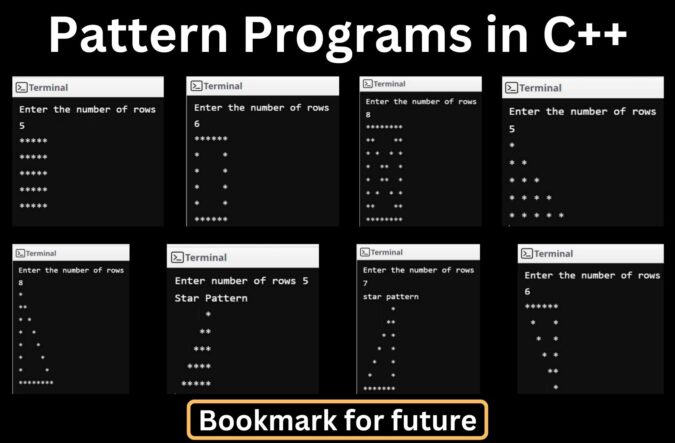
In this article, I will show you the Top 25 Pattern Programs in C++ that you must learn which will help you to understand the usage of nested loops. We will learn to create different geometrical shapes like Squares, triangles, Rhombus, etc. We have provided the code as well as output for all the patterns below.
1. Square Pattern Program in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows " << endl; cin >> n; for(int i=0;i<n;i++) { for(int j=0;j<n;j++) { cout << "*"; } cout << endl; } return 0; }
Output:

2. Hollow Square Pattern Programs in C++
int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; for(int i=1;i<=n;i++) { for(int j=1;j<=n;j++) { if(i==1 ||i==n||j==1||j==n) { cout << "*"; } else cout << " "; } cout << endl; } return 0; }
Output:
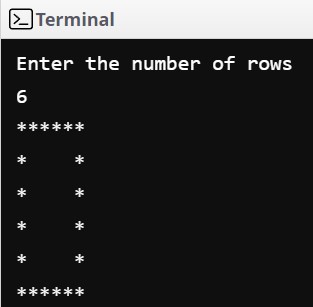
3. Hollow Square Pattern with Diagonal in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; for(int i=1; i<=n; i++) { for(int j=1; j<=n; j++) { if(i==1 ||i==n||j==1||j==n-i+1||i==j||j==n) { cout << "*"; } else { cout << " "; } } cout << endl; } return 0; }
Output:

4. Right Angled Triangle pattern
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; for(int i=1; i<=n; i++) { for(int j=1; j<=i; j++) { cout << "* "; } cout << endl; } return 0; }
Output:

5. Hollow Right Angle Triangle pattern
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; for(int i=1; i<=n; i++) { for(int j=1; j<=i; j++) { if(j==1|| i==j || i==n ) { cout<< "*"; } else cout<< " "; } cout << endl; } return 0; }
Output:
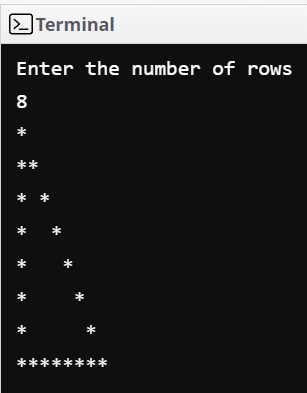
6. Mirrored Right Angle Triangle pattern
#include<iostream> using namespace std; int main() { int i, j, k, rows; cout << "Enter number of rows "; cin >> rows; cout << "Star Pattern\n"; for(i = 1; i <= rows; i++) { for(j = 0; j <= rows - i; j++) { cout << " "; } for(k = 0; k < i; k++) { cout << "*"; } cout << "\n"; } return 0; }
Output:

7. Mirrored Hollow Right Angle Triangle pattern
#include <iostream> using namespace std; int main() { int n; int m = 1; cout << "Enter the number of rows" << endl; cin >> n; cout << "star pattern \n"; for(int i=n; i>=1; i--) { for(int j=1; j<=i-1; j++) { cout << " "; } for(int k=1; k<=m; k++) { if(k==1 || k==m || m==n) cout << "*"; else cout << " "; } cout << endl; m++; } return 0; }
Output:

8. Hollow Inverted Right Angle Triangle
#include <iostream> using namespace std; int main() { int n; int m; cout << "Enter the number of rows" << endl; cin >> n; m=n; for(int i=1; i<=n; i++) { for(int j=1; j<i; j++) { cout << " "; } for(int k=1; k<=m; k++) { if(i==1 || k==1 || k==m) cout << "*"; else cout << " "; } m--; cout << endl; } return 0; }
Output:

9. Hollow Inverted Mirrored Right Angle Triangle
#include <iostream> using namespace std; int main() { int n; int m = 1; cout << "Enter the number of rows" << endl; cin >> n; for(int i=n; i>=1; i--) { for(int j=1; j<=i; j++) { if(j==1 || j==i || i==n) cout << "*"; else cout << " "; } cout << endl; } return 0; }
Output:

10. Inverted Mirrored Right Angle Triangle
#include <iostream> using namespace std; int main() { int n; int m; cout << "Enter the number of rows" << endl; cin >> n; m=n; for(int i=1; i<=n; i++) { for(int j=1; j<i; j++) { cout << " "; } for(int k=1; k<=m; k++) { cout << "*"; } m--; cout << endl; } return 0; }
Output:

11. Inverted Right Angle Triangle pattern
#include <iostream> using namespace std; int main() { int n; int m = 1; cout << "Enter the number of rows" << endl; cin >> n; for(int i=n; i>=1; i--) { for(int j=1; j<=i; j++) { cout << "*"; } cout << endl; } return 0; }
Output:

12. Pyramid Pattern Program in C++
#include <iostream> using namespace std; int main() { int n; int m; cout << "Enter the number of rows" << endl; cin >> n; cout << "Pyramid pattern is\n"; m = n; for(int i=1; i<=n; i++) { for(int j=1; j<=m-1; j++) { cout<< " "; } for(int k=1; k<=2*i-1; k++) { cout << "*"; } m--; cout << endl; } return 0; }
Output:
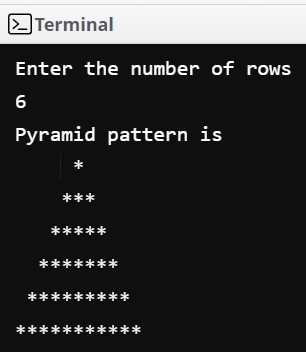
13. Hollow Pyramid Pattern
#include <iostream> using namespace std; int main() { int n; int m; cout << "Enter the number of rows" << endl; cin >> n; cout << "Hollow Pyramid pattern is\n"; m = n; for(int i=1; i<=n; i++) { for(int j=1; j<=m-1; j++) { cout << " "; } for(int k=1; k<=2*i-1; k++) { if(k==1 || k==2*i-1 || i==n) cout << "*"; else cout << " "; } m--; cout << endl; } return 0; }
Output:
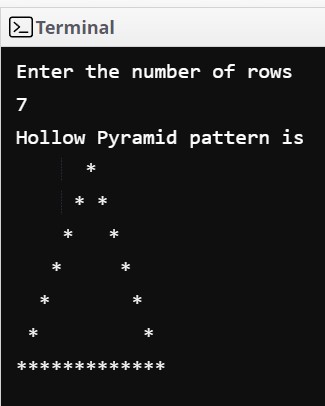
14. Inverted Pyramid Star Pattern
#include <iostream> using namespace std; int main() { int n; int m; cout << "Enter the number of rows" << endl; cin >> n; cout << "Inverted Pyramid pattern is\n"; m = 1; for(int i=n; i>=1; i--) { for(int j=1; j<m; j++) { cout << " "; } for(int k=1; k<=2*i-1; k++) { cout << "*"; } m++; cout << endl; } return 0; }
Output:
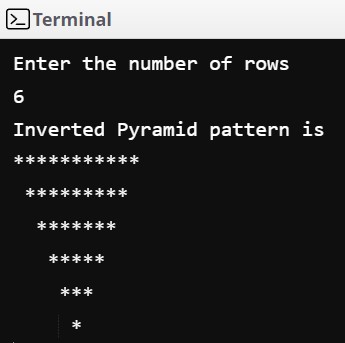
15. Hollow Inverted Pyramid Pattern
#include <iostream> using namespace std; int main() { int n; int m; cout << "Enter the number of rows" << endl; cin >> n; cout << "Hollow Inverted Pyramid pattern is\n"; m = 1; for(int i=n; i>=1; i--) { for(int j=1; j<m; j++) { cout << " "; } for(int k=1; k<=2*i-1; k++) { if(k==1 || k==2*i-1 || i==n) cout << "*"; else cout << " "; } m++; cout << endl; } return 0; }
Output:
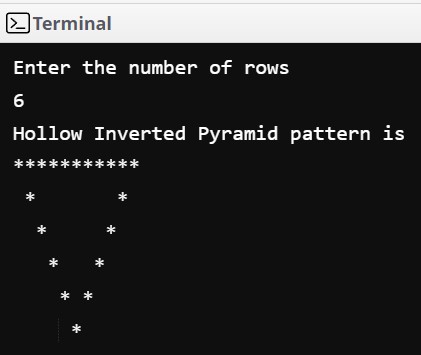
16. Rhombus Pattern in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; cout<< "Rhombus Star Pattern is \n"; for(int i=n; i>=1; i--) { for(int j=1; j<=i-1; j++) { cout << " "; } for(int k=1; k<=n; k++) { cout << "*"; } cout << endl; } return 0; }
Output:

17. Hollow Rhombus Pattern Program in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; cout<< "Hollow Rhombus Star Pattern is \n"; for(int i=n; i>=1; i--) { for(int j=1; j<=i-1; j++) { cout << " "; } for(int k=1; k<=n; k++) { if(i==1 || i==n || k==1 || k==n) cout << "*"; else cout << " "; } cout << endl; } return 0; }
Output:
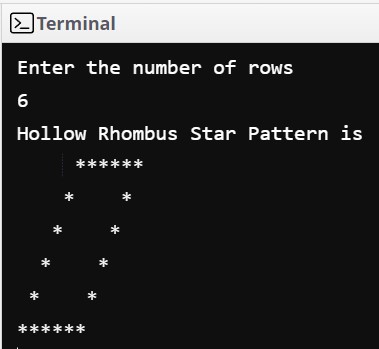
18. Mirrored Rhombus Pattern in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; cout<< "Mirrored Rhombus Star Pattern \n"; for(int i=1; i<=n; i++) { for(int j=1; j<i; j++) { cout << " "; } for(int k=1; k<=n; k++) { cout << "*"; } cout << endl; } return 0; }
Output:

19. Hollow Mirrored Rhombus Pattern
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; cout<< "Hollow Mirrored Rhombus Star Pattern \n"; for(int i=1; i<=n; i++) { for(int j=1; j<i; j++) { cout << " "; } for(int k=1; k<=n; k++) { if(i==1 || i==n || k==1 || k==n) cout << "*"; else cout << " "; } cout << endl; } return 0; }
Output:

20. Diamond Pattern Program in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows" << endl; cin >> n; cout << "Diamond pattern \n"; int spaces=n-1; int stars=1; for(int i=1; i<=n; i++) { for(int j=1; j<=spaces; j++) { cout << " "; } for(int k=1; k<=stars; k++) { cout << "*"; } if(spaces>i) { spaces=spaces-1; stars=stars+2; } if(spaces<i) { spaces=spaces+1; stars=stars-2; } cout << endl; } return 0; }
Output:
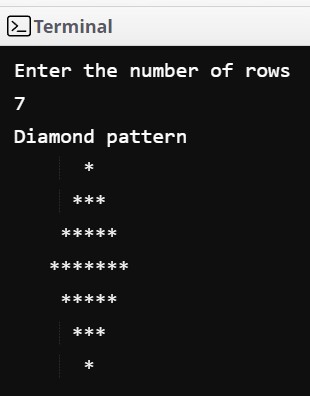
21. Half Diamond Pattern in C++
#include <iostream> using namespace std; int main() { int n, m; cout << "Enter the number of rows" << endl; cin >> n; cout << "Half Diamond pattern \n"; m=1; for(int i=1; i<=n; i++) { for(int j=1; j<=i; j++) { cout << "*"; } cout << endl; } for(int i=n-1; i>=1; i--) { for(int j=1; j<=i; j++) { cout << "*"; } cout << endl; } return 0; }
Output:

22. Plus Pattern in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter the number of rows(only odd)" << endl; cin >> n; cout << "Plus Pattern \n"; for(int i=1; i<=n; i++) { if(i==((n/2)+1)) { for(int j=1; j<=n; j++) { cout << "+"; } } else { for(int j=1; j<=n/2; j++) { cout << " "; } cout << "+"; } cout << endl; } return 0; }
Output:

23. Cross Pattern in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter number of rows"<< endl; cin >> n; int m = 2*n - 1; for ( int i = 1; i <= m; i++ ) { for ( int j = 1; j <= m; j++ ) { if (j == i || j == (m + 1 - i)) cout << "* "; else cout << " "; } cout << endl; } }
Output:

24. Right Arrow Pattern Program in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter number of rows(odd only)"<< endl; cin >> n; for(int i=0; i<n; i++) { for(int j=0; j<i; j++) { cout << " "; } for(int k=1; k<=n-i; k++) { cout << "*"; } cout << endl; } for(int i=1; i<n; i++) { for(int j=1; j<n-i; j++) { cout << " "; } for(int k=1; k<=i+1; k++) { cout << "*"; } cout << endl; } return 0; }
Output:
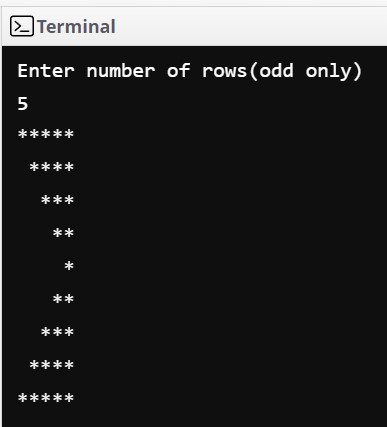
25. Left Arrow Pattern in C++
#include <iostream> using namespace std; int main() { int n; cout << "Enter number of rows(odd only)"<< endl; cin >> n; cout << "Left Arrow Star Pattern \n"; for(int i=1; i<=n; i++) { for(int j=1; j<=n-i; j++) { cout << " "; } for(int k=0; k<=n-i; k++) { cout << "*"; } cout << endl; } for(int i=1; i<n; i++) { for(int j=1; j<i+1; j++) { cout << " "; } for(int k=1; k<=i+1; k++) { cout << "*"; } cout << endl; } return 0; }
Output:
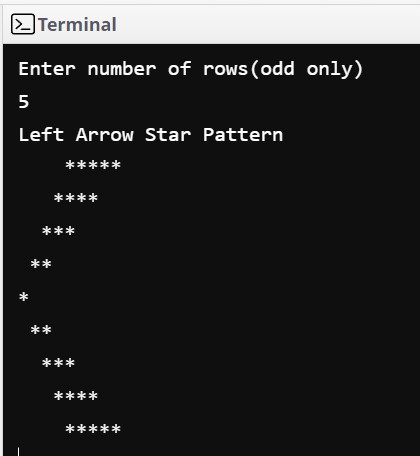
Conclusion
This brings us to the end of the Top 25 Pattern Programs in C++. I hope this article helped you to get more clarity on the fundamentals of C++. Once you are comfortable with the logic of these patterns, you will be able to write most of the pattern printing programs in C++ on your own.
Thank you for visiting our website.
Also Read:
- Falling Stars Animation on Python.Hub October 2024
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers