
Introduction
This is a tutorial for a simple atm program in python. Note that this is an ATM simulation for a single user, let’s say, Ms. ABC, who has already successfully logged into her account on the ATM Machine, now, we will program the next steps she may want to perform.
ATM Transaction Options
1. Just after logging
Here on, she is presented with the following options:

Let’s see what pressing the buttons for all these options does, as well as pressing an invalid button:

2. Pressing 0 in Simple Atm Program in Python
Pressing 0 will simply “log us out” of the ATM.
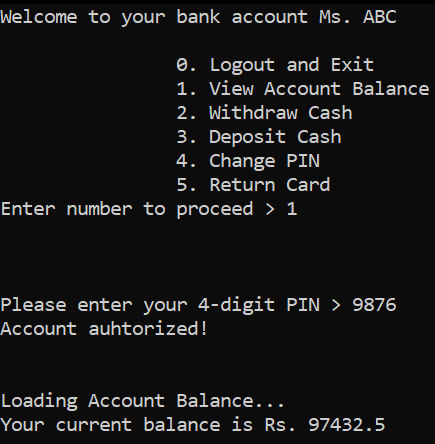
3. Pressing 1
Pressing 1 takes us to the “View Account Balance” option, where, after we’ve entered the correct PIN for our bank account, we are able to see how much money is in our bank account.

4. Pressing 2 in Simple Atm Program in Python
Pressing 2 will load up the cash withdrawal screen, wherein after we have entered the right PIN for our bank account, we will be able to enter an amount to withdraw from our account. Here, in the above screenshot, I have entered an extra 0 in 10000 by mistake, which is where the code tells me that I won’t be able to withdraw an amount that is greater than my account balance and is giving me an error message.
Immediately after that, as seen in the next screenshot, I am being prompted to enter the amount again, where I have put in 10000, and after getting a confirmation from me, it deducts the amount entered, that is 10 thousand rupees in this example, and shows us the updated balance of money in our account.

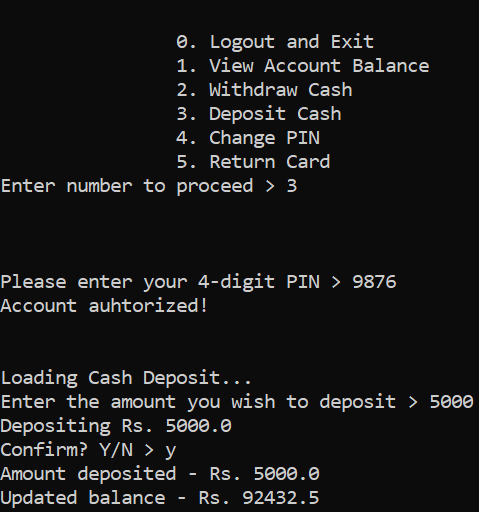
5. Pressing 3
On entering 3, we are taken to the cash deposit option of the ATM, where we’ll be able to deposit an amount into our bank account. Here, I have entered 5000 as the deposit amount, which is then immediately added to the bank balance after I validate that 5000 is indeed the amount I wish to deposit into my account.

6. Pressing 4 in Simple Atm Program in Python
Pressing 4 allows me to change my ATM PIN. Here, I am prompted to enter my old PIN, after which the program allows me to enter the new PIN I want, which I have chosen at 2912.

So after the PIN update, I will not be able to use my old PIN for any processes or transactions as long as my program is running this time.
Further, I will only be able to enter an incorrect PIN a total of 3 times, after which I will be logged out of the account and will be exited from the code execution.
Also, if I enter n or N after the “Confirm? Y/N”, the entire process will be canceled and no changes will be made to the PIN or account balance.


7. Pressing 5
The last option, 5, is for returning the ATM card, as seen above.

Inputting a number that is not in the range of 0 to 6 will give me an “Invalid Input!” message.
Code explanation for Simple Atm Program in Python
Now that we have seen and understood how the entire program works, let’s take a look at the code for each of the above options, as well as for the entry and exit messages –
import time as t
I am using the time module with the alias t for its sleep function. I have used the sleep function simply for the realistic effect it gives to an application, and will explain its implementation as we encounter it further along in the code.
Here, I have hard-coded the user’s PIN and account balance as so:
user_pin = 9876
user_balance = 97432.50
user_name = "Ms. ABC"
print("Welcome to your bank account", user_name, end = "\n\n")
choice = 9
I have also initialized the value of the choice variable.
while (True):
print("\t\t0. Logout and Exit")
print("\t\t1. View Account Balance")
print("\t\t2. Withdraw Cash")
print("\t\t3. Deposit Cash")
print("\t\t4. Change PIN")
print("\t\t5. Return Card")
choice = int(input("Enter number to proceed > "))
print("\n\n")
if choice == 0:
print("Exiting...")
t.sleep(2)
print("You have been logged out. Thank you!\n\n")
break
Now here, after Exiting… is printed, I have added t.sleep(2), where 2 is indicative is the number of seconds the program execution is supposed to wait before going forward with the code.
elif choice in (1,2,3,4,5):
num_of_tries = 3
while (num_of_tries!=0):
input_pin = int(input("Please enter your 4-digit PIN > "))
if input_pin == user_pin:
print("Account auhtorized!\n\n")
if choice == 1:
print("Loading Account Balance...")
t.sleep(1.5)
print("Your current balance is Rs.", user_balance, end = "\n\n\n")
break
elif choice == 2:
print("Opening Cash Withdrawal...")
t.sleep(1.5)
while(True):
withdraw_amt = float(input("Enter the amount you wish to withdraw > "))
if withdraw_amt>user_balance:
print("Can't withdraw Rs.", withdraw_amt)
print("Please enter a lower amount!")
continue
else:
print("Withdrawing Rs.", withdraw_amt)
confirm = input("Confirm? Y/N > ")
if confirm in ('Y', 'y'):
user_balance-=withdraw_amt
print("Amount withdrawn - Rs.", withdraw_amt)
print("Remaining balance - Rs.", user_balance, end = "\n\n\n")
break
else:
print("Cancelling transaction...")
t.sleep(1)
print("Transaction Cancelled!\n\n")
break
break
elif choice == 3:
print("Loading Cash Deposit...")
t.sleep(1.5)
deposit_amt = float(input("Enter the amount you wish to deposit > "))
print("Depositing Rs.", deposit_amt)
confirm = input("Confirm? Y/N > ")
if confirm in ('Y', 'y'):
user_balance+=deposit_amt
print("Amount deposited - Rs.", deposit_amt)
print("Updated balance - Rs.", user_balance, end = "\n\n\n")
else:
print("Cancelling transaction...")
t.sleep(1)
print("Transaction Cancelled!\n\n")
break
elif choice == 4:
print("Loading PIN Change...")
t.sleep(1.5)
pin_new = int(input("Enter your new PIN > "))
print("Changing PIN to", pin_new)
confirm = input("Confirm? Y/N > ")
if confirm in ('Y', 'y'):
user_pin = pin_new
print("PIN changed successfully! \n\n")
else:
print("Cancelling PIN change...")
t.sleep(1)
print("Process Cancelled!\n\n")
break
else:
print("Loading Card Return...")
t.sleep(1.5)
print("Returning your ATM Card")
confirm = input("Confirm? Y/N > ")
if confirm in ('Y', 'y'):
print("Card returned successfully! \n\n")
else:
print("Cancelling process...")
t.sleep(1)
print("Process Cancelled!\n\n")
break
else:
num_of_tries-=1
print("PIN incorrect! Number of tries left -", num_of_tries, end = "\n\n")
else:
print("Exiting...")
t.sleep(2)
print("You have been logged out. Thank you!\n\n")
break
else:
print("Invalid input!")
print("\t\t0. Enter 0 to Logout and Exit!")
continue
You can find the GitHub repository with the entire code here.
We hope this article on the Simple Atm Program in Python helps you.
Keep Learning, Keep Coding
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter