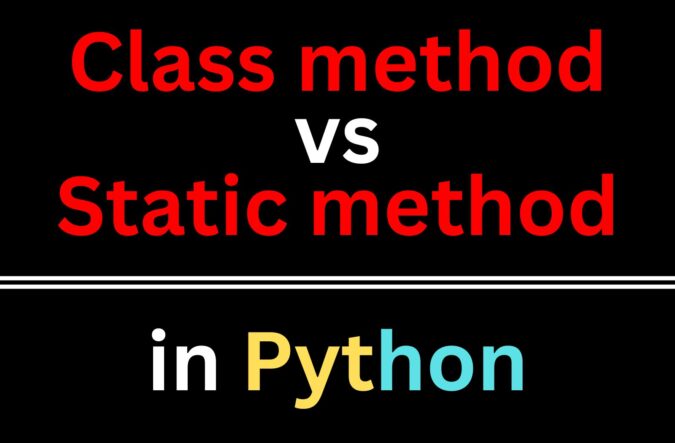
We all know that Python is the most famous language in today’s computer industry. In such a scenario knowing its core concepts gets important. Today, we will discuss the Class method vs Static method in Python.
Particularly for students coming from a Java background, where there are no class methods, this issue of class methods and static methods often arises while studying Python. These may be defined in Python using the corresponding decorators. So now let us take a look at them along with examples.
3 Types of Methods in Python
1. Instance method
Let us understand each method using an example
class MyClass: def instanceMethod(self): return 'instance method called', self
Explanation:
The very first method in MyClass is the instance method, which is a typical instance method. That is the straightforward, hassle-free approach you will most frequently adopt. As you can see, the method only accepts the self parameter, which when called, links to a MyClass object (but of course, instance methods can accept more than just one parameter).
Instance methods have unrestricted access to properties and other methods on the same object through the self-argument. They have a great deal of power to change the status of an item as a result of this. Instance methods have access to the class itself through the self.__class__ property in addition to being able to change object state. As a result, instance methods can alter class state as well.
You can read more about the instance method here as we will be discussing Class method vs Static method.
2. @classmethod
Syntax
class myClass: @classmethod def myfunc (cls, arg1, arg2, ...): # other code
Explanation:
When a class method is invoked, the cls argument, which points to the class rather than the object instance, is sent. ally identify a class, leaving just the function that has to be converted to be supplied as an argument. @classmethod Python has a built-in function called a decorator. It may be used with any class method. By employing this technique, we may also alter the value of variables.
myfunc: The function that has to be turned into a class method is defined by myfunc.
returns: For a function, @classmethod returns a class method. The object instance state cannot be changed by the class method since it only has access to the cls parameter. The class state, which affects all instances of the class, can still be changed by class methods. Therefore, a class method will automatic
Example:
class Company: no_of_leaves = 8 def __init__(self, fname, salary, position): self.name = fname self.salary = salary self.role = position def showDetails (self) : return f"The Name is (self.name). Salary is (self.salary) and role is (self.role)" @classmethod def change_leaves (cls, newleaves) : cls.no_of_leaves = newleaves dhvanil = Company ("Dhvanil", 28500, "Teacher") rohan = Company ("Rohan", 49590, "student") rohan.change_leaves (34) print(rohan.no_of_leaves)
In the above example, we used the class method to change the value of the no_of_leaves. Initially, we assigned the 8 to no_of_leaves, and then in the class method, we defined a function that changes the leaves and updated that variable to 34.
3. @staticmethod
As we are familiar with class methods, understanding a static method is extremely simple. Additionally, because it may be used without an object, it is simpler to implement than a class method. However, we may also use a class or any instance to access it.
Syntax:
class MyClass: @staticmethod def myfunc():
Explanation:
Python’s static methods and class methods are quite similar, however, a static method is connected to a class rather than the objects for that class. The @staticmethod decorator, a built-in decorator, is used to declare a static method. Additionally, using decorators does not need to import any modules. When a class has a static method, we allow only class objects or codes inside the class to access it.
Example:
class Company: no_of_leaves = 8 def __init__(self, aname, asalary, arole): self.name = aname self.salary = asalary self.role = arole def showDetails(self): return f"The Name is {self.name}. Salary is {self.salary} and role is {self.role}" @classmethod def change_leaves(cls, newleaves): cls.no_of_leaves = newleaves @classmethod def from_dash(cls, string): return cls(*string.split("-")) @staticmethod def printgood(string): print("This is good " + string) dhvanil = Company("Dhvanil", 255, "Instructor") rohan = Company("Rohan", 455, "Student") vatsal = Company.from_dash("vatsal-480-Student") Company.printgood("Dhvanil")
Static methods don’t need to know anything about the class in which they are included. Only the instances of the class may access them since they are only produced in the class. For straightforward functionality that is frequently unrelated to the class, we can utilize a static method. For instance, if we want to add two integers together but only want the class or its instances to be able to utilize it, we will build a static function. Since it simply needs the numbers to add, it won’t need any class-related data, but it will still serve its purpose because it functions as a private method that only members of the class may access.
A class method may accomplish the majority of the static methods’ features. However, we prefer the static technique since it might help our program run quicker and more efficiently because we don’t need to supply self as a parameter.
Difference between Class method and Static method in Python
Class method | Static method |
This uses the @classmethod decorator. | Here, the @staticmethod decorator is employed. |
The initial argument to the class method is cls (class). | There are no special parameters required by the static technique. |
The class is passed as a parameter to the class method in order to determine the status of that class. | The status of the class is unknown to static methods. By using a few arguments, these techniques are utilized to carry out several useful operations. |
The class state is accessible and modifiable by class methods | The class state cannot be accessed or changed by static methods. |
Summary
We hope that this article will help you in understanding the concepts of the Class method vs Static method and the difference between Class method and Static method in Python. We made use of examples in order to make this concept easier for you. Thank you for visiting our website.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter