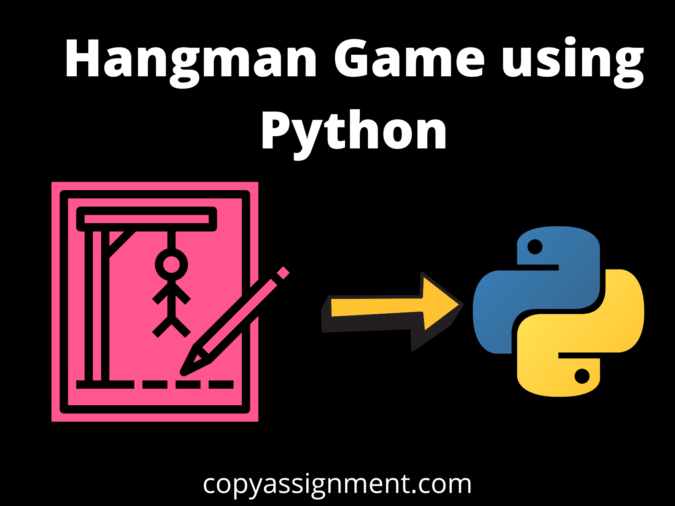
Introduction
In our previous articles, we developed a chessboard, multiple logos, and some basic games using the Turtle library. And today, in this article Hangman Game using Python, we are again going to develop a Hangman Game using Python.
We will use multiple modules, and functions in the turtle library for the development of this amazing game.
Before starting with the scripting of this game Hangman Game using Python, let us take a look at what the game is all about, how it works, some rules, and most importantly what we are going to use.
What the game is all about?
The goal of a Hangman Game in Python is to guess the name of the movie by guessing the letters (A-Z). In this game, if the player correctly guesses the correct letter that is within the word, the letter appears in its proper location. The user must guess the correct word until a man appears to be hanged, and at that point, the game ends. With each correct guess, the empty boxes get filled with that correct letter. And with each wrong guess different parts of the man start to appear and finally when the number of wrong guesses is over, a hanged man is shown and that’s where the game ends.
What we are going to use?
- random module: To make use of random numbers
- time module: This allows us to work with the time
- turtle library: Add graphics to the game.
Rules for development:
- Whenever the letter is repeated by the user, the user must be notified with a message.
- If a user enters a letter then that letter should appear on every box where it should be.
- A user can only make 9 wrong guesses.
Code flow with explanation:
(1) Importing all the required modules:
import random
import turtle
import sys
import time
Explanation:
Here we have imported all the modules that we aim to use for this project. turtle library will help in the graphics section, random module to make random choices and generate random numbers, time module to work with time, and sys module to interact with the python environment.
(2) Declaring all the variables:
flagW=False
flagL=False
flafA=False
flagWr=False
gameFlag=True
wrongCount=0
hmTC=(0,0)
hm=turtle.Turtle()
hm.speed(0)
state=turtle.Turtle()
state.ht()
Explanation:
In the above code, we have declared some variables. We have also used some functions of the turtle module like speed() etc. Some of these variables are used to keep track of the guesses and some are used for the graphics of the hanged man.
(3) Defining functions:
correct()
def correct():
state.clear()
state.color("green")
state.write("Correct!!",font=("Arial",50,"bold"))
Explanation:
This function named correct() is used to display a text “Correct” that will inform the user that the entered letter is correct. In order to display the text, we have used the write() method from the turtle module.
In write() we have passed our text and a font parameter that holds the font category, size, and font-weight.
lose()
def lose():
state.clear()
state.color("white")
state.write("YOU LOSE!!", font=("Arial",50,"bold"))
cordxy=letter[0].pos()
temp=turtle.Turtle()
temp.ht()
temp.speed(0)
temp.color("white")
temp.penup()
temp.setpos(cordxy[0],cordxy[1]+40)
temp.pendown()
temp.write("This was the movie:",align="left", font=("Arial", 30, "bold"))
for i in range(len(movie)):
if(movie[i] not in user_list_correct):
letter[i].color("red")
letter[i].write(movie[i],align="left", font=("Arial", 20, "bold"))
Explanation:
In the above lines, we have defined lose() function, which will come into the picture when the player loses the game.
In lines 1 to 4: We have passed the text “YOU LOSE” using the write() function.
Line 5: And now, we have declared a variable that will store the turtle and we will use this variable throughout the program to access functions, methods, etc that are in the turtle library.
In line 6: To hide the turtle.
Line 7: To set the speed of the turtle using the speed() functions.
In line 8: In this line the color is set to white, using the color() function from the turtle library.
Line 9: penup() helps the user to pick up the pen. Many times we want to pick up the pen and don’t want to leave extra lines on the screen and so penup() is used.
Line 10: setpos() will move the turtle to the desired position, It takes 2 arguments which are basically in the form of x and y coordinates.
Line 11: pendown() is the default state of the turtle. With this command, we can assure that the turtle is ready to draw.
Line 12: This line simply prints “This was the movie”
Line 13 to 16: Here we have used a loop that processes each letter that is entered by the user and does the work of validating that letter if it is present in the name of the movie or not. If not present that print that letter with red color.
win()
def win():
state.clear()
state.color("white")
state.write("YOU WIN!!", font=("Arial",50,"bold"))
Explanation:
This function named win() is called when the user wins the game. It displays the text “YOU WIN” that will inform the user that the game is over and the user has guessed all the letters correctly. In write() we have passed our text and a font parameter that holds the font category, size, and font-weight.
already()
def already():
state.clear()
state.color("green")
state.write("YOU Already pressed that key!!", font=("Arial",50,"bold"))
Explanation:
This function is called when the user has already pressed the letter he pressed earlier. It informs the user about this message by displaying the text “YOU Already pressed that key!!”
wrong()
def wrong():
state.clear()
state.color("red")
state.write("WRONG", font=("Arial",50,"bold"))
Explanation:
wrong() function comes into the picture when the user guesses the letter incorrectly. This function also informs the user by displaying a “WRONG” message.
(4) Evaluating individual letters:
def A():
evaluate(movie,"A")
def B():
evaluate(movie,"B")
def C():
evaluate(movie,"C")
def D():
evaluate(movie,"D")
def E():
evaluate(movie,"E")
def F():
evaluate(movie,"F")
def G():
evaluate(movie,"G")
def H():
evaluate(movie,"H")
def I():
evaluate(movie,"I")
def J():
evaluate(movie,"J")
def K():
evaluate(movie,"K")
def L():
evaluate(movie,"L")
def M():
evaluate(movie,"M")
def N():
evaluate(movie,"N")
def O():
evaluate(movie,"O")
def P():
evaluate(movie,"P")
def Q():
evaluate(movie,"Q")
def R():
evaluate(movie,"R")
def S():
evaluate(movie,"S")
def T():
evaluate(movie,"T")
def U():
evaluate(movie,"U")
def V():
evaluate(movie,"V")
def W():
evaluate(movie,"W")
def X():
evaluate(movie,"X")
def Y():
evaluate(movie,"Y")
def Z():
evaluate(movie,"Z")
Explanation:
In the above lines of code, we have defined separate functions for each letter. Basically, when the player presses a letter, a function named will that letter will be called. We have used evaluate() function that helps us to evaluate whether the letter that is pressed by the user is present in the name of the movie or not.
(5) Designing and controlling the hangman:
def Hangman(num):
global hmTC
if(num==0):
hm.penup()
hm.setpos(-670,-340)
hm.pendown()
hm.ht()
hm.begin_fill()
hm.fd(400)
hm.lt(90)
hm.fd(50)
hm.lt(90)
hm.fd(390)
hm.rt(90)
hm.fd(600)
hm.rt(90)
hm.fd(400)
hm.rt(90)
hm.fd(20)
hm.lt(90)
hm.fd(10)
hm.lt(90)
hmTC=hm.pos()
hm.fd(30)
hm.lt(90)
hm.fd(420)
hm.lt(90)
hm.fd(550)
hm.end_fill()
hm.pensize(5)
Explanation:
The above code will simply draw one horizontal long line and a short vertical line. This is the default drawing that will appear on the screen as the game starts.
Output:

elif(num==1):
hm.penup()
hm.setpos(hmTC[0],hmTC[1])
hm.rt(90)
hm.pendown()
hm.circle(40)
Explanation:
Now if the player guesses the letter incorrectly for 1st time then a circle will appear on the screen and that is the face of the hangman. To draw the circle we have used the circle() function from the turtle library.
Output:

elif(num==2):
hm.penup()
hm.setpos(hmTC[0],hmTC[1]-80)
hm.lt(90)
hm.pendown()
hm.fd(140)
hmTC=hm.pos()
Explanation:
If the player guesses the letter incorrectly for 2nd time then a straight line joining the circle will appear on the screen and that is the body of the hangman. To draw the line we have used fd() and lt() i.e forward and left.
Output:

elif(num==3):
hm.penup()
hm.rt(30)
hm.pendown()
hm.fd(100)
hm.rt(60)
hm.fd(10)
hm.penup()
hm.rt(180)
hm.fd(10)
hm.rt(30)
hm.fd(100)
hm.rt(90)
hm.pendown()
elif(num==4):
hm.penup()
hm.setpos(hmTC[0],hmTC[1])
hm.pendown()
hm.lt(60)
hm.fd(100)
hm.lt(60)
hm.fd(10)
Explanation:
If the player guesses the letter incorrectly for 3rd time then one leg of the hangman will be visible on-screen and if the players guess the wrong letter for 4th time then another leg will appear.
Output:

elif(num==5):
hm.penup()
hm.setpos(hmTC[0],hmTC[1])
hm.lt(90)
hm.fd(90)
hm.lt(180)
hm.rt(30)
hm.pendown()
hm.fd(80)
hm.rt(60)
elif(num==6):
hm.penup()
hm.setpos(hmTC[0],hmTC[1])
hm.rt(90)
hm.fd(90)
hm.lt(180)
hm.lt(30)
hm.pendown()
hm.fd(80)
Explanation for this part of Hangman Game using Python:
Now if the player guesses the letter incorrectly for the 5th time then the left hand of the hangman will appear and if wrong for the 6th time then the right hand will appear.
Output:
elif(num==7):
hm.penup()
hm.setpos(-235.00,265.00)
hm.rt(90)
hm.pendown()
hm.circle(4)
elif(num==8):
hm.penup()
hm.setpos(-265,265.00)
hm.rt(90)
hm.pendown()
hm.circle(4)
Explanation:
The letter guessed incorrectly for the 7th and the 8th time will show the left and right eyes of the hangman in the form of a circle.
Output:

else:
hm.penup()
hm.setpos(hm.pos()[0]+25,hm.pos()[1]-35)
hm.pendown()
hm.rt(30)
hm.circle(10,180)
Explanation:
And finally, if guessed wrong for the 9th time then a sad expression on the face of the hangman will appear. That’s where the game will end and the “YOU LOSE” message will appear.
Output:

(6) Creating empty boxes:
def createDash(num):
dash=[]
letter=[]
for i in range(num):
dash.append(turtle.Turtle())
dash[i].color("#800000","white")
dash[i].pensize(4)
letter.append(turtle.Turtle())
dash[i].ht()
dash[i].speed(0)
letter[i].ht()
dash[i].penup()
letter[i].penup()
dash[i].setpos(-600+i*50,-200)
letter[i].setpos(-595+i*50,-200)
dash[i].pendown()
letter[i].pendown()
if(i in movie_space_position):
dash[i].penup()
continue
dash[i].begin_fill()
dash[i].fd(30)
dash[i].lt(90)
dash[i].fd(30)
dash[i].lt(90)
dash[i].fd(30)
dash[i].lt(90)
dash[i].fd(30)
dash[i].end_fill()
return letter
Explanation:
For the player to type the desired letters there should be empty boxes. To create empty boxes, first of all, we need to set the position of our turtle. Once the position is set we can start creating the boxes. To set the background color to white we have used dash[i].color(“#800000″,”white”).
To increase the width of the cursor we have used the pensize() function. penup() used to pick up the pen when not drawing and pendown() to set the turtle to default state i.e drawing. To make squares we have used fd which moves the cursor in the forwarding direction and lt which moves the cursor in the left direction.
Output:

(7) Working logic of the game:
def evaluate(movie,user_input):
global wrongCount
global gameFlag
if(gameFlag==True):
if(user_input in movie and user_input not in user_list_correct):
tempPositionList=[]
for i in range(len(movie)):
if(movie[i]==user_input):
letter[i].write(user_input,align="left", font=("Arial", 20, "bold"))
user_list_correct.append(user_input)
tempPositionList.append(i)
correct()
if(len(user_list_correct)==len(movie)):
win()
gameFlag=False
else:
if(user_input in user_list_correct):
already()
else:
wrong()
user_list_wrong.append(user_input)
wrongCount+=1
if(wrongCount>=9):
lose()
gameFlag=False
Hangman(wrongCount)
Explanation:
Here for the core working of the game, we have used loops, if & else block.
Line 1 to 3: We have defined our function and in the next two lines we have declared two global variables.
Line 4: if block is used to check if the gameFlag variable is True or not. If it is true then the code flow continues.
Line 5: Again if block is used to check if the user_input is present in the movie and user_list_correct. Then we used a for loop to take user inputs with a range equal to the length of the movie.
In line 6: Declared an empty list.
Line 7: We have used a for loop equal to the length of the movie
Line 8 to 15: Holds the logic of printing the letters in the box that are entered by the player. If the letter entered by the player is present in the name of the movie then it appears on the empty boxes where it should be. A win() function is called if the length of the user_list_correct is equal to the length of the movie.
In line 16: We have used the else block and inside that block other conditional blocks.
Line 17 to 18: Checks if the user has pressed the same letter again, If yes then the already() function is called.
Line 19 to 26: Checks if the user has entered the wrong letter or not. And a call to lose() function is made if the user runs out of guesses and parts of Hangman start to appear on each wrong guess.
f = open("movies.txt")
movie_list=[]
for line in f:
line = line.strip()
movie_list.append(line)
movie = random.choice(movie_list)
movie=movie.upper()
l = len(movie)
movie = list(movie)
movie_space_count=0
movie_space_position=[]
user_list_correct = []
user_list_wrong = []
user_input=[]
for c in range(len(movie)):
if(movie[c]==' '):
movie_space_count+=1
movie_space_position.append(c)
user_list_correct.append(' ')
Explanation:
In line 1: Open the file named movies.txt and store it in the f variable.
Line 2: Empty list named movie_list
In lines 3 to 5: A for loop is used to strip the lines i.e names of the movies in the movies.txt file and append each line to movie_list.
Line 6: Select a random movie from the list and store it in the movie variable.
In line 7: upper() function changes all the letters to capital.
Line 8: l variable stores the length of the movie that is selected using the random module.
In line 9: list() is used to change the elements in the form of a list.
Line 10 to 14: Variable declarations.
In lines 15 to 19: If in any movie there is more than 1 word then there will be space in between them and in order to solve that we have used an if the condition that checks if there is an empty character then the moive_space_count is incremented.
(8) Adding a screen:
wn = turtle.Screen()
wn.setup (width=1920, height=1080, startx=0, starty=0)
wn.bgpic("imain.gif")
wn.tracer(2)
Hangman(0)
letter = createDash(len(movie))
Explanation:
Line 1: To display all the graphics we need a screen that can be added using Turtle.screen()
In line 2: setup() is used to set the height and the width of the screen.
Line 3: A background picture can be added using bgpic()
In line 4: tracer() is used to handle the animations by turning them on and off with a delay timer for updating the graphics.
Line 5: This line is simply a call to the Hangman function with a parameter 0.
In line 6: Stores the function createDash() in variable letter.
Line 7: Sets the delay to 1.
(9) Tracing keys and handling user input:
wn.onkey(A,"a")
wn.onkey(B,"b")
wn.onkey(A,"a")
wn.onkey(A,"A")
wn.onkey(B,"b")
wn.onkey(B,"B")
wn.onkey(C,"c")
wn.onkey(C,"C")
wn.onkey(D,"d")
wn.onkey(D,"D")
wn.onkey(E,"e")
wn.onkey(E,"E")
wn.onkey(F,"f")
wn.onkey(F,"F")
wn.onkey(G,"g")
wn.onkey(G,"G")
wn.onkey(H,"h")
wn.onkey(H,"H")
wn.onkey(I,"i")
wn.onkey(I,"I")
wn.onkey(J,"j")
wn.onkey(J,"J")
wn.onkey(K,"k")
wn.onkey(K,"K")
wn.onkey(L,"l")
wn.onkey(L,"L")
wn.onkey(M,"m")
wn.onkey(M,"M")
wn.onkey(N,"n")
wn.onkey(N,"N")
wn.onkey(O,"o")
wn.onkey(O,"O")
wn.onkey(P,"p")
wn.onkey(P,"P")
wn.onkey(Q,"q")
wn.onkey(Q,"Q")
wn.onkey(R,"r")
wn.onkey(R,"R")
wn.onkey(S,"s")
wn.onkey(S,"S")
wn.onkey(T,"t")
wn.onkey(T,"T")
wn.onkey(U,"u")
wn.onkey(U,"U")
wn.onkey(V,"v")
wn.onkey(V,"V")
wn.onkey(W,"w")
wn.onkey(W,"W")
wn.onkey(X,"x")
wn.onkey(X,"X")
wn.onkey(Y,"y")
wn.onkey(Y,"Y")
wn.onkey(Z,"z")
wn.onkey(Z,"Z")
if(gameFlag==True):
wn.listen()
turtle.mainloop()
Explanation:
The letters that are pressed by the player need to be traced and should be processed such that it verifies if it matches the letters that are in the name of the movie or not.
Line 1 to 26: To add this functionality, we have used onkey() is used to trace the events on the keyboard. To trace each letter we have used onkey() along with the letters from A-Z as parameters in each individual call to onkey().
Line 27 to 28: Checks if the gameFlag is True or False. If True then wn.listen() is called and is simply listens for the keyboard input.
Line 29: turtle.mainloop() is a call to the turtle library.
Final Output:

Required Files:
The below-attached file contains all the movies. Feel free to add yous and extend the list.
Complete Code to make Hangman Game using Python:
#Importing all the required modules import random import turtle import sys import time #Declaring all the variables flagW=False flagL=False flafA=False flagWr=False gameFlag=True wrongCount=0 hmTC=(0,0) hm=turtle.Turtle() hm.speed(0) state=turtle.Turtle() state.ht() #Defining functions def correct(): state.clear() state.color("green") state.write("Correct!!",font=("Arial",50,"bold")) def lose(): state.clear() state.color("white") state.write("YOU LOSE!!", font=("Arial",50,"bold")) cordxy=letter[0].pos() temp=turtle.Turtle() temp.ht() temp.speed(0) temp.color("white") temp.penup() temp.setpos(cordxy[0],cordxy[1]+40) temp.pendown() temp.write("This was the movie:",align="left", font=("Arial", 30, "bold")) for i in range(len(movie)): if(movie[i] not in user_list_correct): letter[i].color("red") letter[i].write(movie[i],align="left", font=("Arial", 20, "bold")) def win(): state.clear() state.color("white") state.write("YOU WIN!!", font=("Arial",50,"bold")) def already(): state.clear() state.color("green") state.write("YOU Already pressed that key!!", font=("Arial",50,"bold")) def wrong(): state.clear() state.color("red") state.write("wrong", font=("Arial",50,"bold")) #Evaluating individual letters def A(): evaluate(movie,"A") def B(): evaluate(movie,"B") def C(): evaluate(movie,"C") def D(): evaluate(movie,"D") def E(): evaluate(movie,"E") def F(): evaluate(movie,"F") def G(): evaluate(movie,"G") def H(): evaluate(movie,"H") def I(): evaluate(movie,"I") def J(): evaluate(movie,"J") def K(): evaluate(movie,"K") def L(): evaluate(movie,"L") def M(): evaluate(movie,"M") def N(): evaluate(movie,"N") def O(): evaluate(movie,"O") def P(): evaluate(movie,"P") def Q(): evaluate(movie,"Q") def R(): evaluate(movie,"R") def S(): evaluate(movie,"S") def T(): evaluate(movie,"T") def U(): evaluate(movie,"U") def V(): evaluate(movie,"V") def W(): evaluate(movie,"W") def X(): evaluate(movie,"X") def Y(): evaluate(movie,"Y") def Z(): evaluate(movie,"Z") #Designing and controlling the hangman def Hangman(num): global hmTC if(num==0): hm.penup() hm.setpos(-670,-340) hm.pendown() hm.ht() hm.begin_fill() hm.fd(400) hm.lt(90) hm.fd(50) hm.lt(90) hm.fd(390) hm.rt(90) hm.fd(600) hm.rt(90) hm.fd(400) hm.rt(90) hm.fd(20) hm.lt(90) hm.fd(10) # print(hmTC[0]) hm.lt(90) hmTC=hm.pos() hm.fd(30) hm.lt(90) hm.fd(420) hm.lt(90) hm.fd(550) hm.end_fill() #hm.end_fill() hm.pensize(5) elif(num==1): hm.penup() hm.setpos(hmTC[0],hmTC[1]) hm.rt(90) hm.pendown() hm.circle(40) #print(hm.pos()) elif(num==2): hm.penup() hm.setpos(hmTC[0],hmTC[1]-80) hm.lt(90) hm.pendown() hm.fd(140) hmTC=hm.pos() elif(num==3): hm.penup() hm.rt(30) hm.pendown() hm.fd(100) hm.rt(60) hm.fd(10) hm.penup() hm.rt(180) hm.fd(10) hm.rt(30) hm.fd(100) hm.rt(90) hm.pendown() elif(num==4): hm.penup() hm.setpos(hmTC[0],hmTC[1]) hm.pendown() hm.lt(60) hm.fd(100) hm.lt(60) hm.fd(10) #hm.rt(90) elif(num==5): hm.penup() hm.setpos(hmTC[0],hmTC[1]) hm.lt(90) hm.fd(90) hm.lt(180) hm.rt(30) hm.pendown() hm.fd(80) hm.rt(60) elif(num==6): hm.penup() hm.setpos(hmTC[0],hmTC[1]) hm.rt(90) hm.fd(90) hm.lt(180) hm.lt(30) hm.pendown() hm.fd(80) elif(num==7): hm.penup() hm.setpos(-235.00,265.00) hm.rt(90) hm.pendown() hm.circle(4) elif(num==8): hm.penup() hm.setpos(-265,265.00) hm.rt(90) hm.pendown() hm.circle(4) else: hm.penup() hm.setpos(hm.pos()[0]+25,hm.pos()[1]-35) hm.pendown() hm.rt(30) hm.circle(10,180) #Creating empty boxes def createDash(num): dash=[] letter=[] for i in range(num): #print(movie_space_position) dash.append(turtle.Turtle()) dash[i].color("#800000","white") dash[i].pensize(4) letter.append(turtle.Turtle()) dash[i].ht() dash[i].speed(0) letter[i].ht() dash[i].penup() letter[i].penup() dash[i].setpos(-600+i*50,-200) letter[i].setpos(-595+i*50,-200) dash[i].pendown() letter[i].pendown() if(i in movie_space_position): dash[i].penup() continue dash[i].begin_fill() dash[i].fd(30) dash[i].lt(90) dash[i].fd(30) dash[i].lt(90) dash[i].fd(30) dash[i].lt(90) dash[i].fd(30) dash[i].end_fill() return letter #Working logic of the game def evaluate(movie,user_input): global wrongCount global gameFlag if(gameFlag==True): if(user_input in movie and user_input not in user_list_correct): tempPositionList=[] for i in range(len(movie)): if(movie[i]==user_input): letter[i].write(user_input,align="left", font=("Arial", 20, "bold")) user_list_correct.append(user_input) tempPositionList.append(i) correct() if(len(user_list_correct)==len(movie)): win() gameFlag=False else: if(user_input in user_list_correct): already() else: wrong() user_list_wrong.append(user_input) wrongCount+=1 if(wrongCount>=9): lose() gameFlag=False Hangman(wrongCount) f = open("movies.txt") movie_list=[] for line in f: line = line.strip() movie_list.append(line) movie = random.choice(movie_list) movie=movie.upper() l = len(movie) movie = list(movie) movie_space_count=0 movie_space_position=[] user_list_correct = [] user_list_wrong = [] user_input=[] for c in range(len(movie)): if(movie[c]==' '): movie_space_count+=1 movie_space_position.append(c) user_list_correct.append(' ') ##Adding a screen wn = turtle.Screen() wn.setup (width=1920, height=1080, startx=0, starty=0) wn.bgpic("imain.gif") wn.tracer(2) Hangman(0) letter = createDash(len(movie)) wn.tracer(1) #Tracing keys and handling user input wn.onkey(A,"a") wn.onkey(B,"b") wn.onkey(A,"a") wn.onkey(A,"A") wn.onkey(B,"b") wn.onkey(B,"B") wn.onkey(C,"c") wn.onkey(C,"C") wn.onkey(D,"d") wn.onkey(D,"D") wn.onkey(E,"e") wn.onkey(E,"E") wn.onkey(F,"f") wn.onkey(F,"F") wn.onkey(G,"g") wn.onkey(G,"G") wn.onkey(H,"h") wn.onkey(H,"H") wn.onkey(I,"i") wn.onkey(I,"I") wn.onkey(J,"j") wn.onkey(J,"J") wn.onkey(K,"k") wn.onkey(K,"K") wn.onkey(L,"l") wn.onkey(L,"L") wn.onkey(M,"m") wn.onkey(M,"M") wn.onkey(N,"n") wn.onkey(N,"N") wn.onkey(O,"o") wn.onkey(O,"O") wn.onkey(P,"p") wn.onkey(P,"P") wn.onkey(Q,"q") wn.onkey(Q,"Q") wn.onkey(R,"r") wn.onkey(R,"R") wn.onkey(S,"s") wn.onkey(S,"S") wn.onkey(T,"t") wn.onkey(T,"T") wn.onkey(U,"u") wn.onkey(U,"U") wn.onkey(V,"v") wn.onkey(V,"V") wn.onkey(W,"w") wn.onkey(W,"W") wn.onkey(X,"x") wn.onkey(X,"X") wn.onkey(Y,"y") wn.onkey(Y,"Y") wn.onkey(Z,"z") wn.onkey(Z,"Z") if(gameFlag==True): wn.listen() turtle.mainloop()
Reference Links:
Official documentation: Click here
Beginners to Advanced Turtle Tutorials and Projects: Click here
EndNote:
We hope you learned something new from the article Hangman Game using Python. We believe building projects is a great way of learning any language. Try upgrading the game as you want to. Try to implement what you have learned from this article in the development of other projects and keep learning and building. Thank you for visiting our website.
Also Read:
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
- 23 AI Tools You Won’t Believe are Free
- Python 3.12.1 is Now Available
- Best Deepfake Apps and Websites You Can Try for Fun
- Amazon launched free Prompt Engineering course: Enroll Now
- 10 GitHub Repositories to Master Machine Learning
- Hello World in 35 Programming Languages
- How to Scrape Data From Any Website with Python?
- Become Job Ready With Free Harvard Computer Science course: Enroll Now
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Udacity Giving Free Python Course: Here is how to Enroll
- Love Babbar’s Income Revealed
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- Microsoft Giving Free Python Course in 2023: Enroll Now
- Top 5 Free Python Courses on YouTube in 2024
- Complete Python Roadmap for Beginners in 2024
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- What is an AI Tool?
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- Top Free AI Tools for Students and Job Seekers
- Unveiling the Future of AI Detector