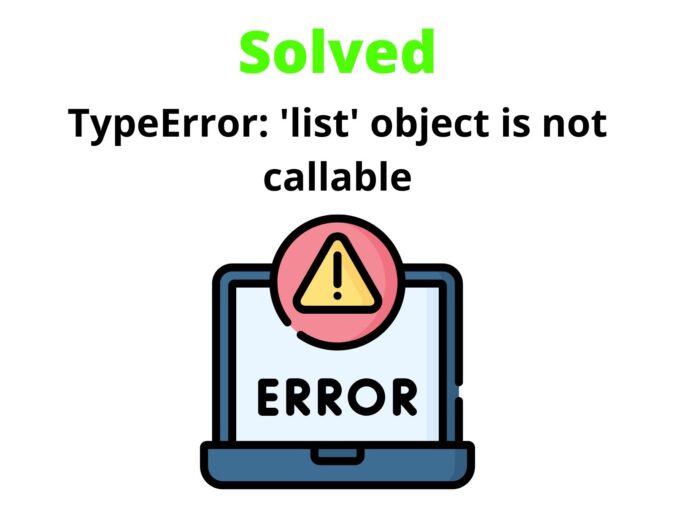
Introduction
The type error is an exception that is raised when we try to perform the operation on an inappropriate object type. The error occurs by the python interpreter if the data types of various different objects in an operation are not compatible with each other. In this Python Tutorial, we will be discussing the TypeError: ‘list’ object is not callable, how to fix TypeError in python along with the examples and proper solution for it.
Try Short Video Solution
What does “TypeError: ‘list’ object is not callable” mean?
There may be any number of ways that may result in the occurrence of this error, we are going to discuss the two most common ways which most Python programmers do:
- Re-assign the built-in name i.e list
- Using Parenthesis for List Indexing
1. Re-assign the built-in name i.e list
This type of error usually occurs as we try to re-assign the built-in name i.e list. We try to overwrite the predefined meaning of the built-in name. So this reassigning of the built-in name causes the predefined value to lose its original function that works as a class object of the Python List.
Example:
list = [1, 2, 3, 4, 5]
myrange = list(range(1 , 10))
print(myrange)
for number in list:
if number in myrange:
print(number, 'is between 1 and 10)
Explanation:
- Here we defined a list whose variable name is “list”.
- We have defined another variable myrange that stores the value in the form of a list.
- Defined the for loop for each number in a list, it will print the number if it’s in the range of the list.
Error Output:

Here we are getting the error as TypeError: ‘list’ object is not callable so the main reason in such case is we are using “list” as the variable name which is a built-in object having a predefined meaning in the python programming language. Most programmers make a common mistake of using the built-in methods or functions as the variable names leading to an error. While this can be either a method or the object of the class.
How to fix this error?
We should make sure that the variable names should not the same as the built-in or predefined names.
Let us change the variable name from “list” to “value”.
values = [1, 2, 3, 4, 5]
myrange = list(range(1, 10))
print(myrange)
for number in values:
if number in myrange:
print(number, 'is between 1 and 10')
Resolved Output:

2. Using Parenthesis for List Indexing
Correct Syntax to get a list value from a list is using square brackets with its index i.e. list_name[index], but sometimes Python programmers use parenthesis instead of square brackets i.e. list_name(index).
Example:
stationary=["pen","pencil","rubber","sharpner"]
for i in range(len(stationary)):
stationary[i] = stationary(i).lower()
print(stationary[i])
print(stationary)
Explanation:
- We have defined the variable “stationary” which consists of 4 items in the list form.
- Defined the for loop that takes the length of the stationary using len() function.
- The stationary variable takes the stationary items one by one and prints them.
Error Output:
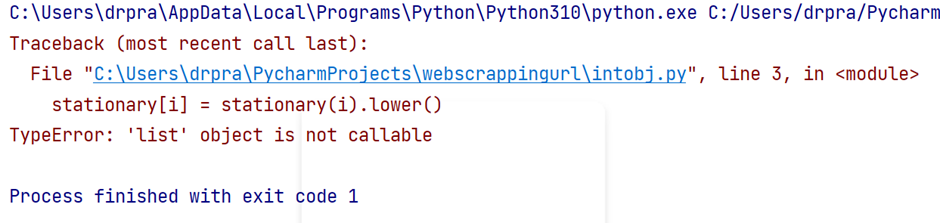
Explanation:
Here we can see another scenario where we get the TypeError: ‘list’ object is not callable. We raise the error because we used parentheses to access the items in the list i.e. stationary(i).lower(). In the Python programming language, we use parentheses to call functions. In this case, we couldn’t able to call the list as we made use of parenthesis instead of square brackets.
How to fix this error?
We should make sure that while calling the list object we should use the square brackets instead of the parenthesis.
stationary=["pen","pencil","rubber","sharpner"]
for i in range(len(stationary)):
stationary[i] = stationary[i].lower()
print(stationary[i])
print(stationary)
Resolved Output:
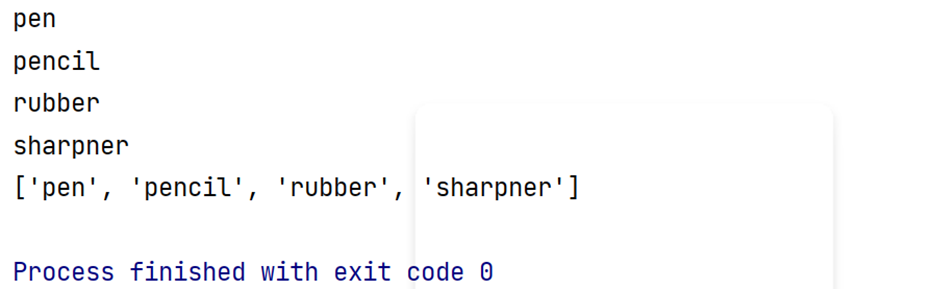
Summary
In this article, we come to know why the TypeError: ‘list’ Object Is Not Callable raises and how to resolve this error which occurs because of some common fundamental flaws in the code. Hence, try not to use “list” as the variable name having the predefined meaning to avoid getting this error.
Thank you for visiting our website.
Also Read:
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- What is web development for beginners?
- Microsoft Giving Free Machine Learning Course: Enroll Now
- Accenture Giving Free Developer Certificate in 2023
- Python | Asking the user for input until they give a valid response
- Amazon Summer Internship 2023
- Amazon Giving Free Machine Learning Course with Certificate: Enroll Now
- Google Summer Internship 2023
- 5 Secret ChatGPT skills to make money
- Free Google Certification Courses
- 5 AI tools for coders better than ChatGPT
- New secrets to Earn money with Python in 2023
- How to utilize ChatGPT to improve your coding skills?
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Radha Krishna using Python Turtle
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++