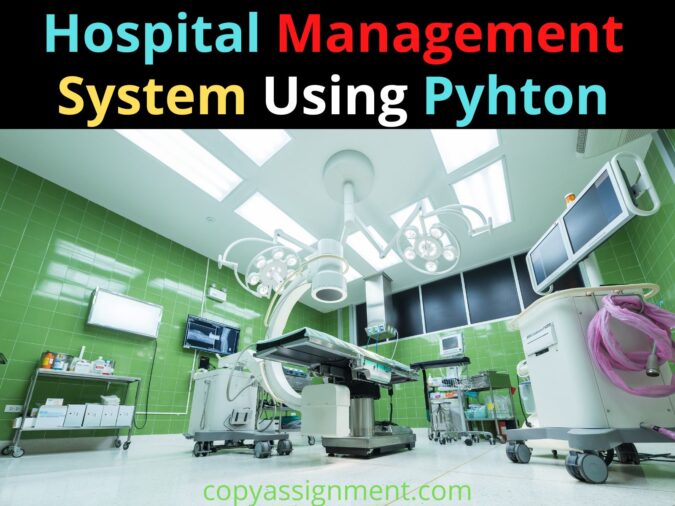
About Hospital Management System in Python
In this article on the hospital management system Project in Python with source code, we are going to learn the entire process step by step. We are going to learn starting from the Registration to the discharge of the patient using Python Programming Language.
We all are aware of the Hospital management work. There comes the registration of new patients, the bed, the doctor’s details, and the medicines information. The nurses’ and the worker’s details and lastly the discharge summary of the patient.
Table of Contents
- About Hospital Management System in Python?
- What are Softwares Used?
- Features and Benefits of Hospital Management System in Python
- Use of Pycharm IDE for Project creation of Hospital Management System
- Installing Mysql Server Database and MySQL-connector-python
- Python Program of Hospital Management system with source code and Output
What are Softwares Used?
Operating System | Windows 10 |
Python Version | 3.10.2 |
Database | MySQL |
Pycharm IDE | For Project Creation |
mysql-connector-python | For database connectivity with the program |
Features and Benefits of Hospital Management System in Python
- Here as we are using MySQL for database creation in Hospital Management, we are able to
- Register new patient
- View the staff, patient information, discharge, and bill payment summary details.
- Delete the details.
- We can add, delete and modify and view the details of the doctors, nurses, and workers.
Use of Pycharm IDE for Project creation of Hospital Management System in python
- First Install Pycharm Community Edition 2021.3.1 (community edition is to be installed)
- Create New Project and enter “Create”.
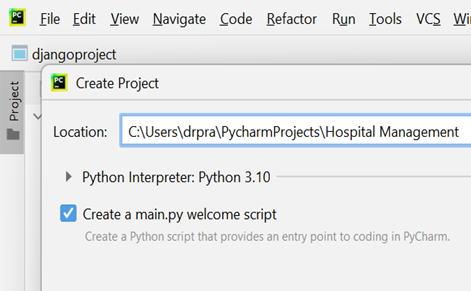
3. Right-click on the project name you have created and Create a New Python File as “hospitalmanage.py”
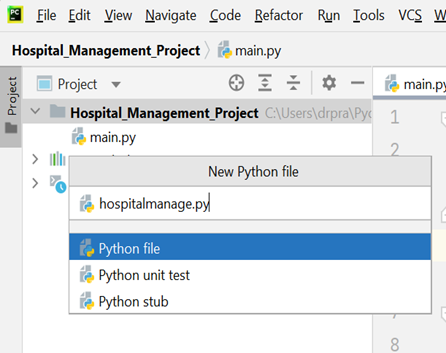
Write the code in the file and Perform the MySQL database connectivity.
4. Execute the Python Program for hospital management system in python

Installing MySQL Server Database and mysql-connector-python
- Download mysql-installer community 8.0.29.0 and install MySQL Database.
- Complete Guide to install MySQL Server Database and mysql-connector-python
- Before Importing the mysql.connector in the program install the connector using the command “ pip3 install mysql-connector-python” in the terminal or command prompt to perform the database connectivity.
Now as we have performed all the database connectivity activity we will understand the code in detail of the Hospital Management System in Python.
Program of Hospital Management system in python with source code and Output
Import the module and perform database connectivity
##creating database connectivity
import mysql.connector
passwd = str(input("Enter the Password Please!!:"))
mysql = mysql.connector.connect(host="localhost", user="root", passwd="your password")
mycursor = mysql.cursor()
mycursor.execute("create database if not exists city_hospitals")
mycursor.execute("use city_hospitals")
Here, we are importing the module mysql.connector to perform all the database connectivity. Use the while loop while writing the code.
The connect() function accepts connection credentials and returns an object of type MySQLConnection for database connection. The mysql.cursor() to communicate with the MySQL database. The mysql.execute() executes the database operations for such as patient details, doctors details, nurse details, and other worker details.
Output

Perform New Registration
u = input("Input your username!!:")
p = input("Input the password (Password must be strong!!!:")
mycursor.execute("insert into user_data values('" + u + "','" + p + "')")
mysql.commit()
In this code block, the patients register themselves by providing a username and password.
Output

Display the list of options the user could select in the Hospital
#enter the username and password of the user whose data is to display
a = int(input("ENTER YOUR CHOICE:"))
# if user wants to enter administration option
if a == 1:
print("""
1. Display the details
2. Add a new member
3. Delete a member
4. Make an exit
""")
b = int(input("Enter your Choice:"))
if b == 1:
print("""
1. Doctors Details
2. Nurse Details
3. Others
""")
In this code block, as we provide the choice option, we can select which service to choose from the list of options.
Output


Adding the data of the administration staff for the Hospital Management System in Python
""")
c = int(input("ENTER YOUR CHOICE:"))
# FOR ENTERING DETAILS OF DOCTORS
if c == 1:
# ASKING THE DETAILS
name = input("Enter the doctor's name")
spe = input("Enter the specilization:")
age = input("Enter the age:")
add = input("Enter the address:")
cont = input("Enter Contact Details:")
fees = input("Enter the fees:")
ms = input("Enter Monthly Salary:")
mycursor.execute(
"insert into doctor_details values('" + name + "','" + spe + "','" + age + "','" + add + "','" + cont + "','" + fees + "','" + ms + "')")
mysql.commit()
print("SUCCESSFULLY ADDED")
ct No.:")
Selecting the add the member choice, the system asks to choose from the administration staff whose information wants to add the doctor, the nurse, or the worker. Similarly, we can add the details of the other staff members
Output
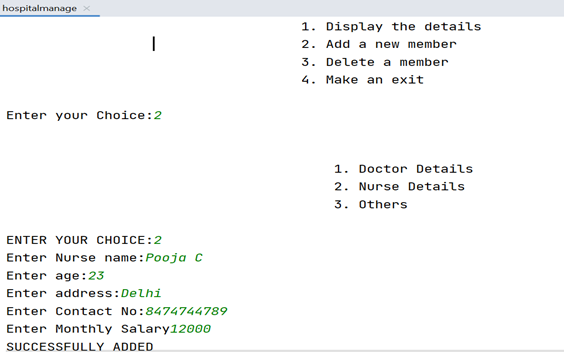
Deleting the details of the administration staff
#if unser wants to delete data
""")
c = int(input("Enter your Choice:")
if c == 1:
name = input("Enter Doctor's Name:")
mycursor.execute("select * from doctor_details where name='" + name + "'")
row = mycursor.fetchall()
print(row)
p = input("you really wanna delete this data? (y/n):")
if p == "y":
mycursor.execute("delete from doctor_details where name='" + name + "'")
mysql.commit()
print("SUCCESSFULLY DELETED!!")
else:
print("NOT DELETED")
Here after entering the name of the staff MySQL executes the queries and fetches data from the databases of name using the fetchall() function deletes the details and prints the message successfully deleted else not deleted.
Output

Displaying the patient’s information and adding the new patient
#Display thee details of the patient by selecting the option
b = int(input("Enter your Choice:"))
if b == 1:
mycursor.execute("select * from patient_details")
row = mycursor.fetchall()
for i in row:
b = 0
v = list(i)
k = ["NAME", "SEX", "AGE", "ADDRESS", "CONTACT"]
d = dict(zip(k, v))
print(d)
# adding new patient
elif b == 2:
name = input("Enter your name ")
sex = input("Enter the gender: ")
age = input("Enter age: ")
address =input("Enter address: ")
contact = input("Contact Details: ")
mycursor.execute(
"insert into patient_details values('" + name + "','" + sex + "','" +
age + "','" + address + "','" + contact + "')")
mysql.commit()
mycursor.execute("select * from patient_details")
for i in mycursor:
v = list(i)
k = ['NAME', 'SEX', 'AGE', 'ADDRESS', 'CONTACT']
print(dict(zip(k, v)))
In this code, we are able to display the information of the patient. The system executes the query and fetches the patient’s information using the fetchall() function.
We are able to add new patient details to the database and complete the information with the commit operation.
Output

Discharge process of the patient and accounting of the bills
name = input("Enter the Patient Name:")
mycursor.execute("select * from patient_details where name='" + name + "'")
row = mycursor.fetchall() print(row)
bill = input("Has he paid all the bills? (y/n):")
if bill == "y":
mycursor.execute("delete from patient_details where name='" + name + "'")
mysql.commit()
Here we are completing the discharge process of the patient by confirming the bill payment in the hospital management system in python.
If the username and password are not present in the database the system will not perform any further operations.
Output

Complete python program of Hospital Management System with Source code
while (True): print(""" ================================ Welcome To CityHospital ================================ """) # creating database connectivity import mysql.connector passwd = str(input("Enter the Password Please!!:")) mysql = mysql.connector.connect( host="localhost", user="root", passwd=passwd) mycursor = mysql.cursor() mycursor.execute("create database if not exists city_hospitals") mycursor.execute("use city_hospitals") # creating the tables we need mycursor.execute( "create table if not exists patient_detail(name varchar(30) primary key,sex varchar(15),age int(3),address varchar(50),contact varchar(15))") mycursor.execute("create table if not exists doctor_details(name varchar(30) primary key,specialisation varchar(40),age int(2),address varchar(30),contact varchar(15),fees int(10),monthly_salary int(10))") mycursor.execute( "create table if not exists nurse_details(name varchar(30) primary key,age int(2),address varchar(30),contact varchar(15),monthly_salary int(10))") mycursor.execute( "create table if not exists other_workers_details(name varchar(30) primary key,age int(2),address varchar(30),contact varchar(15),monthly_salary int(10))") # creating table for storing the username and password of the user mycursor.execute( "create table if not exists user_data(username varchar(30) primary key,password varchar(30) default'000')") while (True): print(""" 1. Sign In 2. Registration """) r = int(input("enter your choice:")) if r == 2: print(""" ======================================= !!!!!!!!!!Register Yourself!!!!!!!! ======================================= """) u = input("Input your username!!:") p = input("Input the password (Password must be strong!!!:") mycursor.execute( "insert into user_data values('" + u + "','" + p + "')") mysql.commit() print(""" ============================================ !!Well Done!!Registration Done Successfully!! ============================================ """) x = input("enter any key to continue:") # IF USER WANTS TO LOGIN elif r == 1: print(""" ================================== !!!!!!!! {{Sign In}} !!!!!!!!!! ================================== """) un = input("Enter Username!!:") ps = input("Enter Password!!:") mycursor.execute( "select password from user_data where username='" + un + "'") row = mycursor.fetchall() for i in row: a = list(i) if a[0] == str(ps): while (True): print(""" 1.Administration 2.Patient(Details) 3.Sign Out """) a = int(input("ENTER YOUR CHOICE:")) if a == 1: print(""" 1. Display the details 2. Add a new member 3. Delete a member 4. Make an exit """) b = int(input("Enter your Choice:")) # details if b == 1: print(""" 1. Doctors Details 2. Nurse Details 3. Others """) c = int(input("Enter your Choice:")) if c == 1: mycursor.execute( "select * from doctor_details") row = mycursor.fetchall() for i in row: b = 0 v = list(i) k = ["NAME", "SPECIALISATION", "AGE", "ADDRESS", "CONTACT", "FEES", "MONTHLY_SALARY"] d = dict(zip(k, v)) print(d) # displays nurses details elif c == 2: mycursor.execute( "select * from nurse_details") row = mycursor.fetchall() for i in row: v = list(i) k = ["NAME", "SPECIALISATION", "AGE", "ADDRESS", "CONTACT", "MONTHLY_SALARY"] d = dict(zip(k, v)) print(d) # displays worker details elif c == 3: mycursor.execute( "select * from other_workers_details") row = mycursor.fetchall() for i in row: v = list(i) k = ["NAME", "SPECIALISATION", "AGE", "ADDRESS", "CONTACT""MONTHLY_SALARY"] d = dict(zip(k, v)) print(d) # IF USER WANTS TO ENTER DETAILS elif b == 2: print(""" 1. Doctor Details 2. Nurse Details 3. Others """) c = int(input("ENTER YOUR CHOICE:")) # enter doctor details if c == 1: # ASKING THE DETAILS name = input("Enter the doctor's name") spe = input("Enter the specilization:") age = input("Enter the age:") add = input("Enter the address:") cont = input("Enter Contact Details:") fees = input("Enter the fees:") ms = input("Enter Monthly Salary:") # Inserting values in doctors details mycursor.execute("insert into doctor_details values('" + name + "','" + spe + "','" + age + "','" + add + "','" + cont + "','" + fees + "','" + ms + "')") mysql.commit() print("SUCCESSFULLY ADDED") # for nurse details elif c == 2: # ASKING THE DETAILS name = input("Enter Nurse name:") age = input("Enter age:") add = input("Enter address:") cont = input("Enter Contact No:") ms = int(input("Enter Monthly Salary")) # INSERTING VALUES ENTERED TO THE TABLE mycursor.execute("insert into nurse_details values('" + name + "','" + age + "','" + add + "','" + cont + "','" + str( ms) + "')") mysql.commit() print("SUCCESSFULLY ADDED") # for entering workers details elif c == 3: # ASKING THE DETAILS name = input("Enter worker name:") age = input("Enter age:") add = input("Enter address:") cont = input("Enter Contact No.:") ms = input("Enter Monthly Salary:") # INSERTING VALUES ENTERED TO THE TABLE mycursor.execute("insert into other_workers_details values('" + name + "','" + age + "','" + add + "','" + cont + "','" + ms + "')") mysql.commit() print("SUCCESSFULLY ADDED") # to delete data elif b == 3: print(""" 1. Doctor Details 2. Nurse Details 3. Others """) c = int(input("Enter your Choice:")) # deleting doctor's details if c == 1: name = input("Enter Doctor's Name:") mycursor.execute( "select * from doctor_details where name='" + name + "'") row = mycursor.fetchall() print(row) p = input( "you really wanna delete this data? (y/n):") if p == "y": mycursor.execute( "delete from doctor_details where name='" + name + "'") mysql.commit() print("SUCCESSFULLY DELETED!!") else: print("NOT DELETED") # deleting nurse details elif c == 2: name = input("Enter Nurse Name:") mycursor.execute( "select * from nurse_details where name='" + name + "'") row = mycursor.fetchall() print(row) p = input( "you really wanna delete this data? (y/n):") if p == "y": mycursor.execute( "delete from nurse_details where name='" + name + "'") mysql.commit() print("SUCCESSFULLY DELETED!!") else: print("NOT DELETED") # deleting other_workers details elif c == 3: name = input("Enter the worker Name") mycursor.execute( "select * from workers_details where name='" + name + "'") row = mycursor.fetchall() print(row) p = input( "you really wanna delete this data? (y/n):") if p == "y": mycursor.execute( "delete from other_workers_details where name='" + name + "'") mysql.commit() print("SUCCESSFULLY DELETED!!") else: print("NOT DELETED") elif b == 4: break # entering the patient details table elif a == 2: print(""" 1. Show Patients Info 2. Add New Patient 3. Discharge Summary 4. Exit """) b = int(input("Enter your Choice:")) # showing the existing details # if user wants to see the details of PATIENT if b == 1: mycursor.execute( "select * from patient_detail") row = mycursor.fetchall() for i in row: b = 0 v = list(i) k = ["NAME", "SEX", "AGE", "ADDRESS", "CONTACT"] d = dict(zip(k, v)) print(d) # adding new patient elif b == 2: name = input("Enter your name ") sex = input("Enter the gender: ") age = input("Enter age: ") address = input("Enter address: ") contact = input("Contact Details: ") mycursor.execute("insert into patient_detail values('" + name + "','" + sex + "','" + age + "','" + address + "','" + contact + "')") mysql.commit() mycursor.execute( "select * from patient_detail") for i in mycursor: v = list(i) k = ['NAME', 'SEX', 'AGE', 'ADDRESS', 'CONTACT'] print(dict(zip(k, v))) print(""" ==================================== !!!!!!!Registered Successfully!!!!!! ==================================== """) # dischare process elif b == 3: name = input("Enter the Patient Name:") mycursor.execute( "select * from patient_detail where name='" + name + "'") row = mycursor.fetchall() print(row) bill = input( "Has he paid all the bills? (y/n):") if bill == "y": mycursor.execute( "delete from patient_detail where name='" + name + "'") mysql.commit() # if user wants to exit elif b == 4: break # SIGN OUT elif a == 3: break # IF THE USERNAME AND PASSWORD IS NOT IN THE DATABASE else: break
Summary
This article gives us an insight into how we can use our skills and ideas to develop a hospital management project in python programming language and use Mysql database connectivity where we can perform all the CRUD operations.
Inquiries
If you have any questions or suggestions about Hospital Management System Project in Python, please feel free to leave the comments below.
Also Read:
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Music Recommendation System in Machine Learning
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- 100+ Java Projects for Beginners 2023
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Courier Tracking System in HTML CSS and JS
- Test Typing Speed using Python App