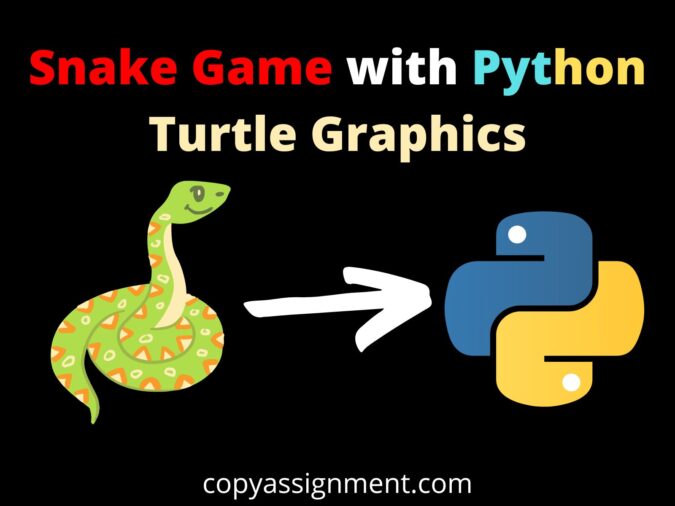
In this tutorial, we will learn how to build the Snake Game with Python Turtle Graphics Module. By the end of this tutorial, you will learn about all the basic concepts of Python and advanced topics of python turtle. First, we will see the explanation where we will discuss the topic and then the actual code.
Requirements:
We will need the turtle module which is built-in with Python. The next module we need is the “random” module which will be used to get a random coordinate to keep the food of the snake. However, if you get the NoSuchModuleError, try the following command in the terminal.
First Part:
- First, import the “turtle” and the “random” module. Then, set the geometry of the screen as w = 500, h = 500, fs = 10 and d = 100. Likewise, create a dictionary name “offsets” where there will be four name and values will be given in coordinates. Set the names and values as { “up”: (0, 20), “down”: (0, -20), “left”: (-20, 0), “right”: (20, 0) }
The Snake Function:
- Likewise, create a function named r() and declare “saap“, “kata“, “khanaT“, “pen“. Then create a list of lists as [[0, 0], [0, 20], [0, 40], [0, 60], [0, 80]]. Similarly, set the kata to “up” and store nun() in khanaT. Position the food to khanaT and call the hall() function.
- Similarly, create a function named hall(). Inside this function, set a global variable name “kata“. Then, set a new_head variable to sapp[-1].copy() and so on as in the code below.
new_head = saap[-1].copy()
new_head[0] = saap[-1][0] + offsets[kata][0]
new_head[1] = saap[-1][1] + offsets[kata][1]
- Create an if else condition where r() will be called if new_head is in sapp[:-1]. Else, the current will be appended to sapp. If it is not khana() pop the value in the first index. Create another condition where if saap[-1][0] greater than w / 2, subtract w from saap[-1][0]. Else if saap[-1][0] less than – w / 2 , add w to saap[-1][0]. Else if saap[-1][1] greater than h / 2, subtract h from saap[-1][1]. At last if saap[-1][1] less than -h / 2, add h to saap[-1][1].
- Clear all the stamps and create a for loop that will loop through saap. Inside this loop, make the turtle go to the first index of the segment as “x” and the second index of the segment as “y” and stamp with the pen.
- Update the screen and call the ontimer method of the turtle with the arguments (hall, d)
Food and Positioning Part:
- Create a function name khana() and set the khanaT variable as global. If dist(saap[-1], khanaT) is less than 20, store the nun() function in khanaT and position the “food” variable to khanaT and return Ture else return False.
- Create another function named nun(). In this function, we will declare the position of the food for the snake with the following code.
x = random.randint(- w / 2 + fs, w / 2 - fs)
y = random.randint(- h / 2 + fs, h / 2 - fs)
return (x, y)
- Create another function named dist() with the parameters “poos1” and “poos2“. Inside this function, set the poos1 as x1 and y1 and poos2 as x2 and y2. Declare the variable “distance” as ((y2 – y1) ** 2 + (x2 – x1) ** 2) ** 0.5 and return the distance.
Moves of the snake:
- Create a function named mathi(). Inside this function, set a global variable kata and if kata is not “down”, set kata as “up”.
- Likewise, create another function named go_right(). Inside this function, set a global variable kata and if kata is not “left”, set kata as “left”.
- Similarly, create another function named go_down(). Inside this function, set a global variable kata and if kata is not “up”, set kata as “down”.
- Accordingly, create another function named go_left(). Inside this function, set a global variable kata and if kata is not “right”, set kata as “left”.
Main Code as Last Part:
- Declare a variable named screen as the screen of the turtle. Set the screen’s geometry up as the (w, h). Set the title of the turtle to “Snake Game” and the background color to “green”. Again, condition the setup to (500, 500). Call the tracer method with the argument “0”.
- Declare a variable name “pen” as the actual turtle and set the shape of the turtle as “square”. Pick the pen up as we are not ready to draw.
- Declare a variable named “food” and set it as a turtle. Set the shape of the turtle as “circle” and the color of the turtle as “white”. Call the shapesize method with the argument (fs / 20). Pick the pen up as we are not ready to draw.
- Then, call the listen() method to focus on the TurtleScreen. After the focus, to record the KeyEvents, call the onkey() method with the arguments (mathi, “up”), screen.onkey(go_right, “Right”), screen.onkey(go_down, “Down”), screen.onkey(go_left, “Left”).
- Lastly, call the r() function and end the code with the method turtle.done()
Complete code for Snake Game with Python Turtle Graphics:
import turtle import random w = 500 h = 500 fs = 10 d = 100 # milliseconds offsets = { "up": (0, 20), "down": (0, -20), "left": (-20, 0), "right": (20, 0) } def r(): global saap, kata, khanaT, pen saap = [[0, 0], [0, 20], [0, 40], [0, 60], [0, 80]] kata = "up" khanaT = nun() food.goto(khanaT) hall() def hall(): global kata new_head = saap[-1].copy() new_head[0] = saap[-1][0] + offsets[kata][0] new_head[1] = saap[-1][1] + offsets[kata][1] if new_head in saap[:-1]: r() else: saap.append(new_head) if not khana(): saap.pop(0) if saap[-1][0] > w / 2: saap[-1][0] -= w elif saap[-1][0] < - w / 2: saap[-1][0] += w elif saap[-1][1] > h / 2: saap[-1][1] -= h elif saap[-1][1] < -h / 2: saap[-1][1] += h pen.clearstamps() #clears all the stamps for segment in saap: pen.goto(segment[0], segment[1]) pen.stamp() screen.update() #updates the turtle.screen screen turtle.ontimer(hall, d) def khana(): global khanaT if dist(saap[-1], khanaT) < 20: khanaT = nun() food.goto(khanaT) return True return False def nun(): x = random.randint(- w / 2 + fs, w / 2 - fs) y = random.randint(- h / 2 + fs, h / 2 - fs) return (x, y) def dist(poos1, poos2): x1, y1 = poos1 x2, y2 = poos2 distance = ((y2 - y1) ** 2 + (x2 - x1) ** 2) ** 0.5 return distance def mathi(): global kata if kata != "down": kata = "up" def go_right(): global kata if kata != "left": kata = "right" def go_down(): global kata if kata != "up": kata = "down" def go_left(): global kata if kata != "right": kata = "left" screen = turtle.Screen() screen.setup(w, h) screen.title("saap") screen.bgcolor("green") screen.setup(500, 500) screen.tracer(0) pen = turtle.Turtle("square") pen.penup() food = turtle.Turtle() food.shape("circle") food.color("white") food.shapesize(fs / 20) food.penup() screen.listen() screen.onkey(mathi, "Up") screen.onkey(go_right, "Right") screen.onkey(go_down, "Down") screen.onkey(go_left, "Left") r() turtle.done()
Output:

Thank You for reading till the end. If you have any queries or confusion, just comment it down. We also have a Beginner’s Guide to Python Turtle. We even have other minor and medium projects on Python Turtle on this website.
Keep Learning, Keep Coding
Also Read:
- Radha Krishna using Python Turtle
- Drawing letter A using Python Turtle
- Stickman Game in Python
- Brick Breaker Game in Python
- Wishing Happy New Year 2023 in Python Turtle
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Draw Goku in Python Turtle
- Draw Mickey Mouse in Python Turtle
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Happy Diwali in Python Turtle
- Draw Halloween in Python Turtle
- Write Happy Halloween in Python Turtle
- Draw Happy Diwali in Python Turtle
- Tetris game in Python Code
- Extract Audio from Video using Python
- Drawing Application in Python Tkinter
- Draw Flag of USA using Python Turtle
- Draw Iron Man Face with Python Turtle: Tony Stark Face
- Draw TikTok Logo with Python Turtle
- Python Games Code | Copy and Paste
- Draw Instagram Logo using Python Turtle
- I Love You Text in ASCII Art
- Python Turtle Shapes- Square, Rectangle, Circle
- Python Turtle Commands and All Methods