
About Contact Management System in Python
Hello everyone, welcome to violet-cat-415996.hostingersite.com. In this tutorial, we are going to learn about the Contact Management System Project in Python programming language. We are making use of the Tkinter module for creating the GUI- based project of contact management system project in python with source code. Hence making it easy to understand and use.
In this system, we are performing all the operations of adding, viewing, deleting, and updating contact lists. This GUI-based Contact Management system in Python provides the simplest management of contact details. This project mainly focuses on the CRUD operations of the database management system. To run this project, you need to install python on your PC first. This GUI-based project is designed for all python beginners.
So let’s get started with the project
Project Overview: Contact Management System Python Project
Project Name: | Contact Management System Project in Python |
Abstract: | This is a GUI-based program in python that basically includes the use of the Tkinter and Sqlite3 database for the execution. |
Language/Technologies Used: | Python, Tkinter |
IDE | Pycharm(Recommended) |
Database | SQLite3 |
Python version (Recommended): | 3.8 or 3.9 |
Type/Category: | Final Year Project using Python |
Features of Contact Management System
The basic task to be performed on this Project are:
1. Add all the details
2. View the details
3. Update the details
4. Delete the details
5. Exit the System
Use of Pycharm IDE for Project
- First Install Pycharm Community Edition 2021.3.1 (community edition is to be installed)
- Create New Project by clicking on File and selecting New Project, writing the project name, and clicking on “Create”.
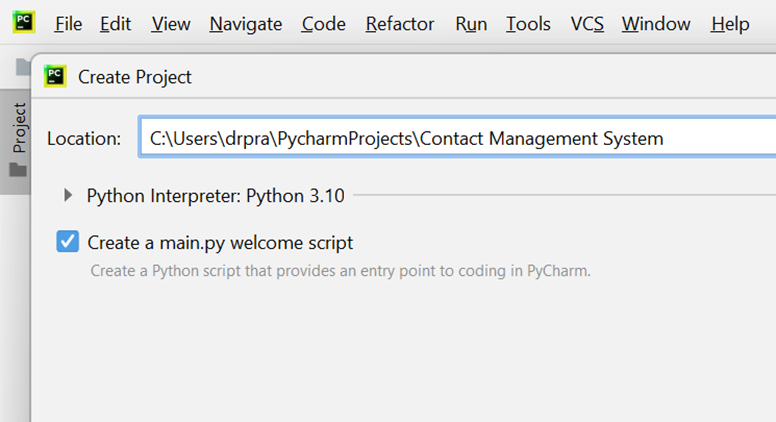
3. Right-click on the project name you have created and Create a New Python File as “contactmanage.py”.

4. Write the code in the file and execute the Python Program for the contact management system by Clicking the Run tab

Note: You can install the modules by going to “File”->” Settings”-> ”Project: New Contact Management”->” Python Interpreter”->click on the”+” sign and write the name of the module want to install.
Code flow: Contact Management System in python with source code
Importing the libraries
#Importing the modules import tkinter import tkinter.ttk as ttk from tkinter import * import sqlite3 import tkinter.messagebox as tkMessageBox
Explanation:
The import function includes these modules in the project
These modules are used for the following purposes:
1. Tkinter – To create the GUI.
2. SQLite3 – To connect the program to the database and store information in it.
3.Tkinter.messagebox – To show a display box, displaying some information or an error or warning
6. Tkinter.ttk – To create the tree where all the information will be displayed.
Declaring the variables for the contact management system python program
# Variables required for storing the values f_name = StringVar() m_name = StringVar() l_name = StringVar() age = StringVar() home_address = StringVar() gender = StringVar() phone_number = StringVar()
Explanation:
Here we are declaring the variables to accept the values in the string format while filling in the details.
Code for exit function of a system
#Function for exiting the system def Exit(): O = tkinter.messagebox.askyesno("Contact Management System", "Do you want to exit the system") if O > 0: root.destroy() return
Explanation:
In the above code, we are defining the exit function where the user is about to take some action to which, the user is to answer yes or no. If yes it will close the GUI else if no then it will return back to the main display of the contact management system in python.
Output:
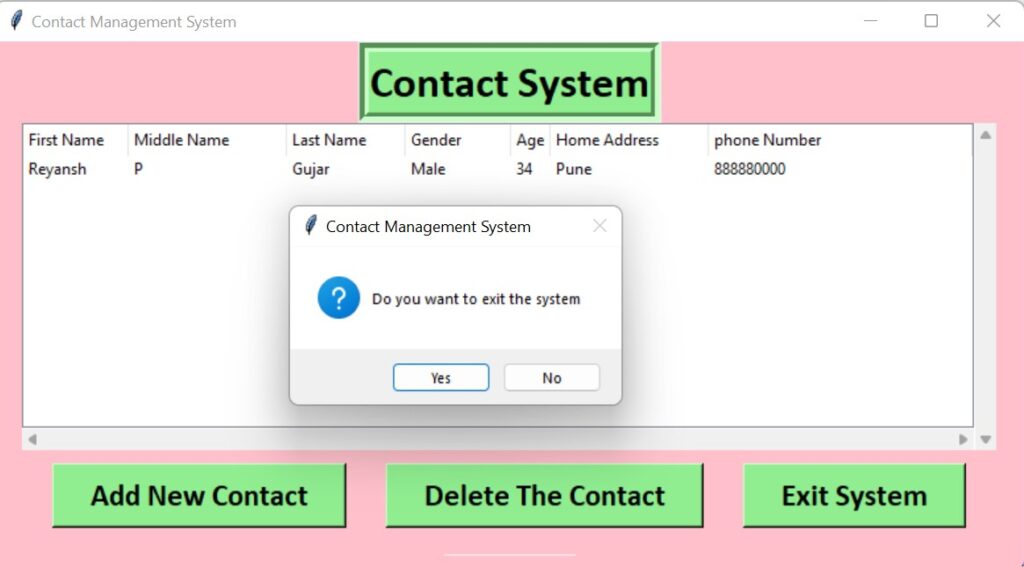
Resetting the values in the system
#Function for resetting the values def Reset(): f_name.set("") m_name.set("") l_name.set("") gender.set("") age.set("") home_address.set("") phone_number.set("")
Explanation:
In this code, the reset function is used for clearing the value you put in the text entry of adding new contacts.
Code for database and table creation
# For creating the database and the table def Database(): connectn = sqlite3.connect("contactdata.db") cursor = connectn.cursor() cursor.execute("CREATE TABLE IF NOT EXISTS `contactinformation` (id INTEGER NOT NULL PRIMARY KEY AUTOINCREMENT, first_name TEXT, middle_name TEXT, last_name TEXT, gender TEXT, age TEXT, home_address TEXT, phone_number TEXT)") cursor.execute("SELECT * FROM `contactinformation` ORDER BY `last_name` ASC") fetchinfo = cursor.fetchall() for data in fetchinfo: tree.insert('', 'end', values=(data)) cursor.close() connectn.close()
Explanation:
In this above code, we are defining the function database where we are creating the “connectn” object to connect the database “contactdata.db” of sqlite. The cursor object is used for the functioning of the SQLite functions .i.e cursor.execute() function will create the table “contactinformation “ if it doesn’t exist and cursor.fetchall() function fetches all the data into the variable fetch and later insert it into the tree.
Code for inserting contacts in the system
Insert Query for inserting into the database
#Insert query for inserting the value in database Table def Submit(): if f_name.get() == "" or m_name.get() == "" or l_name.get() == "" or gender.get() == "" or age.get() == "" or home_address.get() == "" or phone_number.get() == "": msgg = tkMessageBox.showwarning('', 'Please Complete All the Fields', icon="warning") else: tree.delete(*tree.get_children()) connectn = sqlite3.connect("contactdata.db") cursor = connectn.cursor() cursor.execute("INSERT INTO `contactinformation` (first_name, middle_name, last_name, gender, age, home_address, phone_number ) VALUES(?, ?, ?, ?, ?, ?, ?)", (str(f_name.get()), str(m_name.get()), str(l_name.get()), str(gender.get()), int(age.get()), str(home_address.get()), int(phone_number.get()))) connectn.commit() cursor.execute("SELECT * FROM `contactinformation` ORDER BY `last_name` ASC") fetchinfo = cursor.fetchall() for data in fetchinfo: tree.insert('', 'end', values=(data)) cursor.close() connectn.close() f_name.set("") m_name.set("") l_name.set("") gender.set("") age.set("") home_address.set("") phone_number.set("")
Explanation:
The function def submit(): is used for actually inserting the values in the fields. This method demonstrates how to execute INSERT Query from python to add a new row into the SQLite table. In the first steps, it will check if any of the fields are empty and if the value is not filled in the text field it displays a message as” Please Complete All the Fields”. Otherwise once all the text fields are filled it executes the INSERT query and put the details into the database.
Module for frame, labels, text entry, and button for adding new contact form window
#For creating the frame, labels, text entry, and button for add new contact form window def MyNewContact(): global opennewwindow f_name.set("") m_name.set("") l_name.set("") gender.set("") age.set("") home_address.set("") phone_number.set("") Opennewwindow = Toplevel() Opennewwindow.title("Contact Details") Opennewwindow.resizable(0, 0) Opennewwindow.geometry("500x500+0+0") if 'UpdateWindow' in globals(): UpdateWindow.destroy() #############Frames#################### FormTitle = Frame(Opennewwindow) FormTitle.pack(side=TOP) ContactForm = Frame(Opennewwindow) ContactForm.pack(side=TOP, pady=10) RadioGroup = Frame(ContactForm) Male = Radiobutton(RadioGroup, text="Male", variable=gender, value="Male", font=('Calibri', 14)).pack(side=LEFT) Female = Radiobutton(RadioGroup, text="Female", variable=gender, value="Female", font=('Calibri', 14)).pack(side=LEFT) # ===================LABELS============================== label_title = Label(FormTitle, text="Adding New Contacts", bd=12, fg="black", bg="Lightgreen", font=("Calibri", 15, "bold"), pady=2) label_title.pack(fill=X) label_FirstName = Label(ContactForm, text="First Name", font=('Calibri', 14), bd=5) label_FirstName.grid(row=0, sticky=W) label_MiddleName = Label(ContactForm, text="Middle Name", font=('Calibri', 14), bd=5) label_MiddleName.grid(row=1, sticky=W) label_LastName = Label(ContactForm, text="Last Name", font=('Calibri', 14), bd=5) label_LastName.grid(row=2, sticky=W) label_Gender = Label(ContactForm, text="Gender", font=('Calibri', 14), bd=5) label_Gender.grid(row=3, sticky=W) label_Age = Label(ContactForm, text="Age", font=('Calibri', 14), bd=5) label_Age.grid(row=4, sticky=W) label_HomeAddress = Label(ContactForm, text="Home Address", font=('Calibri', 14), bd=5) label_HomeAddress.grid(row=5, sticky=W) label_PhoneNumber = Label(ContactForm, text="Phone Number", font=('Calibri', 14), bd=5) label_PhoneNumber.grid(row=6, sticky=W) # ===================ENTRY=============================== FirstName = Entry(ContactForm, textvariable=f_name, font=('Calibri', 14, 'bold'), bd=3, width=20, justify='left') FirstName.grid(row=0, column=1) MiddleName = Entry(ContactForm, textvariable=m_name, font=('Calibri', 14, 'bold'), bd=3, width=20, justify='left') MiddleName.grid(row=1, column=1) LastName = Entry(ContactForm, textvariable=l_name, font=('Calibri', 14, 'bold'), bd=3, width=20, justify='left') LastName.grid(row=2, column=1) RadioGroup.grid(row=3, column=1) Age = Entry(ContactForm, textvariable=age, font=('Calibri', 14, 'bold'), bd=3, width=20, justify='left') Age.grid(row=4, column=1) HomeAddress = Entry(ContactForm, textvariable=home_address, font=('Calibri', 14, 'bold'), bd=3, width=20, justify='left') HomeAddress.grid(row=5, column=1) PhoneNumber = Entry(ContactForm, textvariable=phone_number, font=('Calibri', 14, 'bold'), bd=3, width=20, justify='left') PhoneNumber.grid(row=6, column=1) # ==================BUTTONS============================== ButtonAddContact = Button(ContactForm, text='Please Save', bd=5, font=('Calibri', 12, 'bold'), fg="black", bg="lightgreen", command=Submit) ButtonAddContact.grid(row=7, columnspan=2, pady=10)

Code for updating the contacts in the system
Update Query for updating the database
#Update Query for updating the table in the database def Update(): if gender.get() == "": msgg = tkMessageBox.showwarning('', 'Please Complete The Required Field', icon="warning") else: tree.delete(*tree.get_children()) connectn = sqlite3.connect("contactdata.db") cursor = connectn.cursor() cursor.execute("UPDATE `contactinformation` SET `first_name` = ?, `middle_name` = ? , `last_name` = ?, `gender` =?, `age` = ?, `home_address` = ?, `phone_number` = ? WHERE `id` = ?", (str(f_name.get()), str(m_name.get()), str(l_name.get()), str(gender.get()), int(age.get()), str(home_address.get()), str(phone_number.get()), int(id))) connectn.commit() cursor.execute("SELECT * FROM `contactinformation` ORDER BY `last_name` ASC") fetchinfo = cursor.fetchall() for data in fetchinfo: tree.insert('', 'end', values=(data)) gender1 = gender.get() if not gender1: tkMessageBox.showerror("Please select the gender") cursor.close() connectn.close() f_name.set("") m_name.set("") l_name.set("") gender.set("") age.set("") home_address.set("") phone_number.set("")
Explanation:
Here we are providing the UPDATE query which allows us to UPDATE the row that is already present in the database. It will generate an error message as” ‘Please Complete The Required Field” specifically for gender if one of the fields is left to be filled.
For updating the row in the database you have to double-click the row which you want to update and perform the further operation.
Module to update contact form window
#Module for the update contact form window def UpdateContact(event): global id, UpdateWindow curItem = tree.focus() contents = (tree.item(curItem)) item = contents['values'] id = item[0] f_name.set("") m_name.set("") l_name.set("") gender.set("") age.set("") home_address.set("") phone_number.set("") f_name.set(item[1]) m_name.set(item[2]) l_name.set(item[3]) age.set(item[5]) home_address.set(item[6]) phone_number.set(item[7]) UpdateWindow = Toplevel() UpdateWindow.title("Contact Information") UpdateWindow.geometry("500x520+0+0") UpdateWindow.resizable(0, 0) if 'Opennewwindow' in globals(): Opennewwindow.destroy()
The code after execution gives the following result:
Output
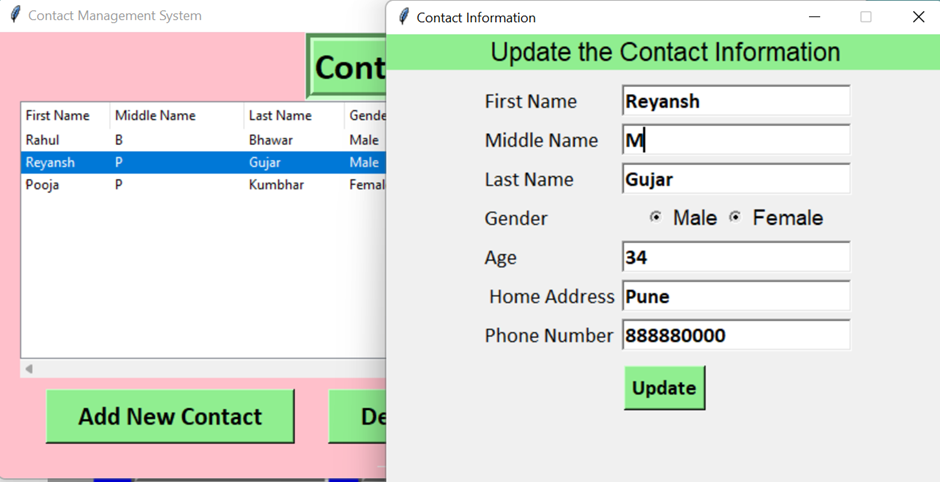
Delete query for deleting the database
#Delete query for deleting the value def Delete(): if not tree.selection(): msgg = tkMessageBox.showwarning('', 'Please Select the data!', icon="warning") else: msgg = tkMessageBox.askquestion('', 'Are You Sure You Want To Delete', icon="warning") if msgg == 'yes': curItem = tree.focus() contents = (tree.item(curItem)) item = contents['values'] tree.delete(curItem) connectn = sqlite3.connect("contactdata.db") cursor = connectn.cursor() cursor.execute("DELETE FROM `contactinformation` WHERE `id` = %d" % item[0]) connectn.commit() cursor.close() connectn.close()
Explanation:
In this code, the user is asked about some action to which, the user answers yes or no. If yes it will delete the contact record in the table and if not it will remain the contact record in the table.
Output
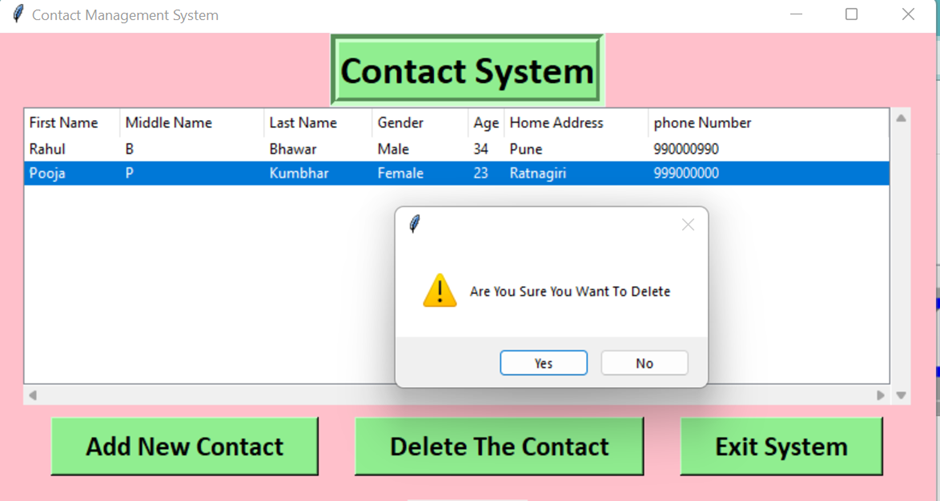
Code for creating a table in the contact management system window
#creating a tables in contact management system scrollbarx = Scrollbar(TableMargin, orient=HORIZONTAL) scrollbary = Scrollbar(TableMargin, orient=VERTICAL) tree = ttk.Treeview(TableMargin, columns=("Id", "First Name", "Middle Name", "Last Name", "Gender", "Age", "Home Address", "Phone Number"), height=400, selectmode="extended", yscrollcommand=scrollbary.set, xscrollcommand=scrollbarx.set) scrollbary.config(command=tree.yview) scrollbary.pack(side=RIGHT, fill=Y) scrollbarx.config(command=tree.xview) scrollbarx.pack(side=BOTTOM, fill=X) tree.heading('Id', text="Id", anchor=W) tree.heading('First Name', text="First Name" ,anchor=W) tree.heading('Middle Name', text="Middle Name", anchor=W) tree.heading('Last Name', text="Last Name", anchor=W) tree.heading('Gender', text="Gender", anchor=W) tree.heading('Age', text="Age", anchor=W) tree.heading('Home Address', text="Home Address", anchor=W) tree.heading('Phone Number', text="phone Number", anchor=W) tree.column('#0', stretch=NO, minwidth=0, width=0) tree.column('#1', stretch=NO, minwidth=0, width=0) tree.column('#2', stretch=NO, minwidth=0, width=80) tree.column('#3', stretch=NO, minwidth=0, width=120) tree.column('#4', stretch=NO, minwidth=0, width=90) tree.column('#5', stretch=NO, minwidth=0, width=80) tree.column('#6', stretch=NO, minwidth=0, width=30) tree.column('#7', stretch=NO, minwidth=0, width=120) tree.pack() tree.bind('<Double-Button-1>', UpdateContact)
Here we can see that we have created a table for inserting the values First name, Middle name, Last name, Gender, Age, Address, Phone Number of the user for that we have made use of scrollbarx and scrollbary for scrolling horizontally and vertically.tree.pack() is used to organize the widgets in boxes or blocks.
Complete Source code of contact management system in Python
Summary
This article on the contact management system in python helps us to put our ideas and skills and develop a user-friendly GUI- based project using the Tkinter modules where we are able to perform all the CRUD operations.
We hope this article on Contact management system in Python with sqlite database is beneficial for you. For more articles like this keep visiting our site.
Thank you for watching this article.
Also Read:
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Music Recommendation System in Machine Learning
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- 100+ Java Projects for Beginners 2023
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Courier Tracking System in HTML CSS and JS