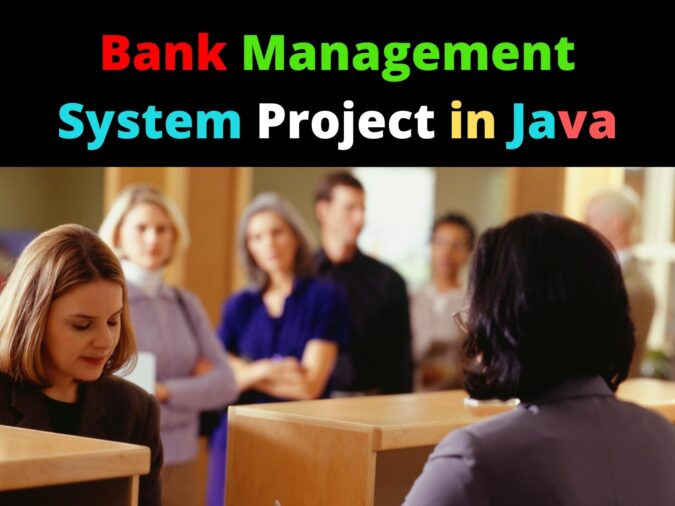
In this article, we will build Bank Management System Project in Java and MySQL. This project is great for those at an intermediate level in Java who want to advance their coding skills. In this project, the users will be able to perform the following functionalities Login, Account details, View account balance, Deposit money and Withdraw the money. Let’s get started!
Bank Management System Project in Java: Project Overview
Project Name: | Bank Management System Project in Java |
Abstract | It’s a GUI-based project used with the Swing module to organize all the elements that work under bank management. |
Language/s Used: | Java |
IDE | IntelliJ Idea Professional(Recommended) |
Java version (Recommended): | Java SE 18.0. 2.1 |
Database: | MySQL |
Type: | Desktop Application |
Recommended for | Final Year Students |
Setting the development environment
You must have java JDK installed on your system and we are using Eclipse IDE to build this project, you can use either this or Netbeans IDE.
The first step will be to create a new project. Name it as you wish. In the src folder create a bank package. In that package, we will be creating some files for different modules.
To connect the system with the database you will need to follow certain steps.
- Have Java JDK already installed and an IDE like Eclipse
- Install MySQL on the system.
- Download MySQL connector from here.
- In Eclipse, under your project expand external libraries and right-click, and select Open Library Settings. Select the libraries tab and click on the + button. Browse your jar file downloaded from the above step and click on it. This will add a dependency to your project. The steps will differ if you are using a different IDE.
MySql Setup for Bank Management System Project in Java
1. Create a database
-- Creating database
create database bank;
2. Select the database
-- Selecting the database
use bank;
3. Create a customer table
-- Creating customer table
create table customer (
id int primary key,
customer_id varchar(25),
customer_name varchar(10),
branch varchar(13),
city varchar(25),
phone varchar(20)
);
4. Create an account table
-- Create account table
create table account (
id int primary key,
account_id varchar(25),
customer_id varchar(25),
int balance
);
5. Create an admin table
-- Create admin table
create table admin (
cust_id varchar(25),
password varchar(25)
);
6. Insert some values in the admin table
-- Inserting values
insert into admin values
("admin1","1234"),
("admin2","9876");
Implementation of Bank Management System Project in Java
1. Login module
This module helps to make a login page so that the user can enter the user name and password to enter into the bank management system in java. Name the file as login.java
package bank; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import javax.swing.JOptionPane; import javax.swing.table.DefaultTableModel; public class login extends javax.swing.JFrame { public login() { initComponents(); } @SuppressWarnings("unchecked") // <editor-fold defaultstate="collapsed" desc="Generated Code"> private void initComponents() { jPanel1 = new javax.swing.JPanel(); jLabel1 = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); txtuser = new javax.swing.JTextField(); txtpass = new javax.swing.JPasswordField(); jButton1 = new javax.swing.JButton(); jButton2 = new javax.swing.JButton(); jLabel3 = new javax.swing.JLabel(); setDefaultCloseOperation(javax.swing.WindowConstants.EXIT_ON_CLOSE); setTitle("Login"); setBackground(new java.awt.Color(0, 102, 255)); setForeground(new java.awt.Color(0, 102, 255)); jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Login")); jLabel1.setText("Username"); jLabel2.setText("Password"); jButton1.setText("Login"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } }); jButton2.setText("Cancel"); jButton2.addMouseListener(new java.awt.event.MouseAdapter() { public void mouseClicked(java.awt.event.MouseEvent evt) { jButton2MouseClicked(evt); } }); Connection con1; PreparedStatement insert; ResultSet rs1; public void load() { try { Class.forName("com.mysql.jdbc.Driver"); con1 = DriverManager.getConnection("jdbc:mysql://localhost/customer","root",""); insert = con1.prepareStatement("SELECT * FROM user WHERE username=? and password=? "); insert.setString(1, txtuser.getText()); insert.setString(2,txtpass.getText()); rs1=insert.executeQuery(); if(rs1.next()) { mainmenu c = new mainmenu(); this.hide(); c.setVisible(true); } else { JOptionPane.showMessageDialog(null, "Username and password do not matched"); txtuser.setText(""); txtpass.setText(""); txtuser.requestFocus(); } } catch (Exception e) { System.out.println("Failed " + e); } }

When the entered user name and password didn’t match the existing records in the database, it will return the “Username and password do not matched”.

Once we enter the correct username and password it takes us to the home page of our bank application.
2. Account details module
In this module, we can create a new account for customers. Name the file as account.java.
package bank; import java.awt.event.KeyEvent; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.JOptionPane; public class account extends javax.swing.JInternalFrame { public account() { initComponents(); autoId(); } Connection con1; PreparedStatement insert; ResultSet rs1; @SuppressWarnings("unchecked") private void initComponents() { txtbal = new javax.swing.JTextField(); jPanel1 = new javax.swing.JPanel(); jLabel3 = new javax.swing.JLabel(); jLabel1 = new javax.swing.JLabel(); txtcust = new javax.swing.JTextField(); jLabel2 = new javax.swing.JLabel(); txtfname = new javax.swing.JTextField(); jLabel7 = new javax.swing.JLabel(); jLabel8 = new javax.swing.JLabel(); jButton2 = new javax.swing.JButton(); jComboBox1 = new javax.swing.JComboBox<>(); jLabel4 = new javax.swing.JLabel(); jButton3 = new javax.swing.JButton(); txtbal.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { txtbalActionPerformed(evt); } }); jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Account")); jLabel3.setText("Account type"); jLabel1.setText("Customer ID"); txtcust.addKeyListener(new java.awt.event.KeyAdapter() { public void keyPressed(java.awt.event.KeyEvent evt) { txtcustKeyPressed(evt); } }); jLabel2.setText("Custmer Name"); jLabel7.setText("Account No"); jLabel8.setFont(new java.awt.Font("Tahoma", 1, 20)); jLabel8.setForeground(new java.awt.Color(0, 102, 255)); jLabel8.setText("jLabel8"); jButton2.setText("Cancel"); jButton2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton2ActionPerformed(evt); } }); jComboBox1.setModel(new javax.swing.DefaultComboBoxModel<>(new String[] { "Saving", "Fix", "Current" })); jLabel4.setText("Balance"); jButton3.setText("Add"); jButton3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton3ActionPerformed(evt); } }); public void autoId() { try { Class.forName("com.mysql.jdbc.Driver"); con1 = DriverManager.getConnection("jdbc:mysql://localhost/customer","root",""); Statement s = con1.createStatement(); ResultSet rs = s.executeQuery("SELECT MAX(acc_id) FROM account"); rs.next(); rs.getString("MAX(acc_id)"); if (rs.getString("MAX(acc_id)") == null) { jLabel8.setText("A0001"); } else { long id = Long.parseLong(rs.getString("MAX(acc_id)").substring(2, rs.getString("MAX(acc_id)").length())); id++; jLabel8.setText("A0" + String.format("%03d", id)); } } catch (Exception ex) { ex.printStackTrace(); } }

We have to enter the required details to create a new account for the customer.
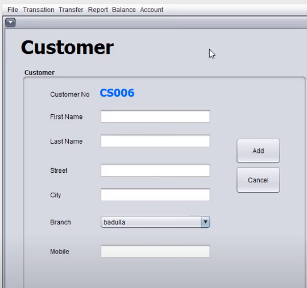
3. View Balance Module
In this module, we can check the balance of our account by entering the required details. Name the file as balance.java
package bank; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.JOptionPane; public class balance extends javax.swing.JInternalFrame { public balance() { initComponents(); } Connection con1; PreparedStatement insert; PreparedStatement insert2; ResultSet rs1; @SuppressWarnings("unchecked") private void initComponents() { jLabel4 = new javax.swing.JLabel(); lbal = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); jPanel1 = new javax.swing.JPanel(); jLabel1 = new javax.swing.JLabel(); txtaccno = new javax.swing.JTextField(); jButton1 = new javax.swing.JButton(); jLabel7 = new javax.swing.JLabel(); jButton3 = new javax.swing.JButton(); jLabel9 = new javax.swing.JLabel(); jLabel5 = new javax.swing.JLabel(); jLabel6 = new javax.swing.JLabel(); jLabel4.setFont(new java.awt.Font("Tahoma", 1, 12)); jLabel4.setText("Balance"); lbal.setFont(new java.awt.Font("Tahoma", 1, 36)); lbal.setForeground(new java.awt.Color(0, 51, 204)); lbal.setText("Balance"); jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Account No")); jLabel1.setFont(new java.awt.Font("Tahoma", 1, 14)); jLabel1.setText("Enter the Acccount No"); jButton1.setText("Find"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } }); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(43, 43, 43) .addComponent(jLabel1)) .addGroup(jPanel1Layout.createSequentialGroup() .addContainerGap() .addComponent(txtaccno, javax.swing.GroupLayout.PREFERRED_SIZE, 239, javax.swing.GroupLayout.PREFERRED_SIZE))) .addGap(0, 12, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup() .addGap(0, 0, Short.MAX_VALUE) .addComponent(jButton1))) .addContainerGap()) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addContainerGap() .addComponent(jLabel1) .addGap(18, 18, 18) .addComponent(txtaccno, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 18, Short.MAX_VALUE) .addComponent(jButton1)) ); jLabel7.setFont(new java.awt.Font("Tahoma", 1, 18)); jLabel7.setText("Customer ID"); jButton3.setText("Cancel"); jButton3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton3ActionPerformed(evt); } }); jLabel9.setText("Customer ID"); jLabel5.setFont(new java.awt.Font("Tahoma", 1, 18)); jLabel5.setText("Firstname"); jLabel6.setFont(new java.awt.Font("Tahoma", 1, 18)); jLabel6.setText("Lastname"); pack(); } private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed String accno = txtaccno.getText(); try { Class.forName("com.mysql.jdbc.Driver"); con1 = DriverManager.getConnection("jdbc:mysql://localhost/customer","root",""); insert = con1.prepareStatement("select c.cust_id,c.firstname,c.lastname,a.balance from customer c,account a where c.cust_id = a.cust_id and a.acc_id = ?"); insert.setString(1, accno); rs1 = insert.executeQuery(); if(rs1.next() == false) { JOptionPane.showMessageDialog(null,"Account No no found"); jLabel5.setText(""); jLabel6.setText(""); lbal.setText(""); } else { String id = rs1.getString(1); String firstname = rs1.getString(2); String laststname = rs1.getString(3); String balance = rs1.getString(4); jLabel7.setText(id.trim()); jLabel5.setText(firstname.trim()); jLabel6.setText(laststname.trim()); lbal.setText(balance.trim()); } } catch (ClassNotFoundException ex) { Logger.getLogger(balance.class.getName()).log(Level.SEVERE, null, ex); } catch (SQLException ex) { Logger.getLogger(balance.class.getName()).log(Level.SEVERE, null, ex); } }

4. Deposit Money Module for bank management system project in java eclipse
In this module, we can add money to our account. Name the file as deposit.java
package bank; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.JOptionPane; public class deposit extends javax.swing.JInternalFrame { public deposit() { initComponents(); date(); } private void initComponents() { jButton3 = new javax.swing.JButton(); jLabel9 = new javax.swing.JLabel(); jLabel4 = new javax.swing.JLabel(); lbal = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); jPanel1 = new javax.swing.JPanel(); jLabel1 = new javax.swing.JLabel(); txtaccno = new javax.swing.JTextField(); jButton1 = new javax.swing.JButton(); txtfname = new javax.swing.JTextField(); txtlame = new javax.swing.JTextField(); jLabel5 = new javax.swing.JLabel(); amount = new javax.swing.JTextField(); jButton2 = new javax.swing.JButton(); jLabel6 = new javax.swing.JLabel(); jLabel7 = new javax.swing.JLabel(); jButton4 = new javax.swing.JButton(); jLabel10 = new javax.swing.JLabel(); jLabel11 = new javax.swing.JLabel(); jButton3.setText("Cancel"); jButton3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton3ActionPerformed(evt); } }); jLabel9.setText("Customer ID"); jLabel4.setFont(new java.awt.Font("Tahoma", 1, 12)); jLabel4.setText("Balance"); lbal.setFont(new java.awt.Font("Tahoma", 1, 24)); lbal.setForeground(new java.awt.Color(0, 51, 204)); lbal.setText("Balance"); jLabel2.setText("Firstname"); jLabel3.setText("Lastname"); jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Account No")); jLabel1.setFont(new java.awt.Font("Tahoma", 1, 14)); jLabel1.setText("Enter the Acccount No"); jButton1.setText("Find"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } }); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(43, 43, 43) .addComponent(jLabel1)) .addGroup(jPanel1Layout.createSequentialGroup() .addContainerGap() .addComponent(txtaccno, javax.swing.GroupLayout.PREFERRED_SIZE, 239, javax.swing.GroupLayout.PREFERRED_SIZE))) .addGap(0, 12, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup() .addGap(0, 0, Short.MAX_VALUE) .addComponent(jButton1))) .addContainerGap()) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addContainerGap() .addComponent(jLabel1) .addGap(18, 18, 18) .addComponent(txtaccno, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 18, Short.MAX_VALUE) .addComponent(jButton1)) ); jLabel5.setFont(new java.awt.Font("Tahoma", 1, 12)); jLabel5.setText("Deposit"); amount.setBackground(new java.awt.Color(172, 3, 3)); amount.setFont(new java.awt.Font("Tahoma", 1, 24)); amount.setForeground(new java.awt.Color(255, 255, 255)); jButton2.setText("OK"); jButton2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton2ActionPerformed(evt); } }); jLabel6.setFont(new java.awt.Font("Tahoma", 1, 18)); jLabel6.setText("jLabel6"); jLabel7.setFont(new java.awt.Font("Tahoma", 1, 18)); jLabel7.setText("jLabel7"); jButton4.setText("Cancel"); jButton4.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton4ActionPerformed(evt); } }); jLabel10.setText("Customer ID"); jLabel11.setText("Date"); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addContainerGap() .addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(layout.createSequentialGroup() .addGap(36, 36, 36) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addComponent(jLabel2) .addGap(18, 18, 18) .addComponent(txtfname, javax.swing.GroupLayout.PREFERRED_SIZE, 156, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(layout.createSequentialGroup() .addComponent(jLabel10) .addGap(18, 18, 18) .addComponent(jLabel7)) .addGroup(layout.createSequentialGroup() .addComponent(jLabel3) .addGap(18, 18, 18) .addComponent(txtlame, javax.swing.GroupLayout.PREFERRED_SIZE, 156, javax.swing.GroupLayout.PREFERRED_SIZE))))) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(82, 82, 82) .addComponent(jLabel4)) .addGroup(layout.createSequentialGroup() .addGap(120, 120, 120) .addComponent(jLabel5)) .addGroup(layout.createSequentialGroup() .addGap(60, 60, 60) .addComponent(lbal))) .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 77, Short.MAX_VALUE) .addComponent(amount, javax.swing.GroupLayout.PREFERRED_SIZE, 158, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(67, 67, 67)))) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addGap(40, 40, 40) .addComponent(jLabel11) .addGap(47, 47, 47) .addComponent(jLabel6) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(18, 18, 18) .addComponent(jButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 82, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(17, 17, 17)) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(39, 39, 39) .addComponent(jLabel4) .addGap(34, 34, 34) .addComponent(lbal)) .addGroup(layout.createSequentialGroup() .addContainerGap() .addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel7) .addComponent(jLabel10)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addComponent(jLabel5) .addGap(14, 14, 14))) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(amount, javax.swing.GroupLayout.PREFERRED_SIZE, 54, javax.swing.GroupLayout.PREFERRED_SIZE) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(txtfname, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel2))) .addGap(28, 28, 28) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel3) .addComponent(txtlame, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 23, Short.MAX_VALUE) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 35, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton4, javax.swing.GroupLayout.PREFERRED_SIZE, 35, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 14, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel11)) .addGap(28, 28, 28)) ); private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed String accno = txtaccno.getText(); try { Class.forName("com.mysql.jdbc.Driver"); con1 = DriverManager.getConnection("jdbc:mysql://localhost/customer","root",""); insert = con1.prepareStatement("select c.cust_id,c.firstname,c.lastname,a.balance from customer c,account a where c.cust_id = a.cust_id and a.acc_id = ?"); insert.setString(1, accno); rs1 = insert.executeQuery(); if(rs1.next() == false) { JOptionPane.showMessageDialog(null,"Account No no found"); txtfname.setText(""); txtlame.setText(""); lbal.setText(""); } else { String id = rs1.getString(1); String firstname = rs1.getString(2); String laststname = rs1.getString(3); String balance = rs1.getString(4); jLabel7.setText(id.trim()); txtfname.setText(firstname.trim()); txtlame.setText(laststname.trim()); lbal.setText(balance.trim()); } } catch (ClassNotFoundException ex) { Logger.getLogger(deposit.class.getName()).log(Level.SEVERE, null, ex); } catch (SQLException ex) { Logger.getLogger(deposit.class.getName()).log(Level.SEVERE, null, ex); } } public void date() { DateTimeFormatter dtf = DateTimeFormatter.ofPattern("yyyy/MM/dd"); LocalDateTime now = LocalDateTime.now(); String date = dtf.format(now); jLabel6.setText(date); }

After giving the necessary details, the amount gets deposited in the corresponding account and the following message is shown.
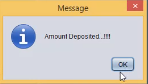
5. Withdraw money module
In this module, we can withdraw money from our account in the bank management system java. Name the file as withdraw.java
package bank; import java.sql.Connection; import java.sql.DriverManager; import java.sql.PreparedStatement; import java.sql.ResultSet; import java.sql.SQLException; import java.time.LocalDateTime; import java.time.format.DateTimeFormatter; import java.util.logging.Level; import java.util.logging.Logger; import javax.swing.JOptionPane; public class withdraw extends javax.swing.JInternalFrame { public withdraw() { initComponents(); date(); } Connection con1; PreparedStatement insert; PreparedStatement insert2; ResultSet rs1; private void initComponents() { jLabel4 = new javax.swing.JLabel(); lbal = new javax.swing.JLabel(); jLabel2 = new javax.swing.JLabel(); jLabel3 = new javax.swing.JLabel(); jPanel1 = new javax.swing.JPanel(); jLabel1 = new javax.swing.JLabel(); txtaccno = new javax.swing.JTextField(); jButton1 = new javax.swing.JButton(); txtfname = new javax.swing.JTextField(); txtlame = new javax.swing.JTextField(); jLabel5 = new javax.swing.JLabel(); amount = new javax.swing.JTextField(); jButton2 = new javax.swing.JButton(); jLabel6 = new javax.swing.JLabel(); jLabel7 = new javax.swing.JLabel(); jButton3 = new javax.swing.JButton(); jLabel8 = new javax.swing.JLabel(); jLabel9 = new javax.swing.JLabel(); jLabel4.setFont(new java.awt.Font("Tahoma", 1, 12)); jLabel4.setText("Balance"); lbal.setFont(new java.awt.Font("Tahoma", 1, 24)); lbal.setForeground(new java.awt.Color(0, 51, 204)); lbal.setText("Balance"); jLabel2.setText("Firstname"); jLabel3.setText("Lastname"); jPanel1.setBorder(javax.swing.BorderFactory.createTitledBorder("Account No")); jLabel1.setFont(new java.awt.Font("Tahoma", 1, 14)); // NOI18N jLabel1.setText("Enter the Acccount No"); jButton1.setText("Find"); jButton1.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton1ActionPerformed(evt); } }); javax.swing.GroupLayout jPanel1Layout = new javax.swing.GroupLayout(jPanel1); jPanel1.setLayout(jPanel1Layout); jPanel1Layout.setHorizontalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGroup(jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addGap(43, 43, 43) .addComponent(jLabel1)) .addGroup(jPanel1Layout.createSequentialGroup() .addContainerGap() .addComponent(txtaccno, javax.swing.GroupLayout.PREFERRED_SIZE, 239, javax.swing.GroupLayout.PREFERRED_SIZE))) .addGap(0, 12, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, jPanel1Layout.createSequentialGroup() .addGap(0, 0, Short.MAX_VALUE) .addComponent(jButton1))) .addContainerGap()) ); jPanel1Layout.setVerticalGroup( jPanel1Layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(jPanel1Layout.createSequentialGroup() .addContainerGap() .addComponent(jLabel1) .addGap(18, 18, 18) .addComponent(txtaccno, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 18, Short.MAX_VALUE) .addComponent(jButton1)) ); jLabel5.setFont(new java.awt.Font("Tahoma", 1, 12)); jLabel5.setText("Withdraw"); amount.setBackground(new java.awt.Color(172, 3, 3)); amount.setFont(new java.awt.Font("Tahoma", 1, 24)); amount.setForeground(new java.awt.Color(255, 255, 255)); jButton2.setText("OK"); jButton2.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton2ActionPerformed(evt); } }); jLabel6.setFont(new java.awt.Font("Tahoma", 1, 18)); jLabel6.setText("jLabel6"); jLabel7.setFont(new java.awt.Font("Tahoma", 1, 18)); jLabel7.setText("jLabel7"); jButton3.setText("Cancel"); jButton3.addActionListener(new java.awt.event.ActionListener() { public void actionPerformed(java.awt.event.ActionEvent evt) { jButton3ActionPerformed(evt); } }); jLabel8.setText("Date"); jLabel9.setText("Customer ID"); javax.swing.GroupLayout layout = new javax.swing.GroupLayout(getContentPane()); getContentPane().setLayout(layout); layout.setHorizontalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addContainerGap() .addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(42, 42, 42) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel2) .addComponent(jLabel3) .addComponent(jLabel8)) .addGap(31, 31, 31)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addContainerGap() .addComponent(jLabel9) .addGap(18, 18, 18))) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(jLabel6) .addComponent(txtlame, javax.swing.GroupLayout.PREFERRED_SIZE, 156, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(txtfname, javax.swing.GroupLayout.PREFERRED_SIZE, 156, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel7)))) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(82, 82, 82) .addComponent(jLabel4)) .addGroup(layout.createSequentialGroup() .addGap(120, 120, 120) .addComponent(jLabel5)) .addGroup(layout.createSequentialGroup() .addGap(60, 60, 60) .addComponent(lbal))) .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, 77, Short.MAX_VALUE) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(amount, javax.swing.GroupLayout.PREFERRED_SIZE, 158, javax.swing.GroupLayout.PREFERRED_SIZE) .addGroup(layout.createSequentialGroup() .addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 89, javax.swing.GroupLayout.PREFERRED_SIZE) .addGap(18, 18, 18) .addComponent(jButton3, javax.swing.GroupLayout.PREFERRED_SIZE, 82, javax.swing.GroupLayout.PREFERRED_SIZE))) .addGap(36, 36, 36)))) ); layout.setVerticalGroup( layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addGap(39, 39, 39) .addComponent(jLabel4) .addGap(34, 34, 34) .addComponent(lbal)) .addGroup(layout.createSequentialGroup() .addContainerGap() .addComponent(jPanel1, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE))) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.UNRELATED) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel7) .addComponent(jLabel9)) .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED)) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createSequentialGroup() .addComponent(jLabel5) .addGap(14, 14, 14))) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addComponent(amount, javax.swing.GroupLayout.PREFERRED_SIZE, 54, javax.swing.GroupLayout.PREFERRED_SIZE) .addGroup(javax.swing.GroupLayout.Alignment.TRAILING, layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(txtfname, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel2))) .addGap(24, 24, 24) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(txtlame, javax.swing.GroupLayout.PREFERRED_SIZE, javax.swing.GroupLayout.DEFAULT_SIZE, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jLabel3)) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.LEADING) .addGroup(layout.createSequentialGroup() .addPreferredGap(javax.swing.LayoutStyle.ComponentPlacement.RELATED, javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jButton2, javax.swing.GroupLayout.PREFERRED_SIZE, 35, javax.swing.GroupLayout.PREFERRED_SIZE) .addComponent(jButton3, javax.swing.GroupLayout.PREFERRED_SIZE, 35, javax.swing.GroupLayout.PREFERRED_SIZE)) .addGap(47, 47, 47)) .addGroup(layout.createSequentialGroup() .addGap(33, 33, 33) .addGroup(layout.createParallelGroup(javax.swing.GroupLayout.Alignment.BASELINE) .addComponent(jLabel8) .addComponent(jLabel6, javax.swing.GroupLayout.PREFERRED_SIZE, 14, javax.swing.GroupLayout.PREFERRED_SIZE)) .addContainerGap(javax.swing.GroupLayout.DEFAULT_SIZE, Short.MAX_VALUE)))) ); pack(); } private void jButton1ActionPerformed(java.awt.event.ActionEvent evt) {//GEN-FIRST:event_jButton1ActionPerformed String accno = txtaccno.getText(); try { Class.forName("com.mysql.jdbc.Driver"); con1 = DriverManager.getConnection("jdbc:mysql://localhost/customer","root",""); insert = con1.prepareStatement("select c.cust_id,c.firstname,c.lastname,a.balance from customer c,account a where c.cust_id = a.cust_id and a.acc_id = ?"); insert.setString(1, accno); rs1 = insert.executeQuery(); if(rs1.next() == false) { JOptionPane.showMessageDialog(null,"Account No no found"); txtfname.setText(""); txtlame.setText(""); lbal.setText(""); } else { String id = rs1.getString(1); String firstname = rs1.getString(2); String laststname = rs1.getString(3); String balance = rs1.getString(4); jLabel7.setText(id.trim()); txtfname.setText(firstname.trim()); txtlame.setText(laststname.trim()); lbal.setText(balance.trim()); } } catch (ClassNotFoundException ex) { Logger.getLogger(withdraw.class.getName()).log(Level.SEVERE, null, ex); } catch (SQLException ex) { Logger.getLogger(withdraw.class.getName()).log(Level.SEVERE, null, ex); } } public void date() { DateTimeFormatter dtf = DateTimeFormatter.ofPattern("yyyy/MM/dd"); LocalDateTime now = LocalDateTime.now(); String date = dtf.format(now); jLabel6.setText(date); }
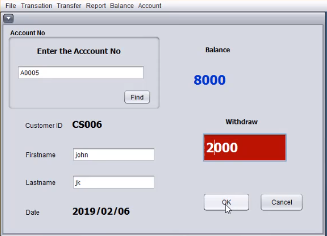
After giving the necessary details, the amount gets deducted from the corresponding account and the following message is shown.

Conclusion
In this project, we developed a GUI-based project, Bank management System with Java and MySQL. The users are able to perform the operations such as login, Account details, View account balance, Deposit money and Withdraw the money. Hope you enjoyed doing this project!
Also Read:
- Dino Game in Java
- Java Games Code | Copy And Paste
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Supply Chain Management System in Java
- Hostel Management System Project in Python
- Survey Management System In Java
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Phone Book in Java
- Brick Breaker Game in Python
- Email Application in Java
- Inventory Management System Project in Java
- Blood Bank Management System Project in Java
- Electricity Bill Management System Project in Java
- CGPA Calculator App In Java
- Chat Application in Java
- CRUD Operations in Servlet & JSP
- 100+ Java Projects for Beginners 2023