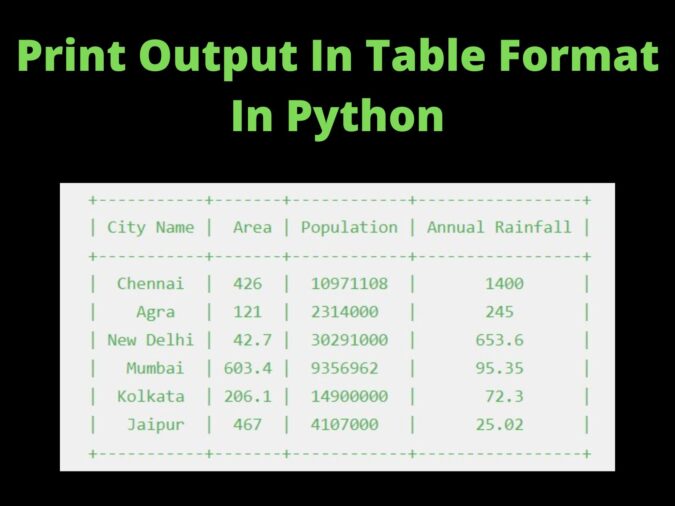
In this article, we will look at how to Print Output In Table Format In Python. We will see all the methods that are used for creating and deleting rows and columns with examples and will go deep into all the methods for better understanding.
There are 4 libraries that are used to Print Output In Table Format In Python:-
- Tabulate Library
- PrettyTable Library
- Texttable Library
- Termtables Library
Tabulate Library in Python
We can use this library to print tabular data in python and to do so we use the tabulate class inside the tabulate library. It is a command line utility.
Installation
pip install tabulate
Usage
# Importing tabulate class from tabulate library
from tabulate import tabulate
# Creating a list that we want to tabulate
my_list = [
["Python", "Guido van Rossum", 1991],
["C", "Dennis Ritchie", 1972],
["Java", "James Gosling", 1995],
["JavaScript", "Brendan Eich", 1995],
["Php", "Rasmus Lerdorf", 1995],
["C++", "Bjarne Stroustrup", 1985],
["C#", " Anders Hejlsberg", 2000]
]
# Printing the tabulated list
print(tabulate(my_list))
Output:
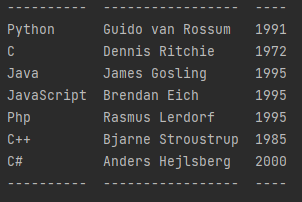
Adding Headers to Table
# Printing the tabulated list
print(tabulate(my_list, headers=["Programming Languages", "Founder", "Release Year"]))
Output:

Show the Index of each row
# Printing the tabulated list
print(tabulate(my_list, headers=["S.on", "Programming Languages", "Founder", "Release Year"], showindex="always"))
Output:
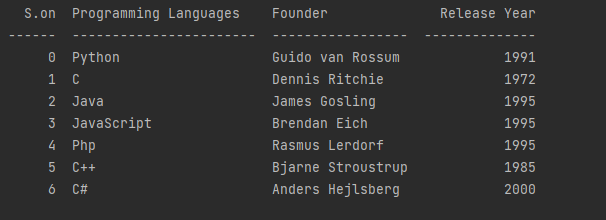
Change Alignment of Values
# Printing the tabulated list
print(tabulate(my_list, headers=["Programming Languages", "Founder", "Release Year"], stralign="right", numalign="right"))
Output:

PrettyTable Library in Python
We have already discussed this library in detail, so click here if you want to know in detail about this library. Let’s have an example of PrettyTable library usage for printing output in Table format.
# Importing PrettyTable class from prettytable library
from prettytable import PrettyTable
# Creating instance of PrettyTable class
my_table = PrettyTable(["Programming Languages", "Founder", "Release Year"])
# Creating a list that we want to tabulate
my_table.add_row(["Python", "Guido van Rossum", 1991])
my_table.add_row(["C", "Dennis Ritchie", 1972])
my_table.add_row(["Java", "James Gosling", 1995])
my_table.add_row(["JavaScript", "Brendan Eich", 1995])
my_table.add_row(["Php", "Rasmus Lerdorf", 1995])
my_table.add_row(["C++", "Bjarne Stroustrup", 1985])
my_table.add_row(["C#", " Anders Hejlsberg", 2000])
# Printing the tabulated list
print(my_table)
Output:

Texttable Library in Python
We use this library to print ASCII Table ( Tabular Form) in python and to do so we use the Texttable class from the Textable library.
Installation
pip install texttable
Usage
# Importing Texttable class from texttable Library
from texttable import Texttable
# Creating an instance of Texttable class
my_table = Texttable()
# Adding rows to our tabular table
my_table.add_rows(
[["Programming Languages", "Founder", "Release Year"],
["Python", "Guido van Rossum", 1991],
["C", "Dennis Ritchie", 1972],
["Java", "James Gosling", 1995],
["JavaScript", "Brendan Eich", 1995],
["Php", "Rasmus Lerdorf", 1995],
["C++", "Bjarne Stroustrup", 1985],
["C#", " Anders Hejlsberg", 2000]]
)
# Printing Tabulated Data Using draw() function of Texttable Class
print(my_table.draw())
Output:
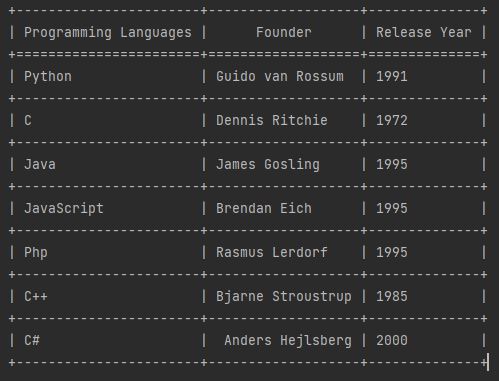
Change Alignment of Values
To change the alignment of our table data in Texttable class, we have to use one String character array as an input parameter of set_cols_align() which is an in-built function of Texttable class. The one String character aligns our data for the corresponding column. Valid string characters for alignment:-
- “l”– side alignment.
- “r”– right side alignment.
- “c”– center alignment.
- “t”– top side alignment.
- “m”– middle alignment.
- “b”– bottom side alignment.
Add the below statement before adding rows to our tabular table in the code
my_table.set_cols_align(["l", "r", "l"])
Output:
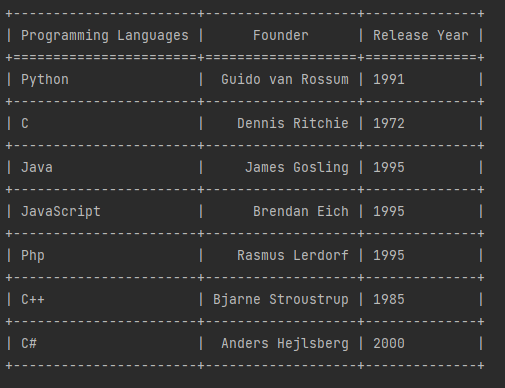
Termtables Library in Python
Installation
pip install termtables
Usage
# Importing Termtables class
import termtables
# Adding data in my_table list
my_table = [
["Python", "Guido van Rossum", 1991],
["C", "Dennis Ritchie", 1972],
["Java", "James Gosling", 1995],
["JavaScript", "Brendan Eich", 1995],
["Php", "Rasmus Lerdorf", 1995],
["C++", "Bjarne Stroustrup", 1985],
["C#", " Anders Hejlsberg", 2000]
]
# Printing tubulated data using termtables
termtables.print(my_table)
Output:

Adding Headers to Table
# Creating a header list for column headers
headers = ["Programming Languages", "Founder", "Release Year"]
# Printing tubulated data using termtables
tt.print(my_table, header = headers)
Output:

Changing Alignment and adding Padding Tables
# Printing tubulated data using termtables
tt.print(my_table, header=headers, padding=(0, 1), alignment="lcr")
Output:
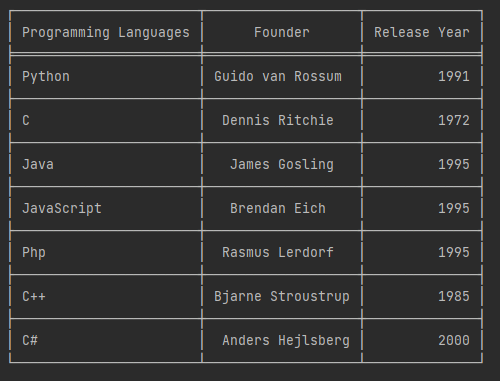
We hope this article on Tabular Data in Python helps you.
Thank you for reading this article, click here to start learning Python in 2022.
Also Read:
- What is web development for beginners?
- What does if __name__ == __main__ do in Python?
- Python | CRUD operations in MongoDB
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App