
Introduction
Today, we are going to create a Pong Game using Python Turtle. Pong is a well-known computer game that is similar to table tennis. The two players in this game control the two paddles on either side of the game window. To hit the moving ball, they move the paddles up and down. A player’s score rises when he or she hits the ball or when the opponent misses the hit.
To create this Python game, we will use the turtle module. To move the left and right paddles in this game, we will use the up, down, W, and S keys. As a player strikes the ball to a predefined speed level, the speed of the ball rises, as does the score. When a player misses a hit, the ball restarts from the center towards the other player, with the opponent’s score increasing.
Step 1. Importing Turtle Module.
# Import the turtle library
import turtle
The turtle module must be imported first. This module will be used to construct the entire game.
Step 2. Creating the Canvas.
# Creating the screen with name and size
screen = turtle.Screen()
screen.title("Python Pong game by violet-cat-415996.hostingersite.com ")
screen.setup(width=1000 , height=600)
Step 3. Creating left and right paddles.
# Creating the left paddle
paddle1 = turtle.Turtle()
#Setting its speed as zero, it moves only when key is pressed
paddle1.speed(0)
#Setting shape, color, and size
paddle1.shape("square")
paddle1.color("blue")
paddle1.shapesize(stretch_wid=6, stretch_len=2)
paddle1.penup()
#The paddle is left-centered initially
paddle1.goto(-400, 0)
# Creating the right paddle
paddle2 = turtle.Turtle()
#Setting its speed as zero, it moves only when key is pressed
paddle2.speed(0)
#Setting shape, color, and size
paddle2.shape("square")
paddle2.color("red")
paddle2.shapesize(stretch_wid=6, stretch_len=2)
paddle2.penup()
#The paddle is right-centered initially
paddle2.goto(400, 0)
The next stage is to make the left and right paddles. They are square in shape and are centered vertically on the left or right ends. Their speed is minimal. They only move when keyboard keys are pushed.
Step 4. Next, we create a ball.
# Ball of circle shape
ball = turtle.Turtle()
#Setting the speed of ball to 0, it moves based on the dx and dy values
ball.speed(0)
#Setting shape, color, and size
ball.shape("circle")
ball.color("green")
ball.penup()
#Ball starts from the centre of the screen
ball.goto(0, 0)
#Setting dx and dy that decide the speed of the ball
ball.dx = 2
ball.dy = -2
It’s now time to make the ball. It is circular in shape and is first situated in the center of the screen. Even though its speed is zero, it moves according to the values dx and dy. The location of the ball is updated by dx and dy every time the main game loop runs, giving the illusion of mobility.
Step 5. Creating a scoreboard and initializing scores.
# Initializing the score of the two players
player1 = 0
player2 = 0
# Displaying the score
score = turtle.Turtle()
score.speed(0)
score.penup()
#Hiding the turtle to show text
score.hideturtle()
#Locating the scoreboard on top of the screen
score.goto(0, 260)
#Showing the score
score.write("Player1 : 0 Player2: 0", align="center", font=("Courier", 20, "bold"))
score.write(“Player1 : 0 Player2: 0″, align=”center”, font=(“Courier”, 20, “bold”))
We then reset the scores to zero. Then, on top of the screen, construct a scoreboard to display the current scores. The write() function is used to display text on the window in this case.
Step 6. functions to move paddles and match with keys.
# Function to move the left paddle up
def movePad1Up():
y = paddle1.ycor() #Getting the current y-coordinated of the left paddle
y += 15
paddle1.sety(y) #Updating the y-coordinated of the paddle
# Function to move the left paddle down
def movePad1Down():
y = paddle1.ycor()#Getting the current y-coordinated of the left paddle
y -= 15
paddle1.sety(y)#Updating the y-coordinated of the paddle
# Function to move the right paddle up
def movePad2Up():
y = paddle2.ycor()#Getting the current y-coordinated of the right paddle
y += 15
paddle2.sety(y)#Updating the y-coordinated of the paddle
# Function to move the right paddle down
def movePad2Down():
y = paddle2.ycor()#Getting the current y-coordinated of the right paddle
y -= 15
paddle2.sety(y)#Updating the y-coordinated of the paddle
# Matching the Keyboard buttons to the above functions=
screen.listen()
screen.onkeypress(movePad1Up, "w")
screen.onkeypress(movePad1Down, "s")
screen.onkeypress(movePad2Up, "Up")
screen.onkeypress(movePad2Down, "Down")
Now we’ll build the functions that will allow us to shift the paddles vertically. We are adjusting the y-coordinate of the paddle by 15 units to give it movement. They function as follows:
1. When you press the left arrow button on your keyboard, the movePad1Up() function is called and the left paddle moves up.
2. When you press the right arrow key on your keyboard, the movePad1Down() function is called, and the left paddle slides down.
3. When you press the up arrow button on your keyboard, the movePad2Up() function is called, and the right paddle travels up.
Step 7. Creating the main Game.
#The main game
while True:
#Updating the screen every time with the new changes
screen.update()
#Moving the ball by updating the coordinates
ball.setx(ball.xcor()+ball.dx)
ball.sety(ball.ycor()+ball.dy)
# Checking if ball hits the top of the screen
if ball.ycor() > 280:
ball.sety(280)
ball.dy *= -1 #Bouncing the ball
# Checking if ball hits the bottom of the screen
if ball.ycor() < -280:
ball.sety(-280)
ball.dy *= -1#Bouncing the ball
#Checking if the ball hits the left or right walls, the player missed the hit
if ball.xcor() > 480 or ball.xcor() < -480:
if(ball.xcor() <-480):
player2 += 1 #Increasing the score of right player if left player missed
else:
player1 += 1 #Increasing the score of left player if right player missed
#Starting ball again from center towards the opposite direction
ball.goto(0, 0)
ball.dx *= -1
ball.dy *= -1
#Updating score in the scoreboard
score.clear()
score.write("Player1 : {} Player2: {}".format(player1, player2), align="center", font=("Courier", 20, "bold"))
#Checking if the left player hit the ball
if (ball.xcor() < -360 and ball.xcor() > -370) and (paddle1.ycor() + 50 > ball.ycor() > paddle1.ycor() - 50):
#Increasing score of left player and updating score board
player1 += 1
score.clear()
score.write("Player A: {} Player B: {}".format(player1, player2), align="center", font=("Courier", 20, "bold"))
ball.setx(-360)
#Increasing speed of the ball with the limit 7
if(ball.dy>0 and ball.dy<5): #If dy is positive increasing dy
ball.dy+=0.5
elif(ball.dy<0 and ball.dy>-5): #else if dy is negative decreasing dy
ball.dy-=0.5
if(ball.dx>0 and ball.dx<5):#If dx is positive increasing dx
ball.dx+=0.5
elif(ball.dx<0 and ball.dx>-5): #else if dx is negative decreasing dx
ball.dx-=0.5
#Changing the direction of ball towards the right player
ball.dx *=-1
#Checking if the right player hit the ball
if (ball.xcor() > 360 and ball.xcor() < 370) and (paddle2.ycor() + 50 > ball.ycor() > paddle2.ycor() - 50):
#Increasing score of right player and updating scoreboard
player2 += 1
score.clear()
score.write("Player A: {} Player B: {}".format(player1, player2), align="center", font=("Courier", 20, "bold"))
ball.setx(360)
#Increasing speed of the ball with the limit 7
if(ball.dy>0 and ball.dy<7):#If dy is positive increasing dy
ball.dy+=1
elif(ball.dy<0 and ball.dy>-7):#else if dy is negative decreasing dy
ball.dy-=1
if(ball.dx>0 and ball.dx<7):#If dx is positive increasing dx
ball.dx+=1
elif(ball.dx<0 and ball.dx>-7): #else if dx is negative decreasing dx
ball.dx-=1
#Changing the direction of ball towards the left player
ball.dx*=-1
Finally, we’ve arrived to write the core game’s code. We write it in a while loop that continues indefinitely until the window is closed.
- We begin by updating the screen. The ball is then moved by updating the dx and dy coordinates.
- We bounce the ball by changing the sign of dy after checking if it touches the top and bottom walls.
- We know that if one of the players fails to hit the ball, it will bounce off the left or right barriers. As a result, we look to see if the ball strikes the left or right walls. Change the opponent’s score and refresh the scoreboard.
- Start the ball in the opposite direction from the middle of the screen.
- Finally, we look to see if any of the paddles have made contact with the ball. Update the scoreboard and raise the score. Increase the speed while staying inside the speed limit. 7 Change the direction of the ball’s dx, causing it to move towards the opposing player.
Complete code to Create a Pong Game using Python Turtle
# Import required library import turtle # Create screen sc = turtle.Screen() sc.title("Pong game") sc.bgcolor("Black") sc.setup(width=1000, height=600) # Left paddle left_pad = turtle.Turtle() left_pad.speed(0) left_pad.shape("square") left_pad.color("yellow") left_pad.shapesize(stretch_wid=6, stretch_len=2) left_pad.penup() left_pad.goto(-400, 0) # Right paddle right_pad = turtle.Turtle() right_pad.speed(0) right_pad.shape("square") right_pad.color("yellow") right_pad.shapesize(stretch_wid=6, stretch_len=2) right_pad.penup() right_pad.goto(400, 0) # Ball of circle shape hit_ball = turtle.Turtle() hit_ball.speed(40) hit_ball.shape("circle") hit_ball.color("pink") hit_ball.penup() hit_ball.goto(0, 0) hit_ball.dx = 5 hit_ball.dy = -5 # Initialize the score left_player = 0 right_player = 0 # Displays the score sketch = turtle.Turtle() sketch.speed(0) sketch.color("blue") sketch.penup() sketch.hideturtle() sketch.goto(0, 260) sketch.write("Left_player : 0 Right_player: 0",align="center", font=("Courier", 24, "normal")) # Functions to move paddle vertically def paddleaup(): y = left_pad.ycor() y += 20 left_pad.sety(y) def paddleadown(): y = left_pad.ycor() y -= 20 left_pad.sety(y) def paddlebup(): y = right_pad.ycor() y += 20 right_pad.sety(y) def paddlebdown(): y = right_pad.ycor() y -= 20 right_pad.sety(y) # Keyboard bindings sc.listen() sc.onkeypress(paddleaup, "w") sc.onkeypress(paddleadown, "s") sc.onkeypress(paddlebup, "Up") sc.onkeypress(paddlebdown, "Down") while True: sc.update() hit_ball.setx(hit_ball.xcor()+hit_ball.dx) hit_ball.sety(hit_ball.ycor()+hit_ball.dy) # Checking borders if hit_ball.ycor() > 280: hit_ball.sety(280) hit_ball.dy *= -1 if hit_ball.ycor() < -280: hit_ball.sety(-280) hit_ball.dy *= -1 if hit_ball.xcor() > 500: hit_ball.goto(0, 0) hit_ball.dy *= -1 left_player += 1 sketch.clear() sketch.write("Left_player : {} Right_player: {}".format(left_player, right_player), align="center",font=("Courier", 24, "normal")) if hit_ball.xcor() < -500: hit_ball.goto(0, 0) hit_ball.dy *= -1 right_player += 1 sketch.clear() sketch.write("Left_player : {} Right_player: {}".format(left_player, right_player), align="center",font=("Courier", 24, "normal")) # Paddle ball collision if (hit_ball.xcor() > 360 and hit_ball.xcor() < 370) and (hit_ball.ycor() < right_pad.ycor()+40 and hit_ball.ycor() > right_pad.ycor()-40): hit_ball.setx(360) hit_ball.dx*=-1 if (hit_ball.xcor()<-360 and hit_ball.xcor()>-370) and (hit_ball.ycor()<left_pad.ycor()+40 and hit_ball.ycor()>left_pad.ycor()-40): hit_ball.setx(-360) hit_ball.dx*=-1
Output:
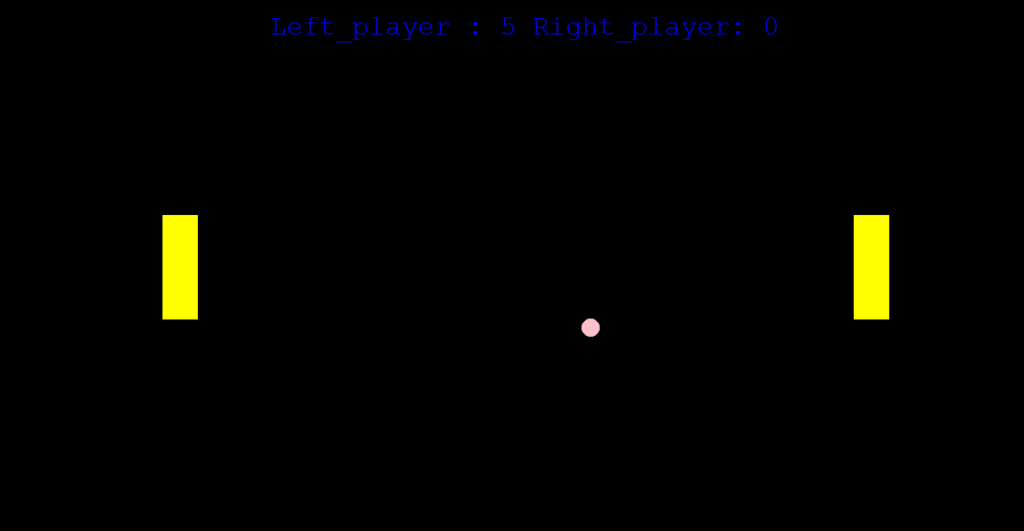
We successfully developed the Pong Game using Python Turtle We learned how to make forms for blocks and balls, as well as how to modify the pace and refresh the screen.
Also Read:
- Draw an Android Logo using Python Turtle
- Create a Stopwatch Using Python Tkinter
- Draw Snowman using Python Turtle
- Draw The Great Indian Flag using Python Turtle
- Draw a Dog using Python Turtle
- Draw a Fish Using Python Turtle
- Draw a Heart Using Python Turtle
- Draw a Christmas Tree using Python Turtle
- Draw Olympic logo using Python Turtle
- Draw Audi Logo in Python Turtle
- Draw Car using Python Turtle
- Draw Penguin using Python Turtle
- Draw Panda using Python Turtle
- Cat using Python Turtle
- Creating a Telegram Bot Using Python
- Get weather forecast using Python
- Hangman Game using Python
- Chess Board using Python
- Google Logo in Python Turtle
- Balloon Shooter Game using Python PyGame
- Complete PyGame Tutorial and Projects
- Draw Windows logo using Python Turtle
- Library Management System Python Project with source code | GUI and Database
- Beginners to Advanced Python Turtle Projects
- Draw Netflix Logo using Python Turtle
- Flappy Bird In Python Pygame with source code
- Create Your Own Web Browser Using Python
- Deploy Machine Learning model using Streamlit
- Tic Tac Toe using Python Project with source code
- Make A Telegram Bot Using Python