
What are CRUD Operations in Java?
In this article, we are going to learn about the CRUD operations in Java with implementation. CRUD is an acronym for the four operations Create, Read, Update and Delete. The four CRUD operations can be called by users to perform different types of operations on selected data within the database. This could be accomplished on the command line or through a graphical user interface. Let’s review each of the four components in-depth with implementation in Java.
Create in CRUD Operations
The Create function allows users to create a new record in the database. In the SQL relational database application, the Create function is called INSERT. For example, in a restaurant, the details of the menu are stored with the attributes, such as dish, cooking time, and price. If the chef decides to add a new dish to the menu, a new record will be created in the database.
Read or Retrieve in CRUD Operations
The Read function is similar to a SEARCH function. It allows users to search and retrieve specific records in the table and read their values. For example, in the restaurant, from the menu stored the staff wants to look for the price of a dish. Then he/she might retrieve and look for the price of the dish from the stored data.
Update in CRUD Operations
The Update function is used to modify existing records that exist in the database. Users may have to modify information in multiple fields to fully change a record. For example, in a restaurant, the manager decides to replace the price of a dish. As a result, the existing record in the database must be changed.
Delete in CRUD Operations
The Delete function allows users to remove records from a database that are no longer needed. For example, in a restaurant, the manager decides to delete a dish permanently from the data. As a result, the existing record in the database will be deleted.
To know more about what are crud operations in a database, click here.
Applications of CRUD Operations in Java
CRUD operations in Java are widely used in many applications supported by relational databases. CRUD is used to describe user interface conventions that allow to view, search, and change information. Most applications we use let us add or create new entries, search for existing ones, make changes to them or delete them. A very common application of the CRUD operations is the Contact list in Smartphones.
Here is the list of some applications of CRUD:
- Filling up the Sign-UP form on any website is a CREATE operation of CRUD
- Checking bank balances is an example of the READ operation in CRUD.
- Whenever you make transactions in the bank, your balance gets updated which is an example of an UPDATE operation in CRUD.
- Deleting a contact from your contact list is an example of the DELETE operation of CRUD.
Example of CRUD operations
Let’s look at an example of how CRUD is implemented. When a new employee is hired, the HR department creates a record for the employee. If the company needs to send a letter to one or more employees, the read function might be used to find the correct mailing address for the employee. If an employee’s salary or contact information changes, the HR department may need to update the existing record of the employee. If an employee leaves the company, the company may choose to delete their information in the database.
Implementation of CRUD operations in Java
To start with the implementation part, we have to create a class crudOperation. Then create a list Employee to store the information about the employee.
// Implementation of CRUD operations in Java class crudOperation { public static void main(String args[]) { List < Employee > c = new ArrayList < Employee > (); Scanner s = new Scanner(System.in); Scanner s1 = new Scanner(System.in); int ch; do { System.out.println("1.INSERT"); System.out.println("2.DISPLAY"); System.out.println("3.SEARCH"); System.out.println("4.DELETE"); System.out.println("5.UPDATE"); System.out.print("Enter your choice : "); ch = s.nextInt();
Insert in CRUD operations in Java
For implementing the INSERT operation, get the Employee details from the user such as Employee no, Employee name, and Employee Salary. Once the user gives the details, insert the details to the Employee list using add function in the Collections.
switch (ch) { //Insert Operation case 1: System.out.print("Enter Empno : "); int eno = s.nextInt(); System.out.print("Enter Empname : "); String ename = s1.nextLine(); System.out.print("Enter Salary : "); int salary = s.nextInt(); c.add(new Employee(eno, ename, salary)); System.out.println("---------------------"); System.out.println("Record Inserted Sucessfully"); System.out.println("---------------------"); break;
Output for Insert Operation:
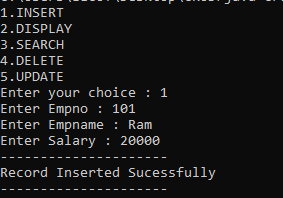
Display in CRUD operations in Java
To display the Employee details we have to iterate through the details of the employee stored in the list. For this operation, we are using Iterator in Collections.
//Display Operation case 2: System.out.println("---------------------"); Iterator < Employee > i = c.iterator(); while (i.hasNext()) { Employee e = i.next(); System.out.println(e); } System.out.println("---------------------"); break;
Output for Display Operation:

Read in CRUD operations in Java
For Search or Read Operation, we use the same iterator object and traverse through each record to find the details we want. After finding the details we require it is displayed in the output console to the user.
//Search Operation case 3: boolean found = false; System.out.print("Enter Empno to Search : "); int empno = s.nextInt(); System.out.println("---------------------"); i = c.iterator(); while (i.hasNext()) { Employee e = i.next(); if (e.getEmpno() == empno) { System.out.println(e); found = true; } } if (!found) { System.out.println("Record Not Found"); } System.out.println("---------------------"); break;
Output for Search Operation:
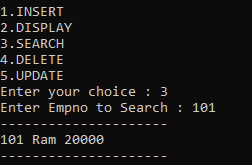
Delete in CRUD operations in Java
Delete operation is performed by using the remove method in Collections. We iterate through the details to find the record which we want to remove, then if the element to delete is found, perform the remove operation to delete that detail permanently from the Employee details.
//Delete Operation case 4: found = false; System.out.print("Enter Empno to Delete : "); empno = s.nextInt(); System.out.println("---------------------"); i = c.iterator(); while (i.hasNext()) { Employee e = i.next(); if (e.getEmpno() == empno) { i.remove(); found = true; } } if (!found) { System.out.println("Record Not Found"); } else { System.out.println("Record is Deleted Sucessfully"); } System.out.println("---------------------"); break;
Output for Delete Operation:

Update in CRUD operations in Java
For the Update operation, we will perform the modifications of the Employee details using a set function in the Collections. The employee name whose details are to be updated is entered by the user, then the new details which need to be rewritten will be received from the user, and an update of the details takes place.
//Update Operation case 5: found = false; System.out.print("Enter Empno to Update : "); empno = s.nextInt(); ListIterator < Employee > li = c.listIterator(); while (li.hasNext()) { Employee e = li.next(); if (e.getEmpno() == empno) { System.out.print("Enter new Name : "); ename = s1.nextLine(); System.out.print("Enter new Salary : "); salary = s.nextInt(); li.set(new Employee(empno, ename, salary)); found = true; } } System.out.println("---------------------"); if (!found) { System.out.println("Record Not Found"); } else { System.out.println("Record is Updated Sucessfully"); } System.out.println("---------------------"); break;
Output for Update operation:

Complete code for CRUD Operations in Java
Here is the complete code to execute the CRUD operations in Java.
import java.util.*; class Employee { private int empno; private String ename; private int salary; Employee(int empno, String ename, int salary) { this.empno = empno; this.ename = ename; this.salary = salary; } public int getEmpno() { return empno; } public int getSalary() { return salary; } public String getEname() { return ename; } public String toString() { return empno + " " + ename + " " + salary; } } class crudOperation { public static void main(String args[]) { List < Employee > c = new ArrayList < Employee > (); Scanner s = new Scanner(System.in); Scanner s1 = new Scanner(System.in); int ch; do { System.out.println("1.INSERT"); System.out.println("2.DISPLAY"); System.out.println("3.SEARCH"); System.out.println("4.DELETE"); System.out.println("5.UPDATE"); System.out.print("Enter your choice : "); ch = s.nextInt(); switch (ch) { //Insert Operation case 1: System.out.print("Enter Empno : "); int eno = s.nextInt(); System.out.print("Enter Empname : "); String ename = s1.nextLine(); System.out.print("Enter Salary : "); int salary = s.nextInt(); c.add(new Employee(eno, ename, salary)); System.out.println("---------------------"); System.out.println("Record Inserted Sucessfully"); System.out.println("---------------------"); break; //Display Operation case 2: System.out.println("---------------------"); Iterator < Employee > i = c.iterator(); while (i.hasNext()) { Employee e = i.next(); System.out.println(e); } System.out.println("---------------------"); break; //Search Opeartion case 3: boolean found = false; System.out.print("Enter Empno to Search : "); int empno = s.nextInt(); System.out.println("---------------------"); i = c.iterator(); while (i.hasNext()) { Employee e = i.next(); if (e.getEmpno() == empno) { System.out.println(e); found = true; } } if (!found) { System.out.println("Record Not Found"); } System.out.println("---------------------"); break; //Delete Operation case 4: found = false; System.out.print("Enter Empno to Delete : "); empno = s.nextInt(); System.out.println("---------------------"); i = c.iterator(); while (i.hasNext()) { Employee e = i.next(); if (e.getEmpno() == empno) { i.remove(); found = true; } } if (!found) { System.out.println("Record Not Found"); } else { System.out.println("Record is Deleted Sucessfully"); } System.out.println("---------------------"); break; //Update Opeartion case 5: found = false; System.out.print("Enter Empno to Update : "); empno = s.nextInt(); ListIterator < Employee > li = c.listIterator(); while (li.hasNext()) { Employee e = li.next(); if (e.getEmpno() == empno) { System.out.print("Enter new Name : "); ename = s1.nextLine(); System.out.print("Enter new Salary : "); salary = s.nextInt(); li.set(new Employee(empno, ename, salary)); found = true; } } System.out.println("---------------------"); if (!found) { System.out.println("Record Not Found"); } else { System.out.println("Record is Updated Sucessfully"); } System.out.println("---------------------"); break; } } while (ch != 0); } }
Output for CRUD operations in Java

Summary
In this article, we have learned about the CRUD operations in Java with implementation. CRUD Operations are frequently used in database and database design cases. To sum up, in CRUD applications, users must be able to create data, have access to the data in the UI by reading the data, update or edit the data, and delete the data.
That’s all for this tutorial, we will have another article to perform all these CRUD operations in Java using JDBC i.e. we will be doing database implementation of these CRUD operations with Java programming language.
Thank you for reading this article.
We hope you enjoyed our article.
Also Read:
- Falling Stars Animation on Python.Hub October 2024
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers