
In this article, we are going to discuss how we can really run our machine learning in Visual Studio Code. Generally, most machine learning projects are developed as ‘.ipynb’ in Jupyter notebook or Google Collaboratory. However, Visual Studio Code is powerful among programming code editors, and also possesses the facility to run ML or Data Science codes.
The VS Code’s marketplace is full of extensions for basically all programming purposes, whether for auto-completing code snippets or enhancing the readability of the code, it contains a variety of options or services as extensions.
Setup Machine Learning in Visual Studio Code
1. Download and Install Python
The major thing you will need is Python installed in your system. Python is an open-source programming language and hence we can see newer versions of it frequently. However, it’s total up to your will if you want to update the existing version of Python or not. But Python 3.x can be most suitable.
To install Python in your system, type in ‘install python’ in your search bar, open the first official website and you will be redirected to the https://www.python.org/downloads/
Download the executable according to your OS.
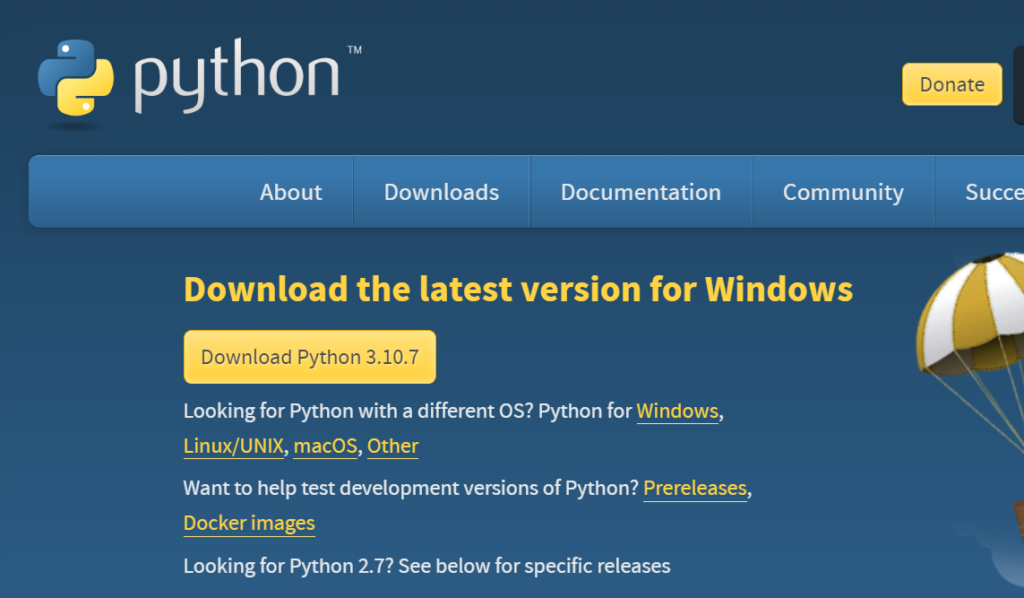
The latest version of Python available right now is 3.10 & Python 3.11 is in the process to release.
Once, the executable is installed, run it, and allow it to run as administrator.
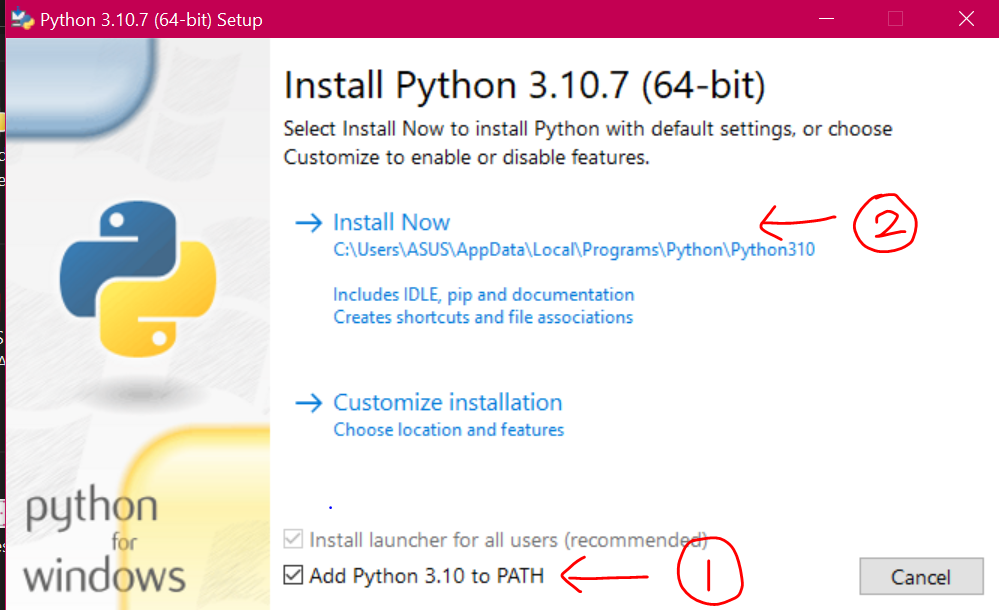
Make sure you are checking this ‘Add Python to PATH’ box because this reduces your work of adding the Python executable manually inside the System’s Environment variables.
By default, Python installs in the C directory under the Programs folder. If you want to change this location, then you can go for the ‘Customize Installation’ option available.
2. Download and Install VS Code
The next and again major tool is, of course, the Visual Studio Code itself. Again you can type in ‘Visual Studio Code and you will see the official website i.e. https://code.visualstudio.com/download
Select the download option according to your OS type.
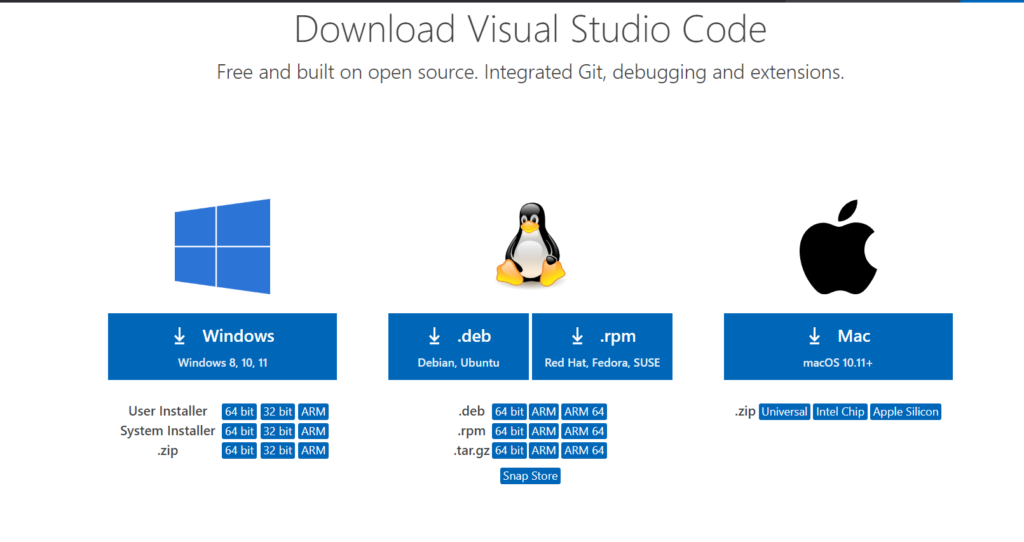
You can download the system installer in the case of Windows.
Run the executable.
- Accept the agreement.
- Select the folder by clicking on ‘Browse’, if you don’t wish to install it in the default folder mentioned there.

3. The next option contains, how you wish to specify the Visual Studio Code at the start menu. This is totally Nomenclature part and you can name it up to your will. By default, it is “Visual Studio Code”. If you don’t wish to create a start menu folder, you can check the box at the bottom.
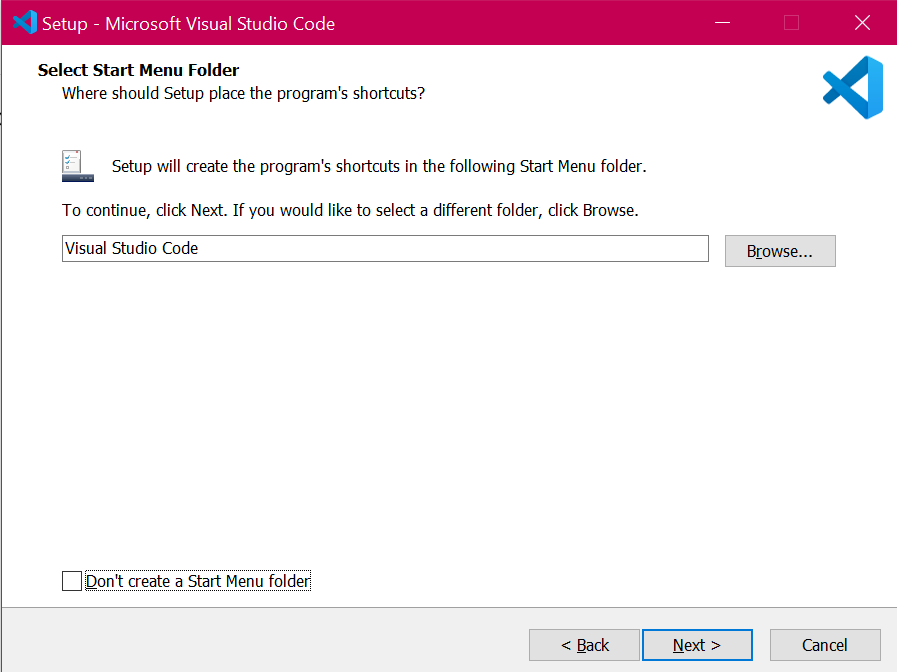
4. Generally it is preferred to check every box of this next options menu.
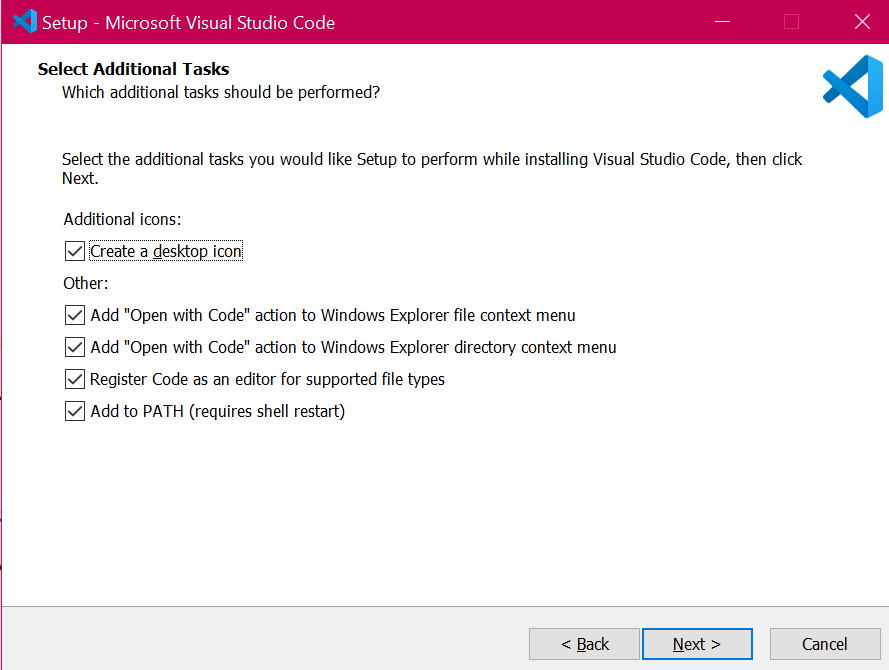
Because the Open with Code is a lot easier. You don’t need to import the files manually inside the VS Code. Open with Code opens all the files under a folder inside a new window of the editor itself. The desktop icon is completely optional. It is checked for the sake of easy accessibility. The last two boxes are by default checked and it is recommended to keep them as it is to reduce the additional work.
4. This is what you have selected, you can verify and Run the Install command.

Now that, VS Code is installed, open it, accept all the agreements, and get started.
3. Setup VS Code extensions

On the left-hand side, you will see this icon. Among them, the second last is for Extensions. Click on it and a search bar will appear, type in ‘Python’. Extension of Python is must for ML in Visual Studio Code.
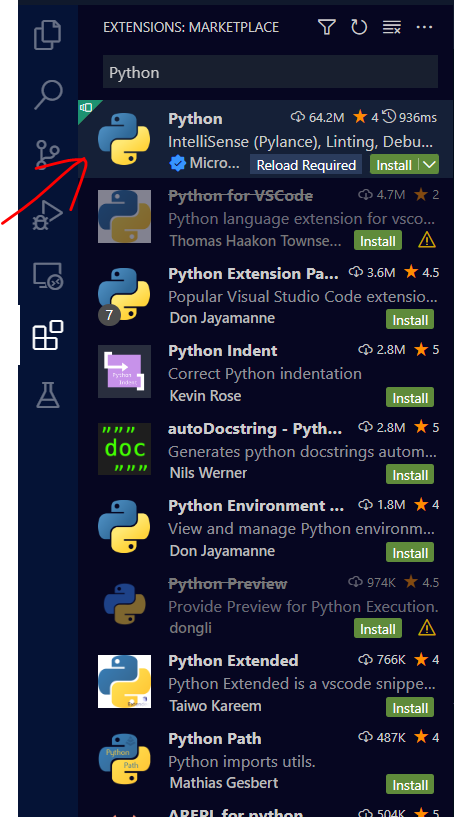
You’ll see a variety of different extensions, among them, select the first one and click on the ‘Install’ option you can see on the green button.

These are the extension details. You can see it is in the Installation stage currently. You can read the details about it.

The extension has been installed.
Now, there are 2 ways to write ML in Visual Studio Code:
- You can create a virtual environment, where all the required modules need to be downloaded from with pip through the command line.
- If you have anaconda or miniconda installed in VS Code, you can directly create a file and start writing the code.
4. Settingup Anaconda
To install anaconda, install it from https://www.anaconda.com/products/distribution according to your OS type. Anaconda is required for all ML packages as these packages are required to run ML in Visual Studio Code. Now, let’s see the steps to setup anaconda in our system:-
1. Run the executable file
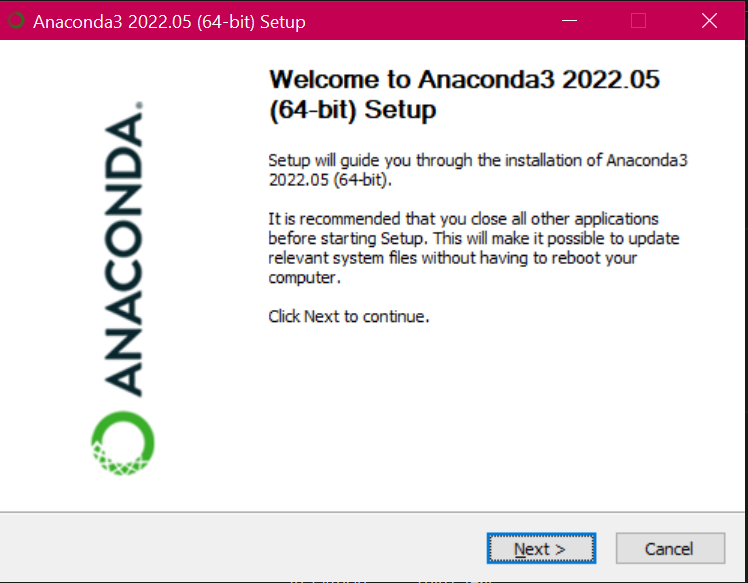
2. Agree to the agreement.

3. Select privileges according to accessibility.

4. Choose the location, recommended is to keep the default one.
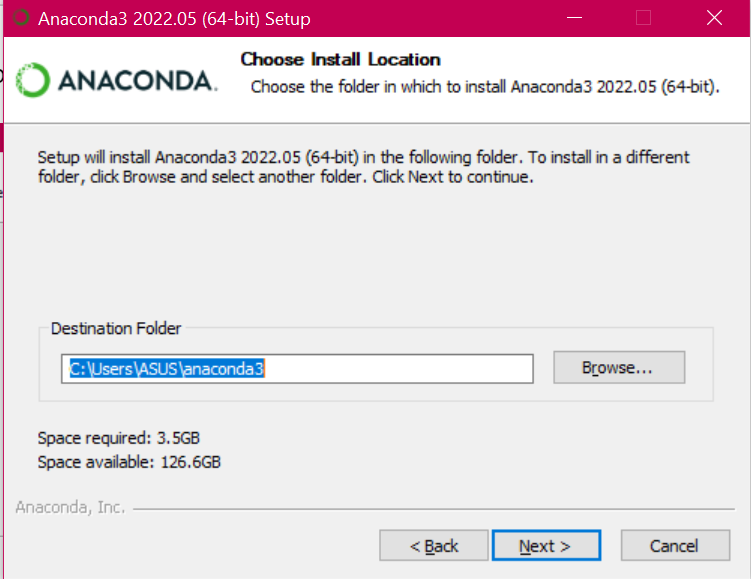
5. I prefer adding the anaconda to the Path, Also you have to check option 2 for the VS code run.

6. Click on install.
This part was the installation of an anaconda.
And this anaconda install will help us create a virtual environment for our ML in Visual Studio Code.
5. Creating Virtual Environment for Machine Learning Projects
Step 1: Open the anaconda command prompt.
You can search for it from the start menu in case it is not available on the desktop.
Step 2: Into the cmd, type in:
conda create -n myenv python=3.9 pandas jupyter seaborn scikit-learn keras tensorflow
Press Enter.
The command will complete its function.
Within seconds, you will see a question popping on the screen stating if the process can continue, type ‘y’ in there.
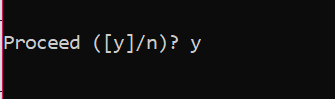
Press Enter.
The extraction and download of the packages will now start.
All these packages are being downloaded for Jupyter hence there will be no need to download any of the packages separately.
This process takes minutes to finish.
Step 3: Create a folder for your project at the desired location.
Name it.
Step 4: Open this folder with VS Code.
Go inside the folder and right-click to open the options menu. Select ‘Open with Code’.

Step 5: Creating New File.
As you stepped inside the VS Code, click ‘New File’ and create a new notebook with the .ipynb extension or you can open the command palette or Ctrl+Shift+P and select ‘Jupyter: Create New Jupyter Notebook’.
Rename and save the file.


Hurray! All setup, now you can see the interactive jupyter window there.
Step 6: Select Kernel
By default, your Python interpreter would be selected, but we need to change it to the anaconda environment we created earlier.


Note: I recommend you to run a basic python command right after you selected the kernel because there might be some chances that something called win32api will become an obstruction in between.
Just in case this happens, try reinstalling it, if you are dealing with the anaconda environment just like above, try to run this command
conda install -n <env name> pywin32 --force-reinstall
And in the case of Python, you can use:
python -m pip install pywin32
Running Machine Learning in Visual Studio Code:
We are plotting graphs for Iris Dataset.
Iris Dataset is one of the most common datasets in machine learning.
You can download the dataset from here.
1. Let’s import and check the dataset.
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
data = pd.read_csv("Iris.csv")
print (data.head(10))
2. Click Ctrl+Enter to run the cell.

The dataset consists of Sepal and Petal lengths, widths, and respectively.
import pandas as pd
import matplotlib.pyplot as plt
iris = pd.read_csv("Iris.csv")
plt.plot(iris.Id, iris["SepalLengthCm"], "r--")
plt.show
The graph is of SepalLength.
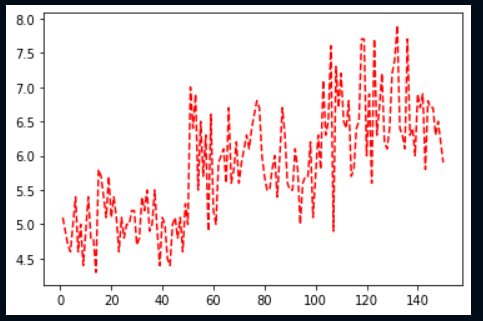
Can you see how effortlessly that code runs? This is not Jupyter but your VS Code.
3. Now, we’ll see a scatter plot
iris.plot(kind ="scatter",
x ='SepalLengthCm',
y ='PetalLengthCm')
plt.grid()
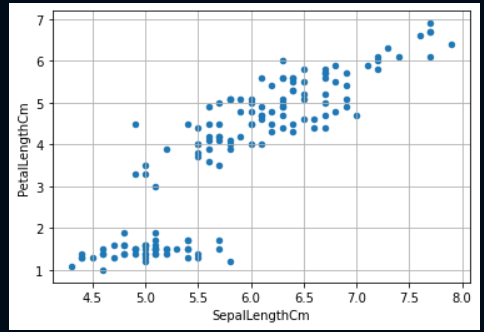
4. Lastly, we’ll try to implement Seaborn here.
import seaborn as sns
iris = sns.load_dataset('iris')
sns.set_style("whitegrid")
sns.FacetGrid(iris, hue ="species",
height = 6).map(plt.scatter,
'sepal_length',
'petal_length').add_legend()
If seaborn is not installed in your system, install it using
pip install seaborn

That’s it.
Now you can run your ML in Visual Studio Code.
Thank-you.
Also Read:
- Flower classification using CNN
- Music Recommendation System in Machine Learning
- Top 15 Machine Learning Projects in Python with source code
- Gender Recognition by Voice using Python
- Top 15 Python Libraries For Data Science in 2022
- Top 15 Python Libraries For Machine Learning in 2022
- Setup and Run Machine Learning in Visual Studio Code
- Diabetes prediction using Machine Learning
- 15 Deep Learning Projects for Final year
- Machine Learning Scenario-Based Questions
- Customer Behaviour Analysis – Machine Learning and Python
- NxNxN Matrix in Python 3
- 3 V’s of Big data
- Naive Bayes in Machine Learning
- Automate Data Mining With Python
- Support Vector Machine(SVM) in Machine Learning
- Convert ipynb to Python
- Data Science Projects for Final Year
- Multiclass Classification in Machine Learning
- Movie Recommendation System: with Streamlit and Python-ML
- Getting Started with Seaborn: Install, Import, and Usage
- List of Machine Learning Algorithms
- Recommendation engine in Machine Learning
- Machine Learning Projects for Final Year
- ML Systems
- Python Derivative Calculator
- Mathematics for Machine Learning
- Data Science Homework Help – Get The Assistance You Need
- How to Ace Your Machine Learning Assignment – A Guide for Beginners
- Top 10 Resources to Find Machine Learning Datasets in 2022