Today, we will learn C++ Array Assignment. We can assign multiple values to C++ Arrays. There are many ways you can initialize a C++ array. You can create different datatypes of arrays in C++ e.g. string, int, etc are the two most common types of C++ arrays that are commonly used. Today, we will see how to initialize and assign values to C++ arrays. Let’s start.
Declaring Empty C++ Array
We can declare an empty array in C++ using square brackets and specifying the number of items.
string city[5];
int id[6];
Declaring and C++ Array Assignment
We can declare and initialize a C++ Array at the same time. To initialize a C++ Array while declaring, we can use curly brackets and then values inside curly brackets.
string city[5] = {"Agra", "Delhi", "Noida", "Gurgaon", "Banglore"};
Accessing the elements of the C++ Array
You can use square brackets to access the elements of the C++ Array. Inside square brackets, use the index number of the element you want to access. Indexing in C++ starts from 0.
cout << city[0];
// Outputs Agra
Looping a C++ Array
#include <iostream>
#include <string>
using namespace std;
int main() {
string city[5] = {"Agra", "Delhi", "Noida", "Gurgaon", "Banglore"};
for(int i = 0; i < 5; i++){
cout << city[i] << "\n";
}
return 0;
}
Output:
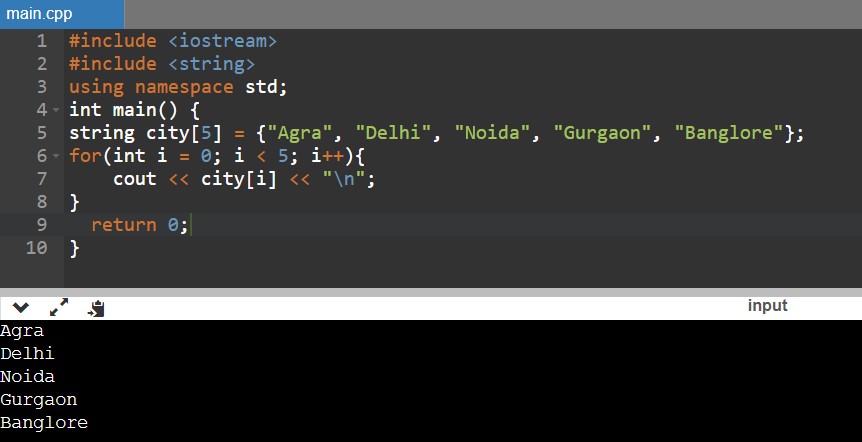
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector