
Introduction
In this article, we’ll look at how to draw an Android Logo using Python Turtle module. Install the turtle python package to create the Android logo. We can create any type of graph using the turtle module. See the complete code for the Android logo below.
Let’s Code!
Step 1. First of all import the libraries to draw the logo
import turtle as t
Turtle is a python library, it is used to draw shapes.
Step 2. Setup the Background and Pen Location
t.bgcolor("gray")
t.penup()
t.goto(-80,80)
t.pendown()
t.pencolor("#3DDC84")
here penup() function will lift the turtle off the digital canvas, the goto function is used to move the cursor to the desired location, and pendown() is mostly useful to reestablish pendown state after using .penup(). Pencolor() function is used to set the pen color
Step 3. Creating a function to draw circles
def circle():
t.begin_fill()
t.fillcolor('white')
t.circle(7)
t.end_fill()
Creating a circle() is used to draw the circles for the eyes. begin_fill() function is used to fill the color in particular shapes. and to choose and fill the color we use fill_color().
Step 4. Drawing the Upper Body of Android Logo.
def draw_upperbody():
t.begin_fill()
t.fillcolor("#3DDC84")
t.forward(150)
t.left(90)
for i in range(238):
t.left(0.76)
t.forward(1)
t.end_fill()
#drawing eyes
#left Eye
t.penup()
t.goto(-46,120)
t.pendown()
circle()
#Right Eye
t.penup()
t.goto(24,120)
t.pendown()
circle()
#Drawing ears
#left ear
t.penup()
t.goto(-40,140)
t.pendown()
t.pensize(4)
t.right(140)
t.forward(50)
#Right ear
t.penup()
t.goto(34,144)
t.pendown()
t.pensize(4)
t.right(80)
t.forward(46)
This function is responsible for creating an android body. in this function, the code is pretty easy to understand. we put one for a loop. And use some by default functions to draw the body
Step 5. Draw the Middle body of the Android logo.
def draw_middlebody():
t.begin_fill()
t.fillcolor("#3DDC84")
t.pensize(1)
t.right(141)
t.forward(100)
for i in range(20):
t.forward(1)
t.left(5)
t.right(9.5)
t.forward(127)
for i in range(20):
t.forward(1)
t.left(5)
t.right(9.5)
t.forward(100)
t.end_fill()
To draw the middle body, We use two for loops first forward(127) right(9.5) and in the second loop we take forward(100) right(9.5) then fill the color “end_fill”.
Step 6. Drawing the hands of Android Logo.
#hand
def draw_hand():
t.begin_fill()
t.fillcolor("#3DDC84")
for i in range(45):
t.right(4)
t.forward(1)
t.forward(70)
for i in range(45):
t.right(4)
t.forward(1)
t.forward(70)
t.end_fill()
This function is used to construct both the left and right hands of the Android logo.
Step 7. Draw the lower body of the Android.
#legs
def draw_legs():
t.begin_fill()
t.fillcolor("#3DDC84")
t.right(91)
t.forward(30)
t.right(90)
t.forward(50)
for i in range(45):
t.right(4)
t.forward(1)
t.end_fill()
This code is responsible for creating both the left and right legs of the Android Logo.
Complete code to draw Android Logo using Python Turtle
import turtle as t t.bgcolor("white") t.penup() t.goto(-80,80) t.pendown() t.speed(8) t.pencolor("#3DDC84") def circle(): t.begin_fill() t.fillcolor('white') t.circle(7) t.end_fill() #Drawing the Head def draw_upperbody(): t.begin_fill() t.fillcolor("#3DDC84") t.forward(150) t.left(90) for i in range(238): t.left(0.76) t.forward(1) t.end_fill() #drawing eyes #left Eye t.penup() t.goto(-46,120) t.pendown() circle() #Right Eye t.penup() t.goto(24,120) t.pendown() circle() #Drawing ears #left ear t.penup() t.goto(-40,140) t.pendown() t.pensize(4) t.right(140) t.forward(50) #Right ear t.penup() t.goto(34,144) t.pendown() t.pensize(4) t.right(80) t.forward(46) #Drawing the middle body def draw_middlebody(): t.begin_fill() t.fillcolor("#3DDC84") t.pensize(1) t.right(141) t.forward(100) for i in range(20): t.forward(1) t.left(5) t.right(9.5) t.forward(127) for i in range(20): t.forward(1) t.left(5) t.right(9.5) t.forward(100) t.end_fill() #hand def draw_hand(): t.begin_fill() t.fillcolor("#3DDC84") for i in range(45): t.right(4) t.forward(1) t.forward(70) for i in range(45): t.right(4) t.forward(1) t.forward(70) t.end_fill() #legs def draw_legs(): t.begin_fill() t.fillcolor("#3DDC84") t.right(91) t.forward(30) t.right(90) t.forward(50) for i in range(45): t.right(4) t.forward(1) t.end_fill() draw_upperbody() t.penup() t.goto(-80,68) t.pendown() draw_middlebody() t.penup() t.goto(80,68) t.pendown() draw_hand() t.penup() t.goto(-124,70) t.pendown() draw_hand() t.penup() t.goto(-50,-50) t.pendown() draw_legs() t.penup() t.goto(14,-50) t.pendown() t.left(1.7) draw_legs() t.hideturtle() t.done()
Output:
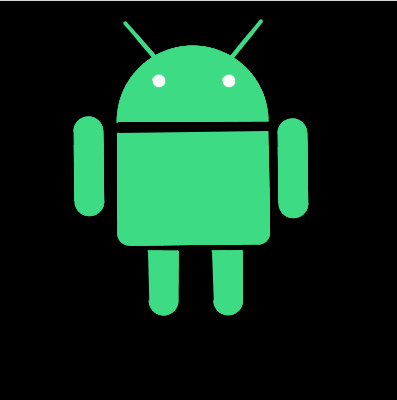
Thank you for reading the article. Visit our home page for more articles like this.
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector