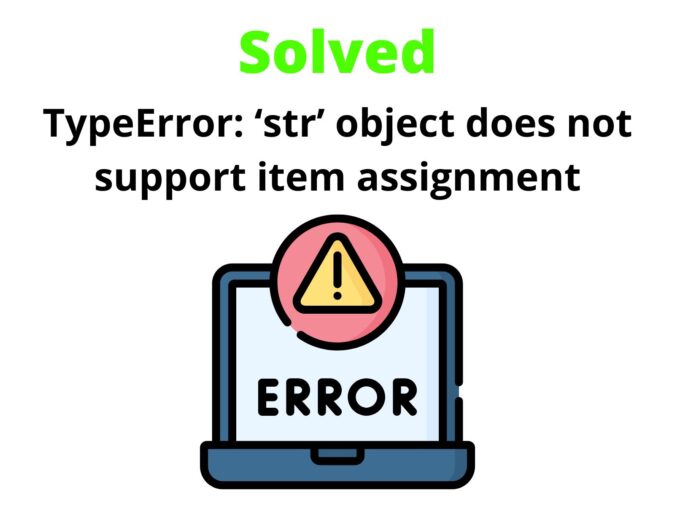
Introduction
Today, we will see what is “TypeError: ‘str’ object does not support item assignment” and will also learn how to solve it. We will see the solution to this error using 5 examples.
Being an interpreted language, the Python interpreter checks the code line by line and throws any error occurring within the line. This is unlike the compiler as the compiler displays all errors and warnings altogether.
There are mainly 3 types of can be happened errors in Python:
- Syntax Errors: The syntax of the code might not be as it should be. Eg. Missing colon or brackets.
- Exceptions: It throws when the interpreter cannot execute the requested action or line of code.
- Logical Errors: These can be human errors i.e. the output is not as expected
To the scope of this article, we will study about TypeError which is an exception in Python.
TypeError
- TypeError is an exceptional error i.e. the interpreter cannot execute the requested action or line of code.
- Generally, TypeError occurs when the data type of an object in an operation or action is inappropriate. In other words, if the datatype is wrong. The object you are operating with is out of its scope to operate over the code.
- A simpler example could be if the code states to subtract an integer from a string.
Let’s understand this TypeError with examples
Example 1
my_string = "copyassignment"
my_integer = 5
print(my_string - my_integer)
Output:
Traceback (most recent call last): File "", line 4, in TypeError: unsupported operand type(s) for -: 'str' and 'int'
Explanation:
However, it’s totally different scope if you are trying to multiply or add a string with an integer because it is basically one of the properties of the data type string.
Now, as the TypeError is clear, we can really understand what does, TypeError: ‘str’ object does not support item assignment means.
Now, it has been crystal clear that this throw of an exception is due to the invalid datatype for an operation.
Exactly, the invalid datatype is ‘string’ here and the operation that has been executed is ‘item assignment’. Now, item assignment here means, assigning an item to the existing item, moreover ‘updating’ the item. On the code view, how does this error occur? Here’s how:
Example 2
my_string = "mango"
my_string[0] = 'f'
print(my_string)
Output:
#Output Traceback (most recent call last): File "<string>", line 2, in <module> TypeError: 'str' object does not support item assignment
Explanation:
The error occurred because, in Python, strings are immutable. Immutable objects are those objects which cannot be modified/updated/changed or even deleted, once created.
Every variable in Python is an object of the class of its datatype. A string variable is an instance of class ‘str’. And the elements or characters in it can’t be altered once they are defined. Another data type that is immutable in Python is a tuple and hence, doesn’t support item assignment.
And hence the TypeError: ‘str’ object does not support item assignment.
Example 3
So, in c or c++ or any other programming language: (For eg. C++):
string my_string = "mango";
my_string[1]='f';
cout<<my_string
Output:
fango
But in Python, it won’t work due to the property of immutability of the string class.
Solution to “TypeError: ‘str’ object does not support item assignment“
One way to fix the error could be by creating a new string with the required change.
Example 1
my_string = "mango"
new_string = "fango"
print(my_string)
print(new_string)
Output:
mango
fango
Example 2
The above code can be also written specifically for the index as-
my_string = "mango"
new_string = ""
for i in range(len(my_string)):
if(i==0):
new_string+='f'
else:
new_string+=my_string[i]
print(new_string)
#output
fango
Hence, it is recommended to use mutable data types like lists, sets, or dictionaries if there’s a need to alter the elements. But if it is strict or must that the elements should not be changed, immutable datatypes are practiced in Python.
Thank you for visiting our website.
Also Read:
- Free Python Certification course from Alison: Good for Resume
- Download 1000+ Projects, All B.Tech & Programming Notes, Job, Resume & Interview Guide, and More – Get Your Ultimate Programming Bundle!
- Top 5 Websites to Learn Programming in 2024
- Python Internship for college students and freshers: Apply Here
- New secrets to Earn money with Python in 2024
- Connect with HR Directly – Job Hack
- Google offering free Python course: Enroll Today
- Google Internship 2024
- TCS Launched Free Certification Course with Industry Recognized Value
- What is web development for beginners?
- Microsoft Giving Free Machine Learning Course: Enroll Now
- Accenture Giving Free Developer Certificate in 2023
- Python | Asking the user for input until they give a valid response
- Amazon Summer Internship 2023
- Amazon Giving Free Machine Learning Course with Certificate: Enroll Now
- Google Summer Internship 2023
- 5 Secret ChatGPT skills to make money
- Free Google Certification Courses
- 5 AI tools for coders better than ChatGPT
- New secrets to Earn money with Python in 2023
- How to utilize ChatGPT to improve your coding skills?
- Create your own ChatGPT with Python
- Bakery Management System in Python | Class 12 Project
- SQLite | CRUD Operations in Python
- Event Management System Project in Python
- Ticket Booking and Management in Python
- Radha Krishna using Python Turtle
- Hostel Management System Project in Python
- Sales Management System Project in Python
- Bank Management System Project in C++