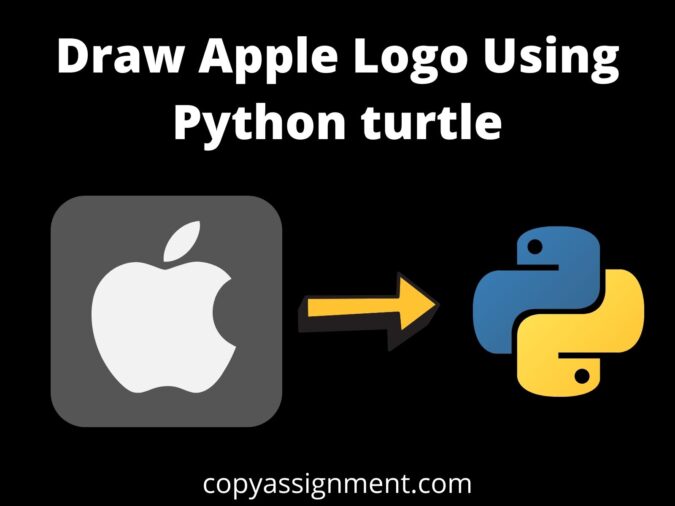
Introduction
Let’s move on to a fantastic project about how to draw Apple Logo Using Python turtle. Before we begin, we will need a Python and Turtle graphics library.
Import the turtle Library
import turtle as t
Setup the Background color of the canvas.
t.begin_fill()
t.Screen().bgcolor('Gray')
Start to Create the Apple Logo.
t.fillcolor('black')
t.left(134)
for i in range(30):
t.forward(1)
t.left(1)
t.right(5)
for i in range(35):
t.forward(1)
t.left(1)
t.left(5)
t.forward(30)
for i in range(15):
t.forward(0.7)
t.right(3)
t.forward(25)
t.left(5)
for i in range(50):
t.forward(1)
t.left(1)
t.right(3)
for i in range(50):
t.forward(1)
t.left(1)
t.right(5)
for i in range(45):
t.forward(2)
t.left(1)
t.right(5)
for i in range(40):
t.forward(2)
t.left(1)
t.left(5)
for i in range(20):
t.forward(1)
t.left(2)
t.left(5)
t.forward(15)
for i in range(9):
t.forward(2)
t.right(3)
t.forward(1)
for i in range(15):
t.forward(1)
t.right(1)
t.right(4)
t.forward(4.5)
t.right(1)
for i in range(27):
t.forward(1)
t.left(2)
t.left(8)
t.forward(5)
for i in range(25):
t.forward(2)
t.left(1)
t.right(3)
t.forward(10)
t.left(83)
for i in range(75):
t.forward(1.3)
t.right(1)
t.right(4)
for i in range(24):
t.forward(1.3)
t.right(1)
t.forward(9.66)
t.end_fill()
t.penup()
t.left(132)
t.forward(100)
t.right(96)
t.pendown()
t.begin_fill()
t.fillcolor('black')
for i in range(60):
t.forward(0.8)
t.right(1)
t.right(120)
for i in range(60):
t.forward(0.8)
t.right(1)
t.hideturtle()
t.end_fill()
t.done()
Source Code To Apple Logo Using Python turtle
import turtle as t t.begin_fill() t.Screen().bgcolor('Gray') t.fillcolor('black') t.left(134) for i in range(30): t.forward(1) t.left(1) t.right(5) for i in range(35): t.forward(1) t.left(1) t.left(5) t.forward(30) for i in range(15): t.forward(0.7) t.right(3) t.forward(25) t.left(5) for i in range(50): t.forward(1) t.left(1) t.right(3) for i in range(50): t.forward(1) t.left(1) t.right(5) for i in range(45): t.forward(2) t.left(1) t.right(5) for i in range(40): t.forward(2) t.left(1) t.left(5) for i in range(20): t.forward(1) t.left(2) t.left(5) t.forward(15) for i in range(9): t.forward(2) t.right(3) t.forward(1) for i in range(15): t.forward(1) t.right(1) t.right(4) t.forward(4.5) t.right(1) for i in range(27): t.forward(1) t.left(2) t.left(8) t.forward(5) for i in range(25): t.forward(2) t.left(1) t.right(3) t.forward(10) t.left(83) for i in range(75): t.forward(1.3) t.right(1) t.right(4) for i in range(24): t.forward(1.3) t.right(1) t.forward(9.66) t.end_fill() t.penup() t.left(132) t.forward(100) t.right(96) t.pendown() t.begin_fill() t.fillcolor('black') for i in range(60): t.forward(0.8) t.right(1) t.right(120) for i in range(60): t.forward(0.8) t.right(1) t.hideturtle() t.end_fill() t.done()
Output

As you can see, we were able to perfectly draw the Apple logo with Python Turtle. I hope you found this tutorial interesting and informative. For more articles like this check out our website violet-cat-415996.hostingersite.com
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector