
Introduction
The Employee Management System Project in Python will be a beginner-friendly Python project that will educate students on how to construct console-based Python applications. We will provide you with the whole source code for the Python project so that you can immediately install it on your PC and begin learning.
Employee Management System Python project assists in the automation of manual operations, saving both time and money. This system protects the professional and personal information of employees and the company. This project can be very helpful if you are creating a final-year project in Python.
This Employee Management System in Python with MySQL database is constructed using the Anaconda Spider in the Python Programming Language. This Simple Project was developed using console-based programming and is linked to a MySQL database as the system’s back-end.
To build an Employee Management System in Python with source code we have to connect the MySQL database to our program, for that, we need to install some Packages
Project Overview: Employee Management System Project in Python
Project Name: | Employee Management System Project in Python |
Abstract: | This is a straightforward GUI-based program that is simple to grasp and utilize. The GUI is created with the Tkinter package. |
Language/Technologies Used: | Python, Tkinter, mysql |
IDE | Pycharm(Recommended) |
Python version (Recommended): | 3.8 or 3.9 |
Type/Category: | Final Year Project using Python |
Developer: | Vatsal Rakholiya |
Updates: | 0 |
Installing Packages
To install the MySQL client package, type the following command:
pip install mysqlclient
To install the mysql-connector-python package, type the following command:
pip install mysql-connector-python
To install the pymysql package, type the following command:
pip install pymysql
Note: If any error comes up like(Can’t connect to MySQL server on ‘localhost:3306’ (10061))
Install MYSQL Community Software(mysql-installer-community)
After you install a MySQL package, you are ready to work with actual databases.
In order to develop an Employee Management System in Python with source code that uses MySQL database, we must connect Python to MySQL.
Code Flow:
Creating a database in MySQL “employee”
import mysql.connector
#making Connection
con = mysql.connector.connect (
host = " localhost " , user = " root " , password = "root")
mycursor= con.cursor() #allows row-by-row processing of the result sets.
mycursor.execute("CREATE DATABASE "employee")
Here we import the connector module from the MySQL library, then create a variable called “con” for the host, user, and password.
Creating a Table in MySQL
import mysql.connector
#making Connection
con = mysql.connector.connect (
host = " localhost " , user = " root " , password = "root" , database = " employee " )
mycursor= con.cursor()
mycursor.execute("CREATE TABLE empdata ( Id INT(11) PRIMARY KEY , Name VARCHAR(1800), Emial_Id TEXT(1800),Phone_no BIGINT( 11 ), Address TEXT(1000), Post TEXT(1000), Salary BIGINT(20))")
Create a table called “empdata” with the following table fields: “Id”, “Name”, “Email Id”, “Phone No. “, “Address”, “Post”, and “Salary”.
Add Employee to employee management system project python
# Function to Add_Employee
def Add_Employ():
print("{:>60}".format("-->>Add Employee Record<<--"))
Id = input("Enter Employee Id: ")
# checking If Employee Id is Exit Or Not
if (check_employee(Id) == True):
print("Employee ID Already Exists\nTry Again..")
press = input("Press Any Key To Continue..")
Add_Employ()
Name = input("Enter Employee Name: ")
# checking If Employee Name is Exit Or Not
if (check_employee_name(Name) == True):
print("Employee Name Already Exists\nTry Again..")
press = input("Press Any Key To Continue..")
Add_Employ
Email_Id = input("Enter Employee Email ID: ")
if(re.fullmatch(regex, Email_Id)):
print("Valid Email")
else:
print("Invalid Email")
press = input("Press Any Key To Continue..")
Add_Employ()
Phone_no = input("Enter Employee Phone No.: ")
if(Pattern.match(Phone_no)):
print("Valid Phone Number")
else:
print("Invalid Phone Number")
press = input("Press Any Key To Continue..")
Add_Employ()
Address = input("Enter Employee Address: ")
Post = input("Enter Employee Post: ")
Salary = input("Enter Employee Salary: ")
data = (Id, Name, Email_Id, Phone_no, Address, Post, Salary)
# Instering Employee Details in
# the Employee (empdata) Table
sql = 'insert into empdata values(%s,%s,%s,%s,%s,%s,%s)'
c = con.cursor()
# Executing the sql Query
c.execute(sql, data)
# Commit() method to make changes in the table
con.commit()
print("Successfully Added Employee Record")
press = input("Press Any Key To Continue..")
menu()
The Add Employee function will request the Employee Id before using the check employee function to see whether or not the employee to be added already exists in our MYSQL database. If the employee’s details do not exist, then Add Employee function will prompt you for them. After obtaining all of these details from the system’s user, the data is simply entered into our Employee details table in our Employee Management System Project in Python.
Check Employee in employee management system project python
# Function To Check if Employee With
# given Name Exist or not
def check_employee_name(employee_name):
# query to select all Rows from
# employee(empdata) table
sql = 'select * from empdata where Name=%s'
# making cursor buffered to make
# rowcount method work properly
c = con.cursor(buffered=True)
data = (employee_name,)
# Execute the sql query
c.execute(sql, data)
# rowcount method to find number
# of rowa with given values
r = c.rowcount
if r == 1:
return True
else:
return False
# Function To Check if Employee With
# given Id Exist or not
def check_employee(employee_id):
# query to select all Rows from
# employee(empdata) table
sql = 'select * from empdata where Id=%s'
# making cursor buffered to make
# rowcount method work properly
c = con.cursor(buffered=True)
data = (employee_id,)
# Execute the sql query
c.execute(sql, data)
# rowcount method to find number
# of rowa with given values
r = c.rowcount
if r == 1:
return True
else:
return False
The check employee function in the Employee Management System Project in Python takes an employee id as input and checks to see whether there are any employees with that id in the employee details record. It checks this using the cursor.rowcount() function, which counts the number of rows that meet the supplied details. It is a utility function that will be used in subsequent operations like the Add employee function, among others.
Adding Display Employee function to python employee management system
def Display_Employ():
print("{:>60}".format("-->> Display Employee Record <<--"))
# query to select all rows from Employee (empdata) Table
sql = 'select * from empdata'
c = con.cursor()
# Executing the sql query
c.execute(sql)
# Fetching all details of all the Employees
r = c.fetchall()
for i in r:
print("Employee Id: ", i[0])
print("Employee Name: ", i[1])
print("Employee Email Id: ", i[2])
print("Employee Phone No.: ", i[3])
print("Employee Address: ", i[4])
print("Employee Post: ", i[5])
print("Employee Salary: ", i[6])
print("\n")
press = input("Press Any key To Continue..")
menu()
The Display Employees function is essentially a SQL select query that retrieves and prints all of the employee information table records line by line.
Add Update Employee function in employee management system python
def Update_Employ():
print("{:>60}".format("-->> Update Employee Record <<--\n"))
Id = input("Enter Employee Id: ")
# checking If Employee Id is Exit Or Not
if(check_employee(Id) == False):
print("Employee Record Not exists\nTry Again")
press = input("Press Any Key To Continue..")
menu()
else:
Email_Id = input("Enter Employee Email ID: ")
if(re.fullmatch(regex, Email_Id)):
print("Valid Email")
else:
print("Invalid Email")
press = input("Press Any Key To Continue..")
Update_Employ()
Phone_no = input("Enter Employee Phone No.: ")
if(Pattern.match(Phone_no)):
print("Valid Phone Number")
else:
print("Invalid Phone Number")
press = input("Press Any Key To Continue..")
Update_Employ()
Address = input("Enter Employee Address: ")
# Updating Employee details in empdata Table
sql = 'UPDATE empdata set Email_Id = %s, Phone_no = %s, Address = %s where Id = %s'
data = (Email_Id, Phone_no, Address, Id)
c = con.cursor()
# Executing the sql query
c.execute(sql, data)
# commit() method to make changes in the table
con.commit()
print("Updated Employee Record")
press = input("Press Any Key To Continue..")
menu()
To change a record in the employee table, use the Update function. Where it determines whether or not the record is available. If it is possible, change the details and update the employee’s record in our simple employee management system project in python with source code.
To Promote Employees in python project on employee management system
# Function to Promote_Employ
def Promote_Employ():
print("{:>60}".format("-->> Promote Employee Record <<--\n"))
Id = input("Enter Employee Id: ")
# checking If Employee Id is Exit Or Not
if(check_employee(Id) == False):
print("Employee Record Not exists\nTry Again")
press = input("Press Any Key To Continue..")
menu()
else:
Amount = int(input("Enter Increase Salary: "))
#query to fetch salary of Employee with given data
sql = 'select Salary from empdata where Id=%s'
data = (Id,)
c = con.cursor()
#executing the sql query
c.execute(sql, data)
#fetching salary of Employee with given Id
r = c.fetchone()
t = r[0]+Amount
#query to update salary of Employee with given id
sql = 'update empdata set Salary = %s where Id = %s'
d = (t, Id)
#executing the sql query
c.execute(sql, d)
#commit() method to make changes in the table
con.commit()
print("Employee Promoted")
press = input("Press Any key To Continue..")
menu()
The Promote Employee function will request the employee’s id and then use the check employee function to determine whether or not the employee to be promoted already exists in our database. If this is the case, it will request the amount by which his compensation will be enhanced. After gathering the necessary information, it raises the salary of the employee with the supplied id by the specified amount.
The Promote Employee function will request the employee’s id and then use the check employee function to determine whether or not the employee to be promoted already exists in our database. If this is the case, it will request the amount by which his compensation will be enhanced. After gathering the necessary information, it raises the salary of the employee with the supplied id by the specified amount.
Remove Employee from employee management system in python with database
# Function to Remove_Employee
def Remove_Employ():
print("{:>60}".format("-->> Remove Employee Record <<--\n"))
Id = input("Enter Employee Id: ")
# checking If Employee Id is Exit Or Not
if(check_employee(Id) == False):
print("Employee Record Not exists\nTry Again")
press = input("Press Any Key To Continue..")
menu()
else:
#query to delete Employee from empdata table
sql = 'delete from empdata where Id = %s'
data = (Id,)
c = con.cursor()
#executing the sql query
c.execute(sql, data)
#commit() method to make changes in the empdata table
con.commit()
print("Employee Removed")
press = input("Press Any key To Continue..")
menu()
Because the primary key in our Employee Details Record is Id, the Remove Employee Function will simply request the employee’s Id to be destroyed. Two employees with the same name are permitted, but each must have a distinct id. The Remove Employee function checks to see if the employee to be eliminated is existing in our database using the check employee function. If they are, the Remove Employee function obtains a valid employee id and deletes the record associated with that id.
Search for Employee
# Function to Search_Employ
def Search_Employ():
print("{:>60}".format("-->> Search Employee Record <<--\n"))
Id = input("Enter Employee Id: ")
# checking If Employee Id is Exit Or Not
if(check_employee(Id) == False):
print("Employee Record Not exists\nTry Again")
press = input("Press Any Key To Continue..")
menu()
else:
#query to search Employee from empdata table
sql = 'select * from empdata where Id = %s'
data = (Id,)
c = con.cursor()
#executing the sql query
c.execute(sql, data)
#fetching all details of all the employee
r = c.fetchall()
for i in r:
print("Employee Id: ", i[0])
print("Employee Name: ", i[1])
print("Employee Email Id: ", i[2])
print("Employee Phone No.: ", i[3])
print("Employee Address: ", i[4])
print("Employee Post: ", i[5])
print("Employee Salary: ", i[6])
print("\n")
press = input("Press Any key To Continue..")
menu()
A more effective method of searching the record To find a specific record, we created a search function. It makes searching very simple. To accomplish this, we run the query, which searches the database table for the record.
Menu Function of employee management system using python
# Menu function to display menu
def menu():
system("cls")
print("{:>60}".format("************************************"))
print("{:>60}".format("-->> Employee Management System <<--"))
print("{:>60}".format("************************************"))
print("1. Add Employee")
print("2. Display Employee Record")
print("3. Update Employee Record")
print("4. Promote Employee Record")
print("5. Remove Employee Record")
print("6. Search Employee Record")
print("7. Exit\n")
print("{:>60}".format("-->> Choice Options: [1/2/3/4/5/6/7] <<--"))
ch = int(input("Enter your Choice: "))
if ch == 1:
system("cls")
Add_Employ()
elif ch == 2:
system("cls")
Display_Employ()
elif ch == 3:
system("cls")
Update_Employ()
elif ch == 4:
system("cls")
Promote_Employ()
elif ch == 5:
system("cls")
Remove_Employ()
elif ch == 6:
system("cls")
Search_Employ()
elif ch == 7:
system("cls")
print("{:>60}7".format("Have A NIce Day :)"))
exit(0)
else:
print("Invalid Choice!")
press = input("Press Any key To Continue..")
menu()
# Calling menu function
menu()
The Menu function displays a menu and prompts the user to choose an option for activities such as Add employee, Remove employee, and so on.
Complete Source Code for Employee Management System Project in Python
# Employee Management System Using Python – violet-cat-415996.hostingersite.com from os import system import re # importing mysql connector import mysql.connector # making Connection con = mysql.connector.connect( host="localhost", user="username", password="yourpassword") # preparing a cursor object cursorObject = con.cursor() # creating database cursorObject.execute("CREATE DATABASE employee") # make a regular expression # for validating an Email regex = r'\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Z|a-z]{2,}\b' # for validating an Phone Number Pattern = re.compile("(0|91)?[7-9][0-9]{9}") # Function to Add_Employ def Add_Employ(): print("{:>60}".format("-->>Add Employee Record<<--")) Id = input("Enter Employee Id: ") # checking If Employee Id is Exit Or Not if (check_employee(Id) == True): print("Employee ID Already Exists\nTry Again..") press = input("Press Any Key To Continue..") Add_Employ() Name = input("Enter Employee Name: ") # checking If Employee Name is Exit Or Not if (check_employee_name(Name) == True): print("Employee Name Already Exists\nTry Again..") press = input("Press Any Key To Continue..") Add_Employ Email_Id = input("Enter Employee Email ID: ") if(re.fullmatch(regex, Email_Id)): print("Valid Email") else: print("Invalid Email") press = input("Press Any Key To Continue..") Add_Employ() Phone_no = input("Enter Employee Phone No.: ") if(Pattern.match(Phone_no)): print("Valid Phone Number") else: print("Invalid Phone Number") press = input("Press Any Key To Continue..") Add_Employ() Address = input("Enter Employee Address: ") Post = input("Enter Employee Post: ") Salary = input("Enter Employee Salary: ") data = (Id, Name, Email_Id, Phone_no, Address, Post, Salary) # Instering Employee Details in # the Employee (empdata) Table sql = 'insert into empdata values(%s,%s,%s,%s,%s,%s,%s)' c = con.cursor() # Executing the sql Query c.execute(sql, data) # Commit() method to make changes in the table con.commit() print("Successfully Added Employee Record") press = input("Press Any Key To Continue..") menu() # Function To Check if Employee With # given Name Exist or not def check_employee_name(employee_name): # query to select all Rows from # employee(empdata) table sql = 'select * from empdata where Name=%s' # making cursor buffered to make # rowcount method work properly c = con.cursor(buffered=True) data = (employee_name,) # Execute the sql query c.execute(sql, data) # rowcount method to find number # of rowa with given values r = c.rowcount if r == 1: return True else: return False # Function To Check if Employee With # given Id Exist or not def check_employee(employee_id): # query to select all Rows from # employee(empdata) table sql = 'select * from empdata where Id=%s' # making cursor buffered to make # rowcount method work properly c = con.cursor(buffered=True) data = (employee_id,) # Execute the sql query c.execute(sql, data) # rowcount method to find number # of rowa with given values r = c.rowcount if r == 1: return True else: return False # Function to Display_Employ def Display_Employ(): print("{:>60}".format("-->> Display Employee Record <<--")) # query to select all rows from Employee (empdata) Table sql = 'select * from empdata' c = con.cursor() # Executing the sql query c.execute(sql) # Fetching all details of all the Employees r = c.fetchall() for i in r: print("Employee Id: ", i[0]) print("Employee Name: ", i[1]) print("Employee Email Id: ", i[2]) print("Employee Phone No.: ", i[3]) print("Employee Address: ", i[4]) print("Employee Post: ", i[5]) print("Employee Salary: ", i[6]) print("\n") press = input("Press Any key To Continue..") menu() # Function to Update_Employ def Update_Employ(): print("{:>60}".format("-->> Update Employee Record <<--\n")) Id = input("Enter Employee Id: ") # checking If Employee Id is Exit Or Not if(check_employee(Id) == False): print("Employee Record Not exists\nTry Again") press = input("Press Any Key To Continue..") menu() else: Email_Id = input("Enter Employee Email ID: ") if(re.fullmatch(regex, Email_Id)): print("Valid Email") else: print("Invalid Email") press = input("Press Any Key To Continue..") Update_Employ() Phone_no = input("Enter Employee Phone No.: ") if(Pattern.match(Phone_no)): print("Valid Phone Number") else: print("Invalid Phone Number") press = input("Press Any Key To Continue..") Update_Employ() Address = input("Enter Employee Address: ") # Updating Employee details in empdata Table sql = 'UPDATE empdata set Email_Id = %s, Phone_no = %s, Address = %s where Id = %s' data = (Email_Id, Phone_no, Address, Id) c = con.cursor() # Executing the sql query c.execute(sql, data) # commit() method to make changes in the table con.commit() print("Updated Employee Record") press = input("Press Any Key To Continue..") menu() # Function to Promote_Employ def Promote_Employ(): print("{:>60}".format("-->> Promote Employee Record <<--\n")) Id = input("Enter Employee Id: ") # checking If Employee Id is Exit Or Not if(check_employee(Id) == False): print("Employee Record Not exists\nTry Again") press = input("Press Any Key To Continue..") menu() else: Amount = int(input("Enter Increase Salary: ")) #query to fetch salary of Employee with given data sql = 'select Salary from empdata where Id=%s' data = (Id,) c = con.cursor() #executing the sql query c.execute(sql, data) #fetching salary of Employee with given Id r = c.fetchone() t = r[0]+Amount #query to update salary of Employee with given id sql = 'update empdata set Salary = %s where Id = %s' d = (t, Id) #executing the sql query c.execute(sql, d) #commit() method to make changes in the table con.commit() print("Employee Promoted") press = input("Press Any key To Continue..") menu() # Function to Remove_Employ def Remove_Employ(): print("{:>60}".format("-->> Remove Employee Record <<--\n")) Id = input("Enter Employee Id: ") # checking If Employee Id is Exit Or Not if(check_employee(Id) == False): print("Employee Record Not exists\nTry Again") press = input("Press Any Key To Continue..") menu() else: #query to delete Employee from empdata table sql = 'delete from empdata where Id = %s' data = (Id,) c = con.cursor() #executing the sql query c.execute(sql, data) #commit() method to make changes in the empdata table con.commit() print("Employee Removed") press = input("Press Any key To Continue..") menu() # Function to Search_Employ def Search_Employ(): print("{:>60}".format("-->> Search Employee Record <<--\n")) Id = input("Enter Employee Id: ") # checking If Employee Id is Exit Or Not if(check_employee(Id) == False): print("Employee Record Not exists\nTry Again") press = input("Press Any Key To Continue..") menu() else: #query to search Employee from empdata table sql = 'select * from empdata where Id = %s' data = (Id,) c = con.cursor() #executing the sql query c.execute(sql, data) #fetching all details of all the employee r = c.fetchall() for i in r: print("Employee Id: ", i[0]) print("Employee Name: ", i[1]) print("Employee Email Id: ", i[2]) print("Employee Phone No.: ", i[3]) print("Employee Address: ", i[4]) print("Employee Post: ", i[5]) print("Employee Salary: ", i[6]) print("\n") press = input("Press Any key To Continue..") menu() # Menu function to display menu def menu(): system("cls") print("{:>60}".format("************************************")) print("{:>60}".format("-->> Employee Management System <<--")) print("{:>60}".format("************************************")) print("1. Add Employee") print("2. Display Employee Record") print("3. Update Employee Record") print("4. Promote Employee Record") print("5. Remove Employee Record") print("6. Search Employee Record") print("7. Exit\n") print("{:>60}".format("-->> Choice Options: [1/2/3/4/5/6/7] <<--")) ch = int(input("Enter your Choice: ")) if ch == 1: system("cls") Add_Employ() elif ch == 2: system("cls") Display_Employ() elif ch == 3: system("cls") Update_Employ() elif ch == 4: system("cls") Promote_Employ() elif ch == 5: system("cls") Remove_Employ() elif ch == 6: system("cls") Search_Employ() elif ch == 7: system("cls") print("{:>60}7".format("Have A NIce Day :)")) exit(0) else: print("Invalid Choice!") press = input("Press Any key To Continue..") menu() # Calling menu function menu()
Output for Employee Management System Project in Python
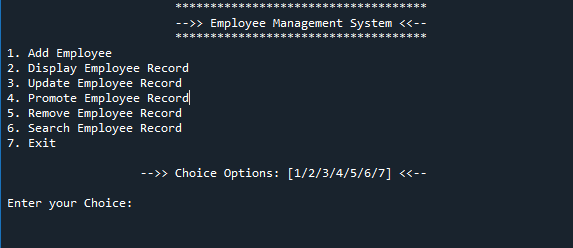
Employee Management System Project in Python is a distributed application designed to keep track of all employees in any firm. It stores information about their employees’ personal information.
Thank you for visiting our website.
Also Read:
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Music Recommendation System in Machine Learning
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++
- Python Download File from URL | 4 Methods
- Python Programming Examples | Fundamental Programs in Python
- Spell Checker in Python
- Portfolio Management System in Python
- Stickman Game in Python
- Contact Book project in Python
- Loan Management System Project in Python
- Cab Booking System in Python
- Brick Breaker Game in Python
- 100+ Java Projects for Beginners 2023
- Tank game in Python
- GUI Piano in Python
- Ludo Game in Python
- Rock Paper Scissors Game in Python
- Snake and Ladder Game in Python
- Puzzle Game in Python
- Medical Store Management System Project in Python
- Creating Dino Game in Python
- Tic Tac Toe Game in Python
- Courier Tracking System in HTML CSS and JS