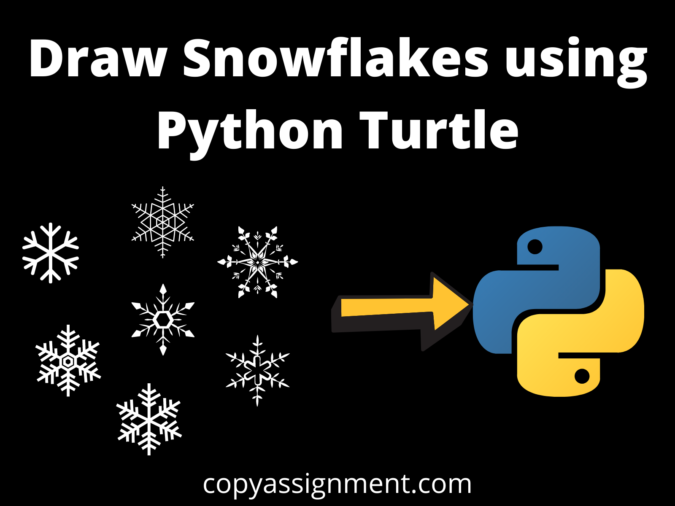
Introduction
Hello everyone, welcome to violet-cat-415996.hostingersite.com. In this tutorial, we are going to learn how to draw snowflakes using python turtle module. This article is super interesting and easy to understand. In this snowflakes article, we have drawn various small and large size snowflakes. For that, we have used the random module of Python turtle. We can create branches of various sizes at various random locations on the screen.
For the complete code, you can go to the bottom of the page you will get the entire code with output.
So let’s start
Import Turtle
import turtle
from random import randint
We have imported the turtle module so that we can access its inbuilt methods and functions. We used the random module an in-built module of Python to generate random numbers. we have used the randint() function, to print random values for a list or string.
Set the turtle Object and the Screen of Snowflakes using Python Turtle
p=turtle.Turtle()
p.shape("turtle")
#speed of turtle
p.speed(1500)
p.pensize(4)
scr=turtle.Screen()
#setting the background color & title
scr.bgcolor("SkyBlue1")
scr.title("Merry Christmas")
In this piece of code, we set the turtle object as p. Turtle shape is of “turtle”.The speed of the turtle is 1500. The screen background color is SkyBlue. Set the title of the screen.
Create the Snowflake function by setting the coordinates, color, and size of snowflakes
#function for setting the colour x and y coordinates and size of snowflake
def snowflake(color,x,y,size):
p.penup()
p.goto(x,y)
p.pendown()
p.color(color)
p.left(90)
#Set the for loop for create the snowflake branches
for i in range(0,branches):
p.forward(100*size/100)
p.backward(40*size/100)
p.left(40)
p.forward(30*size/100)
p.backward(30*size/100)
p.right(80)
p.forward(30*size/100)
p.backward(30*size/100)
p.left(40)
p.backward(40*size/100)
p.left(40)
p.forward(30*size/100)
p.backward(30*size/100)
p.right(80)
p.forward(30 * size / 100)
p.backward(30 * size / 100)
p.left(40)
p.backward(20*size/100)
p.right(360/branches)
In this block of code, we have created a function for snowflakes where we have set the parameters as x and y coordinates, color, and size of the snowflake. So here we have not individually defined the x and y coordinates the size and the color. The actual parameters are responsible for the desired output.
In the for loop block, we create the branches for our snowflakes randomly. It is done by calling the randint(5,10) function. Any number between 5 to 10 is passed randomly to the snowflake(). According to that our snowflake branches are created. The size variable in this block allows for an increase or decrease in the snowflake size. The last function i.e right(360/branches) takes care of the actual number of branches on every iteration.
Setting text color, style, and size in the snowflake python turtle
#writing the text and providing the color
p.color("green")
style=('Courier',20,'italic')
p.write('Beautiful Snow', font=style,align='center')
In this piece of code, we have set the color of the text to green,font=courier,size=20, and italic. p.write function to write the text on the graphics screen.
Passing the actual parameters to the snowflake function
#for loop for to develop 20 snowflakes
for i in range(0,20):
randomX = randint(-200,200)
randomY = randint(-200,200)
#size will be passed randomly between 5 and 40 by using randint
randomSize = randint(5,40)
branches=randint(5,10)
snowflake("white",randomX,randomY,randomSize)
turtle.done()
In this block of code, we have passed actual parameters for snowflakes which are the color,x,y, and size to the snowflake function. The snowflakes are displayed anywhere between -200 and 200 as a result of the randint(). Sizes are any number between 5 and 40. The Colour we have passed for snowflakes is white.
Complete Code Draw Snowflakes using Python Turtle
#import Turtle import turtle from random import randint p=turtle.Turtle() p.shape("turtle") #speed of turtle p.speed(1500) p.pensize(4) scr=turtle.Screen() #setting the background color & title scr.bgcolor("SkyBlue1") scr.title("Merry Christmas") #function for setting the color a and y coordinates and size of snowflake def snowflake(color,x,y,size): p.penup() p.goto(x,y) p.pendown() p.color(color) p.left(90) #Set the for loop for create the snowflake branches for i in range(0,branches): #creates the shape of the snowflake p.forward(100*size/100) p.backward(40*size/100) p.left(40) p.forward(30*size/100) p.backward(30*size/100) p.right(80) p.forward(30*size/100) p.backward(30*size/100) p.left(40) p.backward(40*size/100) p.left(40) p.forward(30*size/100) p.backward(30*size/100) p.right(80) p.forward(30 * size / 100) p.backward(30 * size / 100) p.left(40) p.backward(20*size/100) p.right(360/branches) #writing the text and providing the color p.color("green") style=('Courier',20,'italic') p.write('Beautiful Snow', font=style,align='center') #for loop for to develop 20 snowflakes for i in range(0,20): randomX = randint(-200,200) randomY = randint(-200,200) randomSize = randint(5,40) branches=randint(5,10) snowflake("white",randomX,randomY,randomSize) turtle.done()
Output
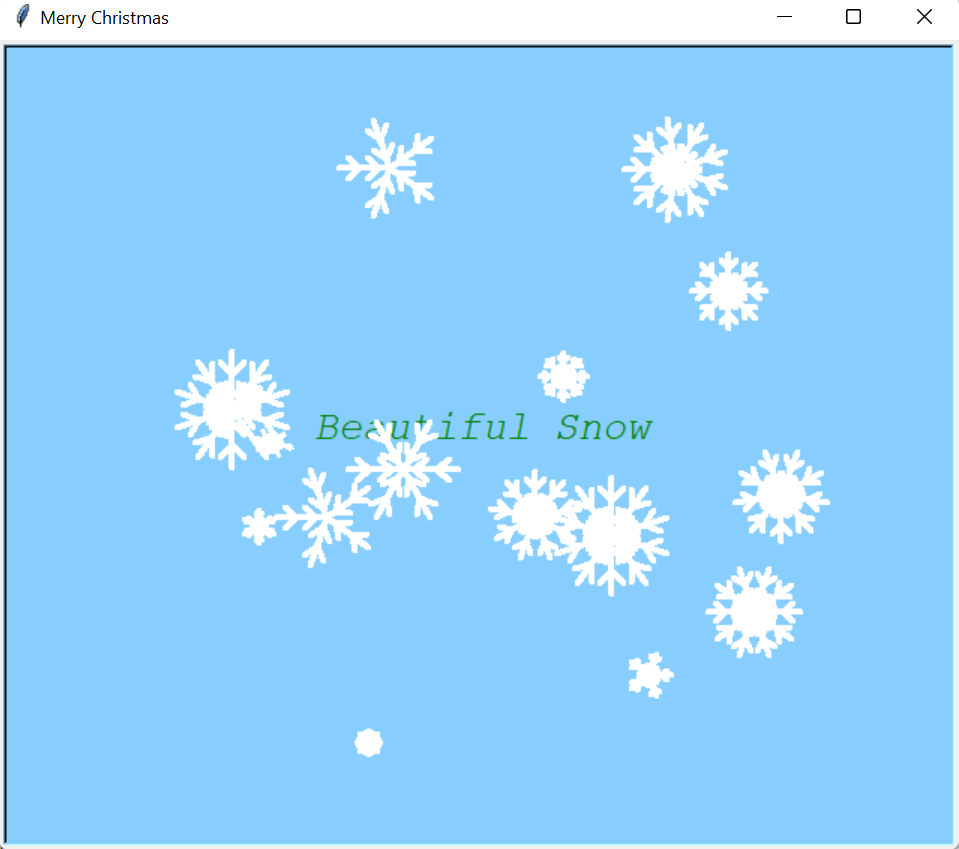
So here you can see we have created beautiful snowflakes. Like this shape of snowflakes, you can create various different shapes of snowflakes you like.
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector