import a_module
Now, you can use this ‘a_module’ inside your program but do you know where Python looks for modules or retrieve a module path? Let’s check.
There are 3 places where Python checks for Python modules:
- Current directory: When you run any program then initially Python looks for modules in the directory in which you are running the program.
- PYTHONPATH: It is an environment variable set by the user when the user installs Python in the system. Click here to know more about PYTHONPATH.
- Other Python directories: If Python fails to find the required modules in the above two, then it checks in other Python directories that were configured at the time of installation of Python.
Try this code:
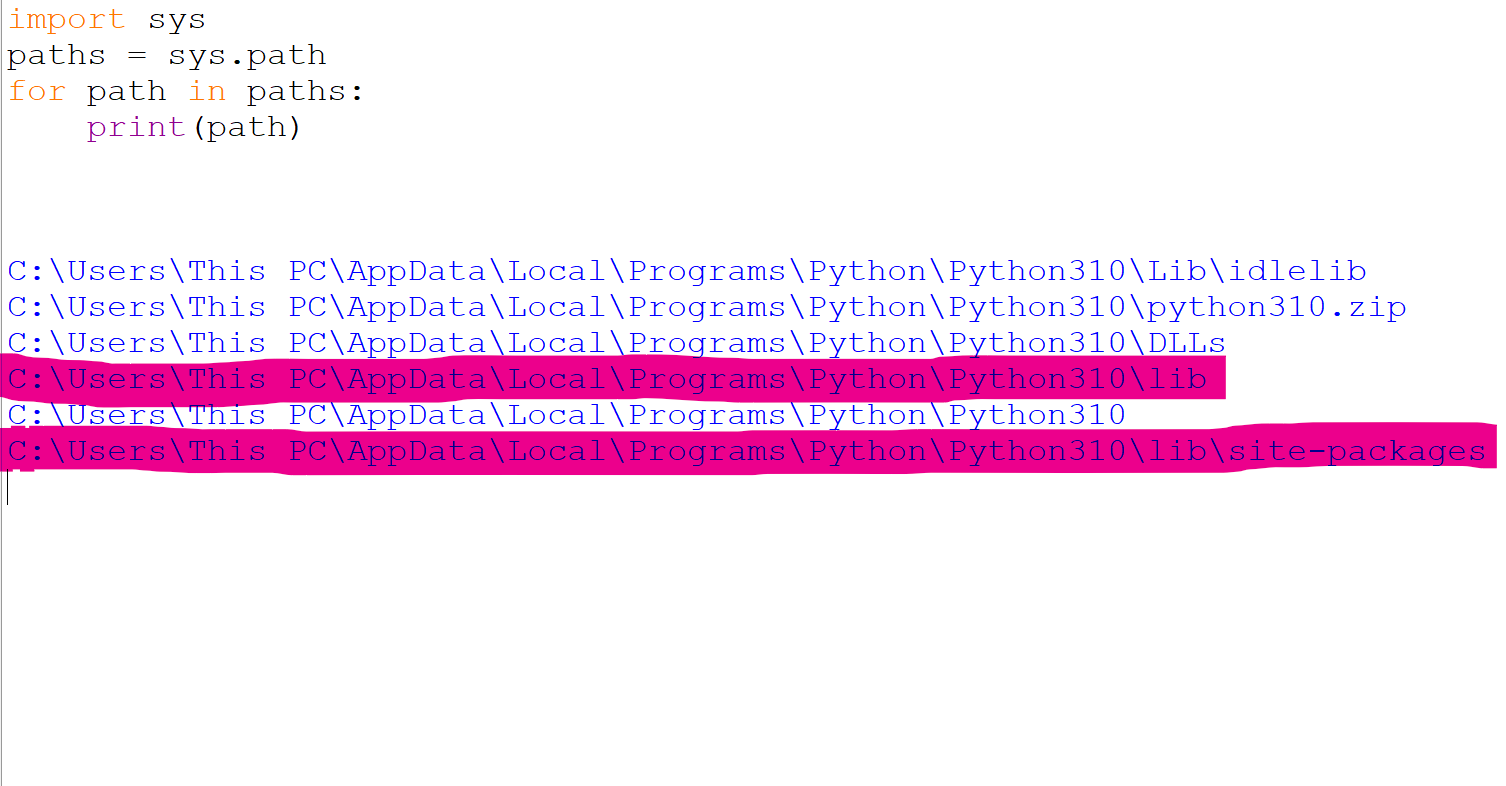
Python looks for modules or retrieve a module path mainly from the given below paths:
‘C:\Users\This PC\AppData\Local\Programs\Python\Python310\lib’
‘C:\Users\This PC\AppData\Local\Programs\Python\Python310\lib\site-packages’
Code to get the path where Python looks for modules or retrieve a module path:
Method 1: Using sys module
import sys
paths = sys.path
for path in paths:
print(path)
Output:
C:\Users\This PC\AppData\Local\Programs\Python\Python310\Lib\idlelib
C:\Users\This PC\AppData\Local\Programs\Python\Python310\python310.zip
C:\Users\This PC\AppData\Local\Programs\Python\Python310\DLLs
C:\Users\This PC\AppData\Local\Programs\Python\Python310\lib
C:\Users\This PC\AppData\Local\Programs\Python\Python310
C:\Users\This PC\AppData\Local\Programs\Python\Python310\lib\site-packages
Method 2: Using os module
import random
path = os.path.abspath(random.__file__)
print(path)
This method will print the absolute path of the module.
Output:
'C:\Users\This PC\AppData\Local\Programs\Python\Python310\lib\random.py'
You can use the method below to print the path to the directory of the module.
import random
path = os.path.dirname(random.__file__)
print(path)
Output:
'C:\Users\This PC\AppData\Local\Programs\Python\Python310\lib'
Another method is using the help() method of the os module, this method will give 1521 lines of output and the path of the os module will be given at the end of all lines.
import os
print(help(os))
Output:
Help on module os:
NAME
os - OS routines for NT or Posix depending on what system we're on.
........
........
........
FILE
c:\users\this pc\appdata\local\programs\python\python310\lib\os.py
Method 3: Using importlib module
This method is fast because it retrieves the module path without loading it.
import importlib.util
print(importlib.util.find_spec("os"))
Output1:
ModuleSpec(name='os', loader=<_frozen_importlib_external.SourceFileLoader object at 0x000001F3E8495690>, origin='C:\\Users\\This PC\\AppData\\Local\\Programs\\Python\\Python310\\lib\\os.py')
import importlib.util
print(importlib.util.find_spec("os").origin)
Output2:
C:\Users\This PC\AppData\Local\Programs\Python\Python310\lib\os.py
If the module/ library is not installed in your system, this method will through AttributeError.
import importlib.util
print(importlib.util.find_spec("requests").origin)
Output3:
Traceback (most recent call last):
File "<pyshell#25>", line 1, in <module>
print(importlib.util.find_spec("requests").origin)
AttributeError: 'NoneType' object has no attribute 'origin'
Method 4: Using inspect module
import inspect
import os
print(inspect.getfile(os))
Output:
C:\Users\This PC\AppData\Local\Programs\Python\Python310\lib\os.py
Method 5: Directly printing module
import os, random
print(os)
print(random)
Output:
<module 'os' from 'C:\\Users\\This PC\\AppData\\Local\\Programs\\Python\\Python310\\lib\\os.py'>
<module 'random' from 'C:\\Users\\This PC\\AppData\\Local\\Programs\\Python\\Python310\\lib\\random.py'>
Thank you for visiting our website.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter