
Introduction to TypeError in Python
In this tutorial on TypeError: can only concatenate str(not “int”) to str we are going to learn what is TypeError. Why does this TypeError occur and how we can resolve the issue of the TypeError can only concatenate str(not “int”)to str with examples.
Many new python beginners, come across this kind of TypeError but don’t worry we will resolve it and understand the solution.
Try Short Video Solution
Let’s understand this in detail
What is the TypeError?
The TypeError occurs whenever an operation is performed on an incorrect object or unsupported object type. This issue will appear when you try to concatenate a string with an integer.
Let’s see an example of the addition of two variables
variable1="hello"
variable2= 2
print(variable1+variable2)
In this example, we have added two variables where variable1 is having the value of string type and variable2 is having the value of integer type. But we know the string and the integer cannot be added together. The only solution for this is to concatenate the operands of having the same data type.
Output

Let’s see another example of TypeError
String_value = "Welcome"
int_value = 50
print(String_value + int_value)
Output

In this example, we can clearly see that the string value and the integer value cannot be concatenated.
What is TypeError: can only concatenate str (not “int”) to str?
Python does not allow concatenating values of different types while other programming language such as javascript supports type coercion which automatically(*) converts values like integers and float so that they can be concatenated.
In Python, we cannot concatenate a string and an integer, string and a list, etc.
Example Scenario
Let us take a simple example to look at this issue.
products = {
"product": "Redmi",
"cost": 1700,
"stock": 12
}
print("We have total " + products["stock"] + " quantities of Product " + products["product"])
Output

Explanation:
In the above example we are getting the error as TypeError: can only concatenate str(not “int”) to str as we are trying to concatenate the string and the integer value together in the print statement.
How to fix TypeError: can only concatenate str(not “int”) to str?
In the Python programming language, before performing the concatenation of the two operands we should make sure that they should be of the same type.
In the above dictionary example, the products[stock] is of type integer and we are concatenating the integer value with strings in the print statement which causes the TypeError: can only concatenate str (not “int”) to str.
We can provide solutions to this problem in different ways
Method1 using str()
The solution to this problem is very simple. As we know that string and integer cannot be concatenated we have to convert the integer value into the string format, before we concatenate and print the result.
Code after we convert the products[stock] into a string
products = {
"product": "Redmi",
"cost": 1700,
"stock": 12
}
print("We are having total " + str(products["stock"])+ " quantities of Product " + products["product"])
Output
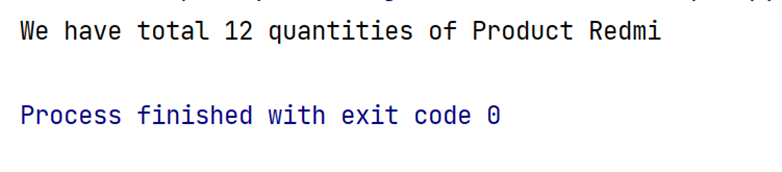
As we can see, after converting the product[stock] into string format we are getting the desired result.
Method2 using .fstring {} in the print statement
print(f"We are having total {(products['stock'])} quantities of Product {products['product']}")
Method3 using the %s symbol in the print statement
print("We are having total " '%s' %(products['stock']), " quantities of products " '%s' %(products['product']))
Method4 using the . format in the print statement
print("We are having total " '{}' .format(products['stock']), " quantities of products " '{}' .format(products['product']))
Output

In these ways, by using different methods we can convert integer format into string format and display the output without any TypeError.
Summary
The TypeError: can only concatenate str(not “int”) to str usually occurs if we are trying to concatenate an integer with a string. Python does not allow concatenating values of different data types. We can resolve the issue by converting the integer values to strings before concatenating them.
Thank you for visiting our website.
Also Read:
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- What is web development for beginners?
- Microsoft Giving Free Machine Learning Course: Enroll Now
- Accenture Giving Free Developer Certificate in 2023
- Python | Asking the user for input until they give a valid response
- Amazon Summer Internship 2023
- Amazon Giving Free Machine Learning Course with Certificate: Enroll Now
- Google Summer Internship 2023
- 5 Secret ChatGPT skills to make money
- Free Google Certification Courses
- 5 AI tools for coders better than ChatGPT
- New secrets to Earn money with Python in 2023
- How to utilize ChatGPT to improve your coding skills?
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Radha Krishna using Python Turtle
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++