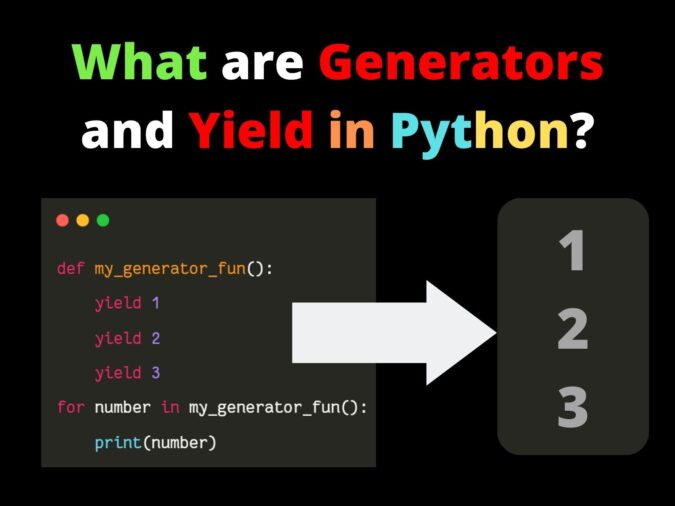
Hello friends, in this article we will see how can we convert a generator to a list in Python. We will see all the methods and will also provide an easy and simple explanation to Convert Generator To List In Python.
What are Generators in Python?
The generator can be an object or a function that returns a generator object. So, we can say a generator is of two types- Generator-function and Generator-object.
Generator function
A generator function uses the yield(not return) keyword to return a value. So, in python, if a function uses the yield keyword, it automatically becomes a Generator function.
We can iterate over the values returned by the Generator function with a loop
Example:
def my_generator_fun():
yield 1
yield 2
yield 3
for number in my_generator_fun():
print(number)
Output:
1
2
3
Generator Object
A Generator object is an iterable object in Python like lists. A Generator object is the overall return value of a Generator function in Python. We can use the next() function to access the values of a Generator object.
Example:
def my_generator_fun():
yield 1
yield 2
yield 3
my_generator_object = my_generator_fun()
print(next(my_generator_object))
print(next(my_generator_object))
print(next(my_generator_object))
Output:
1
2
3
Using next() over List
We will get the error if we will use next() with lists. We will get an error because next() works with iterators only.
Example:
my_list = [1,2,3]
print(next(my_list))
print(next(my_list))
print(next(my_list))
Output:
>>> %Run test.py Traceback (most recent call last): File "D:\run for test\test.py", line 3, in <module> print(next(my_list)) TypeError: 'list' object is not an iterator
Video Tutorial for Generators in Python
Conclusion
In this article, we learned What are generators, How to use generator functions in Python? How to use Generator Objects in Python? What is the use of the Yield keyword in Generator functions in Python? We hope this article on Generators will explain most of the concepts related to generators in Python you will ever need now or in the future.
Thank you for reading this article.
Also Read:
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- MoviePy: Python Video Editing Library
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter