
Hello everyone, in this article we are going to create Snake Game in C++. This will be a simple console-based application in which the player can play the snake game like we usually play in our mobile application.
Features of snake game in C++
- The player will be able to move the snake in four directions by pressing the up, down, left, and right keys
- The snake has to eat the food to get a score and it grows as it keeps on eating the food.
- The snake must not hit the wall or its own tail, if it does then the snake dies, and the game is over.
- After the game is over, the player can hit enter again to replay.
- On pressing the space bar key, the game can be paused.
- The current score count is updated as the snake eats the food
Complete Code for Snake Game in C++
We will use Code::Blocks IDE to develop this application. Open the Code::Blocks IDE and create a new project with the name snakegame, now in the main.cpp file paste the following code and run it.
#include<stdio.h> #include<conio.h> #include<ctype.h> #include<stdlib.h> #include<windows.h> #include<time.h> #include<string.h> #define LEFT 1 #define RIGHT 2 #define UP 3 #define DOWN 4 //This method is used to provide a particular color to elements void textcolor(int fc,int bc=-1){ if(fc<0 || fc>15) return; HANDLE h; h = GetStdHandle(STD_OUTPUT_HANDLE); if(bc>=0 && bc<16) SetConsoleTextAttribute(h,fc|bc*16); else SetConsoleTextAttribute(h,fc); } //This method is used to provide a particular color to elements void textcolor(char *fc,char *bc=""){ int x,y=16; char *colors[]={"Black","Blue","Green","Aqua","Red","Purple","Yellow","White","Gray", "LightBlue","LightGreen","LightAqua","LightRed","LightPurple","LightYellow","BrightWhite"}; for(x=0;x<16;x++) if(strcmpi(colors[x],fc)==0) break; if(strlen(bc)>0) for(y=0;y<16;y++) if(strcmpi(colors[y],bc)==0) break; textcolor(x,y); } //This method is used to provide a particular color to elements void textcolor(char *fc,int bc){ int x; char *colors[]={"Black","Blue","Green","Aqua","Red","Purple","Yellow","White","Gray", "LightBlue","LightGreen","LightAqua","LightRed","LightPurple","LightYellow","BrightWhite"}; for(x=0;x<16;x++) if(strcmpi(colors[x],fc)==0) break; textcolor(x,bc); } //This method is used to provide a particular color to elements void textcolor(int fc,char *bc){ int y; char *colors[]={"Black","Blue","Green","Aqua","Red","Purple","Yellow","White","Gray", "LightBlue","LightGreen","LightAqua","LightRed","LightPurple","LightYellow","BrightWhite"}; if(strlen(bc)>0) for(y=0;y<16;y++) if(strcmpi(colors[y],bc)==0) break; textcolor(fc,y); } //This method is used to set the position to a particular coordinate void gotoxy(int x, int y) { COORD coord; coord.X = x; coord.Y = y; SetConsoleCursorPosition(GetStdHandle(STD_OUTPUT_HANDLE), coord); } //This method is used to draw the texts on the screen void getup(){ HANDLE hout; CONSOLE_CURSOR_INFO cursor; hout = GetStdHandle(STD_OUTPUT_HANDLE); cursor.dwSize=1; cursor.bVisible=false; SetConsoleCursorInfo(hout, &cursor); system("mode con:cols=80 lines=25"); system("title Snake Game"); system("cls"); textcolor("Yellow"); printf("\n %c",218); int x; for(x=0;x<75;x++) printf("%c",196); printf("%c ",191); for(x=0;x<17;x++){ gotoxy(2,x+2); printf("%c",179); gotoxy(78,x+2); printf("%c ",179); } printf(" %c",192); for(x=0;x<75;x++) printf("%c",196); printf("%c ",217); printf(" %c",218); for(x=0;x<21;x++) printf("%c",196); printf("%c\n",191); printf(" %cPress Enter to Replay%c\n",179,179); printf(" %c",192); for(x=0;x<21;x++) printf("%c",196); printf("%c",217); gotoxy(59,20); printf("%c",218); for(x=0;x<18;x++) printf("%c",196); printf("%c",191); gotoxy(59,21); printf("%c SCORE : 100 %c",179,179); gotoxy(59,22); printf("%c STATUS: Playing %c",179,179); gotoxy(59,23); printf("%c",192); for(x=0;x<18;x++) printf("%c",196); printf("%c",217); gotoxy(32,21); printf("Eat the food #"); gotoxy(32,22); printf("Press 'space' to Pause"); textcolor(7); } //This method is used to update the score variable on the right side down screen void score(int sc){ gotoxy(69,21); printf("%6d",sc*10); } //This method is used to update the status variable which is initially set to playing on the right side down screen void status(char *s,int c=7){ gotoxy(69,22); textcolor(c); int x; for(x=0;x<strlen(s);x++) printf("%c",s[x]); for(;x<8;x++) printf(" "); textcolor(7); } // This is the main method, the program starts from here int main(){ getup(); register int flow,size,i,xb,yb; int speed,restart=1,tmp,xpos[100],ypos[100],scr; srand(time(NULL)); while(true){ if(restart){ status("Playing",10); for(int k=1;k<75;k+=2) for(int j=0;j<17;j++){ gotoxy(k+3,j+2); printf(" "); } size=5; speed=200; scr=0; score(scr); flow=RIGHT; xpos[0]=20; for(i=0;i<size;i++){ xpos[i]=xpos[0]-i*2; ypos[i]=10; } for(i=0;i<size;i++){ gotoxy(xpos[i],ypos[i]); printf("*"); } for(tmp=1;true;){ do{ xb=rand()%75+3; }while(xb%2!=0); yb=rand()%17+2; for(i=0;i<size;i++) if(xb==xpos[i] && yb==ypos[i]){ tmp=0; break; } if(tmp) break; } gotoxy(xb,yb); textcolor("lightred"); printf("#"); textcolor(7); restart=0; } while(!kbhit() && !restart) { if(xpos[0]==xb && ypos[0]==yb){ for(tmp=1;true;){ do{ xb=rand()%75+3; }while(xb%2!=0); yb=rand()%17+2; for(i=0;i<size;i++) if(xb==xpos[i] && yb==ypos[i]){ tmp=0; break; } if(tmp) break; } gotoxy(xb,yb); textcolor("lightred"); printf("#"); textcolor(7); size++; scr++; speed-=3; score(scr); } gotoxy(xpos[size-1],ypos[size-1]); for(i=size-1;i>0;i--){ xpos[i]=xpos[i-1]; ypos[i]=ypos[i-1]; } switch(flow){ case RIGHT :xpos[i]+=2; break; case LEFT : xpos[i]-=2; break; case UP : ypos[i]-=1; break; case DOWN : ypos[i]+=1; } tmp=1; for(i=1;i<size;i++) if(xpos[i]==xpos[0] && ypos[i]==ypos[0]){ tmp=0; break; } if(xpos[0]>76 || xpos[0]<4 || ypos[0]<2 ||ypos[0]>18) tmp=0; if(tmp){ printf(" "); gotoxy(xpos[0],ypos[0]); printf("O"); gotoxy(xpos[1],ypos[1]); printf("*"); } else{ textcolor("LIGHTRED"); printf("*"); gotoxy(xpos[1],ypos[1]); printf("O"); for(i=2;i<size;i++){ gotoxy(xpos[i],ypos[i]); printf("*"); } textcolor(7); status("GameOver",12); restart=1; getch(); } //delay(speed); Sleep(speed); } char ch=getch(); switch(tolower(ch)){ case 'x' : system("cls"); return 0; case ' ' : status("Paused"); while(true){ char z=getch(); if(z=='x') return 0; if(z==' ') break; } status("Playing",10); break; case -32: { char chh=getch(); if(chh==72 && flow!=DOWN) flow=UP; else if(chh==80 && flow!=UP) flow=DOWN; else if(chh==75 && flow!=RIGHT) flow=LEFT; else if(chh==77 && flow!=LEFT) flow=RIGHT; break; } } } }
Output for Snake Game in C++
Image Output:
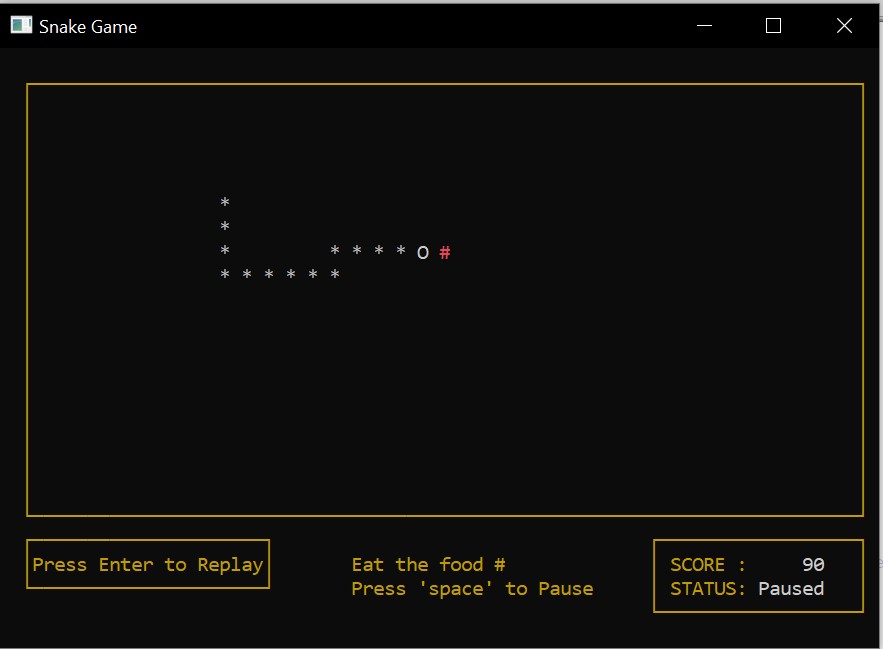
Video Output:
Conclusion
We have successfully developed a simple Snake Game in C++. As it is a console application this is easy to understand and work with. I hope this was helpful, you should try to change the code here and there to understand how the program is working.
Visit the official C++ website.
Thank you for visiting our website.
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector