
This blog will discuss how we can build a Vending Machine with Python Code (console app). The Vending Machine with Python code is a small project that doesn’t contain advanced topics and will help you clear some of the basics. You will be able to learn the following concepts about Python from this blog:
- Dictionary data type Operations: https://copyassignment.com/python/python-dictionary/
- String data type Operations: https://copyassignment.com/python/python-strings/
- Bool with While Loop: https://copyassignment.com/python/python-while-loop/ and https://docs.python.org/3/library/stdtypes.html#boolean-operations-and-or-not
The Concept behind Vending Machine with Python:
There will be a dictionary of all the items with the properties product_id, product_name, and product_price. An empty list of the_item where all the selected products will be added later. A “run” variable is True until the user is satisfied and does not want to buy any more products and the value will become False and won’t loop through.
Now, we will understand the code for Vending Machine with Python Code.
Data for Vending Machine

Printing Menu
A simple loop that will print the vending machine’s menu with all the product’s necessary properties is written.

Calculating Total Price
A function sum() is written, which will loop through the_item list containing all the selected products to buy. First, it will loop through the list and add the property of product_price into the total sum, and the function will return the total sum.

Vending Machine Logic
A function machine() is written in the vending machine with a Python program, which is the program’s primary function. This function will take 3 arguments: the items_in_stock dictionary, the run variable with a boolean value, and the_item list will contain all the intended items by the user. A while loop that will only work when the value of the run variable is True is written.
Here, the user is asked to enter the product id of the intended item, and if the product id is less than the total length of the items_in_stock dictionary, then the whole id properties are added to the list the_item, or else an error will be printed, “THE PRODUCT ID IS WRONG.” The user is asked if they want to add more items; if not, the run variable will become False. There will be a prompt on whether to print the whole receipt or only the total sum.
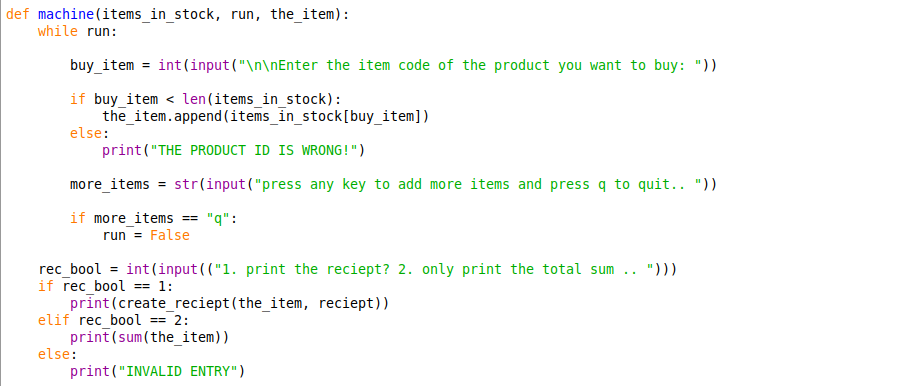
Function for Creating Receipt
Another feature of Vending Machine with Python is that it will create a receipt display on the console. The function create_reciept() will take two arguments, the_item list of selected products and receipt, which is a string of boilerplate menus. It will loop through the_item list, select the product name and price, and print accordingly. Then, at last, this function will again use the previous sum() function to print the total price. Note that the sum() function was previously written outside of this create_reciept() function.
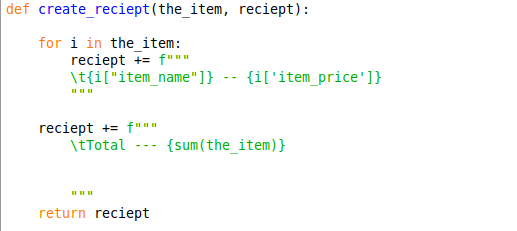
Output

Complete code for Vending Machine with Python
items_in_stock = [ { "item_id": 0, "item_name": "Milky Bar", 'item_price': 60, }, { "item_id": 1, "item_name": "Fanta", 'item_price': 90, }, { "item_id": 2, "item_name": "Kurkure", 'item_price': 25, }, { "item_id": 3, "item_name": "Thumbs Up", 'item_price': 90, }, { "item_id": 4, "item_name": "Wai-Wai", 'item_price': 20, }, ] the_item = [] reciept = """ \t\tPRODUCT -- PRICE """ sum = 0 run = True print("------- Vending Machine with Python-------\n\n") print("----------The Items In Stock Are----------\n\n") for i in items_in_stock: print(f"Item: {i['item_name']} --- Price: {i['item_price']} --- Item ID: {i['item_id']}") def machine(items_in_stock, run, the_item): while run: buy_item = int(input("\n\nEnter the item code of the product you want to buy: ")) if buy_item < len(items_in_stock): the_item.append(items_in_stock[buy_item]) else: print("THE PRODUCT ID IS WRONG!") more_items = str(input("press any key to add more items and press q to quit.. ")) if more_items == "q": run = False rec_bool = int(input(("1. print the reciept? 2. only print the total sum .. "))) if rec_bool == 1: print(create_reciept(the_item, reciept)) elif rec_bool == 2: print(sum(the_item)) else: print("INVALID ENTRY") def sum(the_item): sum = 0 for i in the_item: sum += i["item_price"] return sum def create_reciept(the_item, reciept): for i in the_item: reciept += f""" \t{i["item_name"]} -- {i['item_price']} """ reciept += f""" \tTotal --- {sum(the_item)} """ return reciept if __name__ == "__main__": machine(items_in_stock, run, the_item)
GitHub Link: https://github.com/copyassignment/small_projects/blob/main/vending_machine.py
Thanks for reading this blog until the end. To learn more about basic scripting with Python, you can read other blogs on this website with the category: https://copyassignment.com/category/python-projects/.
We hope this article will help you to learn to create Vending Machine with Python Code.
Also Read:
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Music Recommendation System in Machine Learning
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- 100+ Java Projects for Beginners 2023
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Courier Tracking System in HTML CSS and JS
- Test Typing Speed using Python App