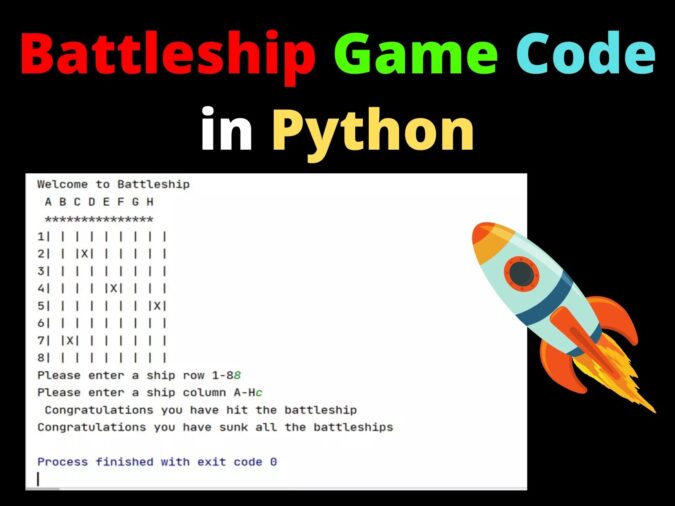
Introduction
Hello friends, in this tutorial we are going to create a Battleship Game Code in Python. This project is not beginner friendly for python learners who just started learning python. But as we are providing the comments and a detailed explanation of the code you may find it easy to understand the python code. So watch the tutorial till the end.
This game is the single-player battleship code written in Python programming language. In this source code of the battleship game in python, we have made used grids. This grid consists of 7 rows and 7 columns of the battleship where you can destroy and sink the ships.
This battleship game written in python is really interesting and challenging. The python learners must try this and get the code executed on the online compiler or you can execute it on the Pycharm IDE terminal which I have used.
You can click here to copy code directly for the battleship game in python.
Let’s begin to understand the code for the python battleship game in detail
Import the module
#Import randint function from random module
from random import randint
The random module is used in which we are importing the randint function which randomly selects the values from the given range.
Defining the patterns
Hidden_Pattern=[[' ']*8 for x in range(8)]
Guess_Pattern=[[' ']*8 for x in range(8)]
let_to_num={'A':0,'B':1, 'C':2,'D':3,'E':4,'F':5,'G':6,'H':7}
In this block of code, we are defining two patterns of size 8 each one pattern that is hidden and another one is the guessed pattern.
The letter to numbers is the dictionary which is enclosed in curly braces which is the form of key-value pair.
Function for printing the board
#Function is defined to print the board of battleship
def print_board(board):
print(' A B C D E F G H')
print(' ***************')
row_num=1
for row in board:
print("%d|%s|" % (row_num, "|".join(row)))
row_num +=1
Here in this piece of code, we are creating a function for printing the board for the battleship game where we are providing 2 print functions for printing the column from A – H and printing the row number and “|” symbol for each row on the board.
Function for getting the ship location for hitting the ship in the Battleship Game Code in Python
def get_ship_location():
#Enter the row number between 1 to 8
row=input('Please enter a ship row 1-8').upper()
while row not in '12345678':
print("Please enter a valid row")
row=input('Please enter a ship row 1-8')
#Enter the Ship column from A TO H
column=input('Please enter a ship column A-H').upper()
while column not in 'ABCDEFGH':
print("Please enter a valid column")
column=input('Please enter a ship column A-H')
return int(row)-1,let_to_num[column]
In this code block, we are defining the function of the ship’s location. Here we have defined the row and column variable to take the values of the row and the column where we have defined the while loops. In the first while loop, while the row values are not in the range of 1 to 8, It will ask for a valid row number. Similarly with the column. While the value of the column is not in the range of A – H. It will ask to enter a valid column. The return function returns the row less by 1 than the value provided and let_to_num[column].
The function defined for creating the ships
#Function that creates the ships
def create_ships(board):
for ship in range(5):
ship_r, ship_cl=randint(0,7), randint(0,7)
while board[ship_r][ship_cl] =='X':
ship_r, ship_cl = randint(0, 7), randint(0, 7)
board[ship_r][ship_cl] = 'X'
Here we have defined the function create ships. The for loop function creates 5 ships where the ship_r is the shipping row and ship_cl is the ship column, the randint(0,7) function randomly assigns the values to the ship’s row and column. In the while loop, the function continues until the boards ship_r and ship_cl == ‘X’, The ship row and ship column values get the random values. The board will get the values ‘X’ once the hidden pattern and guess pattern hits the same ship.
Function for counting the hit ships
def count_hit_ships(board):
count=0
for row in board:
for column in row:
if column=='X':
count+=1
return count
In this piece of code, we have defined a function count_hit_ships. The count is assigned to 0. Here for each row on the board and each column in the row if the column ==’X’ the count increases by 1 and returns the count. This function counts the number of ships hit and sunk.
Code for running the battleship code in python
create_ships(Hidden_Pattern)
#print_board(Hidden_Pattern)
turns = 10
while turns > 0:
print('Welcome to Battleship')
print_board(Guess_Pattern)
row,column =get_ship_location()
if Guess_Pattern[row][column] == '-':
print(' You already guessed that')
elif Hidden_Pattern[row][column] =='X':
print(' Congratulations you have hit the battleship')
Guess_Pattern[row][column] = 'X'
turns -= 1
else:
print('Sorry,You missed')
Guess_Pattern[row][column] = '-'
turns -= 1
if count_hit_ships(Guess_Pattern) == 5:
print("Congratulations you have sunk all the battleships")
break
print(' You have ' +str(turns) + 'turns remaining')
if turns == 0:
print('Game Over')
break
In this code block, we are calling the function create_ship that creates the ship. The turns variable is assigned to 10. While the turns > 0 The user can continue to hit the ships. The print_board (Guess_Pattern) creates the guess_pattern board. The row and column values get the values for the location of the ships.
Here if the guess_pattern == ‘-‘ then it prints “You have already guessed”. If the hidden patterns Hidden_Pattern[row][column] == ‘X’: then it prints ‘ Congratulations you have hit the battleship’ the turns count is decreased by 1 else it prints “’Sorry, You missed‘ . If the count_hit_ships and the guess_pattern are exactly same i.e 5 then it will print “Congratulations you have sunk all the battleships” Once the turns value becomes zero the program will print “ Game over”.
Complete source code for Battleship Game Code in Python
#Random module for randomly accepting the values # ‘X’ indicates the ships hit # ‘-‘ indicates the hits missed from random import randint Hidden_Pattern=[[' ']*8 for x in range(8)] Guess_Pattern=[[' ']*8 for x in range(8)] let_to_num={'A':0,'B':1, 'C':2,'D':3,'E':4,'F':5,'G':6,'H':7} def print_board(board): print(' A B C D E F G H') print(' ***************') row_num=1 for row in board: print("%d|%s|" % (row_num, "|".join(row))) row_num +=1 def get_ship_location(): #Enter the row number between 1 to 8 row=input('Please enter a ship row 1-8 ').upper() while row not in '12345678': print("Please enter a valid row ") row=input('Please enter a ship row 1-8 ') #Enter the Ship column from A TO H column=input('Please enter a ship column A-H ').upper() while column not in 'ABCDEFGH': print("Please enter a valid column ") column=input('Please enter a ship column A-H ') return int(row)-1,let_to_num[column] #Function that creates the ships def create_ships(board): for ship in range(5): ship_r, ship_cl=randint(0,7), randint(0,7) while board[ship_r][ship_cl] =='X': ship_r, ship_cl = randint(0, 7), randint(0, 7) board[ship_r][ship_cl] = 'X' def count_hit_ships(board): count=0 for row in board: for column in row: if column=='X': count+=1 return count create_ships(Hidden_Pattern) #print_board(Hidden_Pattern) turns = 10 while turns > 0: print('Welcome to Battleship') print_board(Guess_Pattern) row,column =get_ship_location() if Guess_Pattern[row][column] == '-': print(' You already guessed that ') elif Hidden_Pattern[row][column] =='X': print(' Congratulations you have hit the battleship ') Guess_Pattern[row][column] = 'X' turns -= 1 else: print('Sorry,You missed') Guess_Pattern[row][column] = '-' turns -= 1 if count_hit_ships(Guess_Pattern) == 5: print("Congratulations you have sunk all the battleships ") break print(' You have ' +str(turns) + ' turns remaining ') if turns == 0: print('Game Over ') break
Output:
Output, when the ships are not sunk, lose the game

Output, when all the ships are sunk, wins the game
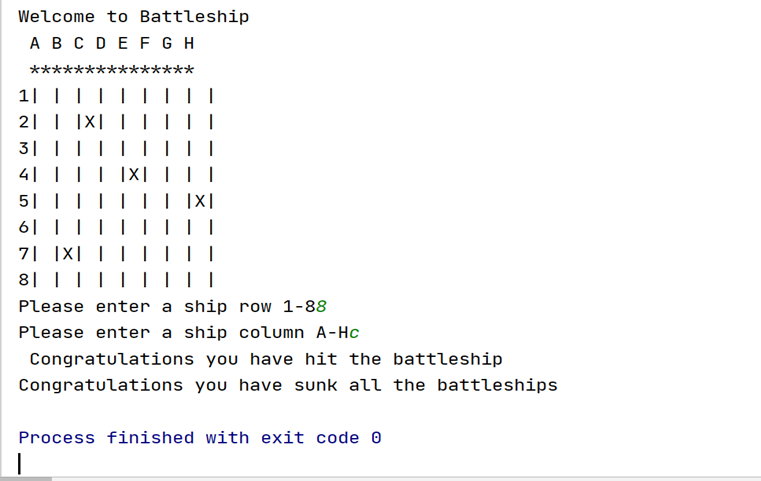
Conclusion
Hence, here we successfully completed the Battleship Game Code in Python. Everyone must try this code. Hope you find this tutorial on the python program of battleship game useful.
For more programs on python please keep visiting our website violet-cat-415996.hostingersite.com
Thank you for reading battleship game python.
Keep Learning, Keep Coding
Also Read:
- What is web development for beginners?
- What does if __name__ == __main__ do in Python?
- Python | CRUD operations in MongoDB
- CRUD operations in Django
- Install and setup Python in Windows 11
- Python Tkinter Button: Tutorial for Beginners
- Sequel Programming Languages(SQL)
- Run Python Code, Install Libraries, Create a Virtual Environment | VS Code
- Calendar using Java with best examples
- How to make a Process Monitor in Python?
- C++ Array Assignment
- Employee Management System Project in Java
- NxNxN Matrix in Python 3
- Calculator Program in Python | On Different IDEs
- Naive Bayes in Machine Learning
- Lee Algorithm in Python | Solution to Maze Routing Problem in Python
- Automate Data Mining With Python
- Support Vector Machine(SVM) in Machine Learning
- Python Increment By 1
- Vending Machine with Python Code
- Python Turtle Shapes- Square, Rectangle, Circle
- Python OOP Projects | Source code and example
- Happy Birthday In Binary Code
- AES in Python | Encrypt & Decrypt | PyCryptodome
- Make Minecraft in Python
- Battleship Game Code in Python
- Convert ipynb to Python
- Simple Atm Program in Python
- Python – Sort All Words In a File And Put It In A List – 3 Easy Method
- Python Docstring Generator | PyCharm and VsCode