
Introduction
Hi everyone, welcome to our website violet-cat-415996.hostingersite.com. In this tutorial, we are going to Draw The Great Indian Flag using Python Turtle. Well, this flag is drawn using the python turtle module. As it provides the inbuilt methods that make our task of drawing the picture easily. The number of coding lines is not very long as we have made use of loops to lessen the repetition of the lines of code.
The Indian flag code is simple and easily understandable for the ones who have just begun to learn python and the turtle module. We have also provided the comments in each section for easy understanding.
For more articles, you can check our website page for python turtle and its projects.
Complete Code to Draw Indian Flag using Python Turtle
#import turtle import turtle from turtle import * # screen for output screen = turtle.Screen() # Defining a turtle Instance t = turtle.Turtle() speed(1) # initially penup() t.penup() t.goto(-200, 125) t.pendown() # Orange Rectangle #white rectangle t.color("orange") t.begin_fill() t.forward(400) t.right(90) t.forward(84) t.right(90) t.forward(400) t.end_fill() t.left(90) t.forward(84) # Green Rectangle t.color("green") t.begin_fill() t.forward(84) t.left(90) t.forward(400) t.left(90) t.forward(84) t.end_fill() # Big Blue Circle t.penup() t.goto(35, 0) t.pendown() t.color("navy") t.begin_fill() t.circle(35) t.end_fill() # Big White Circle t.penup() t.goto(30, 0) t.pendown() t.color("white") t.begin_fill() t.circle(30) t.end_fill() #Mini Blue Circles of Flag t.penup() t.goto(-27, -4) t.pendown() t.color("navy") for i in range(24): t.begin_fill() t.circle(2) t.end_fill() t.penup() t.forward(7) t.right(15) t.pendown() # Small Blue Circle t.color("navy") t.penup() t.goto(10, 0) t.pendown() t.begin_fill() t.circle(10) t.end_fill() #The spokes of India Flag t.penup() t.goto(0, 0) t.pendown() t.pensize(1) for i in range(24): t.forward(30) t.backward(30) t.left(15) #for stick of the flag t.color("Brown") t.pensize(10) t.penup() t.goto(-200,125) t.right(180) t.pendown() t.forward(800) #to hold the #output window turtle.done()
Output
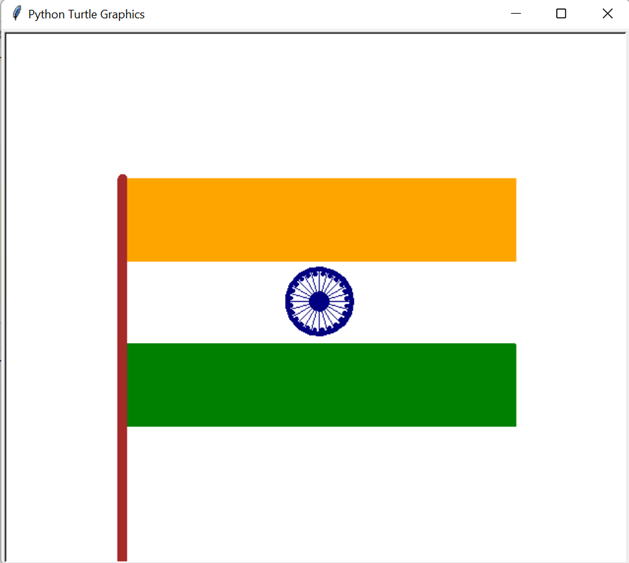
Now, let’s understand our code by breaking it into sub-parts.
1. Import function
import turtle from turtle import *
Importing the turtle module allows us to use its inbuilt methods and functions in our program. Here import * means import all the methods that come in the turtle module for proper creation of the Picture.
2. Setting the turtle and the screen
# screen for output screen = turtle.Screen() # Defining a turtle Instance t = turtle.Turtle() speed(1)
3. Drawing the orange rectangle of the flag
# initially penup() t.penup() t.goto(-200, 125) t.pendown() # Orange Rectangle #white rectangle t.color("orange") t.begin_fill() t.forward(400) t.right(90) t.forward(84) t.right(90) t.forward(400) t.end_fill() t.left(90) t.forward(84)
In this piece of code, we have set the position to goto(200,-125) and started drawing the first rectangle whose length is 400 and width is 84. Filled the rectangle with orange color using the begin_fill method of python turtle. After the orange rectangle is drawn turtle is just moved forward by 84 steps which automatically encompasses the white part of the Flag.
4. Drawing the Green Rectangle of the flag
# Green Rectangle t.color("green") t.begin_fill() t.forward(84) t.left(90) t.forward(400) t.left(90) t.forward(84) t.end_fill()
Here we have drawn the green rectangle of the Indian flag by taking the same length=400 and width=84 as of orange rectangle.
5. Drawing the big Blue Circle
# Big Blue Circle t.penup() t.goto(35, 0) t.pendown() t.color("navy") t.begin_fill() t.circle(35) t.end_fill()
In this part, we have set the turtle position to goto(35,0) to draw the middle blue circle of the Flag. The circle radius is kept at 35 and the color is kept navy blue.
6. Drawing the big White Circle inside the blue circle of the Indian Flag using Python Turtle
# Big White Circle t.penup() t.goto(30, 0) t.pendown() t.color("white") t.begin_fill() t.circle(30) t.end_fill()
In this block, the turtle is moved to 5 steps inside of the navy blue circle. Set it to the position goto(30,0)and kept the radius to 30 and the white circle is drawn.
7. Drawing the 24 minicircles
t.penup()
t.goto(-27, -4)
t.pendown()
t.color(“navy”)
for i in range(24):
t.begin_fill()
t.circle(2)
t.end_fill()
t.penup()
t.forward(7)
t.right(15)
t.pendown()
In this code, we have set the turtle position to goto(-27,-4) and the color as navy. The for loop is used so that turtle will draw 24 mini circles of the Ashok Chakra. The radius of those circles is 2 and given the angle of right(15) so that circle will be drawn at the proper position in the inner circle of the flag.
8. Draw the middle blue circle
# Small Blue Circle
t.color(“navy”)
t.penup()
t.goto(10, 0)
t.pendown()
t.begin_fill()
t.circle(10)
t.end_fill()
Here we have set the turtle at the position goto(10,0) and drawn the innermost navy blue circle of the India Flag.
9. Drawing the spokes
#The spokes of the Indian Flag
t.penup()
t.goto(0, 0)
t.pendown()
t.pensize(1)
for i in range(24):
t.forward(30)
t.backward(30)
t.left(15)
Here, we have set the position of the turtle in the middle i.e goto(0,0) kept the pensize(1), and drawn 24 spokes by using the for loop function.
10. Drawing the stick of the India flag
t.color("Brown") t.pensize(10) t.penup() t.goto(-200,125) t.right(180) t.pendown() t.forward(800)
In this part we have set the turtle position to goto(-200,125) increased the pen size to 10 and drawn the straight line of 800 steps which is nothing but the stick of the flag.
So here we have completed the drawing of The Great Indian Flag using Python turtle successfully.
Thank you for reading this article.
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector
Keywords->python turtle design, turtle, design, 12th class python project, python for kids, 12th class, beautiful turtle designs, python