
Introduction
Before learning, how to Make Minecraft in Python, let’s see what is Minecraft Game.
Minecraft is a popular video game that is enjoyed by many enthusiasts. It is a 3D block game in which players may interact with and manipulate the environment. It also has a story mode and is an adventure game.
Minecraft is a voxel-based game. And what this truly implies is that every single block in Minecraft is a voxel. So these are the things we must make ourselves. So let’s get right to work on our coding.
Step 1. Modules and libraries for Make Minecraft in Python
To make this Minecraft game in Python, we will utilize the ursina Python module. It is a Python cross-platform game creation package similar to PyGame.
To install this library, start a terminal or command prompt in the project folder and paste the following command.
pip install ursina
Step 2. importing library
Import the Ursina Engine and all the Textures that we will require in the future.
from ursina import *
from ursina.prefabs.first_person_controller import FirstPersonController
app = Ursina()
grass_texture = load_texture('assets/grass_block.png')
stone_texture = load_texture('assets/stone_block.png')
brick_texture = load_texture('assets/brick_block.png')
dirt_texture = load_texture('assets/dirt_block.png')
sky_texture = load_texture('assets/skybox.png')
arm_texture = load_texture('assets/arm_texture.png')
punch_sound = Audio('assets/punch_sound',loop = False, autoplay = False)
block_pick = 1
To load the textures and block you need to download the Assets folder and paste it where your Minecraft code Python file is stored.
Now there are a few things we need to do to make this all work, and let’s start by making actual cubes that we can utilize. This will be the most significant modification that we can do. It would appear appropriate if we applied a more appropriate texture to it. To do that we created a couple of textures.
Step 3. Making Voxel(Cubes) for Minecraft in Python
class Voxel(Button):
def __init__(self, position = (0,0,0), texture = grass_texture):
super().__init__(
parent = scene,
position = position,
model = 'assets/block',
origin_y = 0.5,
texture = texture,
color = color.color(0,0,random.uniform(0.9,1)),
scale = 0.5)
The third step is to build a new class for each voxel that we want to construct, therefore the class name will be voxel. And it derives from the button class. The basic explanation is that anytime I click on a voxel, I want to make another voxel just adjacent to it. So I need to know where each voxel is in relation to which button. And in here, we need our init method again and nothing else for the time being, and we also need the super method with an underscore to init. And now we must supply a few parameters, the first of which is parent, as shown above.
The next stage would be to find a position, and the current status is (0,0). Next, I’d want to make a model of what the object would look like. And for the time being, this one will be a cube (parameters), but we may use different forms. The next step is to pass origin_y so that the height and 3D space of this cube are effectively (0.5), and the final and most crucial item is texture and color. And both of these things multiplied.
Step 4. Making Minecraft Arena
for z in range(20):
for x in range(20):
voxel = Voxel(position = (x,0,z))
Now that we have everything, we need to create a button for it. The cube’s current positions are x, y, and z. (0,0,0). To create individual voxels, we must first create a for loop in which the voxel is set. And if you need to make it bigger, increase 35 by 35. One of the current issues with this game is that if you add too many of these fields, the game may slow down. As a result, there would be a significant amount of optimization work to be done.

Step 5. Creating First person Controller(FPC)
from ursina.prefabs.first_person_controller import FirstPersonController
player = FirstPersonController()
The question is how we can develop a first-person character in all of this. and ursina makes this super simple. Because it comes with a number of preset classes that we may utilize to construct a first-person character. We don’t really have to do anything about it. And really, all we have to do is import something else first, which is from ursina. prefabs.first_person_ controller. This is not by default imported into Oceana, so we have to do it ourselves, but once we do it, all we have to do to create FPC (First Person Character) is to create a new variable where we store and then call FPC.
Step 6. Making Interactive UI for Minecraft Python Edition
def input(self,key):
if self.hovered:
if key == 'left mouse down':
punch_sound.play()
if block_pick == 1: voxel = Voxel(position = self.position + mouse.normal, texture = grass_texture)
if block_pick == 2: voxel = Voxel(position = self.position + mouse.normal, texture = stone_texture)
if block_pick == 3: voxel = Voxel(position = self.position + mouse.normal, texture = brick_texture)
if block_pick == 4: voxel = Voxel(position = self.position + mouse.normal, texture = dirt_texture)
if key == 'right mouse down':
punch_sound.play()
destroy(self)
What we need to find out now is how to make this all more interactive. We must also create and destroy blocks, which will be really simple. Because what we want to accomplish is, when you push the voxel button, we want to construct a new block at that location. We develop an input function to do this. Make some transmissions as well. And then make a to change voxel for voxel texture
Step 7. Changing Different Textures for Coding Minecraft in Python
def update():
global block_pick
if held_keys['left mouse'] or held_keys['right mouse']:
hand.active()
else:
hand.passive()
if held_keys['1']: block_pick = 1
if held_keys['2']: block_pick = 2
if held_keys['3']: block_pick = 3
if held_keys['4']: block_pick = 4
To make Minecraft with python, we need to change the cube texture, and for changing the cube texture here we created a loop by clicking one to four keys to alter the voxel texture by Left click and destroying it with the right key. In simple words. If we press any of these buttons this block pick gets a different number.
Step 8. Creating the Sky
class Sky(Entity):
def __init__(self):
super().__init__(
parent = scene,
model = 'sphere',
texture = sky_texture,
scale = 150,
double_sided = True)
There are three other components that can significantly help to convey the game. The first is a Sky Box, the second is a Hand, and the third is noises. And let us take them one step at a time in Minecraft coding Python. The first is a skybox, which we emulated in our game by creating a huge sphere with a sky texture. To do this, we will construct a new class named Sky.
Step 9. Make an FPC Hand
class Hand(Entity):
def __init__(self):
super().__init__(
parent = camera.ui, # This 3D space for Our UI and 2D space for our camera
model = 'assets/arm',
texture = arm_texture,
scale = 0.2,
rotation = Vec3(150,-10,0), #vec3 is represent 3D space and the parameters are for position(x,y,z)
position = Vec2(0.4,-0.6)) #vec2 is represent 2D space and the parameters are for position(x,y,z)
def active(self):
self.position = Vec2(0.3,-0.5)
def passive(self):
self.position = Vec2(0.4,-0.6)
Now the next thing we will going to need is a hand and this one is purely decorative. It does not do anything besides simulating having a hand it’s a straightforward thing by creating a class called hand, this one is also going to be an entity.
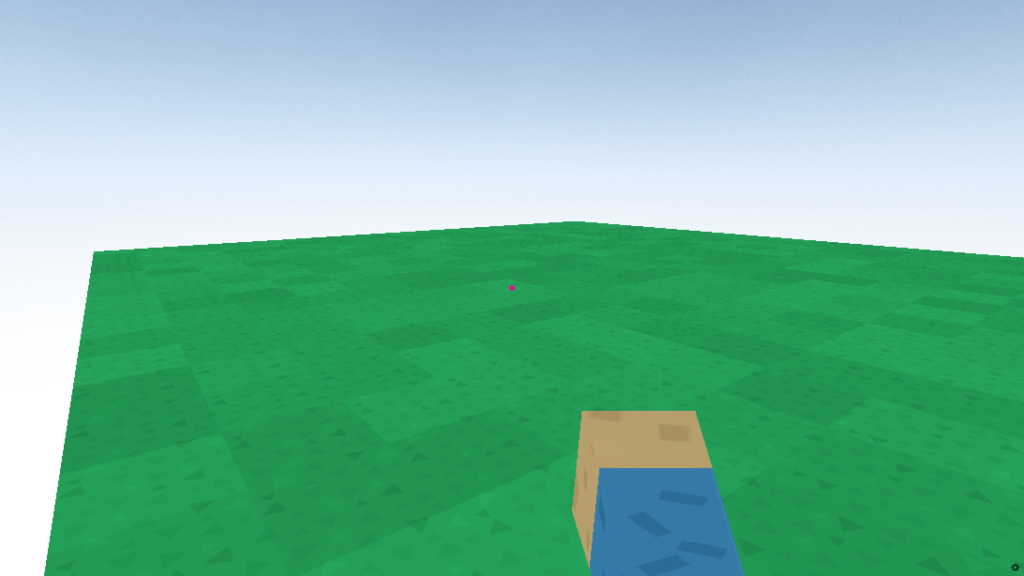
Complete code to Make Minecraft Game in Python
from ursina import * from ursina.prefabs.first_person_controller import FirstPersonController app = Ursina() grass_texture = load_texture('assets/grass_block.png') stone_texture = load_texture('assets/stone_block.png') brick_texture = load_texture('assets/brick_block.png') dirt_texture = load_texture('assets/dirt_block.png') sky_texture = load_texture('assets/skybox.png') arm_texture = load_texture('assets/arm_texture.png') punch_sound = Audio('assets/punch_sound',loop = False, autoplay = False) block_pick = 1 window.fps_counter.enabled = False #To Disable the FPS Counter window.exit_button.visible = False #To Remove the exit(close) Button def update(): global block_pick if held_keys['left mouse'] or held_keys['right mouse']: hand.active() else: hand.passive() if held_keys['1']: block_pick = 1 if held_keys['2']: block_pick = 2 if held_keys['3']: block_pick = 3 if held_keys['4']: block_pick = 4 class Voxel(Button): def __init__(self, position = (0,0,0), texture = grass_texture): super().__init__( parent = scene, position = position, model = 'assets/block', origin_y = 0.5, texture = texture, color = color.color(0,0,random.uniform(0.9,1)), scale = 0.5) def input(self,key): if self.hovered: if key == 'left mouse down': punch_sound.play() if block_pick == 1: voxel = Voxel(position = self.position + mouse.normal, texture = grass_texture) if block_pick == 2: voxel = Voxel(position = self.position + mouse.normal, texture = stone_texture) if block_pick == 3: voxel = Voxel(position = self.position + mouse.normal, texture = brick_texture) if block_pick == 4: voxel = Voxel(position = self.position + mouse.normal, texture = dirt_texture) if key == 'right mouse down': punch_sound.play() destroy(self) class Sky(Entity): def __init__(self): super().__init__( parent = scene, model = 'sphere', texture = sky_texture, scale = 150, double_sided = True) class Hand(Entity): def __init__(self): super().__init__( parent = camera.ui, model = 'assets/arm', texture = arm_texture, scale = 0.2, rotation = Vec3(150,-10,0), position = Vec2(0.4,-0.6)) def active(self): self.position = Vec2(0.3,-0.5) def passive(self): self.position = Vec2(0.4,-0.6) for z in range(20): for x in range(20): voxel = Voxel(position = (x,0,z)) player = FirstPersonController() sky = Sky() hand = Hand() app.run()
Output for Minecraft Python Coding:
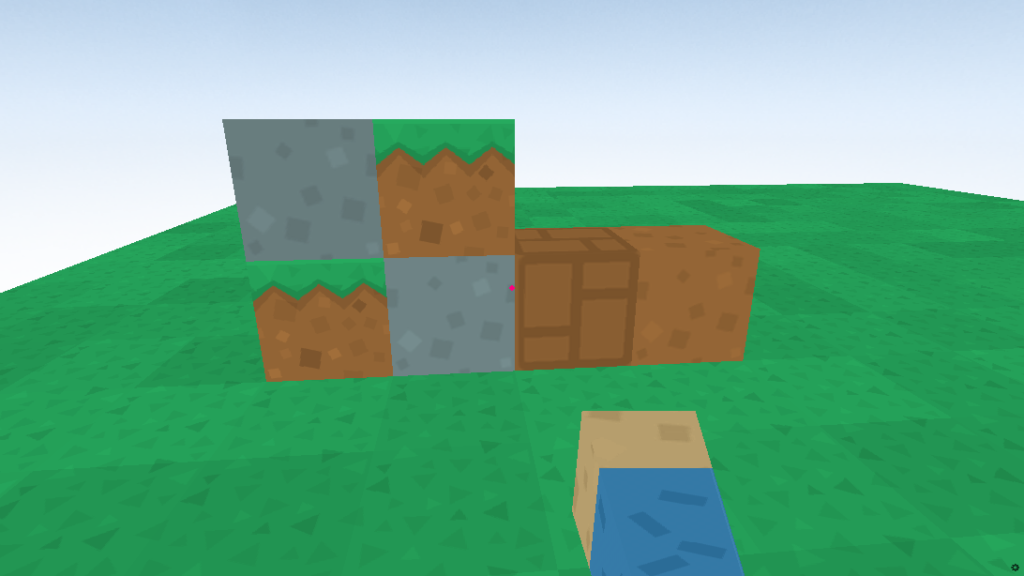
This extensive instruction on constructing a source-code-based Minecraft game in Python was offered. I hope the game went well for you. This game lacks the qualities of the original. This game may be upgraded with new features and capabilities. Be advised that this game is merely a clone and not an effort to sell the game’s source code.
We hope this article on Make Minecraft in Python will help you to create game.
Keep Learning, Keep Coding
Also Read:
- What is web development for beginners?
- What does if __name__ == __main__ do in Python?
- Python | CRUD operations in MongoDB
- CRUD operations in Django
- Install and setup Python in Windows 11
- Python Tkinter Button: Tutorial for Beginners
- Sequel Programming Languages(SQL)
- Run Python Code, Install Libraries, Create a Virtual Environment | VS Code
- Calendar using Java with best examples
- How to make a Process Monitor in Python?
- C++ Array Assignment
- Employee Management System Project in Java
- NxNxN Matrix in Python 3
- Calculator Program in Python | On Different IDEs
- Naive Bayes in Machine Learning
- Lee Algorithm in Python | Solution to Maze Routing Problem in Python
- Automate Data Mining With Python
- Support Vector Machine(SVM) in Machine Learning
- Python Increment By 1
- Vending Machine with Python Code
- Python Turtle Shapes- Square, Rectangle, Circle
- Python OOP Projects | Source code and example
- Happy Birthday In Binary Code
- AES in Python | Encrypt & Decrypt | PyCryptodome
- Make Minecraft in Python
- Battleship Game Code in Python
- Convert ipynb to Python
- Simple Atm Program in Python
- Python – Sort All Words In a File And Put It In A List – 3 Easy Method
- Python Docstring Generator | PyCharm and VsCode