
Before Attendance Management System Project in Python with SQLite3 database, we have learned a lot about Python and its libraries by developing various utility projects, games etc. We have developed multiple games using turtle library etc but this time it’s going to be different. Today, in this article we are not going to develop a game but a perfect project that you can put up on your resume.
We are going to develop a full-fledged Attendance Management System in Python. This time we are going to use some new libraries of Python in order to create this project. As our project involves data and so we will use a new library SQLite3 in order to handle data. This project will ship with a lot of utility and a lot of learnings. Before starting with the actual coding, we will see what we actually need to build and what features it should have. So let’s get to the point and start learning & building.
Attendance Management System Project in Python Features:
- There should be a feature to add new subjects
- An option to manage attendance that shows today’s attendance data, add attendance for each subject, etc
- Update any subject attendance
- Delete record
Importance of Attendance management system project in python with source code
- Python attendance management system is a good project for final year students
- This is good if you have to practice medium size projects having GUI in Python
- With SQLite database features, you can learn database in Python
- This is a GUI based project which uses tkinter module in Python for GUI purposes
- There are many good features in attendance management system using python with source code
Libraries used:
For the development of this project, we are going to use 2 libraries:
- Tkinter
- SQLite3
Tkinter is a GUI toolkit library that helps us to create rich interfaces.
SQLite3 is a lightweight database library that helps us to deal with data and in this project obviously, we are going to accept the data from users and thus we will use this library.
Code Flow:
Importing required libraries:
import tkinter as tk
from tkinter import messagebox
import sqlite3 as sql
Explanation:
Here we have imported Tkinter and will use it as tk. We have imported messagebox from Tkinter and we imported SQLite3 and will use it as sql.
Class: AttendanceManager
class AttendanceManager(tk.Tk):
def __init__(self,*args,**kwargs):
tk.Tk. __init__(self,*args,**kwargs)
container=tk.Frame(self)
container.pack(side="top",fill="both",expand=True)
container.grid_rowconfigure(0,weight=1)
container.grid_columnconfigure(0,weight=1)
self.frames=dict()
for F in (StartPage,NewRecord,ManageAttendance,DeleteRecord,EditRecord,AddSubjects,TodayData):
frame=F(container,self)
self.frames[F]=frame
frame.grid(row=0,column=0,sticky="nsew")
self.show_frame(StartPage)
def show_frame(self,cont):
frame=self.frames[cont]
frame.tkraise()
Explanation for above part of student attendance management system project in python:
Line 1: Class declaration with the name AttendanceManager
In Line 2: It allows the class to initialize class objects.
Line 3: We used *args and **kwargs to pass a variable number of arguments.
In Line 4: A variable container holds the frame that is used to handle some other widgets in a group.
Line 5 to 7: grid_rowconfigure and grid_columnconfigure used to configure the rows and columns. The weight parameter decides how wide the column and row will be.
Line 8: Declared a dictionary.
In Line 9 to 12: for loop sets the frames in a grid form that are in the dictionary.
Line 13: called the show_frame
Line14: Here we defined a function named self_show and we used frame.tkraise() to raise the frame to the top among multiple frames.
Class: StartPage
This class is responsible for displaying the text and the button for different functionalities in Attendance Management System Project in Python.
class StartPage(tk.Frame):
def __init__(self,parent,controller):
tk.Frame.__init__(self,parent)
label1=tk.Label(self,text="Attendance Management System Project in Python",font=("Times",26))
bt1=tk.Button(self,text="Add new record",font=("Times",16),height=2,width=17,bg="blue" ,command=lambda:controller.show_frame(NewRecord))
bt2=tk.Button(self,text="Manage attendance",font=("Times",16),height=2,width=17,bg="yellow",command=lambda:controller.show_frame(ManageAttendance))
bt3=tk.Button(self,text="Delete record",font=("Times",16),height=2,width=17,bg="red",command=lambda:controller.show_frame(DeleteRecord))
bt4=tk.Button(self,text="Edit record",font=("Times",16),height=2,width=17,bg="orange",command=lambda:controller.show_frame(EditRecord))
label1.pack()
bt1.pack()
bt2.pack()
bt3.pack()
bt4.pack()
Output:
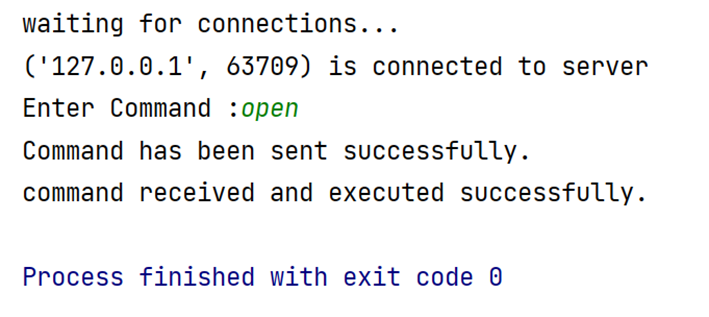
Explanation:
In Line 1: Class declaration with name StartPage.
Line 2: We passed two parameters namely parent and controller other than self.
Line 3: Here, tk.Frame.__init__(self, parent), we called this in order to bring our object to work.
On line 4: A label is a widget that we used to give a label. We can change the font by passing the font name to the font parameter.
Line 5 to 8: tk.Button() adds a button to our Attendance Management System Project in Python GUI. We have passed various parameters like text by which we have displayed our desired text on the button, the font we have used to change the font family, width, and height was passed to set the width and height of the button. In the end, we use a lambda function.
Line 9 to 13: The pack geometry manager arranges widgets in relation to the previous one. Tkinter stacks all of the widgets in a window one after the other. pack() can perform the following tasks:
- Put a widget within a frame (or any other container widget) and have it fill the frame completely.
- Stack a few widgets on top of one another.
- Place a number of widgets next to each other.
Class: NewRecord
This class is responsible for adding new records when the user clicks “Add new record” this class will come into action.
class NewRecord(tk.Frame):
def __init__(self,parent,controller):
tk.Frame.__init__(self,parent)
label1=tk.Label(self,text="New Record",font=("Times",24))
label2=tk.Label(self,text="NOTE: If you want a new record, previous one will be deleted,continue?",font=("Times",14))
bt2=tk.Button(self,text="YES",font=("Times",16),bg="orange",height=2,width=17,command=lambda:controller.show_frame(AddSubjects))
bt3=tk.Button(self,text="NO",font=("Times",16),bg="red",height=2,width=17,command=lambda:controller.show_frame(StartPage))
label1.pack()
label2.pack()
bt2.pack()
bt3.pack()
Output:

Explanation for above part of student attendance management system project in python with source code:
On line 1: Class declaration with name NewRecord.
Line 2: We passed two parameters namely parent and controller other than self.
On line 3: tk.Frame.__init__(self, parent) we called this in order to bring our object to work.
In Line 4: We added the label “New Record” in the same way as we did in the “StartPage” class.
Line 5: We added another label to notify the user.
On lines 6 to 7: Here, we used two buttons in order to take the input in the form of “Yes” or “No” using buttons.
Line 8 to 11: And finally we used pack() to pack the labels and button (Refer to lines 9 to 13 in the “StartPage” class)
Class: ManageAttendance
Whenever the user clicks on “Manage attendance” this class will come into the picture. This class offers multiple options to users like Show status, Today’s data and a Back to the home button.
class ManageAttendance(tk.Frame):
def __init__(self,parent,controller):
tk.Frame.__init__(self,parent)
label1=tk.Label(self,text="Manage Attendance",font=("Times",24))
label1.pack()
bt2=tk.Button(self,text="Show status", bg="green",font=("Times",16),height=2,width=17,command=lambda:self.showstatus(controller))
bt3=tk.Button(self,text="Today's data", bg="orange",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(TodayData))
bt1=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage))
bt2.pack()
bt3.pack()
bt1.pack()
def showstatus(self,controller):
try:
conn=sql.connect("attend")
cur=conn.cursor()
cur.execute('SELECT * FROM attable')
text=""
for w in cur:
if w[2]==0 and w[3]==0:
per="0"
else:
per=w[2]/(w[2]+w[3])
per=per*100
per=str(int(per))
text=text+"sub id "+str(w[0])+" "+w[1]+" "+per+"%\n"
messagebox.showinfo("status", text)
except:
messagebox.showinfo("Alert!", "There is no record")
Output:

Explanation:
Line 1: Class declaration with name ManageAttendance.
On line 2: Passed two parameters namely parent and controller other than self.
Line 3: Here, tk.Frame.__init__(self, parent), we called this in order to bring our object to work.
On lines 4 to 5: We added the label “Manage Attendance” using the label widget and packed it using pack().
Line 6 to 8: We used two buttons in order to give users the option of “Show status”, “Today’s data”, and “Back to home”. Color is added to these buttons using bg=”<color_name>”
Line 9 to 11: And finally again we used pack() to pack the labels and button (Refer to explanation for “StartPage” class)
Line 12: Defined a function named showstatus that fetches data from the database and shows it on the screen. And then initiated a try block in the next line.
On line 13: sql.connect(“attend”), used to connect to database named attend.
Line 14: Here, the cursor() is the instance of a class that helps us to initiate methods like executing SQLite statements, fetching data from the database etc.
Line 15: Variable declaration named text.
On lines 16 to 17: We used a for loop to calculate the percentage of attendance in each subject.
Line 18: Now, messagebox.showinfo() is basically used to show the message that is passed as a parameter in this function in box form.
On line 19: commit() is used to save all the changes that took place because of transactions made to the database.
Line 20: close() is used to disconnect to the database. This command is very important. Whenever there is no need for a database connection then close() should be mentioned. Here’s the end of the try block.
Line 21: And finally the except block will come into the picture if there’s no record in the database and the user still requests to show status.
Class: DeleteRecord
class DeleteRecord(tk.Frame):
def __init__(self,parent,controller):
tk.Frame.__init__(self,parent)
label1=tk.Label(self,text="Delete Record",font=("Times",24))
label2=tk.Label(self,text="This action will delete the record,continue?",font=("Times",12))
bt2=tk.Button(self,text="YES",bg="green",font=("Times",16),height=2,width=17,command=lambda:self.delrecord(controller))
bt1=tk.Button(self,text="NO",bg="yellow",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage))
bt3=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage))
label1.pack()
label2.pack()
bt2.pack()
bt1.pack()
bt3.pack()
def delrecord(self,controller):
conn=sql.connect('attend')
cur=conn.cursor()
cur.execute('DROP TABLE IF EXISTS attable')
conn.commit()
conn.close()
messagebox.showinfo("Alert!", "records deleted")
controller.show_frame(StartPage)
Output:
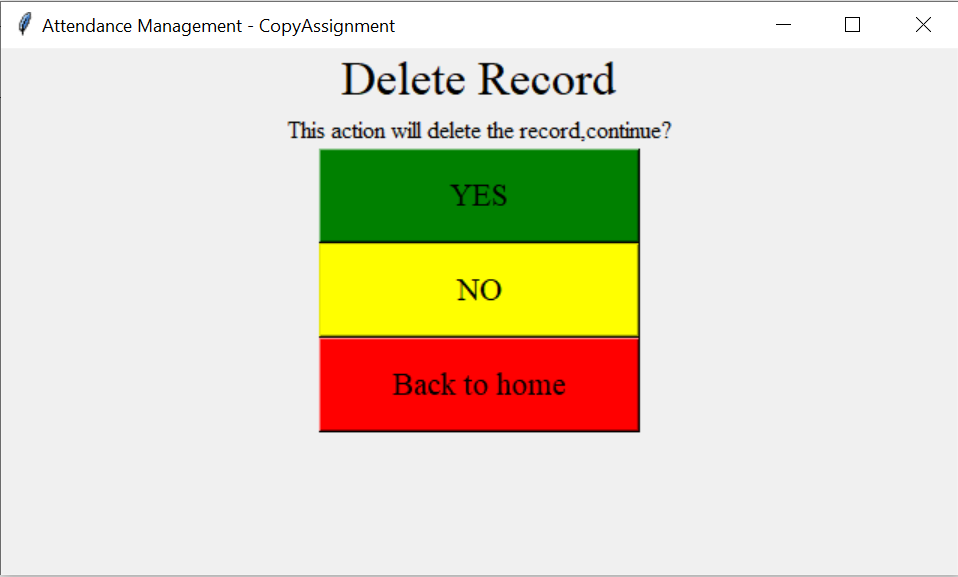
Explanation:
Line 1: Class declaration with name DeleteRecord.
In line 2: Passed two parameters namely parent and controller other than self.
Line 3: Here, tk.Frame.__init__(self, parent) we called this in order to bring our object to work.
On lines 4 to 5: We used the label widget to display the message.
Line 6 to 8: Used buttons to display options like “Yes”, “No” and “Back to home”.
On lines 9 to 13: Packed labels and buttons as usual using pack (Refer to lines 9 to 13 in the “StartPage” class)
Line 14: Declared a function named “delrecord” which is responsible for handling the deletion process.
In line 15: Again, sql.connect(“attend”), to connect to the database named attend.
Line 16: We again used cursor() and it is the instance of a class that helps us to initiate methods like executing SQLite statements, fetching data from the database etc.
Line 17: cur.execute(‘DROP TABLE IF EXISTS attable’) this line will execute a command that will drop the table named “attable”. If the table doesn’t exist then an error will be shown.
On line 18: commit() is used to save all the changes that took place because of transactions made to the database.
Line 19: close() is used to disconnect to the database.
On line 20: A message is displayed using messagebox.showinfo().
Line 21: The last line takes the user to the Start page with the help of the show_frame function.
Class: EditRecord
class EditRecord(tk.Frame):
def __init__(self,parent,controller):
tk.Frame.__init__(self,parent)
label1=tk.Label(self,text="Edit Record",font=("Times",24))
bt1=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage))
label1.pack()
lb2=tk.Label(self,text="Input Subject ID: ",font=("Times",15))
txt1=tk.Entry(self)
lb2.pack()
txt1.pack()
lb3=tk.Label(self,text="Number of times attended:",font=("Times",15))
txt2=tk.Entry(self)
lb4=tk.Label(self,text="Number of times bunked:",font=("Times",15))
txt3=tk.Entry(self)
lb3.pack()
txt2.pack()
lb4.pack()
txt3.pack()
bt3=tk.Button(self,text="Update",bg="green",font=("Times",16),height=2,width=17,command=lambda:self.update(txt1.get(),txt2.get(),txt3.get()))
bt2=tk.Button(self,text="Show subjects ID",bg="yellow",font=("Times",16),height=2,width=17,command=lambda:self.showid(controller))
bt2.pack()
bt3.pack()
bt1.pack()
def update(self,i,p,b):
i=int(i)
if p=="" or p==" " or p=="\n":
p=0
else:
p=int(p)
if b=="" or b==" " or b=="\n":
b=0
else:
b=int(b)
try:
conn=sql.connect("attend")
cur=conn.cursor()
cur.execute("SELECT * FROM attable WHERE subid=?",(i,))
kk=cur.fetchone()
np=p
nb=b
cur.execute("UPDATE attable SET attended = ? WHERE subid= ?",(np,i))
cur.execute("UPDATE attable SET bunked = ? WHERE subid= ?",(nb,i))
conn.commit()
conn.close()
messagebox.showinfo("Alert!", "Updated")
except:
messagebox.showinfo("Alert!", "There is no record")
def showid(self,controller):
try:
conn=sql.connect("attend")
cur=conn.cursor()
cur.execute('SELECT * FROM attable')
text=""
for w in cur:
text=text+"sub id "+str(w[0])+" "+w[1]+"\n"
messagebox.showinfo("Subject ID: ", text)
conn.commit()
conn.close()
except:
messagebox.showinfo("Alert!", "There is no record")
Output:
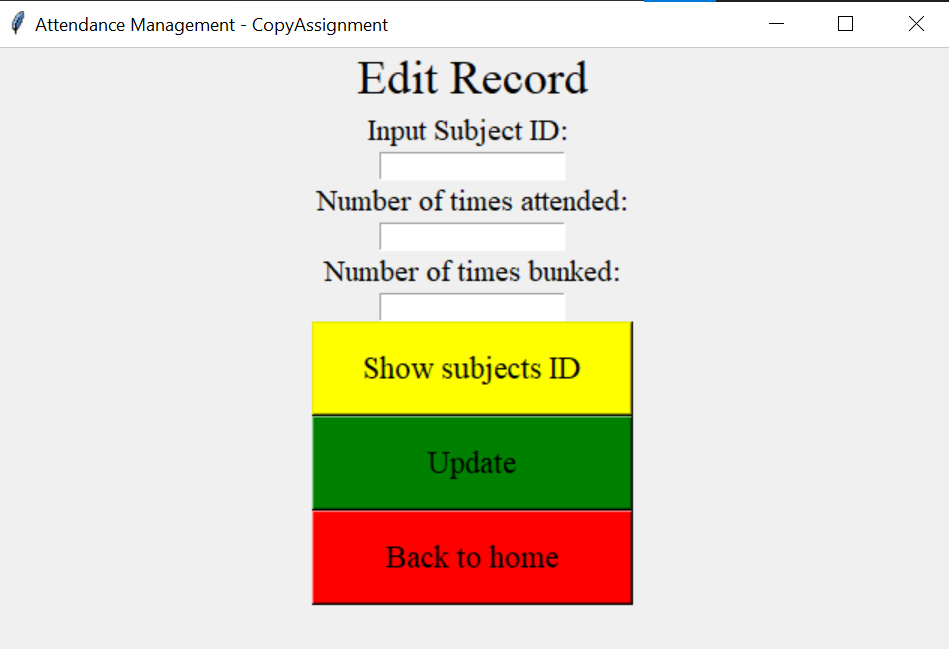
Explanation for above part of attendance management system in python with mysql database:
Line 1: Class declaration with name EditRecord.
On line 2: Passed two parameters namely parent and controller other than self.
Line 3: Here, tk.Frame.__init__(self, parent) we called this in order to bring our object to work.
On lines 4 to 6: We used the label widget, and button and packed them.
Line 7: Again a label to display “Input Subject ID: “
Line 8: In order to take a single line input from the user we used the Entry() widget. The input from the user will be stored in txt1.
Line 9 to 10: Packing of labels and buttons
On lines 11 to 14: Again two labels along with the Entry() widget.
Line 15 to 18: Packed those labels and entry fields i.e. txt2 and txt3 using packed()
On lines 19 to 20: Added buttons for “Update” and “Show subjects ID” and in lines, 21 t0 23 packings are done.
Line 24 to 45: Here comes the main logic for the update button. The update button is responsible for updating the records but how?
- First of all, we defined an update() function in which we used try and except block.
- The try block holds the logic for updating the records that are already there in the database.
- This is done using the cur.execute(“SELECT * FROM attable WHERE subid=?”,(I,)) on line 37, this helps us to select the record based on the id mentioned by the user.
- Now to update the record for that id we used
cur.execute(“UPDATE attable SET attended = ? WHERE subid= ?”,(np,I)) and
cur.execute(“UPDATE attable SET bunked = ? WHERE subid= ?”,(nb,i)) - In the above commands, we used UPDATE SQLite3 command that will SET the value as mentioned by the user on that subject id.
Line 46 to 47: And finally, the except block shows the error if there’s no record in the table and still the user wants to update the records.
Line 48 to 60: Here in between these lines we declared a function named showid that is responsible to display the IDs of the subject when the user clicks on Show subject’s ID.
Here we again made use of SELECT to select the IDs from the database and display it to the user. In the end, we used an except block that will run if the user request IDs even if there’s no record in the database.
Class: AddSubjects
class AddSubjects(tk.Frame):
def __init__(self,parent,controller):
tk.Frame.__init__(self,parent)
label1=tk.Label(self,text="Add subject's name seperated by commas(,)",font=("Times",16))
txt1=tk.Text(self,font=("Times",16),width=48,height=3)
bt2=tk.Button(self,text="Add subjects!",bg="orange",font=("Times",16),height=2,width=17,command=lambda:self.addsub(txt1.get("1.0",tk.END),controller))
bt1=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage))
label1.pack()
txt1.pack()
bt2.pack()
bt1.pack()
def addsub(self,a,controller):
conn=sql.connect('attend')
cur=conn.cursor()
cur.execute('DROP TABLE IF EXISTS attable')
a=a[0:len(a)-1]
a=a.split(",")
if len(a)==1 and a[0]=="" :
messagebox.showinfo("Alert!", "Please enter the subjects")
else:
sid=1
cur.execute('CREATE TABLE attable(subid INTEGER,subject TEXT,attended INTEGER,bunked INTEGER)')
for sub in a:
cur.execute('INSERT INTO attable (subid,subject,attended,bunked) VALUES(?,?,?,?)',(sid,sub,0,0))
sid=sid+1
conn.commit()
conn.close()
messagebox.showinfo("congratulations!", "subjects are added")
controller.show_frame(StartPage)
Output:

Explanation:
Line 1: Class declaration with name AddSubjects.
On line 2: Passed two parameters namely parent and controller other than self.
Line 3: Here, tk.Frame.__init__(self, parent) we called this in order to bring our object to work.
On line 4: Used the label widget to add a label: “Add subject’s name separated by commas(,)”
Line 5: Used the text () widget to take multiline input from the user.
On lines 6 to 7: Used the 2 button widgets for “Add subjects!” and “Back to home”.
Line 8 to 11: Packed the label, text and button widget.
On line 12: Defined a function named “addsub”
Line 13 to 15: First of all a connection is made to the database attend. Then we used conn.cursor() which helps us to invoke SQLite statements. And the 12th line is simply a command to drop the table if there exists any.
Line 16 to 17: This is the logic to store the entered subjects by the user in the list. And splitting them with a comma (,) using split()
Line 18 to 19: An error message is displayed using the messagebox.showinfo() if no subjects are added.
Line 20 to 29: Now in the else block, we used an SQLite query to create a table of the entered subjects that the user entered.For creating a table we used:
- cur.execute(“CREATE TABLE attable(subid INTEGER,subject TEXT,attended INTEGER,bunked INTEGER)”)
This command creates a table named attable with a field named subid (integer value), subject field (text value), attended field (integer value) and bunked field (integer value)
Now to insert the value into the table we used:
- cur.execute(‘INSERT INTO attable (subid,subject,attended,bunked) VALUES(?,?,?,?)’,(sid,sub,0,0))
This command will insert the values into the table. INSERT INTO <table_name> helps to insert value into the specified table. Inserted these values into the table using a for a loop.
Used commit() and close() in the end and finally displayed a success message.
Class: TodayData
class TodayData(tk.Frame):
def __init__(self,parent,controller):
tk.Frame.__init__(self,parent)
label1=tk.Label(self,text="Enter data of today",font=("Times",24))
label1.pack()
bt2=tk.Button(self,text="Show id of subjects",bg="yellow",font=("Times",16),height=2,width=17,command=lambda:self.showid(controller))
bt1=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage))
lb2=tk.Label(self,text="Input the corresponding Subject ID: ",font=("Times",15))
txt1=tk.Entry(self)
lb2.pack()
txt1.pack()
lb3=tk.Label(self,text="Number of times attended:",font=("Times",15))
txt2=tk.Entry(self)
lb4=tk.Label(self,text="Number of times bunked:",font=("Times",15))
txt3=tk.Entry(self)
lb3.pack()
txt2.pack()
lb4.pack()
txt3.pack()
bt3=tk.Button(self,text="Add to record",bg="orange",font=("Times",16),height=2,width=17,command=lambda:self.addrecord(txt1.get(),txt2.get(),txt3.get()))
bt3.pack()
bt2.pack()
bt1.pack()
def showid(self,controller):
try:
conn=sql.connect("attend")
cur=conn.cursor()
cur.execute('SELECT * FROM attable')
text=""
for w in cur:
text=text+"sub id "+str(w[0])+" "+w[1]+"\n"
messagebox.showinfo("Subject ID: ", text)
conn.commit()
conn.close()
except:
messagebox.showinfo("Alert!", "There is no record")
def addrecord(self,i,p,b):
i=int(i)
if p=="" or p==" " or p=="\n":
p=0
else:
p=int(p)
if b=="" or b==" " or b=="\n":
b=0
else:
b=int(b)
try:
conn=sql.connect("attend")
cur=conn.cursor()
cur.execute("SELECT * FROM attable WHERE subid=?",(i,))
kk=cur.fetchone()
np=kk[2]+p
nb=kk[3]+b
cur.execute("UPDATE attable SET attended = ? WHERE subid= ?",(np,i))
cur.execute("UPDATE attable SET bunked = ? WHERE subid= ?",(nb,i))
conn.commit()
conn.close()
messagebox.showinfo("Alert!", "Done")
except:
messagebox.showinfo("Alert!", "There is no record")
Output:

Explanation for above part of attendance management system project in python with source code:
Line 1: Class declaration with name TodayData.
On line 2: Passed two parameters namely parent and controller other than self.
Line 3: tk.Frame.__init__(self, parent) we called this in order to bring our object to work.
On line 4: We used the label widget to add to display: “Enter data of today”.
Line 5: Packed the label.
Line 6 to 7: Used 2 button widgets to give users the option of “Show id of subjects” and “Back to home”.
On-Line 8 to 9: To take input from the user, we use a label and the Entry() widget.
Line 10 to 11: Packed label and txt1.
Line 12 to 23: Used Label and button widgets to display required labels and in between these lines, did the packing of each widget.
On lines 24 to 36: A function showid() that shows the IDs of the subjects. Here in order to display the IDs, we used the SELECT command to select data from the database and then display it using for loop.
Line 37 to 60: Used a addrecord() function to make it possible for the user to add a record. This is done using a try and except block, wherein in a try block, first, we take the id and corresponding to that id we allow the user to input “Number of times attended” and “Number of times bunked”. Used the UPDATE command for this. And in the end, we used an except block, if the user tries to add a record to that ID that is not present in the database.
main() function:
def main():
app=AttendanceManager()
app.title("Attendance Management - CopyAssignment")
app.mainloop()
Explanation:
This function is a call to AttendanceManager() and sets the title of the window to “Attendance Management – CopyAssignment” using title().
mainloop() instructs Python to run event loops in Tkinter. Used this mainly when our application is ready to run.
Call to main() function:
if __name__=="__main__":
main()
Explanation:
Here, if __name__==”__main__” satisfies then called the main() function. After importing modules, we may use and if name == “main” block to allow or prevent pieces of code from being called. When the Python interpreter reads a file, the name variable is set to main if the module is being run, or to the module’s name if it is imported, .
Complete Code for Attendance Management System Project in Python:
import tkinter as tk from tkinter import messagebox import sqlite3 as sql class AttendanceManager(tk.Tk): def __init__(self,*args,**kwargs): tk.Tk. __init__(self,*args,**kwargs) container=tk.Frame(self) container.pack(side="top",fill="both",expand=True) container.grid_rowconfigure(0,weight=1) container.grid_columnconfigure(0,weight=1) self.frames=dict() for F in (StartPage,NewRecord,ManageAttendance,DeleteRecord,EditRecord,AddSubjects,TodayData): frame=F(container,self) self.frames[F]=frame frame.grid(row=0,column=0,sticky="nsew") self.show_frame(StartPage) def show_frame(self,cont): frame=self.frames[cont] frame.tkraise() class StartPage(tk.Frame): def __init__(self,parent,controller): tk.Frame.__init__(self,parent) label1=tk.Label(self,text="ATTENDANCE MANAGEMENT SYSTEM Project using Python",font=("Times",26)) bt1=tk.Button(self,text="Add new record",font=("Times",16),height=2,width=17,bg="blue" ,command=lambda:controller.show_frame(NewRecord)) bt2=tk.Button(self,text="Manage attendance",font=("Times",16),height=2,width=17,bg="yellow",command=lambda:controller.show_frame(ManageAttendance)) bt3=tk.Button(self,text="Delete record",font=("Times",16),height=2,width=17,bg="red",command=lambda:controller.show_frame(DeleteRecord)) bt4=tk.Button(self,text="Edit record",font=("Times",16),height=2,width=17,bg="orange",command=lambda:controller.show_frame(EditRecord)) label1.pack() bt1.pack() bt2.pack() bt3.pack() bt4.pack() class NewRecord(tk.Frame): def __init__(self,parent,controller): tk.Frame.__init__(self,parent) label1=tk.Label(self,text="New Record",font=("Times",24)) label2=tk.Label(self,text="NOTE: If you want a new record, previous one will be deleted,continue?",font=("Times",14)) bt2=tk.Button(self,text="YES",font=("Times",16),bg="orange",height=2,width=17,command=lambda:controller.show_frame(AddSubjects)) bt3=tk.Button(self,text="NO",font=("Times",16),bg="red",height=2,width=17,command=lambda:controller.show_frame(StartPage)) label1.pack() label2.pack() bt2.pack() bt3.pack() class ManageAttendance(tk.Frame): def __init__(self,parent,controller): tk.Frame.__init__(self,parent) label1=tk.Label(self,text="Manage Attendance",font=("Times",24)) label1.pack() bt2=tk.Button(self,text="Show status", bg="green",font=("Times",16),height=2,width=17,command=lambda:self.showstatus(controller)) bt3=tk.Button(self,text="Today's data", bg="orange",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(TodayData)) bt1=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage)) bt2.pack() bt3.pack() bt1.pack() def showstatus(self,controller): try: conn=sql.connect("attend") cur=conn.cursor() cur.execute('SELECT * FROM attable') text="" for w in cur: if w[2]==0 and w[3]==0: per="0" else: per=w[2]/(w[2]+w[3]) per=per*100 per=str(int(per)) text=text+"sub id "+str(w[0])+" "+w[1]+" "+per+"%\n" messagebox.showinfo("status", text) except: messagebox.showinfo("Alert!", "There is no record") class DeleteRecord(tk.Frame): def __init__(self,parent,controller): tk.Frame.__init__(self,parent) label1=tk.Label(self,text="Delete Record",font=("Times",24)) label2=tk.Label(self,text="This action will delete the record,continue?",font=("Times",12)) bt2=tk.Button(self,text="YES",bg="green",font=("Times",16),height=2,width=17,command=lambda:self.delrecord(controller)) bt1=tk.Button(self,text="NO",bg="yellow",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage)) bt3=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage)) label1.pack() label2.pack() bt2.pack() bt1.pack() bt3.pack() def delrecord(self,controller): conn=sql.connect('attend') cur=conn.cursor() cur.execute('DROP TABLE IF EXISTS attable') conn.commit() conn.close() messagebox.showinfo("Alert!", "records deleted") controller.show_frame(StartPage) class EditRecord(tk.Frame): def __init__(self,parent,controller): tk.Frame.__init__(self,parent) label1=tk.Label(self,text="Edit Record",font=("Times",24)) bt1=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage)) label1.pack() lb2=tk.Label(self,text="Input Subject ID: ",font=("Times",15)) txt1=tk.Entry(self) lb2.pack() txt1.pack() lb3=tk.Label(self,text="Number of times attended:",font=("Times",15)) txt2=tk.Entry(self) lb4=tk.Label(self,text="Number of times bunked:",font=("Times",15)) txt3=tk.Entry(self) lb3.pack() txt2.pack() lb4.pack() txt3.pack() bt3=tk.Button(self,text="Update",bg="green",font=("Times",16),height=2,width=17,command=lambda:self.update(txt1.get(),txt2.get(),txt3.get())) bt2=tk.Button(self,text="Show subjects ID",bg="yellow",font=("Times",16),height=2,width=17,command=lambda:self.showid(controller)) bt2.pack() bt3.pack() bt1.pack() def update(self,i,p,b): i=int(i) if p=="" or p==" " or p=="\n": p=0 else: p=int(p) if b=="" or b==" " or b=="\n": b=0 else: b=int(b) try: conn=sql.connect("attend") cur=conn.cursor() cur.execute("SELECT * FROM attable WHERE subid=?",(i,)) kk=cur.fetchone() np=p nb=b cur.execute("UPDATE attable SET attended = ? WHERE subid= ?",(np,i)) cur.execute("UPDATE attable SET bunked = ? WHERE subid= ?",(nb,i)) conn.commit() conn.close() messagebox.showinfo("Alert!", "Updated") except: messagebox.showinfo("Alert!", "There is no record") def showid(self,controller): try: conn=sql.connect("attend") cur=conn.cursor() cur.execute('SELECT * FROM attable') text="" for w in cur: text=text+"sub id "+str(w[0])+" "+w[1]+"\n" messagebox.showinfo("Subject ID: ", text) conn.commit() conn.close() except: messagebox.showinfo("Alert!", "There is no record") class AddSubjects(tk.Frame): def __init__(self,parent,controller): tk.Frame.__init__(self,parent) label1=tk.Label(self,text="Add subject's name seperated by commas(,)",font=("Times",16)) txt1=tk.Text(self,font=("Times",16),width=48,height=3) bt2=tk.Button(self,text="Add subjects!",bg="orange",font=("Times",16),height=2,width=17,command=lambda:self.addsub(txt1.get("1.0",tk.END),controller)) bt1=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage)) label1.pack() txt1.pack() bt2.pack() bt1.pack() def addsub(self,a,controller): conn=sql.connect('attend') cur=conn.cursor() cur.execute('DROP TABLE IF EXISTS attable') a=a[0:len(a)-1] a=a.split(",") if len(a)==1 and a[0]=="" : messagebox.showinfo("Alert!", "Please enter the subjects") else: sid=1 cur.execute('CREATE TABLE attable(subid INTEGER,subject TEXT,attended INTEGER,bunked INTEGER)') for sub in a: cur.execute('INSERT INTO attable (subid,subject,attended,bunked) VALUES(?,?,?,?)',(sid,sub,0,0)) sid=sid+1 conn.commit() conn.close() messagebox.showinfo("congratulations!", "subjects are added") controller.show_frame(StartPage) class TodayData(tk.Frame): def __init__(self,parent,controller): tk.Frame.__init__(self,parent) label1=tk.Label(self,text="Enter data of today",font=("Times",24)) label1.pack() bt2=tk.Button(self,text="Show id of subjects",bg="yellow",font=("Times",16),height=2,width=17,command=lambda:self.showid(controller)) bt1=tk.Button(self,text="Back to home",bg="red",font=("Times",16),height=2,width=17,command=lambda:controller.show_frame(StartPage)) lb2=tk.Label(self,text="Input the corresponding Subject ID: ",font=("Times",15)) txt1=tk.Entry(self) lb2.pack() txt1.pack() lb3=tk.Label(self,text="Number of times attended:",font=("Times",15)) txt2=tk.Entry(self) lb4=tk.Label(self,text="Number of times bunked:",font=("Times",15)) txt3=tk.Entry(self) lb3.pack() txt2.pack() lb4.pack() txt3.pack() bt3=tk.Button(self,text="Add to record",bg="orange",font=("Times",16),height=2,width=17,command=lambda:self.addrecord(txt1.get(),txt2.get(),txt3.get())) bt3.pack() bt2.pack() bt1.pack() def showid(self,controller): try: conn=sql.connect("attend") cur=conn.cursor() cur.execute('SELECT * FROM attable') text="" for w in cur: text=text+"sub id "+str(w[0])+" "+w[1]+"\n" messagebox.showinfo("Subject ID: ", text) conn.commit() conn.close() except: messagebox.showinfo("Alert!", "There is no record") def addrecord(self,i,p,b): i=int(i) if p=="" or p==" " or p=="\n": p=0 else: p=int(p) if b=="" or b==" " or b=="\n": b=0 else: b=int(b) try: conn=sql.connect("attend") cur=conn.cursor() cur.execute("SELECT * FROM attable WHERE subid=?",(i,)) kk=cur.fetchone() np=kk[2]+p nb=kk[3]+b cur.execute("UPDATE attable SET attended = ? WHERE subid= ?",(np,i)) cur.execute("UPDATE attable SET bunked = ? WHERE subid= ?",(nb,i)) conn.commit() conn.close() messagebox.showinfo("Alert!", "Done") except: messagebox.showinfo("Alert!", "There is no record") def main(): app=AttendanceManager() app.title("Attendance Management - CopyAssignment") app.mainloop() if __name__=="__main__": main()
Reference Links:
Here’s the link to the official documentation of both the libraries:
Endnote:
We hope this article on Attendance Management System Project in Python was a good source of learning for you. This time we used a completely new library and learned a lot about it. We will be back with another amazing project, till then Learn, Execute and Build. Thank you for visiting us.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter