
Introduction
Welcome, my dear friends to copyassignment.com, So let’s begin with our today’s topic without wasting any time. We are going to draw a Christmas Tree using Python Turtle. To draw this we have provided the code line by line along with comments to understand the code easily.
You can check our website page for more understanding of Python’s Turtle and its related projects.
For the code of our topic, please go to the bottom of the page and where you can get the proper code along with its output.
1. Import function
import turtle
Importing the turtle module allows us to use its inbuilt methods and functions in our program.
2. Setting the turtle
window = turtle.Screen() tur=turtle.Turtle() scr=tur.getscreen() scr.title("Merry Christmas") scr.bgcolor("Light Blue")
In this code, we have created the turtle and screen objects. With scr object, we have set the screen’s color and title.
3. Draw the right half of the Christmas tree
tur.color("green")
tur.pensize(5)
tur.begin_fill()
tur.forward(100)
tur.left(150)
tur.forward(90)
tur.right(150)
tur.forward(60)
tur.left(150)
tur.forward(60)
tur.right(150)
tur.forward(40)
tur.left(150)
tur.forward(100)
tur.end_fill()
In this piece of code, we have set the color of the tree to green and drawn the right half of the tree, Forward function is to move forward using the steps i.e forward(90), with left and right we have the set angle position to 150 degrees.
4. Draw the left half
tur.begin_fill()
tur.left(60)
tur.forward(100)
tur.left(150)
tur.forward(40)
tur.right(150)
tur.forward(60)
tur.left(150)
tur.forward(60)
tur.right(150)
tur.forward(90)
tur.left(150)
tur.forward(133)
tur.end_fill()
In this code, we have drawn the right part of the tree the same as the left and filled the tree with green color.
5. Draw the trunk
tur.color("red")
tur.pensize(1)
tur.begin_fill()
tur.right(90)
tur.forward(80)
tur.right(90)
tur.forward(40)
tur.right(90)
tur.forward(80)
tur.end_fill()
Here, we have created the trunk of the tree, and set the color to red We used only the right and forward functions to draw the trunk,
6. Draw balls on the Christmas Tree
tur.penup() tur.color("red") tur.goto(110,-10) tur.begin_fill() tur.circle(10) tur.end_fill() tur.penup() tur.color("red") tur.goto(-120,-10) tur.begin_fill() tur.circle(10) tur.end_fill() tur.penup() tur.color("yellow") tur.goto(100,40) tur.begin_fill() tur.circle(10) tur.end_fill() tur.penup() tur.color("yellow") tur.goto(-105,38) tur.begin_fill() tur.circle(10) tur.end_fill() tur.penup() tur.color("red") tur.goto(85,70) tur.begin_fill() tur.circle(7) tur.end_fill() tur.penup() tur.color("red") tur.goto(-95,70) tur.begin_fill() tur.circle(7) tur.end_fill()
With the above code, we have drawn 6 balls on the tree 3 on both the left and right sides respectively
6. Drawing the bells
tur.shape("triangle")
tur.fillcolor("yellow")
tur.goto(-20,30)
tur.setheading(90)
tur.stamp()
tur.fillcolor("red")
tur.goto(20,60)
tur.setheading(90)
tur.stamp()
tur.goto(-40,75)
tur.setheading(90)
tur.stamp()
.
In the above lines of code, we have simply drawn the bells. These are in the shape of a triangle. We have set the heading position to 90 degrees so that it points North.
The stamp () function puts the triangle shape at the given coordinates on the screen.
7. Draw the star
tur.speed(1)
tur.penup()
tur.color("yellow")
tur.goto(-20,110)
tur.begin_fill()
tur.pendown()
for i in range(5):
tur.forward(40)
tur.right(144)
tur.end_fill()
For the star, we have set the color and position of the star. We have used the for loop so that the turtle moves forward 40 steps and the angle in the right direction of 144 degrees.
8. Write a message On the screen
tur.penup() message="Merry Christmas!!!" tur.goto(-10,-180) tur.color("Orange") tur.pendown() tur.write(message,move=False,align="center",font=("Arial",15,"bold")) tur.hideturtle() turtle.done()
In the last part, we have put the message in the message object. The message object is passed using the write function to display on the screen.
Complete Code to draw Christmas Tree using Python Turtle
#Import the turtle import turtle #Set the turtle screen window = turtle.Screen() tur=turtle.Turtle() scr=tur.getscreen() #title of the screen scr.title("Merry Christmas") #backgroundcolor of screen scr.bgcolor("Light Blue") tur.color("green") tur.pensize(5) tur.begin_fill() # Creating Right half of the tree tur.forward(100) tur.left(150) tur.forward(90) tur.right(150) tur.forward(60) tur.left(150) tur.forward(60) tur.right(150) tur.forward(40) tur.left(150) tur.forward(100) tur.end_fill() #left half of the tree tur.begin_fill() tur.left(60) tur.forward(100) tur.left(150) tur.forward(40) tur.right(150) tur.forward(60) tur.left(150) tur.forward(60) tur.right(150) tur.forward(90) tur.left(150) tur.forward(133) tur.end_fill() #Creating the trunck of the tree tur.color("red") tur.pensize(1) tur.begin_fill() tur.right(90) tur.forward(80) tur.right(90) tur.forward(40) tur.right(90) tur.forward(80) tur.end_fill() #Creating the balls on the Christmas Tree tur.penup() tur.color("red") tur.goto(110,-10) tur.begin_fill() tur.circle(10) tur.end_fill() tur.penup() tur.color("red") tur.goto(-120,-10) tur.begin_fill() tur.circle(10) tur.end_fill() tur.penup() tur.color("yellow") tur.goto(100,40) tur.begin_fill() tur.circle(10) tur.end_fill() tur.penup() tur.color("yellow") tur.goto(-105,38) tur.begin_fill() tur.circle(10) tur.end_fill() tur.penup() tur.color("red") tur.goto(85,70) tur.begin_fill() tur.circle(7) tur.end_fill() tur.penup() tur.color("red") tur.goto(-95,70) tur.begin_fill() tur.circle(7) tur.end_fill() #Drawing the bells using turtle. tur.shape("triangle") tur.fillcolor("yellow") tur.goto(-20,30) tur.setheading(90) tur.stamp() tur.fillcolor("red") tur.goto(20,60) tur.setheading(90) tur.stamp() tur.goto(-40,75) tur.setheading(90) tur.stamp() # Printing the star using for loop tur.speed(1) tur.penup() tur.color("yellow") tur.goto(-20,110) tur.begin_fill() tur.pendown() for i in range(5): tur.forward(40) tur.right(144) tur.end_fill() # Display the message on the screen tur.penup() message="Merry Christmas!!!" tur.goto(-10,-180) tur.color("Orange") tur.pendown() tur.write(message,move=False,align="center",font=("Arial",15,"bold")) tur.hideturtle() turtle.done()
Output
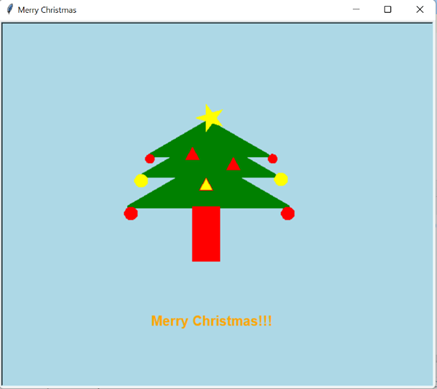
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector