
In this tutorial, we will be discussing why UnboundLocalError: local variable referenced before assignment occurs. When we used functions in our code we come across this type of error as we try to use a local variable referenced before the assignment. Here we will try to understand what this error means, why it is raised and how it can be resolved with the help of examples.
What is UnboundLocalError: local variable referenced before assignment?
In python, the scope of the variable is determined depending on whether the variable is defined inside the function or outside. If the variable is declared inside the function then its scope is within the function itself and we can access it inside the function only.
The error UnboundLocalError: local variable referenced before assignment occurs because we try to assign a value to the variable that does not have a local scope. As the global variable has a global scope the user tries to access to use the variable within the function.
There are two types of variables local variables and global variables.
Difference between local variables and global variables
Local Variables | Global Variables |
local variable can be defined inside the only function. | The global variable can be declared both inside and outside the function |
It can be accessed within the function itself. | It can be accessed from both inside and outside the function or anywhere within the program. |
No need to use keywords to declare it is considered local by default. | The global keyword is used to declare a global variable. |
Example:
The below example code demonstrates the code where the program will end up with the “local variable referenced before assignment” error.
num= 5
def calculate():
num = num + 1
print(num)
calculate()
Explanation:
- We have declared the variable num =5.
- Defined a function calculate() in which we have increased the num variable by 1 and stored it in the variable itself.
- Printed the num value.
- Called the function to calculate.
Error Output:
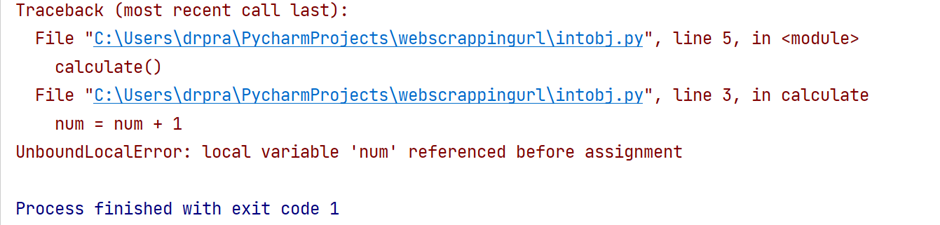
Explanation:
What went wrong here? We have declared the num variable outside the function while the function cannot access it until you declared it global using the global keyword.
Solution
Solution with the global keyword
num=5
def calculate():
global num
num = num + 1
print(num)
calculate()
Here we initialized the num variable with the global keyword so that it can be accessed inside and outside the function.
Resolved Output:

Solution with passing parameter
Here we can pass the parameter to the function so there is no need of declaring the variable as local or global. So the need for variable declaration is resolved.
def calculate(num):
num = num + 1
print(num)
calculate(5)
Resolved Output:

Summary
Hence, in this article, we came to know the reasons for the error “UnboundLocalError: local variable referenced before assignment”. We have understood how the use of global keywords and passing the parameter solved this type of error.
For more articles on python keep visiting our website violet-cat-415996.hostingersite.com
Thank you for visiting our website.
Also Read:
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- What is web development for beginners?
- Microsoft Giving Free Machine Learning Course: Enroll Now
- Accenture Giving Free Developer Certificate in 2023
- Python | Asking the user for input until they give a valid response
- Amazon Summer Internship 2023
- Amazon Giving Free Machine Learning Course with Certificate: Enroll Now
- Google Summer Internship 2023
- 5 Secret ChatGPT skills to make money
- Free Google Certification Courses
- 5 AI tools for coders better than ChatGPT
- New secrets to Earn money with Python in 2023
- How to utilize ChatGPT to improve your coding skills?
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Radha Krishna using Python Turtle
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++