text(HTML('<b>This text is bold</b>'))
print_formatted_text(HTML('<i>This text is italic</i>'))
print_formatted_text(HTML('<u>This text is underlined</u>'))
The Output of print color text using Creating an HTML Object:
Another Example: As all the HTML tags are mapped to classes from a style sheet, you can also appoint a style for a custom tag.
from prompt_toolkit import print_formatted_text, HTML
from prompt_toolkit.styles import Style
sty = Style.from_dict({'y': '#44ff00 bold',})
print_formatted_text(HTML('<red> Hello and welcome to <u>at Copyassignment.com!</u></red>'), style=sty)
Output:
That’s all for this tutorial, thank you for reading this article.
Also Read:
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- MoviePy: Python Video Editing Library
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter