
Introduction
We are all familiar with the stopwatch. And, it is possible to create a Stopwatch Using Python. According to our information, the DateTime module is already available in Python. We can use that module to create a timer in Python. We are hoping that this content will be fantastic. You can use this as a primary-level Python mini-project. Click here for the complete source code. Let’s get started.
Implementation for Stopwatch Using Python in Tkinter
Step1. Importing libraries and initializing variables
from tkinter import *
import sys
import time
global count
count =0
To make a timer in Python, we must first import the Tkinter package. To obtain a time, import the DateTime module. Declaring the count as global and setting it to zero.
Step 2. Stopwatch Class
class stopwatch():
def reset(self):
global count
count=1
self.t.set('00:00:00')
In Python code, create a stopwatch class. Create a function to conduct a reset action after creating a class. This function resets the timing to 00:00:00, akin to restarting the stopwatch.
Step 3. Start method for Stopwatch Using Python
def start(self):
global count
count=0
self.timer()
To start the stopwatch, write a function called start. This feature is useful for starting the stopwatch.
Step 4. Stop method
def stop(self):
global count
count=1
To stop the stopwatch, we need a stop function. To conduct the stop operation in the stopwatch, we must write another function called to stop.
Step 5. Close method
def close(self):
self.root.destroy()
When creating applications, always include an exit button. That will make an excellent first impression on your application. To conduct the exit procedure, we must develop a function called close. When we click the exit button, it will exit the application.
Step 6. Create the timer method
def timer(self):
global count
if(count==0):
self.d = str(self.t.get())
h,m,s = map(int,self.d.split(":"))
h = int(h)
m=int(m)
s= int(s)
if(s<59):
s+=1
elif(s==59):
s=0
if(m<59):
m+=1
elif(m==59):
m=0
h+=1
if(h<10):
h = str(0)+str(h)
else:
h= str(h)
if(m<10):
m = str(0)+str(m)
else:
m = str(m)
if(s<10):
s=str(0)+str(s)
else:
s=str(s)
self.d=h+":"+m+":"+s
self.t.set(self.d)
if(count==0):
self.root.after(1000,self.timer)
Create a timer function to run the stopwatch. I hope you saw that I named a functioning self.timer(). So, when the program sees that sentence, it will call this function and do the task specified in the timer function.
Step 7. Creating the init method for Stopwatch Using Python
def __init__(self):
self.root=Tk()
self.root.title("Stop Watch")
self.root.geometry("600x200")
self.t = StringVar()
self.t.set("00:00:00")
self.lb = Label(self.root,textvariable=self.t,font=("Times 40 bold"),bg="white")
self.bt1 = Button(self.root,text="Start",command=self.start,font=("Times 12 bold"),bg=("#F88379"))
self.bt2 = Button(self.root,text="Stop",command=self.stop,font=("Times 12 bold"),bg=("#F88379"))
self.bt3 = Button(self.root,text="Reset",command=self.reset,font=("Times 12 bold"),bg=("#DE1738"))
self.bt4 = Button(self.root, text="Exit", command=self.close,font=("Times 12 bold"),bg=("#DE1738"))
self.lb.place(x=160,y=10)
self.bt1.place(x=120,y=100)
self.bt2.place(x=220,y=100)
self.bt3.place(x=320,y=100)
self.bt4.place(x=420,y=100)
self.label = Label(self.root,text="",font=("Times 40 bold"))
self.root.configure(bg='white')
self.root.mainloop()
a=stopwatch()
Following that, we add an init function in this program, as well as a button for each operation. Furthermore, this function will invoke all of the functions. To improve our timer, we’re changing the backdrop colors of the letters.
Complete source code for Stopwatch using Python Tkinter
from tkinter import * import sys import time global count count =0 class stopwatch(): def reset(self): global count count=1 self.t.set('00:00:00') def start(self): global count count=0 self.timer() def stop(self): global count count=1 def close(self): self.root.destroy() def timer(self): global count if(count==0): self.d = str(self.t.get()) h,m,s = map(int,self.d.split(":")) h = int(h) m=int(m) s= int(s) if(s<59): s+=1 elif(s==59): s=0 if(m<59): m+=1 elif(m==59): m=0 h+=1 if(h<10): h = str(0)+str(h) else: h= str(h) if(m<10): m = str(0)+str(m) else: m = str(m) if(s<10): s=str(0)+str(s) else: s=str(s) self.d=h+":"+m+":"+s self.t.set(self.d) if(count==0): self.root.after(1000,self.timer) def __init__(self): self.root=Tk() self.root.title("Stop Watch") self.root.geometry("600x200") self.t = StringVar() self.t.set("00:00:00") self.lb = Label(self.root,textvariable=self.t,font=("Times 40 bold"),bg="white") self.bt1 = Button(self.root,text="Start",command=self.start,font=("Times 12 bold"),bg=("#F88379")) self.bt2 = Button(self.root,text="Stop",command=self.stop,font=("Times 12 bold"),bg=("#F88379")) self.bt3 = Button(self.root,text="Reset",command=self.reset,font=("Times 12 bold"),bg=("#DE1738")) self.bt4 = Button(self.root, text="Exit", command=self.close,font=("Times 12 bold"),bg=("#DE1738")) self.lb.place(x=160,y=10) self.bt1.place(x=120,y=100) self.bt2.place(x=220,y=100) self.bt3.place(x=320,y=100) self.bt4.place(x=420,y=100) self.label = Label(self.root,text="",font=("Times 40 bold")) self.root.configure(bg='white') self.root.mainloop() a=stopwatch()
Output:
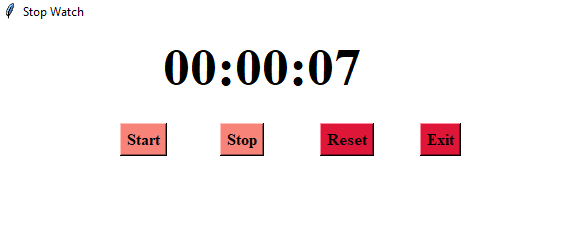
In this tutorial, we learned how to create a Stopwatch Using Python Tkinter. This article will be useful for all Python beginners. Learn it completely. Try to solve the code on your own. If you have any questions, please leave them in the comment section.
Also Read:
- Create your own ChatGPT with Python
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Test Typing Speed using Python App
- Scientific Calculator in Python
- GUI To-Do List App in Python Tkinter
- Scientific Calculator in Python using Tkinter
- GUI Chat Application in Python Tkinter