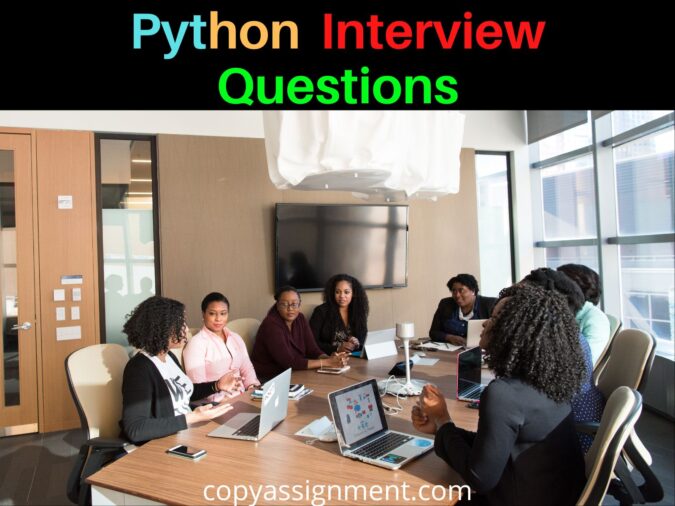
In Python Interview Questions and Answers, we will see frequently asked questions that are asked in most of the Python Interviews. We will also give you a proper explanation for all python programming questions.
We request you to understand all python questions in deep because any of these questions you may be asked in your interviews.
Nearly all of the frequently asked python interview questions for 2022 have been addressed. We offer more than 25+ questions about Python programming that may be used by people of all skill levels to help you get the most out of our site. This post is for both experienced and inexperienced people, and it will undoubtedly aid you in preparing for interviews. We have divided this article into 3 categories. So let’s rub our hands on these questions.
- Beginners Level Python Interview Questions
- Intermediate Level Python Interview Questions
- Advanced Level Python Interview Questions
Beginners Level Python Interview Questions And Answers
This set is designed keeping in mind the concepts known to beginners. These python developer interview questions are especially for the freshers and we believe these are the most frequently asked questions on python. This set of python interview questions for freshers includes questions on Built-in functions, Type conversion, List, Dictionary, etc.
1. What are Python namespaces and their types?
Answer: In a namespace-based system, each and every Python object has a special name. A variable or a method are examples of objects. It simply ensures that the names are unique. A Python dictionary serves as the namespace that Python itself maintains. Depending on the namespace, the Python interpreter can determine the specific method or variable one is trying to reference in the code. A namespace comes in three varieties:
- Global namespace
- Local namespace
- Built-in namespace
2. What is the zip() function used for?
Answer: The zip() function will return a zip object, which is an iterator of tuples where the first and second items in each provided iterator are coupled together, and so on. The iterator with the fewest elements determines the length of the new iterator if the lengths of the previous iterators were different.
t1 = ("CopyAssignment", "Interview")
t2 = ("Leader in Python", "Questions and Answers")
x = zip(t1, t2)
print(tuple(x))
Output:

3. Explain docstring usage with an example?
This question is one of the favorite questions of interviewers when it comes to python basic interview questions. Even I was asked this question in one of my python interviews.
Answer: A docstring is a string that is added as the object’s definition’s opening sentence. It is useful to describe the classes, methods, and other components. Docstrings are written immediately following the definition of the method and in between “”” and “””
def addfunc(a,b):
"""This function adds two numbers.This is docstring."""
print(a+b)
print(addfunc.__doc__)
addfunc(12,13)
Output:

4. Does Python allows type conversion. If yes then state some of the type conversion functions?
Answer: Yes !! Python offers the much-needed type conversion capability, which allows us to change one data type into the one we require. Some of the built-in functions are:
Functions | Description |
tuple() | Converts to a tuple |
dict() | Converts tuple to the dictionary |
str() | Converts into string |
int() | Converts into integer |
set() | Converts into set |
5. What’s the difference between remove() and del()?
This question is not the favorite question of interviewers of python but may be good for python interview questions for freshers.
Answer:
del: It is a keyword and it takes the index of the element that we want to delete. Please note that del is a keyword.
mylist = [11, 22, 33, 44, 55, 66, 77]
del mylist[4]
print("Element at index 4 to be deleted:")
print("After deletetion: ", mylist)
Output:

remove(): It is a method and it takes the element that is to be removed.
mylist = [11, 22, 33, 44, 55, 66, 77]
mylist.remove(44)
print("44 number is to be deleted:")
print("After removal: ", mylist)
Output:

6. Explain how you will make a Python script executable in UNIX?
Answer: As the script’s first line, insert the following:
#!/usr/bin/env python3
To make copyassignment.py executable, enter the following commands at the UNIX command prompt:
$ chmod +x copyassignment.py
7. Difference between bytes() and bytearray()?
Answer: bytes(): It can produce empty bytes objects of the desired size or transform items into bytes objects.
bytearray(): Simply returns an object but it is an array of bytes
prime_numbers = [2, 3, 5, 7]
byte_array = bytearray(prime_numbers)
print(byte_array)
Output:

Bytes() and bytearray() return different types of objects: bytes() returns an immutable object, whereas bytearray() returns an alterable object.
8. How will you read a random line from the file?
We found this question tricky for beginners and may be asked in python interview questions and answers.
Answer: We will use a choice() method. It simply returns any random element from the given sequence of elements. The sequence can be of any type, including strings, ranges, lists, tuples, and more.
import random
def readRandomLine(f):
lines = open(f).read().splitlines()
return random.choice(lines)
print("This is the random line read from the file:\n")
print(readRandomLine ('main.txt'))
Output:

Explanation:
Here, we imported a random library in the first line. Then, using the reading approach, we developed a function that opens a file. The splitlines() function divides the file’s content into lines. Now we accessed a random line using the random library’s choice() method.
9. Which of the below option will create a dictionary?
1) a = {}
2) b = {“john”:40, “peter”:45}
3) c = (40:”john”, 45:”50”)
4) d = {40:”john”, 45:”peter”}
Output:

Answer: 1,2 and 4 will create the dictionary. It is created using the key and value pairs
10. What is __init__ in Python?
This question is also one of the favorite questions of interviewers when it comes to python coding interview questions. We suggest you prepare this question really hard.
Answer: It is a Python method that is automatically invoked when any new object needs memory allocated. Initializing the new object’s properties is the goal of init. When creating an object, we utilize the init() function to set values to its properties and perform other essential tasks.
Intermediate Level Python Interview Questions
This set of questions includes all the python coding questions and answers that are suitable for the intermediate-level developer. These python developer interview questions include tricky concepts like Multithreading, File Handling, *args and **kwargs, etc
1. What will be your approach to adding two integers (>0) without the use of the plus operator and in less than 10 lines?
def adding_two_num(i1, i2):
while i2 != 0:
data = i1 & i2
i1 = i1 ^ i2
i2 = data << 1
return i1
print("Adding without using plus operator")
print(adding_two_num(12,15))
Output:
output for adding two integers (>0) without the + operator
Explanation:
Line 2: While loop
Line 3: Bitwise AND is used to get carry bit.
Line 4: Sum is calculated with XOR.
Line 5: << is a left shift operator. Its function is to move the bits to the left and also it fills the empty spaces with 0
2. Explain the map() function and illustrate it with code?
map() is a very important function in Python and has many uses, and is frequently asked in python programming questions.
Answer: The map() method takes an iterable and applies a specified function to each item. A list of the outcomes is then returned. Following that, functions like list() and set can receive the value returned by the map() function ().
def addition(n):
return n + n
numbers = (10, 20, 30,40)
result = map(addition, numbers)
print("Using map() function\n")
print(list(result))
Output:
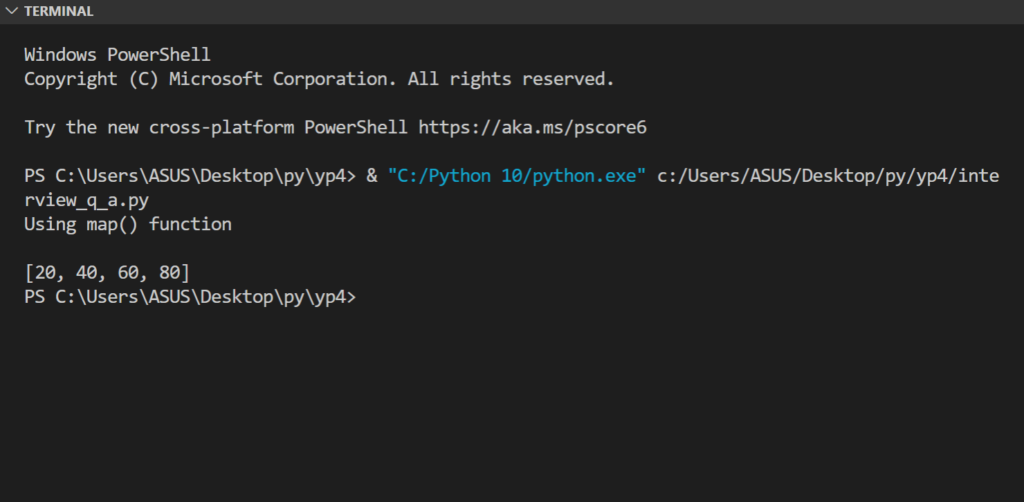
3. State an efficient way to read a CSV file in just two lines?
from numpy import genfromtxt
csv_data = genfromtxt('sample_file.csv', delimiter=',')
Answer: genfromtext() provides functionality to load the data from a text file. Here inside this function we also passed a delimiter that will separate the values with a comma (,)
4. What is the output of the below-given code?
def extendList(val, list=[]):
list.append(val)
return list
list1 = extendList(10)
list2 = extendList("CopyAssignment",[])
list3 = extendList('a')
print("Output:\n")
print(list1,list2,list3)
Expected Output: ([10],[‘CopyAssignment’],[‘a’])
Original Output:

Explanation: This is due to the fact that each time we use the function, the list argument is not initialized to its default value ([]). The function generates a new list once it has been defined. The list is then used each time we use it again without a list argument. This is due to the fact that when we declare the function rather than when we call it, it calculates the expressions in the default arguments.
5. Implement a palindrome program?
I must say, this is one of the favorite questions of all interviewers when it comes to questions on python. Python interviewers usually ask this question to test the candidate’s logic.
def isPalindrome(s):
return s == s[::-1]
s = "rotator"
ans = isPalindrome(s)
if ans:
print("Yes 'rotator' is palindrome")
else:
print("No 'rotator' is not palindrome")
Output:

Answer: Here we simply defined a function that reverses the entered string using the string slicing method and then this reversed string is compared with the original string. If they both match then the string is known as palindrome and a message is printed.
6. What do you mean by deep and shallow copy?
We have found that this question is also frequently asked in python coding questions.
Answer:
Deep copy: The values that have previously been copied are kept in deep copies. The reference pointers are not copied during the deep copy. It creates an object reference and stores the new object that is pointed to by another object. Any other copies that use the object won’t be impacted by the modifications made to the original copy. Deep copy slows down program execution since it makes specific copies of each object that is called.
Shallow copy: When a new instance type is formed, a shallow copy is employed, keeping the copied values in the new instance. Similar to how it copies values, a shallow copy is also used to copy reference points. The modifications made to any member of the class will also affect the original copy of it because these references point to the original objects. Shallow copy depends on the amount of data being used and speeds up program performance.
7. What will be the output of the below-given code?
list = [ [ ] ] * 6
print(list)
list[0].append(99)
print(list)
list[1].append(98)
print(list)
list.append(97)
print(list)
Output:

Explanation:
The purpose of the first line of output, list = [[]] * 6, is clear and obvious: it prints a list of six lists. The first line’s output will appear as follows: [[], [], [], [], [], []].
Here, it’s crucial to keep in mind that list = [[]] * 6 constructs a list with six references to the same list rather than six different lists. Now, let’s examine further code lines.
list[0] append(99) – append(99) appends 99 to the list’s beginning. The outcome is [[99], [99], [99], [99], [99], [99], [99]] since all six lists are related to the same list.
list[1].append(98) The function append(98) adds the value 98 to the second list. But because each of the six lists is related to same list, the output is [[99, 98], [99, 98], [99, 98], [99, 98], [99, 98], [99, 98]]
Line must have duped tricked you as well because list.append(97) adds a brand-new member to the “outer” list. The outcome will be as follows: [[99, 98], [99, 98], [99, 98], [99, 98], [99, 98], [99, 98], 97].
8. Explain Multithreading in Python?
Prepare this question seriously as part of your python programming interview questions because this is an important computer science topic and is usually asked in all programming interviews.
Answer: Although Python provides a multi-threading module, it’s typically not a good idea to utilize it if you want to multi-thread to speed up your code.
Known as the Global Interpreter Lock in Python (GIL). The GIL guarantees that only one of your “threads” can operate concurrently. A thread obtains the GIL, does some work, and then finally transfers the GIL to the following thread. Because of how quickly this occurs, your threads may appear to be running simultaneously to the human eye, but in reality, they are simply sharing the same CPU core. This excessive GIL passing slows down execution. This indicates that utilizing the threading package will help your code run quicker.
9. Write a function that is capable of taking a variable number of arguments?
def var_arguments(*var):
for i in var:
print(i)
var_arguments(100)
var_arguments(200,300,400)
Explanation: Here we used the concept of *args that helps us to accept the variable number of arguments for the function.
10. What is PYTHONPATH?
This is a very simple question in python viva questions but is asked in many python interviews.
Answer: The PYTHONPATH variable instructs the interpreter where to find the imported module files in a program. Therefore, it must also contain the directories holding the Python source code and the Python source library. Although PYTHONPATH can be explicitly specified, the Python installation often does.
Advanced Level Python Interview Questions
This advanced set of python coding questions and answers includes an advanced concept of Python. These questions on python include concepts like NumPY, Regular Expression, OOPS interview questions and various knows libraries, etc.
1. How will you send an email using Python?
import smtplib
s = smtplib.SMTP('smtp.gmail.com', 587)
# TLS for network security
s.starttls()
# User email Authentication
s.login("sender_email_id", "sender_email_id_password")
# Message to be sent
message = "Message_sender_need_to_send"
# Sending the mail
s.sendmail("sender_email_id", "receiver@email_id", message)
Explanation:
Line 1 and 2: Importing smtplib and calling SMTP
Line 3: For network security we used TLS
Line 4: Using login() we authenticate the sender’s mail id.
Line 5: The message variable holds the message
Line 6: sendmail() is used to actually send the email.
2. Suppose you have a numpy array of 3 columns. You have to replace the second column with the below-given column. How will you do?
New column:
[ 10
10
10 ]
import numpy as np
inputArray = np.array([[3,5,6],[7,12,2],[4,8,5]])
new_col = np.array([[10,10,10]])
arr = np.delete(inputArray , 1, axis = 1)
arr = np.insert(arr , 1, new_col, axis = 1)
print (arr)
Output:

Explanation:
Line 1: Importing numpy as np
Line 2: Creating an array.
Line 3: new_col holds the new column that is to be added.
Line 4: delete() take 3 parameters. 1st is the array from which we want to delete. 2nd is the integer that indicates the index of the sub-array that is to be deleted and 3rd one is the axis along which the sub-array is to be deleted.
Line 5: insert() also takes 3 parameters and here the 2nd parameter is the new values that are to be added.
3. Write a program to count the number of every element in the file. (Do not use the conventional method)? OR Using collection and print library count number of every element in the file.
We suggest you to prepare for this(collections) topic, this library is very useful and sometimes solve big problems in python coding logic so, you must prepare this for python coding questions and answers.
import collections
import pprint
with open("main.txt", 'r') as data:
count_data = collections.Counter(data.read().upper())
count_value = pprint.pformat(count_data)
print(count_value)
Output:

Explanation:
Line 1: and 2: Basic library import
Line 3: Using with block to open the file as data
Line 4: counter() is used to keep the count of the elements in the form of a dictionary. Elements as keys and the count as value.
Line 5: Returns the formatted representation of the data.
4. A program to print the content of the file in reversed order?
filename=input("Enter file name: ")
for line in reversed(list(open(filename))):
print(line.rstrip())
Output:

5. Is all memory de-allocated when a user exits Python?
Answer: In this case, no. When Python is closed, some modules having circular references to other objects or to objects referred to from global namespaces are not always released. Additionally, the memory that the C library has reserved cannot be released.
6. Find a word that rhymes with “cake” from the given string?
import re
rhyme=re.search('.ake','I would make a cake, but I hate to bake')
rhyme.group()
print(rhyme)
Explanation: Imported regular expression library. Then we used a search() which stops as soon as it finds the first match and here the word “make” rhymes with cake.
7. How will you create an empty class in Python?
This is simple and easy question to answer but is good to prepare for your python interview programs.
class EmptyClassDemo:
pass
obj=EmptyClassDemo()
obj.name="CopyAssignment"
print("Name created= ",obj.name)
Explanation: A class that is empty has no defined members. By employing the pass keyword, it is made (the pass command does nothing in python). Outside of the class, we can produce objects for this class.
Reference Links
Endnote
To sum up, we hope this set of Python Interview Questions and Answers will help you ace the Python interview. These questions on python are specially curated by the copyassignment team and we tried including all the most frequently asked python developer interview questions along with their detailed answer explanation. We wish you all the best in your interview.
Thank you for visiting our website

Also Read:
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Music Recommendation System in Machine Learning
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- 100+ Java Projects for Beginners 2023
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Courier Tracking System in HTML CSS and JS